POJ 1195:Mobile phones 二维树状数组
Time Limit: 5000MS | Memory Limit: 65536K | |
Total Submissions: 16893 | Accepted: 7789 |
Description
number of active mobile phones inside a square can change because a phone is moved from a square to another or a phone is switched on or off. At times, each base station reports the change in the number of active phones to the main base station along with
the row and the column of the matrix.
Write a program, which receives these reports and answers queries about the current total number of active mobile phones in any rectangle-shaped area.
Input
integers according to the following table.
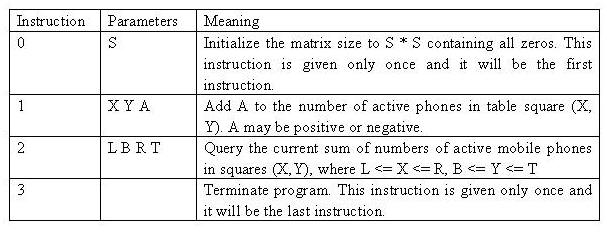
The values will always be in range, so there is no need to check them. In particular, if A is negative, it can be assumed that it will not reduce the square value below zero. The indexing starts at 0, e.g. for a table of size 4 * 4, we have 0 <= X <= 3 and
0 <= Y <= 3.
Table size: 1 * 1 <= S * S <= 1024 * 1024
Cell value V at any time: 0 <= V <= 32767
Update amount: -32768 <= A <= 32767
No of instructions in input: 3 <= U <= 60002
Maximum number of phones in the whole table: M= 2^30
Output
Sample Input
- 0 4
- 1 1 2 3
- 2 0 0 2 2
- 1 1 1 2
- 1 1 2 -1
- 2 1 1 2 3
- 3
Sample Output
- 3
- 4
在一个矩阵中动态改动数字,然后不断询问某一个子矩阵的和。
二维树状数组,和一维的思想一样。
代码:
- #include <iostream>
- #include <algorithm>
- #include <cmath>
- #include <vector>
- #include <string>
- #include <cstring>
- #pragma warning(disable:4996)
- using namespace std;
- #define MY_MAX 1100
- int C[MY_MAX][MY_MAX];
- int s;
- int lowbit(int x)
- {
- return x&(-x);
- }
- void Add(int y,int x,int a)
- {
- while(y<=s)
- {
- int temp=x;
- while(temp<=s)
- {
- C[y][temp]+=a;
- temp +=lowbit(temp);
- }
- y +=lowbit(y);
- }
- }
- int QuerySum(int y,int x)
- {
- int nSum=0;
- while(y>0)
- {
- int temp=x;
- while(temp>0)
- {
- nSum += C[y][temp];
- temp -= lowbit(temp);
- }
- y -=lowbit(y);
- }
- return nSum;
- }
- int main()
- {
- //freopen("i.txt","r",stdin);
- //freopen("o.txt","w",stdout);
- int oper;
- int x,y,a,le,b,r,t;
- while(true)
- {
- scanf("%d",&oper);
- if(oper==0)
- {
- scanf("%d",&s);
- memset(C,0,sizeof(C));
- }
- else if(oper==1)
- {
- scanf("%d%d%d",&x,&y,&a);
- Add(x+1,y+1,a);
- }
- else if(oper==2)
- {
- scanf("%d%d%d%d",&le,&b,&r,&t);
- printf("%d\n",QuerySum(r+1,t+1) + QuerySum(le,b)- QuerySum(le,t+1) - QuerySum(r+1,b));
- }
- else if(oper==3)
- {
- break;
- }
- }
- //system("pause");
- return 0;
- }
版权声明:本文为博主原创文章,未经博主允许不得转载。
POJ 1195:Mobile phones 二维树状数组的更多相关文章
- poj 1195 Mobile phones(二维树状数组)
树状数组支持两种操作: Add(x, d)操作: 让a[x]增加d. Query(L,R): 计算 a[L]+a[L+1]……a[R]. 当要频繁的对数组元素进行修改,同时又要频繁的查询数组内任一 ...
- 【poj1195】Mobile phones(二维树状数组)
题目链接:http://poj.org/problem?id=1195 [题意] 给出一个全0的矩阵,然后一些操作 0 S:初始化矩阵,维数是S*S,值全为0,这个操作只有最开始出现一次 1 X Y ...
