javascript实现集合Set、字典Dictionary、HashTable
集合是由一组无序且唯一(即不能重复)的项组成的。这个数据结构使用了与有限集合相同的数学概念,但应用在计算机科学的数据结构中。
function Set() {
this.items = {};
} Set.prototype = {
constructer: Set,
has: function(value) {
return value in this.items;
},
add: function(value) {
if (!this.has(value)) {
this.items[value] = value;
return true;
}
return false;
},
remove: function(value) {
if (this.has(value)) {
delete this.items[value];
return true;
}
return false;
},
clear: function() {
this.items = {};
},
size: function() {
return Object.keys(this.items).length;
},
values: function() {
return Object.keys(this.items); //values是数组
},
union: function(otherSet) {
var unionSet = new Set();
var values = this.values();
for (var i = 0; i < values.length; i++) {
unionSet.add(values[i]);
}
values = otherSet.values();
for (var i = 0; i < values.length; i++) {
unionSet.add(values[i]);
}
return unionSet;
},
intersection: function(otherSet) {
var intersectionSet = new Set();
var values = this.values();
for (var i = 0; i < values.length; i++) {
if (otherSet.has(values[i])) {
intersectionSet.add(values[i]);
}
}
return intersectionSet;
},
difference: function(otherSet) {
var differenceSet = new Set();
var values = otherSet.values();
for (var i = 0; i < values.length; i++) {
if (!this.has(values[i])) {
differenceSet.add(values[i]);
}
}
return differenceSet;
},
subset: function(otherSet) {
if (this.size() > otherSet.size()) {
return false;
} else {
var values = this.values();
for (var i = 0; i < values.length; i++) {
if (!otherSet.has(values[i])) {
return false;
}
}
}
return true;
},
}
集合表示一组互不相同的元素(不重复的元素)。在字典中,存储的是[键,值] 对,其中键名是用来查询特定元素的。字典和集合很相似,集合以[值,值]的形式存储元素,字 典则是以[键,值]的形式来存储元素。字典也称作映射。
function Dictionary() {
this.items = {};
}
Dictionary.prototype = {
constructor: Dictionary,
has: function(key) {
return key in this.items;
},
set: function(key, value) {
this.items[key] = value;
},
remove: function(key) {
if (this.has(key)) {
delete this.items[key];
return true;
}
return false;
},
get: function(key) {
return this.has(key) ? this.items[key] : undefined;
},
values: function() {
var values = [];
for (var key in this.items) {
if (this.has(key)) {
values.push(key);
}
}
return values;
},
clear: function() {
this.items = {};
},
size: function() {
return Object.keys(this.items).length;
},
keys: function() {
return Object.keys(this.items);
},
getItems: function() {
return this.items;
}
};
散列表
HashTable类,也叫HashMap类,是Dictionary类的一种散列表实现方式。散列算法的作用是尽可能快地在数据结构中找到一个值。如果使用散列函数,就知道值的具体位置,因此能够快速检索到该值。散列函数的作用是给定一个键值,然后 返回值在表中的地址。
//lose-lose散列函数
function loseloseHashCode(key) {
var hash = 0;
for (var i = 0; i < key.length; i++) {
hash += key.charCodeAt(i);
}
return hash % 37;
} function HashTable() {
this.table = [];
}
HashTable.prototype = {
constructor: HashTable,
put: function(key, value) {
var position = loseloseHashCode(key);
console.log(position + '- ' + key);
this.table[position] = value;
},
get: function(key) {
return this.table[loseloseHashCode(key)];
},
remove: function(key) {
this.table[loseloseHashCode(key)] = undefined;
}
}; var hash = new HashTable();
hash.put('Gandalf', 'gandalf@email.com');
