UVa——1600Patrol Robot(A*或普通BFS)
Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
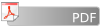
A robot has to patrol around a rectangular area which is in a form of mxn grid (m rows and n columns). The rows are labeled from 1 to m. The columns are labeled from 1 to n. A cell (i, j) denotes the cell in row i and column j in the grid. At each step, the robot can only move from one cell to an adjacent cell, i.e. from (x, y) to (x+ 1, y), (x, y + 1), (x - 1, y) or (x, y - 1). Some of the cells in the grid contain obstacles. In order to move to a cell containing obstacle, the robot has to switch to turbo mode. Therefore, the robot cannot move continuously to more than k cells containing obstacles.
Your task is to write a program to find the shortest path (with the minimum number of cells) from cell (1, 1) to cell (m, n). It is assumed that both these cells do not contain obstacles.
Input
The input consists of several data sets. The first line of the input file contains the number of data sets which is a positive integer and is not bigger than 20. The following lines describe the data sets.
For each data set, the first line contains two positive integer numbers m and n separated by space (1m, n
20). The second line contains an integer number k(0
k
20). The ith line of the next m lines contains n integer aij separated by space (i = 1, 2,..., m;j = 1, 2,..., n). The value of aij is 1 if there is an obstacle on the cell (i,j), and is 0 otherwise.
Output
For each data set, if there exists a way for the robot to reach the cell (m, n), write in one line the integer number s, which is the number of moves the robot has to make; -1 otherwise.
Sample Input
3
2 5
0
0 1 0 0 0
0 0 0 1 0
4 6
1
0 1 1 0 0 0
0 0 1 0 1 1
0 1 1 1 1 0
0 1 1 1 0 0
2 2
0
0 1
1 0
Sample Output
7
10
-1
一开始想用A*来着,g设为当前步数,h为曼哈顿距离,但似乎第三组死循环了……看来A*应用不够熟练啊,然后改为普通bfs,只用了二维的数组记录状态WA……抱着试一试的心态加了一维表示个当前点被穿过k步走过的记录,没想到A了……
代码:
#include<iostream>
#include<algorithm>
#include<cstdlib>
#include<sstream>
#include<cstring>
#include<cstdio>
#include<string>
#include<deque>
#include<stack>
#include<cmath>
#include<queue>
#include<set>
#include<map>
using namespace std;
#define INF 0x3f3f3f3f
#define MM(x,y) memset(x,y,sizeof(x))
typedef pair<int,int> pii;
typedef long long LL;
const double PI=acos(-1.0);
const int N=25;
int m,n,k;
int pos[N][N];
int vis[N][N][N];
struct info
{
int x;
int y;
int g;
int kk;
};
queue<info>Q;
info direct[4]={{0,1,1,0},{1,0,1,0},{0,-1,1,0},{-1,0,1,0}};
inline info operator+(const info &a,const info &b)
{
info c;
c.x=a.x+b.x;
c.y=a.y+b.y;
c.g=a.g+b.g;
return c;
}
bool check(const info &a)
{
return (a.x>=0&&a.x<m&&a.y>=0&&a.y<n&&a.kk<=k);
}
void init()
{
MM(pos,0);
MM(vis,0);
while (!Q.empty())
Q.pop();
}
int main(void)
{
int tcase,i,j;
scanf("%d",&tcase);
while (tcase--)
{
init();
scanf("%d%d",&m,&n);
scanf("%d",&k);
for (i=0; i<m; ++i)
{
for (j=0; j<n; ++j)
scanf("%d",&pos[i][j]);
}
int r=-1;
info S={0,0,0,0};
vis[S.x][S.y][S.kk]=1;
Q.push(S);
while (!Q.empty())
{
info now=Q.front();
Q.pop();
if(now.x==m-1&&now.y==n-1)
{
r=now.g;
break;
}
for (i=0; i<4; i++)
{
info v=now+direct[i];
if(!pos[v.x][v.y])
v.kk=0;
else
v.kk=now.kk+1;
if(check(v)&&!vis[v.x][v.y][v.kk])
{
Q.push(v);
vis[v.x][v.y][v.kk]=1;
}
}
}
printf("%d\n",r);
}
return 0;
}
A*代码:
#include<iostream>
#include<algorithm>
#include<cstdlib>
#include<sstream>
#include<cstring>
#include<cstdio>
#include<string>
#include<deque>
#include<stack>
#include<cmath>
#include<queue>
#include<set>
#include<map>
using namespace std;
#define INF 0x3f3f3f3f
#define MM(x,y) memset(x,y,sizeof(x))
typedef pair<int,int> pii;
typedef long long LL;
const double PI=acos(-1.0);
const int N=25;
int m,n,k;
int pos[N][N];
int vis[N][N][N];
struct info
{
int x;
int y;
int g;
int kk;
int h;
bool operator<(const info &b)const
{
if(h+g!=b.h+b.g)
return h+g>b.h+b.g;
if(g!=b.g)
return g>b.g;
return kk>b.kk;
}
};
priority_queue<info>Q;
info direct[4]={{0,1,1,0,0},{1,0,1,0,0},{0,-1,1,0,0},{-1,0,1,0,0}};
inline info operator+(const info &a,const info &b)
{
info c;
c.x=a.x+b.x;
c.y=a.y+b.y;
c.g=a.g+b.g;
return c;
}
bool check(const info &a)
{
return (a.x>=0&&a.x<m&&a.y>=0&&a.y<n&&a.kk<=k&&!vis[a.x][a.y][a.kk]);
}
void init()
{
MM(pos,0);
MM(vis,0);
while (!Q.empty())
Q.pop();
}
int main(void)
{
int tcase,i,j;
scanf("%d",&tcase);
while (tcase--)
{
init();
scanf("%d%d",&m,&n);
scanf("%d",&k);
for (i=0; i<m; ++i)
{
for (j=0; j<n; ++j)
{
scanf("%d",&pos[i][j]);
}
}
int r=-1;
info S={0,0,0,0,n+m-2};
vis[S.x][S.y][S.kk]=1;
Q.push(S);
while (!Q.empty())
{
info now=Q.top();
Q.pop();
if(now.x==m-1&&now.y==n-1)
{
r=now.g;
break;
}
for (i=0; i<4; i++)
{
info v=now+direct[i];
if(!pos[v.x][v.y])
v.kk=0;
else
v.kk=now.kk+1;
if(check(v))
{
v.h=m-1+n-1-v.x-v.y;
Q.push(v);
vis[v.x][v.y][v.kk]=1;
}
}
}
printf("%d\n",r);
}
return 0;
}
UVa——1600Patrol Robot(A*或普通BFS)的更多相关文章
- UVa 439骑士的移动(BFS)
https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem& ...
