利用vs pcl库将多个PCD文件合并成一张PCD地图
主机环境:win10系统,pcl库1.11.1,
vs2019 pcl库安装以及环境配置如下连接:
https://www.jb51.net/article/190710.htm
代码很简单,主要是做个坐标转换,如下:
#include <fstream>
#include <string>
#include <vector>
#include <iostream>
#include <iostream> //标准输入输出流
#include <pcl/io/pcd_io.h> //PCL的PCD格式文件的输入输出头文件
#include <pcl/point_types.h> //PCL对各种格式的点的支持头文件
#include <pcl/visualization/pcl_visualizer.h>
#include <pcl/common/transforms.h>
#include <pcl/visualization/cloud_viewer.h>
void GetAllFiles(string path, vector<string>& files)
{
long hFile = 0;
//文件信息
struct _finddata_t fileinfo;
string p;
if ((hFile = _findfirst(p.assign(path).append("\\*").c_str(), &fileinfo)) != -1)
{
do
{
if ((fileinfo.attrib & _A_SUBDIR))
{
if (strcmp(fileinfo.name, ".") != 0 && strcmp(fileinfo.name, "..") != 0)
{
files.push_back(p.assign(path).append("\\").append(fileinfo.name));
}
}
else
{
files.push_back(p.assign(path).append("\\").append(fileinfo.name));
}
} while (_findnext(hFile, &fileinfo) == 0);
_findclose(hFile);
}
}
void GetAllFormatFiles(string path, vector<string>& files, string format)
{
//文件句柄
long long hFile = 0;
//文件信息
struct _finddata_t fileinfo;
string p;
if ((hFile = _findfirst(p.assign(path).append("\\*" + format).c_str(), &fileinfo)) != -1)
{
do
{
if ((fileinfo.attrib & _A_SUBDIR))
{
if (strcmp(fileinfo.name, ".") != 0 && strcmp(fileinfo.name, "..") != 0)
{
//files.push_back(p.assign(path).append("\\").append(fileinfo.name) );
GetAllFormatFiles(p.assign(path).append("\\").append(fileinfo.name), files, format);
}
}
else
{
files.push_back(p.assign(path).append("\\").append(fileinfo.name));
}
} while (_findnext(hFile, &fileinfo) == 0);
_findclose(hFile);
}
}
{
string filePath = "C:\\Users\\CTOS\\Desktop\\newpcd";
vector<string> files;
const char* distAll = "*.pcd";
string format = ".pcd";
GetAllFormatFiles(filePath, files, format);
ofstream ofn(distAll);
int size = files.size();
cout << size << endl;
ofn << size << endl;
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>); // 创建点云(指针)
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_prt(new pcl::PointCloud<pcl::PointXYZ>); // 创建点云(指针)
pcl::PointCloud<pcl::PointXYZ>::Ptr transformed_cloud(new pcl::PointCloud<pcl::PointXYZ>); // 创建点云(指针)
for (int i = 0; i < size; i++)
{
ofn << files[i] << endl;
cout << files[i] << endl;
if (pcl::io::loadPCDFile<pcl::PointXYZ>(files[i], *cloud_prt) == -1) //* 读入PCD格式的文件,如果文件不存在,返回-1
{
cout << "Couldn't read file pcd \n"<< endl; //文件不存在时,返回错误,终止程序。
return (-1);
}
Eigen::Vector4f p = cloud_prt->sensor_origin_.matrix();
Eigen::Quaternionf q;
q = cloud_prt->sensor_orientation_.matrix();
Eigen::Vector3f eulerAngle = q.matrix().eulerAngles(2, 1, 0);
Eigen::Affine3f transform = Eigen::Affine3f::Identity();
transform.translation() << p[0], p[1], p[2];
transform.rotate(q);
std::cout << transform.matrix() << std::endl;
pcl::transformPointCloud(*cloud_prt, *transformed_cloud, transform);
*cloud += *transformed_cloud;
cloud_prt->clear();
transformed_cloud->clear();
Sleep(1);
}
pcl::visualization::CloudViewer viewer("pcd viewer");
viewer.showCloud(cloud);
while (!viewer.wasStopped())
{
}
ofn.close();
return 0;
}
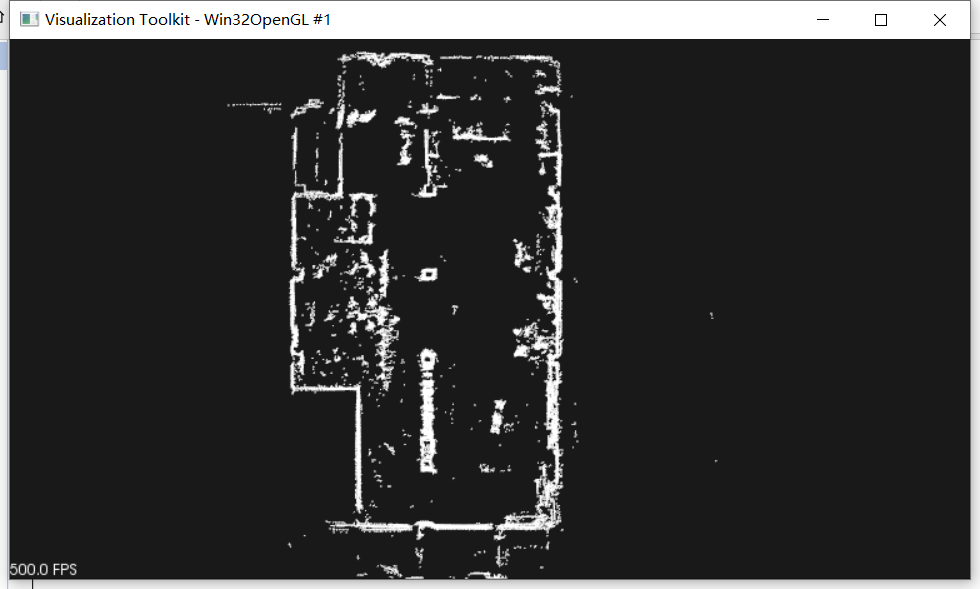
利用vs pcl库将多个PCD文件合并成一张PCD地图的更多相关文章
- PCL点云库中怎样读取指定的PCD文件,又一次命名,处理后保存到指定目录
我一直想把处理后的pcd文件重命名,然后放到指定的目录,尝试了好久最终做到了: 比方我想读取 "table_scene_lms400.pcd" 把它进行滤波处理,重命名为 &qu ...
