OWIN 自宿主模式WebApi项目,WebApi层作为单独类库供OWIN调用
OWIN是Open Web Server Interface for .NET的首字母缩写,他的定义如下:
为什么我们需要OWIN
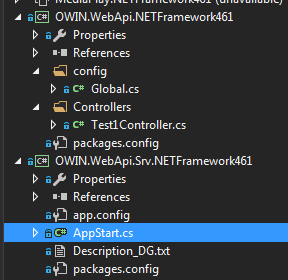
OWIN.WebApi WebApi层
OWIN.WebApi.Sv WebApi服务层,将要作为启动项!
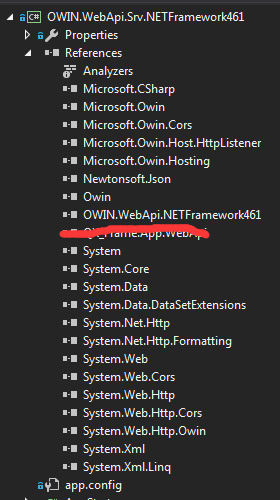
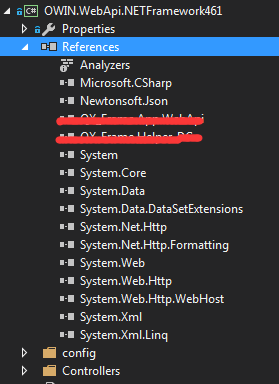
- using System.Web.Http;
- using System.Web.Http.Dispatcher;
- using System;
- using System.Collections.Concurrent;
- using System.Collections.Generic;
- using System.Linq;
- using System.Net;
- using System.Net.Http;
- using System.Web.Http.Controllers;
- namespace OWIN.WebApi.config
- {
- public class WebApiApplication : System.Web.HttpApplication
- {
- protected void Application_Start()
- {
- //ignore the xml return it`s setting let json return only
- GlobalConfiguration.Configuration.Formatters.JsonFormatter.SerializerSettings.ReferenceLoopHandling = Newtonsoft.Json.ReferenceLoopHandling.Ignore;
- GlobalConfiguration.Configuration.Formatters.Remove(GlobalConfiguration.Configuration.Formatters.XmlFormatter);
- GlobalConfiguration.Configuration.Services.Replace(typeof(IHttpControllerSelector),
- new WebApiControllerSelector(GlobalConfiguration.Configuration));
- }
- }
- /// <summary>
- /// the WebApiControllerSelector
- /// author:qixiao
- /// time:2017-1-31 19:24:32
- /// </summary>
- public class WebApiControllerSelector : DefaultHttpControllerSelector
- {
- private const string NamespaceRouteVariableName = "Namespace";
- private readonly HttpConfiguration _configuration;
- private readonly Lazy<ConcurrentDictionary<string, Type>> _apiControllerCache;
- public WebApiControllerSelector(HttpConfiguration configuration)
- : base(configuration)
- {
- _configuration = configuration;
- _apiControllerCache = new Lazy<ConcurrentDictionary<string, Type>>(
- new Func<ConcurrentDictionary<string, Type>>(InitializeApiControllerCache));
- }
- private ConcurrentDictionary<string, Type> InitializeApiControllerCache()
- {
- IAssembliesResolver assembliesResolver = this._configuration.Services.GetAssembliesResolver();
- var types = this._configuration.Services.GetHttpControllerTypeResolver()
- .GetControllerTypes(assembliesResolver).ToDictionary(t => t.FullName, t => t);
- return new ConcurrentDictionary<string, Type>(types);
- }
- public IEnumerable<string> GetControllerFullName(HttpRequestMessage request, string controllerName)
- {
- object namespaceName;
- var data = request.GetRouteData();
- IEnumerable<string> keys = _apiControllerCache.Value.ToDictionary<KeyValuePair<string, Type>, string, Type>(t => t.Key,
- t => t.Value, StringComparer.CurrentCultureIgnoreCase).Keys.ToList();
- if (!data.Values.TryGetValue(NamespaceRouteVariableName, out namespaceName))
- {
- return from k in keys
- where k.EndsWith(string.Format(".{0}{1}", controllerName,
- DefaultHttpControllerSelector.ControllerSuffix), StringComparison.CurrentCultureIgnoreCase)
- select k;
- }
- string[] namespaces = (string[])namespaceName;
- return from n in namespaces
- join k in keys on string.Format("{0}.{1}{2}", n, controllerName,
- DefaultHttpControllerSelector.ControllerSuffix).ToLower() equals k.ToLower()
- select k;
- }
- public override HttpControllerDescriptor SelectController(HttpRequestMessage request)
- {
- Type type;
- if (request == null)
- {
- throw new ArgumentNullException("request");
- }
- string controllerName = this.GetControllerName(request);
- if (string.IsNullOrEmpty(controllerName))
- {
- throw new HttpResponseException(request.CreateErrorResponse(HttpStatusCode.NotFound,
- string.Format("No route providing a controller name was found to match request URI '{0}'", new object[] { request.RequestUri })));
- }
- IEnumerable<string> fullNames = GetControllerFullName(request, controllerName);
- )
- {
