poj1178 floyd+枚举
http://poj.org/problem?id=1178
Description
at random on distinct squares.
The Board is an 8x8 array of squares. The King can move to any adjacent square, as shown in Figure 2, as long as it does not fall off the board. A Knight can jump as shown in Figure 3, as long as it does not fall off the board.
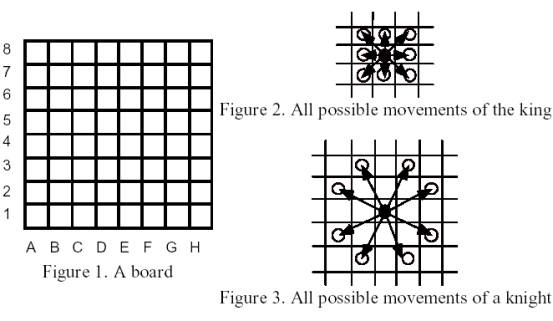
During the play, the player can place more than one piece in the same square. The board squares are assumed big enough so that a piece is never an obstacle for other piece to move freely.
The player's goal is to move the pieces so as to gather them all in the same square, in the smallest possible number of moves. To achieve this, he must move the pieces as prescribed above. Additionally, whenever the king and one or more knights are placed in
the same square, the player may choose to move the king and one of the knights together henceforth, as a single knight, up to the final gathering point. Moving the knight together with the king counts as a single move.
Write a program to compute the minimum number of moves the player must perform to produce the gathering.
Input
ones those of the knights. Each position is a letter-digit pair. The letter indicates the horizontal board coordinate, the digit indicates the vertical board coordinate.
0 <= number of knights <= 63
Output
Sample Input
D4A3A8H1H8
Sample Output
10
/**
poj 1178 floyd+枚举
题目大意:在一个8*8的棋盘里有一个国王和一些骑士,我们须要把他们送到同一顶点上去,骑士和国王的行动方式如图所看到的。国王能够选择一名骑士作为坐骑。上马后相当和该骑士
一起行动(相当于一个骑士),同一位置能够同一时候有多个骑士和国王。问最少走的步数
解题思路:把8*8棋盘变成0~63的数,Floyd求出随意两点之间的最短路径。8*8枚举就可以。枚举终点,骑士上马点,国王上哪个骑士,终于负责度O(64^4)。
*/
#include <string.h>
#include <algorithm>
#include <iostream>
#include <stdio.h>
using namespace std; char s[105];
int cx[8][2]= {{-2,-1},{-2,1},{-1,-2},{-1,2},{1,-2},{1,2},{2,-1},{2,1}};
int dx[8][2]= {{-1,-1},{-1,0},{-1,1},{0,-1},{0,1},{1,-1},{1,0},{1,1}};
int a[65][65],b[65][65],rking[65],king; bool judge(int i,int j)
{
if(i>=0&&i<8&&j>=0&&j<8)
return true;
return false;
} void init()
{
for(int i=0; i<64; i++)
{
for(int j=0; j<64; j++)
{
if(i==j)
a[i][j]=b[i][j]=0;
else
a[i][j]=b[i][j]=999;
}
}
for(int i=0; i<8; i++)
{
for(int j=0; j<8; j++)
{
for(int k=0; k<8; k++)
{
int x=i+cx[k][0];
int y=j+cx[k][1];
int xx=i+dx[k][0];
int yy=j+dx[k][1];
if(judge(x,y))
{
a[i+j*8][x+y*8]=1;
}
if(judge(xx,yy))
{
b[i+j*8][xx+yy*8]=1;
}
}
}
}
for(int k=0; k<64; k++)
{
for(int i=0; i<64; i++)
{
for(int j=0; j<64; j++)
{
a[i][j]=min(a[i][j],a[i][k]+a[k][j]);
b[i][j]=min(b[i][j],b[i][k]+b[k][j]);
}
}
}
}
int main()
{
init();
while(~scanf("%s",s))
{
int n=strlen(s);
king=s[0]-'A'+(s[1]-'1')*8;
int cnt=0;
for(int i=2; i<n; i+=2)
{
int x=s[i+1]-'1';
int y=s[i]-'A';
rking[cnt++]=x*8+y;
}
int ans=9999999;
for(int i=0;i<64;i++)///终点
{
for(int j=0;j<64;j++)///国王上马点
{
for(int k=0;k<cnt;k++)///国王所上的骑士
{
int sum=0;
for(int l=0;l<cnt;l++)
{
if(l==k)continue;
sum+=a[rking[l]][i];
}
sum+=b[king][j]+a[rking[k]][j]+a[j][i];
ans=min(ans,sum);
}
}
}
printf("%d\n",ans);
}
return 0;
}
poj1178 floyd+枚举的更多相关文章
- poj 1161 Floyd+枚举
题意是: 给出n个点,围成m个区域.从区域到另一个区域间需穿过至少一条边(若两区域相邻)——边连接着两点. 给出这么一幅图,并给出一些点,问从这些点到同一个区域的穿过边数最小值. 解题思路如下: 将区 ...
