Spring 4 整合RMI技术及发布多个服务(xjl456852原创)
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.xiejl</groupId>
<artifactId>test</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<spring-version>4.3.7.RELEASE</spring-version>
</properties>
<dependencies>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-beans -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>${spring-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>${spring-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>${spring-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>${spring-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>${spring-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>${spring-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>${spring-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring-version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.aspectj/aspectjrt -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>1.8.10</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.aspectj/aspectjweaver -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.8.10</version>
</dependency>
</dependencies>
</project>
package com.xjl456852.rmi.spring;
/**
* 定义一个远程接口
*
* @author leizhimin 2009-8-17 13:53:38
*/
public interface HelloService {
/**
* 简单的返回“Hello World!"字样
*
* @return 返回“Hello World!"字样
*/
public String helloWorld();
/**
* 一个简单的业务方法,根据传入的人名返回相应的问候语
*
* @param someBodyName 人名
* @return 返回相应的问候语
*/
public String sayHelloToSomeBody(String someBodyName);
}
package com.xjl456852.rmi.spring;
import org.springframework.stereotype.Service;
/**
* 远程的接口的实现
*
* @author leizhimin 2009-8-17 13:54:38
*/
@Service
public class HelloServiceImpl implements HelloService {
public HelloServiceImpl() {
}
/**
* 简单的返回“Hello World!"字样
*
* @return 返回“Hello World!"字样
*/
public String helloWorld() {
return "Hello World!";
}
/**
* 一个简单的业务方法,根据传入的人名返回相应的问候语
*
* @param someBodyName 人名
* @return 返回相应的问候语
*/
public String sayHelloToSomeBody(String someBodyName) {
return "你好," + someBodyName + "!";
}
}
package com.xjl456852.rmi.spring;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
/**
* 通过Spring发布RMI服务
*
* @author leizhimin 2009-8-17 14:22:06
*/
public class HelloHost {
public static void main(String[] args) {
ApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml");
System.out.println("RMI服务伴随Spring的启动而启动了.....");
}
}
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:task="http://www.springframework.org/schema/task"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd
http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-3.2.xsd">
<!-- component-scan自动搜索@Component , @Controller , @Service , @Repository等标注的类 -->
<context:component-scan base-package="com.xjl456852" />
<bean id="serviceExporter" class="org.springframework.remoting.rmi.RmiServiceExporter">
<property name="serviceName" value="rmiSpring"/>
<property name="serviceInterface" value="com.xjl456852.rmi.spring.HelloService"/>
<property name="registryPort" value="8888"/>
<property name="service" ref="helloServiceImpl"/>
</bean>
</beans>

package com.xjl456852.rmi.spring;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import javax.annotation.Resource;
import java.rmi.RemoteException;
/**
* 通过Spring来调用RMI服务
*
* @author leizhimin 2009-8-17 14:12:46
*/
public class HelloClient {
@Resource
private HelloService helloService;
public static void main(String[] args) throws RemoteException {
ApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml");
HelloService hs = (HelloService) ctx.getBean("helloService");
System.out.println(hs.helloWorld());
System.out.println(hs.sayHelloToSomeBody("xjl456852"));
}
}
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:task="http://www.springframework.org/schema/task"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd
http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-3.2.xsd">
<!-- component-scan自动搜索@Component , @Controller , @Service , @Repository等标注的类 -->
<context:component-scan base-package="com.xjl456852" />
<bean id="helloService" class="org.springframework.remoting.rmi.RmiProxyFactoryBean">
<property name="serviceUrl" value="rmi://192.168.176.131:8888/rmiSpring"/>
<property name="serviceInterface" value="com.xjl456852.rmi.spring.HelloService"/>
</bean>
</beans>
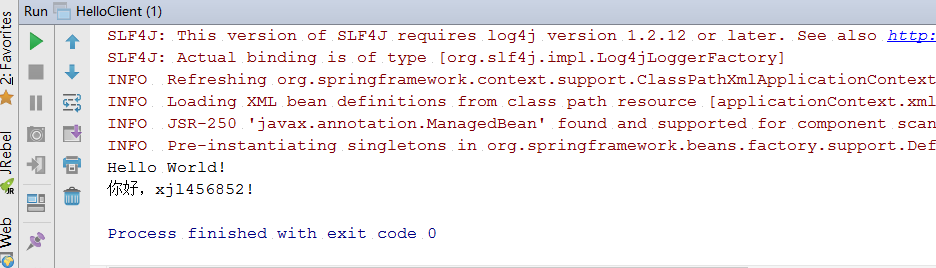
package com.xjl456852.rmi.spring;
/**
* Created by xjl on 2017/3/19.