- POJ 2155 Matrix【二维树状数组+YY(区间计数)】
题目链接:http://poj.org/problem?id=2155 Matrix Time Limit: 3000MS Memory Limit: 65536K Total Submissio ...
- POJ 2155 Matrix(二维树状数组+区间更新单点求和)
题意:给你一个n*n的全0矩阵,每次有两个操作: C x1 y1 x2 y2:将(x1,y1)到(x2,y2)的矩阵全部值求反 Q x y:求出(x,y)位置的值 树状数组标准是求单点更新区间求和,但 ...
- POJ 2155 Matrix (二维树状数组)
Matrix Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 17224 Accepted: 6460 Descripti ...
- POJ 2155 Matrix 【二维树状数组】(二维单点查询经典题)
<题目链接> 题目大意: 给出一个初始值全为0的矩阵,对其进行两个操作. 1.给出一个子矩阵的左上角和右上角坐标,这两个坐标所代表的矩阵内0变成1,1变成0. 2.查询某个坐标的点的值. ...
- POJ 2155 Matrix (二维树状数组)题解
思路: 没想到二维树状数组和一维的比只差了一行,update单点更新,query求和 这里的函数用法和平时不一样,query直接算出来就是某点的值,怎么做到的呢? 我们在更新的时候不止更新一个点,而是 ...
- POJ 2155:Matrix 二维树状数组
Matrix Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 21757 Accepted: 8141 Descripti ...
- POJ 2155 Matrix(二维树状数组)
与以往不同的是,这个树状数组是二维的,仅此而已 #include <iostream> #include <cstdio> #include <cstring> # ...
随机推荐
- 「Luogu P3680 凸轮廓线」
一道神奇的计算几何题 前置芝士 正三角形,正方形,圆:什么,您都会,那真是太好了. 三角函数的运用:因为我不是很想在这一块写太多,具体可以自行百度. 推导公式 对于一串是圆和正方形开头和结尾时是十分好 ...
- redhat 7.6 VI编辑操作
模式一: 浏览模式 0 : 光标到行首 $ : 光标到行尾 gg:光标到首行 G:光标到尾行 yy:复制光标所在行 dd:剪切光标所在行,删除行 y11y:复制光标所在行,往下数,一共10行 p:粘贴 ...
- 「NOI2005」维护数列
「NOI2005」维护数列 传送门 维护过程有点像线段树. 但我们知道线段树的节点并不是实际节点,而平衡树的节点是实际节点. 所以在向上合并信息时要加入根节点信息. 然后节点再删除后编号要回退(栈), ...
- SpringMVC:自定义视图及其执行过程
一:自定义视图 1.自定义一个实现View接口的类,添加@Component注解,将其放入SpringIOC容器 package com.zzj.view; import java.io.PrintW ...
- 详述ThreadLocal
ThreadLocal的作用和目的:用于实现线程内的数据共享,即对于相同的程序代码,多个模块在同一个线程中运行时要共享一份数据,而在另外线程中运行时又共享另外一份数据. 举一个反面例子,当我们使用简单 ...
- (C#)Image.FromFile 方法会锁住文件的原因及可能的解决方法
Image.FromFile 一旦使用后,对应的文件在一直调用其生成的Image对象被Disponse前都不会被解除锁定,这就造成了一个问题,就是在这个图形被解锁前无法对图像进行操作(比如删除,修改等 ...
- ch8 高度相等的列--CSS方法
如下图所示效果,可以使用表格实现,本文采用在CSS中实现. 标记如下: <div class="wrapper"> <div class="box&qu ...
- Tomcat+JSP经典配置实例
经常看到jsp的初学者问tomcat下如何配置jsp.servlet和bean的问题,于是总结了一下如何tomcat下配置jsp.servlet和ben,希望对那些初学者有所帮助. 一.开发环境配置 ...
- windos常见软件库
1.护卫神软件库 http://soft.huweishen.com/special/1.html 2.护卫神windows资料库 http://v.huweishen.com/ 3.国超软件下载 h ...
- SpringBoo-Thymeleaf
SpringBoo-Thymeleaf SpringBoo-Thymeleaf简介 SpringBoot并不推荐使用JSP,它推荐我们使用模板引擎Thymeleaf,它与Velocity.Free ...