- POJ 2139 Six Degrees of Cowvin Bacon (Floyd)
题意:如果两头牛在同一部电影中出现过,那么这两头牛的度就为1, 如果这两头牛a,b没有在同一部电影中出现过,但a,b分别与c在同一部电影中出现过,那么a,b的度为2.以此类推,a与b之间有n头媒介牛, ...
- floyd最短路
floyd可以在O(n^3)的时间复杂度,O(n^2)的空间复杂度下求解正权图中任意两点间的最短路长度. 本质是动态规划. 定义f[k][i][j]表示从i出发,途中只允许经过编号小于等于k的点时的最 ...
- ZOJ 1232 【灵活运用FLOYD】 【图DP】
题意: copy自http://blog.csdn.net/monkey_little/article/details/6637805 有A个村子和B个城堡,村子标号是1~A,城堡标号是A+1~B.马 ...
- 简单的floyd——初学
前言: (摘自https://www.cnblogs.com/aininot260/p/9388103.html): 在最短路问题中,如果我们面对的是稠密图(十分稠密的那种,比如说全连接图),计算多 ...
- 套题T3
秋实大哥与线段树 Time Limit: 3000/1000MS (Java/Others) Memory Limit: 65535/65535KB (Java/Others) Submit ...
- bzoj千题计划123:bzoj1027: [JSOI2007]合金
http://www.lydsy.com/JudgeOnline/problem.php?id=1027 因为x+y+z=1,所以z=1-x-y 第三维可以忽略 将x,y 看做 平面上的点 简化问题: ...
- code1167 树网的核
floyd+枚举 看点: 1.floyd同时用数组p记录转移节点k,这样知道线段的端点u v就可以得到整条线段 2.任意一点c到线段a b的距离=(d[a][c]+d[c][b]-d[a][b])/2 ...
- APIO2017
商旅 在广阔的澳大利亚内陆地区长途跋涉后,你孤身一人带着一个背包来到了科巴.你被这个城市发达而美丽的市场所 深深吸引,决定定居于此,做一个商人.科巴有个集市,集市用从1到N的整数编号,集市之间通过M条 ...
随机推荐
- 洛谷——P2384 最短路
P2384 最短路 题目背景 狗哥做烂了最短路,突然机智的考了Bosh一道,没想到把Bosh考住了...你能帮Bosh解决吗? 他会给你10000000000000000000000000000000 ...
- Python开发基础-Day9-生成器、三元表达式、列表生成式、生成器表达式
生成器 生成器函数:函数体内包含有yield关键字,该函数执行的结果是生成器,生成器在本质上就是迭代器. def foo(): print('first------>') yield 1 pri ...
- 五种常用的C/C++编译器对64位整型的支持
变量定义 输出方式 gcc(mingw32) g++(mingw32) gcc(linux i386) g++(linux i386) MicrosoftVisual C++ 6.0 long lon ...
- [BZOJ1563][NOI2009]诗人小G(决策单调性优化DP)
模板题. 每个决策点都有一个作用区间,后来的决策点可能会比先前的优.于是对于每个决策点二分到它会比谁在什么时候更优,得到新的决策点集合与区间. #include<cstdio> #incl ...
- XMPP聊天之Openfire 的安装和配置---Mac OS
一.下载并安装openfire 1.下载最新的openfire安装文件 官方下载站点:http://www.igniterealtime.org/downloads/index.jsp#openfir ...
- Problem B: 年龄问题
#include<stdio.h> int xx(int n,int m,int k) { int a; ) { a=k; } else { a=xx(n-,m,k)+m; } retur ...
- js 数字键盘
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/ ...
- Inno Setup入门(二十)——Inno Setup类参考(6)
http://379910987.blog.163.com/blog/static/3352379720112515819485/ 存储框 存储框也是典型的窗口可视化组件,同编辑框类似,可以输入.显示 ...
- JavaWeb对RSA的使用
由于公司的网站页面的表单提交是明文的post,虽说是https的页面,但还是有点隐患(https会不会被黑?反正明文逼格是差了点你得承认啊),所以上头吩咐我弄个RSA加密,客户端JS加密,然后服务器J ...
- http://blog.csdn.net/congcong68/article/details/39256307
http://blog.csdn.net/congcong68/article/details/39256307