- PCD文件格式详解及在PCL下读取PCD文件
一.PCD简介 1.1 PCD版本 在点云库PCL 1.0发布之前,PCD文件格式就已经发展更新了许多版本.这些新旧不同的版本用PCD_Vx来编号(例如PCD_V5.PCD_V6和PCD_V7等),分 ...
- PCL Save VTK File With Texture Coordinates 使用PCL库来保存带纹理坐标的VTK文件
我之前有一篇博客Convert PLY to VTK Using PCL 1.6.0 or PCL 1.8.0 使用PCL库将PLY格式转为VTK格式展示了如何将PLY格式文件转化为VTK格式的文件, ...
- 利用私有的库MobileCoreServices检测正在安装的应用
利用的私有库检测正在安装的app 分为两步:第一,通过placeholderApplications获得所有的正在安装的app的信息 第二,遍历正在安装的app的信息,根据名称获得你想检测的app是否 ...
- Convert PLY to VTK Using PCL 1.6.0 使用PCL库将PLY格式转为VTK格式
PLY格式是比较流行的保存点云Point Cloud的格式,可以用MeshLab等软件打开,而VTK是医学图像处理中比较常用的格式,可以使用VTK库和ITK库进行更加复杂的运算处理.我们可以使用Par ...
- c# 利用动态库DllImport("kernel32")读写ini文件(提供Dmo下载)
c# 利用动态库DllImport("kernel32")读写ini文件 自从读了设计模式,真的会改变一个程序员的习惯.我觉得嘛,经验也可以从一个人的习惯看得出来,看他的代码编写习 ...
- PCL 库安装
参考资料: http://www.cnblogs.com/newpanderking/articles/4022322.html VS2010+PCL配置 PCL共有两种安装方式 安全安装版,个人配置 ...
- Window7下手动编译最新版的PCL库
PCL简介 PCL是Point Cloud Library的缩写,是一个用于处理二维图像,三维深度图像和三维点云的C++库.该库是完全开源的,可免费用于商业和学术研究. 官方网站:http://poi ...
- Ubuntu 16.04 安装PCL库以及测试
参考链接:https://blog.csdn.net/dantengc/article/details/78446600 参考博客,官网一直安装不成功,后来参照一篇博客终于安装成功了,记录如下. 1. ...
随机推荐
- property内置装饰器函数和@name.setter、@name.deleter
# property # 内置装饰器函数 只在面向对象中使用 # 装饰后效果:将类的方法伪装成属性 # 被property装饰后的方法,不能带除了self外的任何参数 from math import ...
- 算法题目:北邮python 3-C 排队前进
一道python作业的题目,比较有意思,题目如下: 题目描述 有 n 个人排队向一个方向前进,他们前进的速度并不一定相同. 最开始即 t=0 时,每个人的位置并不相同.可以把他们放在数轴上,设他们前进 ...
- 微信公众号获取openid(php实例)
微信公众号获取openid 公众号获取openid的方法跟小程序获取openid其实是一样的,只是code获取的方式不一样 小程序获取code: 用户授权登录时调用wx.login即可获取到code ...
- zabbix的搭建及操作(1)server-client架构
实验环境 Server端 Centos7:192.168.10.10 server.zabbix.com 可连外网 Agent 端 Centos7:192.168.10.20 ...
- jmeter压测mysql数据库
jmeter连接并压测mysql数据库,之前一直想用jmeter一下测试mysql数据库的性能,今天偶然看到一篇博客,于是乎开始自己动手实践. 一.准备工作 1.安装好mysql数据库,可以安装在本地 ...
- 分析 5种分布式事务方案,还是选了阿里的 Seata(原理 + 实战)
好长时间没发文了,最近着实是有点忙,当爹的第 43 天,身心疲惫.这又赶上年底,公司冲 KPI 强制技术部加班到十点,晚上孩子隔两三个小时一醒,基本没睡囫囵觉的机会,天天处于迷糊的状态,孩子还时不时起 ...
- mybatis 动态SQL 源码解析
摘要 mybatis是个人最新喜欢的半自动ORM框架,它实现了SQL和业务逻辑的完美分割,今天我们来讨论一个问题,mybatis 是如何动态生成SQL SqlSessionManager sqlSes ...
- 创建topic
sh kafka-topics.sh --create --zookeeper localhost:2181 --replication-factor 1 --partitions 1 --topic ...
- C# 9.0新特性详解系列之三:模块初始化器
1 背景动机 关于模块或者程序集初始化工作一直是C#的一个痛点,微软内部外部都有大量的报告反应很多客户一直被这个问题困扰,这还不算没有统计上的客户.那么解决这个问题,还有基于什么样的考虑呢? 在库加载 ...
- 2020年的UWP(4)——UWP和等待Request的Desktop Extension
上一篇我们讨论了UWP和Desktop Extension交互中,Desktop Extension执行后立即退出的场景.下图是提到的四种场景分类: 执行后立即退出 等待request,处理完后退出 ...