hash.put('John', 'johnsnow@email.com');
hash.put('Tyrion', 'tyrion@email.com');
console.log(hash.get('Gandalf')); //gandalf@email.com
console.log(hash.get('Loiane')); //undefined
hash.remove('Gandalf');
console.log(hash.get('Gandalf')); //undefined
分离链接:分离链接法包括为散列表的每一个位置创建一个链表并将元素存储在里面。它是解决冲突的最简单的方法,但是它在HashTable实例之外还需要额外的存储空间。
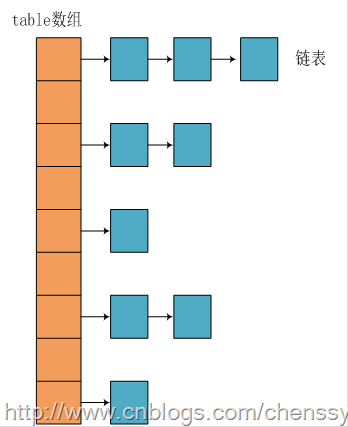
function HashTable() {
this.table = [];
//lose-los散列函数
function loseloseHashCode(key) {
var hash = 0;
for (var i = 0; i < key.length; i++) {
hash += key.charCodeAt(i);
}
//console.log(key + " - " + (hash % 37));
return hash % 37;
} function ValuePair(key, value) {
this.key = key;
this.value = value;
this.toString = function() {
return '[' + this.key + ' - ' + this.value + ']';
}
}
if ((typeof this.put !== 'function') && (typeof this.put !== 'string')) {
HashTable.prototype.put = function(key, value) {
var position = loseloseHashCode(key);
if (this.table[position] === undefined) {
this.table[position] = new LinkedList();
}
this.table[position].append(new ValuePair(key, value));
};
HashTable.prototype.get = function(key) {
var position = loseloseHashCode(key);
if (this.table[position] !== undefined) {
var current = this.table[position].getHead();
while (current.next) {
if (current.element.key === key) {
return current.element.value;
}
current = current.next;
}
//第一个元素或者最后一个元素
if (current.element.key === key) {
return current.element.value;
}
} else {
return undefined;
}
};
HashTable.prototype.remove = function(key) {
var position = loseloseHashCode(key);
if (this.table[position] !== undefined) {
var current = this.table[position].getHead();
while (current.next) {
if (current.element.key === key) {
this.table[position].remove(current.element);
if (this.table[position].isEmpty()) {
this.table[position] = undefined;
}
return true;
}
current = current.next;
}
//检查是否是第一个或者最后一个
if (current.element.key === key) {
this.table[position].remove(current.element);
if (this.table[position].isEmpty()) {
this.table[position] = undefined;
}
return true;
}
}
return false;
};
}
}
var hash = new HashTable();
hash.put('Gandalf', 'gandalf@email.com');
hash.put('John', 'johnsnow@email.com');
//下面两个hash值相同
hash.put('Aaron', 'huang@gmail.com');
hash.put('Tyrion', 'tyrion@email.com');
console.log(hash.get('Gandalf')); //gandalf@email.com
console.log(hash.get('Loiane')); //undefined
hash.remove('Gandalf');
console.log(hash.get('Gandalf')); //undefined
javascript实现集合Set、字典Dictionary、HashTable的更多相关文章
- JavaScript实现集合与字典
JavaScript实现集合与字典 一.集合结构 1.1.简介 集合比较常见的实现方式是哈希表,这里使用JavaScript的Object类进行封装. 集合通常是由一组无序的.不能重复的元素构成. 数 ...
- python 数据类型: 字符串String / 列表List / 元组Tuple / 集合Set / 字典Dictionary
#python中标准数据类型 字符串String 列表List 元组Tuple 集合Set 字典Dictionary 铭记:变量无类型,对象有类型 #单个变量赋值 countn00 = '; #整数 ...
- JavaScript数据结构——集合、字典和散列表
集合.字典和散列表都可以存储不重复的值. 在集合中,我们感兴趣的是每个值本身,并把它当作主要元素.在字典和散列表中,我们用 [键,值] 的形式来存储数据. 集合(Set 类):[值,值]对,是一组由无 ...
- C#中数组、集合(ArrayList)、泛型集合List<T>、字典(dictionary<TKey,TValue>)全面对比
C#中数组.集合(ArrayList).泛型集合List<T>.字典(dictionary<TKey,TValue>)全面对比 为什么把这4个东西放在一起来说,因为c#中的这4 ...
