Poj(3522),UVa(1395),枚举生成树
题目链接:http://poj.org/problem?id=3522
Time Limit: 5000MS | Memory Limit: 65536K | |
Total Submissions: 7522 | Accepted: 3988 |
Description
Given an undirected weighted graph G, you should find one of spanning trees specified as follows.
The graph G is an ordered pair (V, E), where V is a set of vertices {v1, v2, …, vn} and E is a set of undirected edges {e1, e2, …, em}. Each edge e ∈ E has its weight w(e).
A spanning tree T is a tree (a connected subgraph without cycles) which connects all the n vertices with n − 1 edges. The slimness of a spanning tree T is defined as the difference between the largest weight and the smallest weight among the n − 1 edges of T.
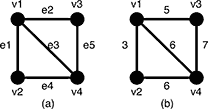
Figure 5: A graph G and the weights of the edges
For example, a graph G in Figure 5(a) has four vertices {v1, v2, v3, v4} and five undirected edges {e1, e2, e3, e4, e5}. The weights of the edges are w(e1) = 3, w(e2) = 5, w(e3) = 6, w(e4) = 6, w(e5) = 7 as shown in Figure 5(b).
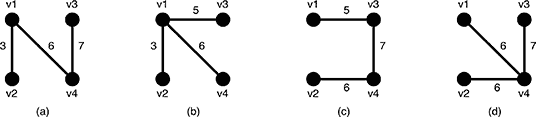
Figure 6: Examples of the spanning trees of G
There are several spanning trees for G. Four of them are depicted in Figure 6(a)~(d). The spanning tree Ta
in Figure 6(a) has three edges whose weights are 3, 6 and 7. The
largest weight is 7 and the smallest weight is 3 so that the slimness of
the tree Ta is 4. The slimnesses of spanning trees Tb, Tc and Td
shown in Figure 6(b), (c) and (d) are 3, 2 and 1, respectively. You can
easily see the slimness of any other spanning tree is greater than or
equal to 1, thus the spanning tree Td in Figure 6(d) is one of the
slimmest spanning trees whose slimness is 1.
Your job is to write a program that computes the smallest slimness.
Input
The
input consists of multiple datasets, followed by a line containing two
zeros separated by a space. Each dataset has the following format.
n | m | |
a1 | b1 | w1 |
⋮ | ||
am | bm | wm |
Every
input item in a dataset is a non-negative integer. Items in a line are
separated by a space. n is the number of the vertices and m the number
of the edges. You can assume 2 ≤ n ≤ 100 and 0 ≤ m ≤ n(n − 1)/2. ak and bk (k = 1, …, m) are positive integers less than or equal to n, which represent the two vertices vak and vbk connected by the kth edge ek. wk is a positive integer less than or equal to 10000, which indicates the weight of ek. You can assume that the graph G = (V, E)
is simple, that is, there are no self-loops (that connect the same
vertex) nor parallel edges (that are two or more edges whose both ends
are the same two vertices).
Output
For
each dataset, if the graph has spanning trees, the smallest slimness
among them should be printed. Otherwise, −1 should be printed. An output
should not contain extra characters.
Sample Input
4 5
1 2 3
1 3 5
1 4 6
2 4 6
3 4 7
4 6
1 2 10
1 3 100
1 4 90
2 3 20
2 4 80
3 4 40
2 1
1 2 1
3 0
3 1
1 2 1
3 3
1 2 2
2 3 5
1 3 6
5 10
1 2 110
1 3 120
1 4 130
1 5 120
2 3 110
2 4 120
2 5 130
3 4 120
3 5 110
4 5 120
5 10
1 2 9384
1 3 887
1 4 2778
1 5 6916
2 3 7794
2 4 8336
2 5 5387
3 4 493
3 5 6650
4 5 1422
5 8
1 2 1
2 3 100
3 4 100
4 5 100
1 5 50
2 5 50
3 5 50
4 1 150
0 0
Sample Output
1
20
0
-1
-1
1
0
1686
50
Source
#include <stdio.h>
#include <algorithm> using namespace std; #define MAXN 6000
#define INF 0x3f3f3f3f struct Edge
{
int u,v;
int w;
} edge[MAXN]; int father[MAXN]; int Find_Set (int x)
{
if(x!=father[x])
father[x] = Find_Set(father[x]);
return father[x];
} int n,m;
bool cmp(Edge a,Edge b)
{
return a.w<b.w;
} int main()
{
//freopen("input.txt","r",stdin);
while(scanf("%d%d",&n,&m),n)
{
bool flag = false; for(int i=; i<m; i++)
scanf("%d%d%d",&edge[i].u,&edge[i].v,&edge[i].w);
sort(edge,edge+m,cmp);
//for(int i=0; i<m; i++)
//printf("%d ",edge[i].w);
// puts(""); int ans = INF;
int i,j;
for(i=; i<m; i++)
{
for(int i=; i<=n; i++)
father[i] = i;
int cnt = ;
for(j=i; j<m; j++)
{
int fx = Find_Set(edge[j].u);
int fy = Find_Set(edge[j].v);
if(fx==fy)
continue; father[fy] = fx;
cnt++;
if(cnt==n-)
{
flag = true;
break;
}
}
if(cnt==n-)
ans = min(ans,edge[j].w-edge[i].w);
}
if(flag)
printf("%d\n",ans);
else puts("-1");
}
return ;
}
Poj(3522),UVa(1395),枚举生成树的更多相关文章
- POJ 3522 最小差值生成树(LCT)
题目大意:给出一个n个节点的图,求最大边权值减去最小边权值最小的生成树. 题解 Flash Hu大佬一如既往地强 先把边从小到大排序 然后依次加入每一条边 如果已经连通就把路径上权值最小的边删去 然后 ...
