iOS tableView Section圆角方案
给tableView的section设置圆角
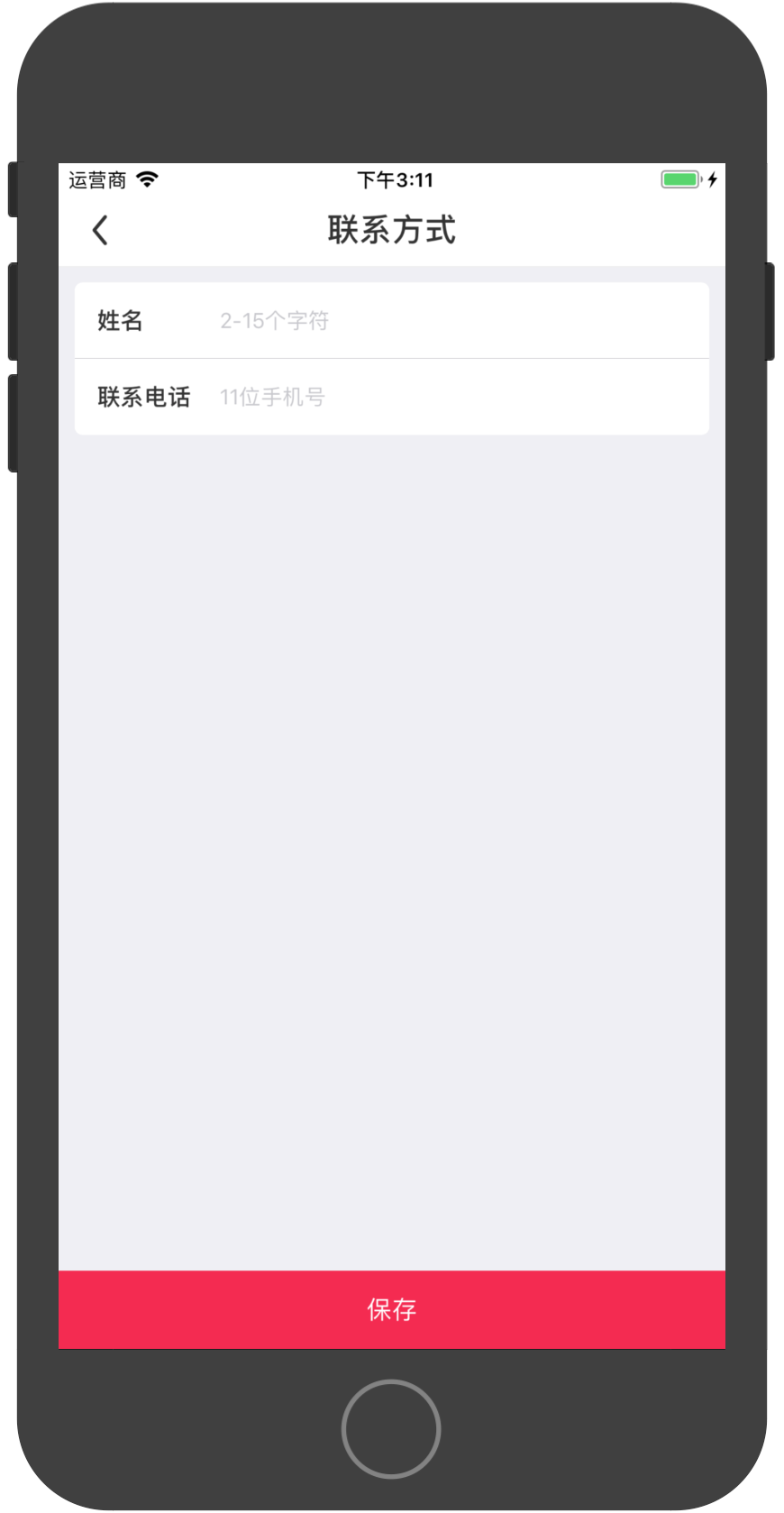
- 首先给让cell左右偏移一点的距离,通过重写cell的setframe方法来实现
-(void)setFrame:(CGRect)frame{
CGFloat margin = 10;
frame.origin.x = margin;
frame.size.width = SCREEN_WIDTH - margin*2;
[super setFrame:frame];
}
- 实现tableView的willDisplayCell方法,给section绘制圆角
- (void)tableView:(UITableView *)tableView willDisplayCell:(UITableViewCell *)cell forRowAtIndexPath:(NSIndexPath *)indexPath
{
if ([cell respondsToSelector:@selector(tintColor)]) {
if (tableView == self.tableView) {
// 圆角弧度半径
CGFloat cornerRadius = 5.f;
// 设置cell的背景色为透明,如果不设置这个的话,则原来的背景色不会被覆盖
cell.backgroundColor = UIColor.clearColor;
// 创建一个shapeLayer
CAShapeLayer *layer = [[CAShapeLayer alloc] init];
CAShapeLayer *backgroundLayer = [[CAShapeLayer alloc] init]; //显示选中
// 创建一个可变的图像Path句柄,该路径用于保存绘图信息
CGMutablePathRef pathRef = CGPathCreateMutable();
// 获取cell的size
CGRect bounds = CGRectInset(cell.bounds, 0, 0);
// CGRectGetMinY:返回对象顶点坐标
// CGRectGetMaxY:返回对象底点坐标
// CGRectGetMinX:返回对象左边缘坐标
// CGRectGetMaxX:返回对象右边缘坐标
// 这里要判断分组列表中的第一行,每组section的第一行,每组section的中间行
BOOL addLine = NO;
// CGPathAddRoundedRect(pathRef, nil, bounds, cornerRadius, cornerRadius);
if (indexPath.row == 0) {
// 初始起点为cell的左下角坐标
CGPathMoveToPoint(pathRef, nil, CGRectGetMinX(bounds), CGRectGetMaxY(bounds));
// 起始坐标为左下角,设为p1,(CGRectGetMinX(bounds), CGRectGetMinY(bounds))为左上角的点,设为p1(x1,y1),(CGRectGetMidX(bounds), CGRectGetMinY(bounds))为顶部中点的点,设为p2(x2,y2)。然后连接p1和p2为一条直线l1,连接初始点p到p1成一条直线l,则在两条直线相交处绘制弧度为r的圆角。
CGPathAddArcToPoint(pathRef, nil, CGRectGetMinX(bounds), CGRectGetMinY(bounds), CGRectGetMidX(bounds), CGRectGetMinY(bounds), cornerRadius);
CGPathAddArcToPoint(pathRef, nil, CGRectGetMaxX(bounds), CGRectGetMinY(bounds), CGRectGetMaxX(bounds), CGRectGetMidY(bounds), cornerRadius);
// 终点坐标为右下角坐标点,把绘图信息都放到路径中去,根据这些路径就构成了一块区域了
CGPathAddLineToPoint(pathRef, nil, CGRectGetMaxX(bounds), CGRectGetMaxY(bounds));
addLine = YES;
} else if (indexPath.row == [tableView numberOfRowsInSection:indexPath.section]-1) {
// 初始起点为cell的左上角坐标
