easyexcel: The maximum length of cell contents (text) is 32,767 characters
easyexcel The maximum length of cell contents (text) is 32,767 characters
.xls和.xlsx文件格式有32,767个字符的硬限制. Apache POI只是强制执行Excel限制的文件格式.您可以在Microsoft文档中查看这些限制的详细信息,也可以在 this Apache POI javadoc page中很好地捕获
org.apache.poi.ss.SpreadsheetVersion
中只用excel97 和excel2007俩个选项 ,XSSFCell 类被修饰为final 不能被继承重写版本校验方法。在自己的项目文件夹下创建org.apache.poi.ss.SpreadsheetVersion 类,复制poi中的该类源码,excel2007中的最后一个值改为int类型最大值。重试导出问题解决。

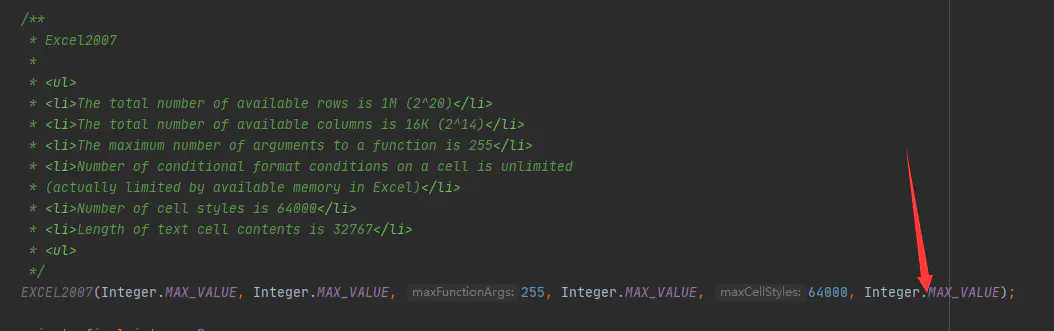
方法二:截取,分成多列存储


1 private static void writeRowsToExcel(XSSFWorkbook wb, Sheet sheet, List<List<Object>> rows, int rowIndex) {
2 int colIndex = 0;
3
4 Font dataFont = wb.createFont();
5 dataFont.setFontName("simsun");
6 dataFont.setColor(IndexedColors.BLACK.index);
7
8 XSSFCellStyle dataStyle = wb.createCellStyle();
9 dataStyle.setAlignment(XSSFCellStyle.ALIGN_CENTER);
10 dataStyle.setVerticalAlignment(XSSFCellStyle.VERTICAL_CENTER);
11 dataStyle.setFont(dataFont);
12 setBorder(dataStyle, BorderStyle.THIN, new XSSFColor(new Color(0, 0, 0)));
13
14 for (List<Object> rowData : rows) {
15 Row dataRow = sheet.createRow(rowIndex);
16 colIndex = 0;
17 for (Object cellData : rowData) {
18 colIndex = setCell(cellData.toString(), dataRow, colIndex, dataStyle);
19 }
20 rowIndex++;
21 }
22 }
23
24 private static int setCell(String data, Row dataRow, Integer colIndex, XSSFCellStyle dataStyle) {
25
26 int max = 32767;
27 if (data == null || data.length() < max) {
28 Cell cell = dataRow.createCell(colIndex);
29 cell.setCellStyle(dataStyle);
30 cell.setCellValue(data == null ? "" : data);
31 colIndex++;
32 } else {
33 int num = data.length() / max;
34 for (int i = 0; i < num; i++) {
35 Cell cell = dataRow.createCell(colIndex);
36 cell.setCellStyle(dataStyle);
37 cell.setCellValue(data.substring(i * max, max * (i + 1)));
38 colIndex++;
39 }
40 int extra = data.length() % max;
41 if (extra > 0) {
42 Cell cell = dataRow.createCell(colIndex);
43 cell.setCellStyle(dataStyle);
44 cell.setCellValue(data.substring(num * max, num * max + extra));
45 colIndex++;
46 }
47 }
48 return colIndex;
49 }
示例
方法三:切换文件格式 (推荐)
如果您确实需要长文本,则需要切换到另一种文件格式,例如CSV
CSV是逗号分隔文件(Comma Separated Values)的首字母英文缩写,是一种用来存储数据的纯文本格式,通常用于电子表格或数据库软件。在 CSV文件中,数据“栏”以逗号分隔,可允许程序通过读取文件为数据重新创建正确的栏结构,并在每次遇到逗号时开始新的一栏。如:
1
,张三,男
2
,李四,男
3
,小红,女


1 package com.yph.omp.common.util;
2
3 import java.io.BufferedWriter;
4 import java.io.File;
5 import java.io.FileInputStream;
6 import java.io.FileNotFoundException;
7 import java.io.FileOutputStream;
8 import java.io.IOException;
9 import java.io.InputStream;
10 import java.io.OutputStream;
11 import java.io.OutputStreamWriter;
12 import java.util.ArrayList;
13 import java.util.Iterator;
14 import java.util.LinkedHashMap;
15 import java.util.List;
16 import java.util.Map;
17
18 import javax.servlet.http.HttpServletRequest;
19 import javax.servlet.http.HttpServletResponse;
20
21 import org.apache.commons.beanutils.BeanUtils;
22 import org.junit.Test;
23
24 /**
25 * Java生成CSV文件
26 */