*/
public interface OtherService {
public int random();
}
package com.xjl456852.rmi.spring;
import org.springframework.stereotype.Service;
import java.util.Random;
/**
* Created by xjl on 2017/3/19.
*/
@Service
public class OtherServiceImpl implements OtherService{
Random random = new Random();
public int random() {
System.out.println("invoke random method");
return random.nextInt(100);
}
}
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:task="http://www.springframework.org/schema/task"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd
http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-3.2.xsd">
<!-- component-scan自动搜索@Component , @Controller , @Service , @Repository等标注的类 -->
<context:component-scan base-package="com.xjl456852" />
<bean id="serviceExporter" class="org.springframework.remoting.rmi.RmiServiceExporter">
<property name="serviceName" value="rmiSpring"/>
<property name="serviceInterface" value="com.xjl456852.rmi.spring.HelloService"/>
<property name="registryPort" value="8888"/>
<property name="service" ref="helloServiceImpl"/>
</bean>
<bean id="serviceExporter_Other" class="org.springframework.remoting.rmi.RmiServiceExporter">
<property name="serviceName" value="rmiSpringOther"/>
<property name="serviceInterface" value="com.xjl456852.rmi.spring.OtherService"/>
<property name="registryPort" value="8888"/>
<property name="service" ref="otherServiceImpl"/>
</bean>
</beans>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:task="http://www.springframework.org/schema/task"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd
http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-3.2.xsd">
<!-- component-scan自动搜索@Component , @Controller , @Service , @Repository等标注的类 -->
<context:component-scan base-package="com.xjl456852" />
<bean id="helloService" class="org.springframework.remoting.rmi.RmiProxyFactoryBean">
<property name="serviceUrl" value="rmi://192.168.176.131:8888/rmiSpring"/>
<property name="serviceInterface" value="com.xjl456852.rmi.spring.HelloService"/>
</bean>
<bean id="otherService" class="org.springframework.remoting.rmi.RmiProxyFactoryBean">
<property name="serviceUrl" value="rmi://192.168.176.131:8888/rmiSpringOther"/>
<property name="serviceInterface" value="com.xjl456852.rmi.spring.OtherService"/>
</bean>
</beans>
package com.xjl456852.rmi.spring;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import javax.annotation.Resource;
import java.rmi.RemoteException;
/**
* 通过Spring来调用RMI服务
*
* @author leizhimin 2009-8-17 14:12:46
*/
public class HelloClient {
@Resource
private HelloService helloService;
@Resource
private OtherService otherService;
public static void main(String[] args) throws RemoteException {
ApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml");
HelloService hs = (HelloService) ctx.getBean("helloService");
System.out.println(hs.helloWorld());
System.out.println(hs.sayHelloToSomeBody("xjl456852"));
OtherService os = (OtherService) ctx.getBean("otherService");
System.out.println("otherService:" + os.random());
}
}


Spring 4 整合RMI技术及发布多个服务(xjl456852原创)的更多相关文章
- 黑马_13 Spring Boot:05.spring boot 整合其他技术
13 Spring Boot: 01.spring boot 介绍&&02.spring boot 入门 04.spring boot 配置文件 05.spring boot 整合其他 ...
- Spring+CXF整合来管理webservice(服务器启动发布webservice)
Spring+CXF整合来管理webservice 实现步骤: 1. 添加cxf.jar 包(集成了Spring.jar.servlet.jar ),spring.jar包 ,serv ...
- Spring Boot 整合视图层技术,application全局配置文件
目录 Spring Boot 整合视图层技术 Spring Boot 整合jsp Spring Boot 整合freemarker Spring Boot 整合视图层技术 Spring Boot 整合 ...
- Spring 5.x 、Spring Boot 2.x 、Spring Cloud 与常用技术栈整合
项目 GitHub 地址:https://github.com/heibaiying/spring-samples-for-all 版本说明: Spring: 5.1.3.RELEASE Spring ...