CGPathMoveToPoint(pathRef, nil, CGRectGetMinX(bounds), CGRectGetMinY(bounds));
CGPathAddArcToPoint(pathRef, nil, CGRectGetMinX(bounds), CGRectGetMaxY(bounds), CGRectGetMidX(bounds), CGRectGetMaxY(bounds), cornerRadius);
CGPathAddArcToPoint(pathRef, nil, CGRectGetMaxX(bounds), CGRectGetMaxY(bounds), CGRectGetMaxX(bounds), CGRectGetMidY(bounds), cornerRadius);
// 添加一条直线,终点坐标为右下角坐标点并放到路径中去
CGPathAddLineToPoint(pathRef, nil, CGRectGetMaxX(bounds), CGRectGetMinY(bounds));
} else {
// 添加cell的rectangle信息到path中(不包括圆角)
CGPathAddRect(pathRef, nil, bounds);
addLine = YES;
}
// 把已经绘制好的可变图像路径赋值给图层,然后图层根据这图像path进行图像渲染render
layer.path = pathRef;
backgroundLayer.path = pathRef;
// 注意:但凡通过Quartz2D中带有creat/copy/retain方法创建出来的值都必须要释放
CFRelease(pathRef);
// 按照shape layer的path填充颜色,类似于渲染render
// layer.fillColor = [UIColor colorWithWhite:1.f alpha:0.8f].CGColor;
layer.fillColor = [UIColor whiteColor].CGColor;
// 添加分隔线图层
if (addLine == YES) {
CALayer *lineLayer = [[CALayer alloc] init];
CGFloat lineHeight = (1.f / [UIScreen mainScreen].scale);
lineLayer.frame = CGRectMake(CGRectGetMinX(bounds), bounds.size.height-lineHeight, bounds.size.width, lineHeight);
// 分隔线颜色取自于原来tableview的分隔线颜色
lineLayer.backgroundColor = tableView.separatorColor.CGColor;
[layer addSublayer:lineLayer];
}
// view大小与cell一致
UIView *roundView = [[UIView alloc] initWithFrame:bounds];
// 添加自定义圆角后的图层到roundView中
[roundView.layer insertSublayer:layer atIndex:0];
roundView.backgroundColor = UIColor.clearColor;
//cell的背景view
//cell.selectedBackgroundView = roundView;
cell.backgroundView = roundView;
//以上方法存在缺陷当点击cell时还是出现cell方形效果,因此还需要添加以下方法
UIView *selectedBackgroundView = [[UIView alloc] initWithFrame:bounds];
backgroundLayer.fillColor = tableView.separatorColor.CGColor;
[selectedBackgroundView.layer insertSublayer:backgroundLayer atIndex:0];
selectedBackgroundView.backgroundColor = UIColor.clearColor;
cell.selectedBackgroundView = selectedBackgroundView;
}
}
}
iOS tableView Section圆角方案的更多相关文章
- UITableView section 圆角 阴影
在UITableView实现图片上面的效果,百度一下看了别人的实现方案有下面2种: 1.UITableView section里面嵌套UITableView然后在上面实现圆角和阴影, 弊端代码超 ...