27 public class CSVUtil {
28
29 /**
30 * 生成为CVS文件
31 *
32 * @param exportData
33 * 源数据List
34 * @param map
35 * csv文件的列表头map
36 * @param outPutPath
37 * 文件路径
38 * @param fileName
39 * 文件名称
40 * @return
41 */
42 @SuppressWarnings("rawtypes")
43 public static File createCSVFile(List exportData, LinkedHashMap map,
44 String outPutPath, String fileName) {
45 File csvFile = null;
46 BufferedWriter csvFileOutputStream = null;
47 try {
48 File file = new File(outPutPath);
49 if (!file.exists()) {
50 file.mkdir();
51 }
52 // 定义文件名格式并创建
53 csvFile = File.createTempFile(fileName, ".csv",
54 new File(outPutPath));
55 // UTF-8使正确读取分隔符","
56 csvFileOutputStream = new BufferedWriter(new OutputStreamWriter(
57 new FileOutputStream(csvFile), "GBK"), 1024);
58 // 写入文件头部
59 for (Iterator propertyIterator = map.entrySet().iterator(); propertyIterator
60 .hasNext();) {
61 java.util.Map.Entry propertyEntry = (java.util.Map.Entry) propertyIterator
62 .next();
63 csvFileOutputStream
64 .write("\"" + (String) propertyEntry.getValue() != null ? (String) propertyEntry
65 .getValue() : "" + "\"");
66 if (propertyIterator.hasNext()) {
67 csvFileOutputStream.write(",");
68 }
69 }
70 csvFileOutputStream.newLine();
71 // 写入文件内容
72 for (Iterator iterator = exportData.iterator(); iterator.hasNext();) {
73 Object row = (Object) iterator.next();
74 for (Iterator propertyIterator = map.entrySet().iterator(); propertyIterator
75 .hasNext();) {
76 java.util.Map.Entry propertyEntry = (java.util.Map.Entry) propertyIterator
77 .next(); /*-------------------------------*/
78 //以下部分根据不同业务做出相应的更改
79 StringBuilder sbContext = new StringBuilder("");
80 if (null != BeanUtils.getProperty(row,(String) propertyEntry.getKey())) {
81 if("证件号码".equals(propertyEntry.getValue())){
82 //避免:身份证号码 ,读取时变换为科学记数 - 解决办法:加 \t(用Excel打开时,证件号码超过15位后会自动默认科学记数)
83 sbContext.append(BeanUtils.getProperty(row,(String) propertyEntry.getKey()) + "\t");
84 }else{
85 sbContext.append(BeanUtils.getProperty(row,(String) propertyEntry.getKey()));
86 }
87 }
88 csvFileOutputStream.write(sbContext.toString());
89 /*-------------------------------*/
90 if (propertyIterator.hasNext()) {
91 csvFileOutputStream.write(",");
92 }
93 }
94 if (iterator.hasNext()) {
95 csvFileOutputStream.newLine();
96 }
97 }
98 csvFileOutputStream.flush();
99 } catch (Exception e) {
100 e.printStackTrace();
101 } finally {
102 try {
103 csvFileOutputStream.close();
104 } catch (IOException e) {
105 e.printStackTrace();
106 }
107 }
108 return csvFile;
109 }
110
111 /**
112 * 下载文件
113 *
114 * @param response
115 * @param csvFilePath
116 * 文件路径
117 * @param fileName
118 * 文件名称
119 * @throws IOException
120 */
121 public static void exportFile(HttpServletRequest request,
122 HttpServletResponse response, String csvFilePath, String fileName)
123 throws IOException {
124 response.setCharacterEncoding("UTF-8");
125 response.setContentType("application/csv;charset=GBK");
126
127 response.setHeader("Content-Disposition", "attachment; filename="
128 + new String(fileName.getBytes("GB2312"), "ISO8859-1"));