- UVA 11624 Fire!(两次BFS+记录最小着火时间)
题目链接:https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem ...
- F - Robot Motion 栈加BFS
A robot has been programmed to follow the instructions in its path. Instructions for the next direct ...
- [Uva 10085] The most distant state (BFS)
题目链接:http://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem& ...
- CodeForces 589J Cleaner Robot (DFS,或BFS)
题意:给定n*m的矩阵,一个机器人从一个位置,开始走,如果碰到*或者边界,就顺时针旋转,接着走,问你最后机器人最多能走过多少格子. 析:这个题主要是题意读的不大好,WA了好几次,首先是在*或者边界才能 ...
- HDU 4166 & BNU 32715 Robot Navigation (记忆化bfs)
题意:给一个二维地图,每个点为障碍或者空地,有一个机器人有三种操作:1.向前走:2.左转90度:3.右转90度.现给定起点和终点,问到达终点最短路的条数. 思路:一般的题目只是求最短路的长度,但本题还 ...
- UVa 816 Abbott的复仇(BFS)
寒假的第一道题目,在放假回家颓废了两天后,今天终于开始刷题了.希望以后每天也能多刷几道题. 题意:这道BFS题还是有点复杂的,给一个最多9*9的迷宫,但是每个点都有不同的方向,每次进入该点的方向不同, ...
- [ACM_模拟] UVA 12503 Robot Instructions [指令控制坐标轴上机器人移动 水]
Robot Instructions You have a robot standing on the origin of x axis. The robot will be given som ...
- UVA 11624 Fire!【两点BFS】
Joe works in a maze. Unfortunately, portions of the maze have caught on fire, and the owner of the m ...
随机推荐
- SQLServer外键查询删除信息
SELECT FK.NAME,FK.OBJECT_ID,OBJECT_NAME(FK.PARENT_OBJECT_ID) AS REFERENCETABLENAMEFROM SYS.FOREIGN_K ...
- HDU 3033 I love sneakers! 我爱运动鞋 (分组背包+01背包,变形)
题意: 有n<=100双鞋子,分别属于一个牌子,共k<=10个牌子.现有m<=10000钱,问每个牌子至少挑1双,能获得的最大价值是多少? 思路: 分组背包的变形,变成了相反的,每组 ...
- 鼠标点击后的CSS3跑马灯效果
代码: CSS: /*旋转木马*/ #rotate_container li { width: 128px; box-shadow: 0 1px 3px rgba(0, 0, 0, .5); posi ...
- 开源项目: circular-progress-button
带进度条显示的按钮, 其效果如下所示: 其由三部分动画组成: 初始状态->圆环状态->完成状态. 0. 实现从初始到圆环的简单实现: 继承自button 类, 设置其背景 public c ...
- 特别困的学生 UVa12108(模拟题)
一.题目 课堂上有n个学生(n<=10).每个学生都有一个“睡眠-清醒”周期,其中第i个学生醒Ai分钟后睡Bi分钟,然后重复(1<=Ai,Bi<=5),初始第i个同学处于他的周期的C ...
- python基础面试题整理---从零开始 每天十题(02)
书接上回,我们继续来说说python的面试题,我在各个网站搜集了一些,我给予你们一个推荐的答案,你们可以组织成自己的语言来说出来,让我们更好的做到面向工资编程 一.Q:说说你对zen of pytho ...
- Window命令行杀进程
Window命令行杀进程 1.查看任务列表 tasklist 2.以映象名杀 taskkill -t -f -im xx.exe 3.以进程杀死 taskkill /pid pid号 /f 4.针对w ...
- Xcode 6 创建 Empty Application
1.创建一个 Single View Application: 2.删除工程目录下的 Main.storyboard 和 LaunchScreen.xib: 3.打开 Supporting Files ...
- iOS UIView中的坐标转换convertPoint --- iOS开发系列 ---项目中成长的知识六
如果你的UITableViewCell里面有一个Button需要响应事件,你会怎么做? 在Controller中使用 button父类的父类? 例如:UITableViewCell *parent ...
- MySQL中的字符串
MySQL的字符串是从1开始编号的,这与计算机编程语言有所不同,在MySQL中1代表第一个字符,-1代表最后一个字符,以此类推. MySQL中百分号“%”代表的是任意个字符,下划线“_”代表的是任意一 ...