- [iOS]UIImageView增加圆角
[iOS]UIImageView增加圆角 "如何给一个UIImageView增加圆角?有几种方法?各自区别?" 备注:本文参考自http://www.jianshu.com/p/d ...
- iOS tableview cell 的展开收缩
iOS tableview cell 的展开收缩 #import "ViewController.h" @interface ViewController ()<UITabl ...
- iOS tableview的常用delegate和dataSource执行顺序
在这次项目中遇到了一个特别奇葩的问题:表视图创建的cell在7以上的系统能正常运行显示,在模拟器上就不能正常实现......为解决这个问题,纠结了好久...... 对在7系统上不显示的猜测: 用mas ...
- iOS 中的 HotFix 方案总结详解
相信HotFix大家应该都很熟悉了,今天主要对于最近调研的一些方案做一些总结.iOS中的HotFix方案大致可以分为四种: WaxPatch(Alibaba) Dynamic Framework(Ap ...
- ios tableview 上加 textfiled
ios tableview 上加 textfiled 首先附上我项目中用曾经用到的几张图 并说明一下我的用法: 图1: 图2: 图3: 心在你我说一下 我当初的实现 方法 ,希望能给你们一些 启 ...
- iOS TableView多级列表
代码地址如下:http://www.demodashi.com/demo/15006.html 效果预览 ### 一.需求 TableView多级列表:分级展开或合并,逐级获取并展示其子级数据,可以设 ...
- iOS TableView如何刷新指定的cell或section
指定的section单独刷新 NSIndexSet *indexSet=[[NSIndexSet alloc]initWithIndex:indexPath.row]; [tableview relo ...
- iOS tableview 优化总结
根据网络上的优化方法进行了总括.并未仔细进行语言组织.正在这些优化方法进行学习,见另一篇文章 提高app流畅度 1.cell子控件创建写在 initWithStyle:reuseIdentifier ...
随机推荐
- Python的扩展接口[2] -> 动态链接库DLL[0] -> 动态链接库及辅助工具
动态链接库 / Dynamic Link Library 目录 动态链接库简介 函数封装DLL 组件对象模型COM 如何判断.dll文件是COM还是DLL 辅助工具 1 动态链接库简介 / DLL I ...
- bean装配--auto
1,Dao package com.songyan.autoZhuangpei; public interface UserDao { public void say(); } package com ...
- Ubuntu中彻底修改用户名及密码
转自:http://blog.csdn.net/sailor201211/article/details/52305591 方案二:修改与用户和组相关的配置文件 这种方法更加本质,直接修改与用户和组相 ...
- tensorflow seq2seq.py接口实例
以简单英文问答问题为例测试tensorflow1.4 tf.contrib.legacy_seq2seq中seq2seq文件的几个seq2seq接口 github:https://github.com ...
- leetcode题解:Construct Binary Tree from Inorder and Postorder Traversal(根据中序和后序遍历构造二叉树)
题目: Given inorder and postorder traversal of a tree, construct the binary tree. Note:You may assume ...
- pip virtualenv requirements
pip可以很方便的安装.卸载和管理Python的包.virtualenv则可以建立多个独立的虚拟环境,各个环境中拥有自己的python解释器和各自的package包,互不影响.pip和virtuale ...
- jquery给多个span赋值
因为我想在页面载入完毕后,有几个地方显示当前时间,所以我须要给多个span赋值. span代码的写法例如以下: <span name="currentDate">< ...
- WEB接口测试之Jmeter接口测试自动化 (三)(数据驱动测试) 接口测试与数据驱动
转载:http://www.cnblogs.com/chengtch/p/6576117.html 1简介 数据驱动测试,即是分离测试逻辑与测试数据,通过如excel表格的形式来保存测试数据,用测试脚 ...
- iOS音乐后台播放及锁屏信息显示
实现音乐的后台播放.以及播放时,能够控制其暂停,下一首等操作,以及锁屏图片歌曲名等的显示 此实例须要真机调试.效果图例如以下: project下载:githubproject下载 实现步骤: 1.首先 ...
- eclipse黄色警告(finally block does not complete normally) ,不建议在finally中使用return语句
在eclipse中编写例如以下的代码,eclipse会给出黄色告警:finally block does not complete normally. public class Test { publ ...