- throw new HttpResponseException(request.CreateErrorResponse(HttpStatusCode.NotFound,
- string.Format("No route providing a controller name was found to match request URI '{0}'", new object[] { request.RequestUri })));
- }
- if (this._apiControllerCache.Value.TryGetValue(fullNames.First(), out type))
- {
- return new HttpControllerDescriptor(_configuration, controllerName, type);
- }
- throw new HttpResponseException(request.CreateErrorResponse(HttpStatusCode.NotFound,
- string.Format("No route providing a controller name was found to match request URI '{0}'", new object[] { request.RequestUri })));
- }
- }
- }
4、在OWIN.WebApi.Srv层里面新建AppStart.cs类,并且写如下代码:
- using Microsoft.Owin.Hosting;
- using System;
- using Owin;
- using System.Web.Http;
- using System.Web.Http.Dispatcher;
- using QX_Frame.App.WebApi.Extends;
- using System.Web.Http.Cors;
- namespace OWIN.WebApi.Srv
- {
- class AppStart
- {
- static void Main(string[] args)
- {
- //string baseAddress = "http://localhost:3999/"; //localhost visit
- string baseAddress = "http://+:3999/"; //all internet environment visit
- try
- {
- WebApp.Start<StartUp>(url: baseAddress);
- Console.WriteLine("BaseIpAddress is " + baseAddress);
- Console.WriteLine("\nApplication Started !");
- }
- catch (Exception ex)
- {
- Console.WriteLine(ex.ToString());
- }
- for (;;)
- {
- Console.ReadLine();
- }
- }
- }
- //the start up configuration
- class StartUp
- {
- public void Configuration(IAppBuilder appBuilder)
- {
- HttpConfiguration config = new HttpConfiguration();
- // Web API configuration and services
- //跨域配置 //need reference from nuget
- config.EnableCors(new EnableCorsAttribute("*", "*", "*"));
- //enabing attribute routing
- config.MapHttpAttributeRoutes();
- // Web API Convention-based routing.
- config.Routes.MapHttpRoute(
- name: "DefaultApi",
- routeTemplate: "api/{controller}/{id}",
- defaults: new { id = RouteParameter.Optional },
- namespaces: new string[] { "OWIN.WebApi" }
- );
- config.Services.Replace(typeof(IHttpControllerSelector), new OWIN.WebApi.config.WebApiControllerSelector(config));
- //if config the global filter input there need not write the attributes
- //config.Filters.Add(new App.Web.Filters.ExceptionAttribute_DG());
- //new ClassRegisters(); //register ioc menbers
- appBuilder.UseWebApi(config);
- }
- }
- }
里面对地址进行了配置,当然可以根据需求自行配置,显示信息也进行了适当的展示,需要说明的一点是,我这里进行了跨域的配置,没有配置或者是不需要的请注释掉并忽略!
这里需要注意的是第53行,这里引用的是刚才的OWIN.WebApi层的Global.cs里面的类,请对照上述两段代码进行查找。
- using QX_Frame.App.WebApi;
- using QX_Frame.Helper_DG;
- using System.Web.Http;
- namespace OWIN.WebApi
- {
- /*
- * author:qixiao
- * time:2017-2-27 10:32:57
- **/
- public class Test1Controller:ApiController
- {
- //access http://localhost:3999/api/Test1 get method
- public IHttpActionResult GetTest()
- {
- //throw new Exception_DG("login id , pwd", "argumets can not be null", 11111, 2222);
- return Json(new { IsSuccess = true, Msg = "this is get method" });
- }
- //access http://localhost:3999/api/Test1 post method
- public IHttpActionResult PostTest(dynamic queryData)
- {
- return Json(new { IsSuccess = true, Msg = "this is post method",Data=queryData });
- }
- //access http://localhost:3999/api/Test1 put method
- public IHttpActionResult PutTest()
- {
- return Json(new { IsSuccess = true, Msg = "this is put method" });
- }
- //access http://localhost:3999/api/Test1 delete method
- public IHttpActionResult DeleteTest()
- {
- return Json(new { IsSuccess = true, Msg = "this is delete method" });
- }
- }
- }
这里我是用的是RESTFull风格的WebApi控制器接口。
然后我们可以进行试运行:
服务启动成功!
测试通过,我们可以尽情地探索后续开发步骤!
OWIN 自宿主模式WebApi项目,WebApi层作为单独类库供OWIN调用的更多相关文章
- webapi从入门到放弃(一)OWIN 自寄宿模式
1.创建web空项目 2.创建完如图 3.安装如下程序包Microsoft.AspNet.WebApi.Core (5.2.4)Microsoft.Owin.Host.SystemWeb (4.0. ...