- Spring Boot从入门到精通之:二、Spring Boot整合JPA
springboot-jpa 开发工具 系统: windows10 开发工具: Intellij IDEA 2018.2.6 springboot: 2.0.6.RELEASE jdk: 1.8.0_ ...
- Spring MVC & Boot & Cloud 技术教程汇总(长期更新)
昨天我们发布了Java成神之路上的知识汇总,今天继续. Java成神之路技术整理(长期更新) 以下是Java技术栈微信公众号发布的关于 Spring/ Spring MVC/ Spring Boot/ ...
- spring boot 2.0(一)权威发布spring boot2.0
Spring Boot2.0.0.RELEASE正式发布,在发布Spring Boot2.0的时候还出现一个小插曲,将Spring Boot2.0同步到Maven仓库的时候出现了错误,然后Spring ...
- spring.jar是包含有完整发布的单个jar 包,spring.jar中包含除了spring-mock.jar里所包含的内容外其它所有jar包的内容,因为只有在开发环境下才会用到 spring-mock.jar来进行辅助测试,正式应用系统中是用不得这些类的。
Spring jar包的描述:针对3.2.2以上版本 org.springframework spring-aop ——Spring的面向切面编程,提供AOP(面向切面编程)实现 org.spring ...
- WebService之Spring+CXF整合示例
一.Spring+CXF整合示例 WebService是一种跨编程语言.跨操作系统平台的远程调用技术,它是指一个应用程序向外界暴露一个能通过Web调用的API接口,我们把调用这个WebService的 ...
随机推荐
- Python---scikit-learn(sklearn)模块
Python在机器学习方面一个非常强力的模块---scikit-learn模块,它作为数据挖掘和数据分析方面的一个简单而有效的工具,主要包括6大功能:分类(Classification),回归(Reg ...
- EasyUI Datagrid 分页显示(客户端)
转自:https://blog.csdn.net/metal1/article/details/17536185 EasyUI Datagrid 分页显示(客户端) By ZYZ 在使用JQuery ...
- 技嘉,u盘安装win7,提示“找不到驱动器设备驱动程序”
错误图: 解决办法: 网上说什么换usb2.0,修复用命令启动芸芸,反正对我来说没发现有什么卵用 详细步骤: 点击进入详细步骤页面地址
- HTML5来了,7个混合式移动开发框架
在这个时间开始学习移动开发真是最好不过了,每个人应该都有一些移动应用的创意,而且你并不需要任何的原生应用编程经验,你只需要一些HTML的相关知识,懂一些CSS和JavaScript就够了.如果你总听别 ...
- [App Store Connect帮助]五、管理构建版本(2)查看构建版本和文件大小
您可以查看您为某个 App 上传的所有构建版本,和由 App Store 创建的变体版本的大小.一些构建版本在该 App 发布到 App Store 上后可能不会显示. 必要职能:“帐户持有人”职能. ...
- JPA中关联关系(OneToOne、OneToMany、ManyToMany,ManyToOne)映射代码片段
在使用Hibernate的时候我们常常会在类里边配置各种的关联关系,但是这个并不是很好配置,配置不当会出现各种各样的问题,下面具体来看一下: 首先我们来看User类里边有一个IdentityCard类 ...
- [C++ STL] vector使用详解
一.vector介绍: vector(向量): 是一种序列式容器,事实上和数组差不多,但它比数组更优越.一般来说数组不能动态拓展,因此在程序运行的时候不是浪费内存,就是造成越界.而vector正好弥补 ...
- 25 C#类的继承
继承是面向对象编程的一个重要特性.任何类都可以从另一个类中继承,这就是说,这个类拥有它继承的类的所有成员.在OOP 中,被继承的类称为父类(也称为基类).注意,C#中的对象仅能直接派生于一个基类,当然 ...
- centos源码编译安装nginx过程记录
前言:Centos系统编译安装LNMP环境是每来一台新服务器或换电脑都需要做的事情.这里仅做一个记录.给初学者一个参考! 一.安装前的环境 这里用的是centos 7系统. 我们默认把下载的软件放在 ...
- 【C++】Item18. Make interfaces easy to use correctly and hard to use incorrectly
接口容易被正确使用,不易被误用 c++简单工厂模式时,初级实现为ITest* CreateTestOld(), 然后用户负责释放返回的对象.如果忘记释放就会造成memory leak,所以在设计工厂接 ...