129 InputStream in = null;
130 try {
131 in = new FileInputStream(csvFilePath);
132 int len = 0;
133 byte[] buffer = new byte[1024];
134 OutputStream out = response.getOutputStream();
135 while ((len = in.read(buffer)) > 0) {
136 out.write(buffer, 0, len);
137 }
138 } catch (FileNotFoundException e1) {
139 System.out.println(e1);
140 } finally {
141 if (in != null) {
142 try {
143 in.close();
144 } catch (Exception e1) {
145 throw new RuntimeException(e1);
146 }
147 }
148 }
149 }
150
151 /**
152 * 删除该目录filePath下的所有文件
153 *
154 * @param filePath
155 * 文件目录路径
156 */
157 public static void deleteFiles(String filePath) {
158 File file = new File(filePath);
159 if (file.exists()) {
160 File[] files = file.listFiles();
161 for (int i = 0; i < files.length; i++) {
162 if (files[i].isFile()) {
163 files[i].delete();
164 }
165 }
166 }
167 }
168
169 /**
170 * 删除单个文件
171 *
172 * @param filePath
173 * 文件目录路径
174 * @param fileName
175 * 文件名称
176 */
177 public static void deleteFile(String filePath, String fileName) {
178 File file = new File(filePath);
179 if (file.exists()) {
180 File[] files = file.listFiles();
181 for (int i = 0; i < files.length; i++) {
182 if (files[i].isFile()) {
183 if (files[i].getName().equals(fileName)) {
184 files[i].delete();
185 return;
186 }
187 }
188 }
189 }
190 }
191
192 @SuppressWarnings({ "unchecked", "rawtypes" })
193 @Test
194 public void createFileTest() {
195 List exportData = new ArrayList<Map>();
196 Map row1 = new LinkedHashMap<String, String>();
197 row1.put("1", "11");
198 row1.put("2", "12");
199 row1.put("3", "13");
200 row1.put("4", "14");
201 exportData.add(row1);
202 row1 = new LinkedHashMap<String, String>();
203 row1.put("1", "21");
204 row1.put("2", "22");
205 row1.put("3", "23");
206 row1.put("4", "24");
207 exportData.add(row1);
208 LinkedHashMap map = new LinkedHashMap();
209 map.put("1", "第一列");
210 map.put("2", "第二列");
211 map.put("3", "第三列");
212 map.put("4", "第四列");
213
214 String path = "d:/export";
215 String fileName = "文件导出";
216 File file = CSVUtil.createCSVFile(exportData, map, path, fileName);
217 String fileNameNew = file.getName();
218 String pathNew = file.getPath();
219 System.out.println("文件名称:" + fileNameNew );
220 System.out.println("文件路径:" + pathNew );
221 }
222 }//注:BeanUtils.getProperty(row,(String) propertyEntry.getKey()) + "\t" ,只为解决数字格式超过15位后,在Excel中打开展示科学记数问题。
Java生成CSV文件
https://www.jianshu.com/p/9e73399b6c38
easyexcel: The maximum length of cell contents (text) is 32,767 characters的更多相关文章
- Maximum length of a table name in MySQL
http://dev.mysql.com/doc/refman/5.7/en/identifiers.html The following table describes the maximum le ...
- The maximum number of cell styles was exceeded. You can define up to 4000 styles
POI操作Excel中,导出的数据不是很大时,则不会有问题,而数据很多或者比较多时, 就会报以下的错误,是由于cell styles太多create造成,故一般可以把cellstyle设置放到循环外面 ...
- [LeetCode] Maximum Length of Repeated Subarray 最长的重复子数组
Given two integer arrays A and B, return the maximum length of an subarray that appears in both arra ...
