poj 1915 KnightMoves(bfs)
Time Limit: 1000MS | Memory Limit: 30000K | |
Total Submissions: 24094 | Accepted: 11364 |
Description
Mr Somurolov, fabulous chess-gamer indeed, asserts that no one else but him can move knights from one position to another so fast. Can you beat him?
The Problem
Your task is to write a program to calculate the minimum number of moves needed for a knight to reach one point from another, so that you have the chance to be faster than Somurolov.
For people not familiar with chess, the possible knight moves are shown in Figure 1.
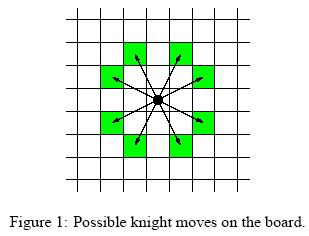
Input
Next follow n scenarios. Each scenario consists of three lines containing integer numbers. The first line specifies the length l of a side of the chess board (4 <= l <= 300). The entire board has size l * l. The second and third line contain pair of integers {0, ..., l-1}*{0, ..., l-1} specifying the starting and ending position of the knight on the board. The integers are separated by a single blank. You can assume that the positions are valid positions on the chess board of that scenario.
Output
Sample Input
3
8
0 0
7 0
100
0 0
30 50
10
1 1
1 1
Sample Output
5
28
0
分析:简单BFS,分8个方向遍历。
Java AC 代码
import java.util.LinkedList;
import java.util.Queue;
import java.util.Scanner; public class Main { static int chessLength; static int dx[] = {-2, -2, -1, -1, 1, 1, 2, 2};
static int dy[] = {1, -1, 2, -2, 2, -2, 1, -1}; static Point original, goal; static Queue<Point> queue; static boolean marked[][]; static int steps[][]; public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int testNumber = sc.nextInt(); for(int i = 1; i <= testNumber; i++) {
chessLength = sc.nextInt();
original = new Point(sc.nextInt(), sc.nextInt());
goal = new Point(sc.nextInt(), sc.nextInt());
marked = new boolean[chessLength][chessLength];
steps = new int[chessLength][chessLength];
queue = new LinkedList<Point> ();
bfs();
System.out.println(steps[goal.row][goal.col]);
}
} public static void bfs() {
queue.add(original);
marked[original.row][original.col] = true;
steps[original.row][original.col] = 0; while(!queue.isEmpty()) {
Point head = queue.poll();
for(int i = 0; i < 8; i++) {
int _row = head.row + dy[i];
int _col = head.col + dx[i];
if(_row >= 0 && _row < chessLength && _col >= 0 && _col < chessLength && !marked[_row][_col]) {
queue.add(new Point(_row, _col));
marked[_row][_col] = true;
steps[_row][_col] = steps[head.row][head.col] + 1;
}
}
if(marked[goal.row][goal.col])
return;
}
}
} class Point {
int row;
int col;
public Point(int row, int col) {
this.row = row;
this.col = col;
}
}
poj 1915 KnightMoves(bfs)的更多相关文章
- POJ 1915 Knight Moves
POJ 1915 Knight Moves Knight Moves Time Limit: 1000MS Memory Limit: 30000K Total Submissions: 29 ...
- POJ 1915 Knight Moves(BFS+STL)
Knight Moves Time Limit: 1000MS Memory Limit: 30000K Total Submissions: 20913 Accepted: 9702 ...
- POJ 1915 经典马步 双向bfs
拿这个经典题目开刀...........可是双向时间优势在这题上的效果不太明显 #include <iostream> #include <algorithm> #includ ...
- POJ 3026(BFS+prim)
http://poj.org/problem?id=3026 题意:任意两个字母可以连线,求把所有字母串联起来和最小. 很明显这就是一个最小生成树,不过这个题有毒.他的输入有问题.在输入m和N后面,可 ...
- OpenJudge/Poj 1915 Knight Moves
1.链接地址: http://bailian.openjudge.cn/practice/1915 http://poj.org/problem?id=1915 2.题目: 总Time Limit: ...
- hdu 1043 pku poj 1077 Eight (BFS + 康拓展开)
http://acm.hdu.edu.cn/showproblem.php?pid=1043 http://poj.org/problem?id=1077 Eight Time Limit: 1000 ...
- POJ 3414 Pots bfs打印方案
题目: http://poj.org/problem?id=3414 很好玩的一个题.关键是又16ms 1A了,没有debug的日子才是好日子.. #include <stdio.h> # ...
- [POJ] 1606 Jugs(BFS+路径输出)
题目地址:http://poj.org/problem?id=1606 广度优先搜索的经典问题,倒水问题.算法不需要多说,直接BFS,路径输出采用递归.最后注意是Special Judge #incl ...
- POJ:2049Finding Nemo(bfs+优先队列)
http://poj.org/problem?id=2049 Description Nemo is a naughty boy. One day he went into the deep sea ...
随机推荐
- charts_03
table 数值获取: 1.http://www.w3school.com.cn/jsref/dom_obj_all.asp 2.http://blog.csdn.net/xs_zgsc/articl ...
- [Java]将算术表达式(中序表达式Infix)转成后续表达式Postfix
Inlet类: package com.hy; import java.io.BufferedReader; import java.io.IOException; import java.io.In ...
- python自然语言处理学习笔记2
基础语法 搜索文本----词语索引使我们看到词的上下 text1.concordance("monstrous") 词出现在相似的上下文中 text1.similar(" ...
- 浏览器端-W3School-JavaScript-HTML DOM:HTML DOM Element 对象
ylbtech-浏览器端-W3School-JavaScript-HTML DOM:HTML DOM Element 对象 1.返回顶部 1. HTML DOM Element 对象 HTML DOM ...
- c#协变 抗变
public class Fa : TranOut<Fa>, TranIn<Fa> { } public class son : Fa, TranOut<son>, ...
- json -- fastjson如何序列化@Transient的字段
今天把fastjson包改成了1.2.58,发现@Transient标注的字段序列化后不见了,但是项目需要把@Transient字段序列化,处理方法: 原文:https://github.com/al ...
- apache禁止指定的user_agent访问
user_agent:也就是浏览器标识#禁止指定user_agent <IfModule mod_rewrite.c> RewriteEngine on RewriteCond %{HTT ...
- Idea创建项目后,提交项目到码云的问题
1.创建git仓库:vcs->import int version control->Create git repository 2.在项目头点击右键->git->add 3. ...
- [转] 浅谈JS中的变量及作用域
Situation One <script> var i; function sayHello() { var x=100; alert(x); x++; } sayHello(); ...
- vc/vs常见报错:/****error C2106: '=' : left operand must be l-value****/
一.错误信息解析: 1.error,表示这是一条出错信息. C语言信息一般有error(出错)和warning(警告)两种. error是编译器遇到了致命错误,无法继续进行编译,必须修改. warni ...