- POJ 3525/UVA 1396 Most Distant Point from the Sea(二分+半平面交)
Description The main land of Japan called Honshu is an island surrounded by the sea. In such an isla ...
- POJ 3522 Slim Span 最小差值生成树
Slim Span Time Limit: 20 Sec Memory Limit: 256 MB 题目连接 http://poj.org/problem?id=3522 Description Gi ...
- POJ 3522 Slim Span 暴力枚举 + 并查集
http://poj.org/problem?id=3522 一开始做这个题的时候,以为复杂度最多是O(m)左右,然后一直不会.最后居然用了一个近似O(m^2)的62ms过了. 一开始想到排序,然后扫 ...
- UVa 1395 苗条的生成树(Kruskal+并查集)
https://vjudge.net/problem/UVA-1395 题意: 给出一个n结点的图,求苗条度(最大边减最小边的值)尽量小的生成树. 思路: 主要还是克鲁斯卡尔算法,先仍是按权值排序,对 ...
- POJ 3522 Slim Span (Kruskal枚举最小边)
题意: 求出最小生成树中最大边与最小边差距的最小值. 分析: 排序,枚举最小边, 用最小边构造最小生成树, 没法构造了就退出 #include <stdio.h> #include < ...
- 紫书 例题 11-2 UVa 1395(最大边减最小边最小的生成树)
思路:枚举所有可能的情况. 枚举最小边, 然后不断加边, 直到联通后, 这个时候有一个生成树.这个时候,在目前这个最小边的情况可以不往后枚举了, 可以直接更新答案后break. 因为题目求最大边减最小 ...
- POJ 1873 UVA 811 The Fortified Forest (凸包 + 状态压缩枚举)
题目链接:UVA 811 Description Once upon a time, in a faraway land, there lived a king. This king owned a ...
- Poj 3522 最长边与最短边差值最小的生成树
题意: 让你求一颗生成树,使得最长边和最短边长度差值最小. 思路: 额!!!感觉这个思路会超时,但是ac了,暂时没什么别的好思路,那么就先说下这个思路,大牛要是有好的思路希望能在 ...
随机推荐
- ssh两台机器建立信任关系无密码登陆
在建立信任关系之前先看看基于公钥.私钥的加密和认证. 私钥签名过程 消息-->[私钥]-->签名-->[公钥]-->认证 私钥数字签名,公钥验证 Alice生成公钥和私钥,并将 ...
- 转:Busy Developers' Guide to HSSF and XSSF Features
Busy Developers' Guide to Features Want to use HSSF and XSSF read and write spreadsheets in a hurry? ...
- [原创]java WEB学习笔记89:Hibernate学习之路-- -Hibernate检索方式(5种),HQL介绍,实现功能,实现步骤,
本博客的目的:①总结自己的学习过程,相当于学习笔记 ②将自己的经验分享给大家,相互学习,互相交流,不可商用 内容难免出现问题,欢迎指正,交流,探讨,可以留言,也可以通过以下方式联系. 本人互联网技术爱 ...
- Python学习总结11:获取当前运行类名和函数名
一. 使用内置方法和修饰器方法获取类名.函数名 1. 外部获取 从外部的情况好获取,可以使用指向函数的对象,然后用__name__属性. def a(): pass a.__name__ 或者 get ...
- linux第12天 线程
今天主要学习了共享内存,信号量的封装,还有线程. POSIX线程库 与线程有关的函数构成了一个完整的系列,绝大多数函数的名字都是以“pthread_”打头的 要使用这些函数库,要通过引入头文<p ...
- URAL 1018 Binary Apple Tree(树DP)
Let's imagine how apple tree looks in binary computer world. You're right, it looks just like a bina ...
- bzoj4137 [FJOI2015]火星商店问题
比较容易想到的做法是线段树套字典树,修改操作时在字典树上经过的节点维护一个最近被访问过的时间,这样询问操作只经过满足时间条件的节点,时间复杂度O(NlogN^2)但是因为线段树每个节点都要套个字典树, ...
- UVALive 7148 LRIP【树分治+线段树】
题意就是要求一棵树上的最长不下降序列,同时不下降序列的最小值与最大值不超过D. 做法是树分治+线段树,假设树根是x,y是其当前需要处理的子树,对于子树y,需要处理出两个数组MN,MX,MN[i]表示以 ...
- CCF真题之相邻数对
201409-1 问题描述 给定n个不同的整数,问这些数中有多少对整数,它们的值正好相差1. 输入格式 输入的第一行包含一个整数n,表示给定整数的个数. 第二行包含所给定的n个整数. 输出格式 输出一 ...
- AS-demo09
,mainifast: <uses-permission android:name="android.permission.SET_WALLPAPER"/> , < ...