- [Swift]LeetCode718. 最长重复子数组 | Maximum Length of Repeated Subarray
Given two integer arrays A and B, return the maximum length of an subarray that appears in both arra ...
- oracle 导入报错:field in data file exceeds maximum length
今天用sqlldr导入数据时候报错: " Record 1: Rejected - Error on table ks_test, column khname.Field in data f ...
- [LeetCode]Maximum Length of Repeated Subarray
Maximum Length of Repeated Subarray: Given two integer arrays A and B, return the maximum length of ...
- sqlldr Field in data file exceeds maximum length "
使用sqlldr导数时出现如下错误: " Record 1: Rejected - Error on table PC_PLANNAME, column PLANNAME.Field in ...
- LeetCode Maximum Length of Pair Chain
原题链接在这里:https://leetcode.com/problems/maximum-length-of-pair-chain/description/ 题目: You are given n ...
- 【POI】导出xls文件报错:The maximum number of cell styles was exceeded. You can define up to 4000 styles in a .xls workbook
使用POI导出xls文件,由于数据过多,导致导出xls报错如下: The maximum number of cell styles was exceeded. You can define up t ...
- FireDAC 出现Variable length column[*] overflow. Value length - [80], column maximum length
FireDAC 出现Variable length column[*] overflow. Value length - [80], column maximum length FireDAC的 TF ...
随机推荐
- [转帖]FIO使用说明
FIO介绍: FIO是测试IOPS的非常好的工具,用来对磁盘进行压力测试和验证.磁盘IO是检查磁盘性能的重要指标,可以按照负载情况分成照顺序读写,随机读写两大类.FIO是一个可以产生很多线程或进程并执 ...
- [转帖] 容器内的Linux诊断工具0x.tools
https://www.cnblogs.com/codelogs/p/16242999.html 原创:扣钉日记(微信公众号ID:codelogs),欢迎分享,转载请保留出处. 简介# Linux上有 ...
- [转帖]Linux下进程管理知识(详细)总结
一.简介 本文主要详细介绍进程相关的命令的使用.进程管理及调度策略的知识. 二.常用的命令解析 1.ps命令 命令选项 解析 -a 显示一个终端所有的进程 -u 显示进程的归属用户和内存占用情况 -x ...
- OpenEuler2203安装Redislabs的简单记录
OpenEuler2203安装Redislabs的简单记录 背景 操作系统国产化的需求下 想着都转型到openEuler上面来. 应用和容器都没什么问题了,现在考虑一下一些企业软件 最近一直在想研究一 ...
- 【一个小发现】VictoriaMetrics 中 vmselect 的 `-search.denyPartialResponse` 选项不应该开启
作者:张富春(ahfuzhang),转载时请注明作者和引用链接,谢谢! cnblogs博客 zhihu Github 公众号:一本正经的瞎扯 一直以为vmselect 的 -search.denyPa ...
- ABP vNext系列文章04---DynamicClient动态代理
一.动态代理在ABP系统中的应用 1.它主要在做什么事件 之前开发系统想要在后台调用别的服务都是用HttpClient发起请求,在abp vnext中不需要我们这样做了, 你只要知道服务调用的接口方法 ...
- vim 从嫌弃到依赖(5)——普通模式的一些操作
通过前面几章内容的铺垫,基本已经介绍完了普通模式的大部分内容,按照进度下面会依次介绍插入模式.命令模式.选择模式的一些操作.根据不同模式提供功能的多少和使用频率,篇幅会有长有短.本来这篇文章应该介绍插 ...
- TienChin 渠道管理-更新渠道接口开发
ChannelController /** * 修改渠道 */ @PreAuthorize("hasPermission('tienchin:channel:edit')") @L ...
- 5.1 内存CRC32完整性检测
CRC校验技术是用于检测数据传输或存储过程中是否出现了错误的一种方法,校验算法可以通过计算应用与数据的循环冗余校验(CRC)检验值来检测任何数据损坏.通过运用本校验技术我们可以实现对特定内存区域以及磁 ...
- Yarp 与 Nginx性能大比拼不出所料它胜利了!
Yarp 与 Nginx 性能大比拼 测试环境: Ubuntu 22.04.3 LTS (GNU/Linux 6.5.0-14-generic x86_64) Intel(R) Xeon(R) CPU ...