- 你真的了解字典(Dictionary)吗? C# Memory Cache 踩坑记录 .net 泛型 结构化CSS设计思维 WinForm POST上传与后台接收 高效实用的.NET开源项目 .net 笔试面试总结(3) .net 笔试面试总结(2) 依赖注入 C# RSA 加密 C#与Java AES 加密解密
你真的了解字典(Dictionary)吗? 从一道亲身经历的面试题说起 半年前,我参加我现在所在公司的面试,面试官给了一道题,说有一个Y形的链表,知道起始节点,找出交叉节点.为了便于描述,我把上面 ...
- 索引器、哈希表Hashtabl、字典Dictionary(转)
一.索引器 索引器类似于属性,不同之处在于它们的get访问器采用参数.要声明类或结构上的索引器,使用this关键字. 示例: 索引器示例代码 /// <summary> /// 存储星 ...
- 使用JavaScriptSerializer序列化集合、字典、数组、DataTable为JSON字符串 分类: 前端 数据格式 JSON 2014-10-30 14:08 169人阅读 评论(0) 收藏
一.JSON简介 JSON(JavaScript Object Notation,JavaScript对象表示法)是一种轻量级的数据交换格式. JSON是"名值对"的集合.结构由大 ...
- Java 集合系列11之 Hashtable详细介绍(源码解析)和使用示例
概要 前一章,我们学习了HashMap.这一章,我们对Hashtable进行学习.我们先对Hashtable有个整体认识,然后再学习它的源码,最后再通过实例来学会使用Hashtable.第1部分 Ha ...
- [Python]字典Dictionary、列表List、元组Tuple差异化理解
概述:Python中这三种形式的定义相近,易于混淆,应注意区分. aDict={'a':1, 'b':2, 'c':3, 'd':4, 'e':5} aList=[1,2,3,4,5] aTuple= ...
- [Swift]遍历集合类型(数组、集合和字典)
Swift提供了三种主要的集合类型,称为数组,集合和字典,用于存储值集合. 数组是有序的值集合. 集是唯一值的无序集合. 字典是键值关联的无序集合. Swift中无法再使用传统形式的for循环. // ...
随机推荐
- BIOS将MBR读入0x7C00地址处(x86平台下)
BIOS将MBR读入0x7C00地址处(x86平台下) https://www.cnblogs.com/jikebiancheng/p/6193953.html http://www.ruanyife ...
- Navicat for MySQL 设置定时任务(事件)
1.查询界面输入命令,查看定时任务是否开启,未开始时OFF: show variables like '%event_scheduler%'; 2. 查询界面输入命令,开启定时任务: set glob ...
- mysql启动失败,unit not found
1 mysql启动 Failed to start mysqld.service: Unit not found. 2 查询/etc/init.d/下是否存在mysqld ll /etc/init ...
- centos 6.4系统双网卡绑定配置详解
Linux双网卡绑定实现就是使用两块网卡虚拟成为一块网卡(需要交换机支持),这个聚合起来的设备看起来是一个单独的以太网接口设备,通俗点讲就是两块网卡具有相同的IP地址而并行链接聚合成一个逻辑链路工作. ...
- asp.net 设计音乐网站
第一步 收集资料 http://www.logoko.com.cn/ --设计logo网站 设计音乐文档 https://wenku.baidu.com/view/3d957617f18583 ...
- Shell脚本相关
cat /proc/17616/cmdline 17616代表进程号 用这个可以完整打印出当前的进程的全名 当前shell的进程号.你可以使用ps -A 看你自己shell 的pid.是内置变量. $ ...
- java—锁的学习研究
摘抄自博客:https://www.cnblogs.com/qifengshi/p/6831055.html 标题:Java中的锁分类 锁的分类: 公平锁/非公平锁 可重入锁 独享锁/共享锁 互斥锁/ ...
- 接口自动化平台——httprunnermanager
Windows 环境搭建 1. 下载安装pip install httprunner==1.4.2hrun -V #1.4.2har2case -V #0.1.8 2. httprunnermanag ...
- MNPR--造福人类的人值得被感激
https://artineering.io/research/MNPR/ https://mnpr.artineering.io https://pdfs.semanticscholar.org/0 ...
- iphone bandwidth
iPhone 8, 8 Plus, X peak throughput of ~24GBs iPhone XS, XS Max, XR peak throughput of ~34GBs 在iphon ...