- .Net Core3.0 WebApi 项目框架搭建 五:仓储模式
.Net Core3.0 WebApi 项目框架搭建:目录 理论介绍 仓储(Respository)是存在于工作单元和数据库之间单独分离出来的一层,是对数据访问的封装.其优点: 1)业务层不需要知道它 ...
- .Net Core3.0 WebApi 项目框架搭建 五: 轻量型ORM+异步泛型仓储
.Net Core3.0 WebApi 项目框架搭建:目录 SqlSugar介绍 SqlSugar是国人开发者开发的一款基于.NET的ORM框架,是可以运行在.NET 4.+ & .NET C ...
- Restful WebApi项目开发实践
前言 踩过了一段时间的坑,现总结一下,与大家分享,愿与大家一起讨论. Restful WebApi特点 WebApi相较于Asp.Net MVC/WebForm开发的特点就是前后端完全分离,后端使用W ...
- Asp.net WebApi 项目示例(增删改查)
1.WebApi是什么 ASP.NET Web API 是一种框架,用于轻松构建可以由多种客户端(包括浏览器和移动设备)访问的 HTTP 服务.ASP.NET Web API 是一种用于在 .NET ...
- Angularjs,WebAPI 搭建一个简易权限管理系统 —— WebAPI项目主体结构(四)
目录 前言 Angularjs名词与概念 Angularjs 基本功能演示 系统业务与实现 WebAPI项目主体结构 Angularjs 前端主体结构 5.0 WebAPI项目主体结构 5.1 总体结 ...
- SNF快速开发平台MVC-EasyUI3.9之-WebApi和MVC-controller层接收的json字符串的取值方法和调用后台服务方法
最近项目组很多人问我,从前台页面传到后台controller控制层或者WebApi 时如何取值和运算操作. 今天就都大家一个在框架内一个取值技巧 前台JS调用代码: 1.下面是选中一行数据后右键点击时 ...
- c#搭建webapi项目
一.添加WebApi项目 二.nuget下载WebApi所需的类库引用 install-package Microsoft.AspNet.WebApi install-package Micr ...
- .Net Core3.0 WebApi 项目框架搭建:目录
一.目录 .Net Core3.0 WebApi 项目框架搭建 一:实现简单的Resful Api .Net Core3.0 WebApi 项目框架搭建 二:API 文档神器 Swagger .Net ...
随机推荐
- javascript的getter和setter(转)
显然这是一个无关IE(高级IE除外)的话题,尽管如此,有兴趣的同学还是一起来认识一下ECMAScript5标准中getter和setter的实现.在一个对象中,操作其中的属性或方法,通常运用最多的就是 ...
- React组件实现越级传递属性
如果有这样一个结构:三级嵌套,分别是:一级父组件.二级子组件.三级孙子组件,且前者包含后者,结构如图: 如果把一个属性,比如color,从一级传递给三级,一般做法是使用props逐一向下传递,代码如下 ...
- 【linux 爱好者群】程序猿的那些聊天记录
分享&&交流&&开放 you should get it 声明:好吧,我们的群只有5个人,但是有句话不是说的很对吗,一个项目最理想的不就是5个人么.我是写文本那个. 下 ...
- wpf之StackPanel、WrapPanel、WrapPanel之间的关系
一.StackPanel StackPanel是以堆叠的方式显示其中的控件 1.可以使用Orientation属性更改堆叠的顺序分为水平方向(Orientation="Horizontal& ...
- 百度开源上传组件WebUploader的formData动态传值技巧
基于Web页面的文件上传一直是互联网应用开发中避免不了的,从asp时代的AspUpload组件.到asp无组件上传,到.Net时代的FileUpload,再到HTML5时代的各种基于jQuery的上传 ...
- 【lucene系列学习】排序
用lucene3实现搜索多字段并排序功能(设置权重)
- 今天打补丁出问题了,害得我组长被扣了1k奖金。
今天是第三次给mxdw打补丁和打包,外加公司高管说有一个东西必须要今天之内搞定外放. 我当时问策划为什么这么着急?策划说大佬决定的(这种做事方式真的很不习惯).我等屁民加班加点的搞事情,把功能搞出去了 ...
- 在Windows上安装MongoDB
原文官方文档:https://docs.mongodb.org/v2.6/tutorial/install-mongodb-on-windows/ 基于版本:MongoDB 2.6 概览 通过这个示例 ...
- winXP/win7/win10系统关闭445端口方法全攻略
近日有多个高校发布了关于连接校园网的电脑大面积中勒索病毒的消息,这种病毒致使许多高校毕业生的毕业论文(设计)被锁.受害机器的磁盘文件会被篡改为相应的后缀,图片.文档.视频.压缩包等各类资料都无法正常打 ...
- java内存模型6-final
与前面介绍的锁和volatile相比较,对final域的读和写更像是普通的变量访问.对于final域,编译器和处理器要遵守两个重排序规则: 在构造函数内对一个final域的写入,与随后把这个被构造对象 ...