Jungle Roads_hdu_1301(prim算法)
Jungle Roads
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 3614 Accepted Submission(s): 2609
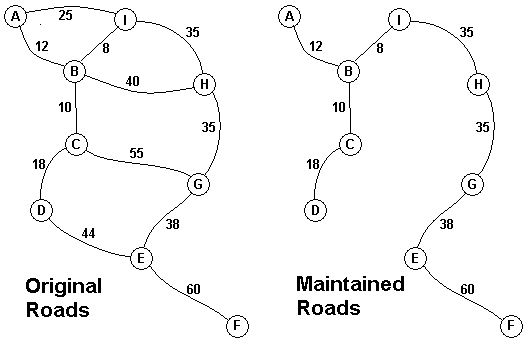
The Head Elder of the tropical island of Lagrishan has a problem. A burst of foreign aid money was spent on extra roads between villages some years ago. But the jungle overtakes roads relentlessly, so the large road network is too expensive to maintain. The Council of Elders must choose to stop maintaining some roads. The map above on the left shows all the roads in use now and the cost in aacms per month to maintain them. Of course there needs to be some way to get between all the villages on maintained roads, even if the route is not as short as before. The Chief Elder would like to tell the Council of Elders what would be the smallest amount they could spend in aacms per month to maintain roads that would connect all the villages. The villages are labeled A through I in the maps above. The map on the right shows the roads that could be maintained most cheaply, for 216 aacms per month. Your task is to write a program that will solve such problems.
The input consists of one to 100 data sets, followed by a final line containing only 0. Each data set starts with a line containing only a number n, which is the number of villages, 1 < n < 27, and the villages are labeled with the first n letters of the alphabet, capitalized. Each data set is completed with n-1 lines that start with village labels in alphabetical order. There is no line for the last village. Each line for a village starts with the village label followed by a number, k, of roads from this village to villages with labels later in the alphabet. If k is greater than 0, the line continues with data for each of the k roads. The data for each road is the village label for the other end of the road followed by the monthly maintenance cost in aacms for the road. Maintenance costs will be positive integers less than 100. All data fields in the row are separated by single blanks. The road network will always allow travel between all the villages. The network will never have more than 75 roads. No village will have more than 15 roads going to other villages (before or after in the alphabet). In the sample input below, the first data set goes with the map above.
The output is one integer per line for each data set: the minimum cost in aacms per month to maintain a road system that connect all the villages. Caution: A brute force solution that examines every possible set of roads will not finish within the one minute time limit.
A 2 B 12 I 25
B 3 C 10 H 40 I 8
C 2 D 18 G 55
D 1 E 44
E 2 F 60 G 38
F 0
G 1 H 35
H 1 I 35
3
A 2 B 10 C 40
B 1 C 20
0
30
import java.util.Scanner; public class Main{//prim算法,最优路径
private static int map[][];
private static boolean vis[];
private static int d[];
private static int MAXN=0x7ffffff;
public static void main(String[] args) {
Scanner input=new Scanner(System.in);
while(true){
int n=input.nextInt();
if(n==0)
break;
map=new int[n+1][n+1];
vis=new boolean[n+1];
d=new int[n+1];
for(int i=1;i<=n;i++)
for(int j=1;j<=n;j++)
map[i][j]=MAXN;
for(int k=1;k<n;++k){
String s=input.next();
int a=input.nextInt();
for(int i=0;i<a;i++){
String s1=input.next();
int b=input.nextInt();
int x=s.charAt(0)-'A'+1;
int y=s1.charAt(0)-'A'+1;
//System.out.println(x+"-----"+y);
map[x][y]=map[y][x]=b;
}
}
//prim算法
for(int i=1;i<=n;i++){
d[i]=map[1][i];
}
vis[1]=true;
d[1]=0;
int sum=0;
for(int i=2;i<=n;i++){
int min=MAXN;
int x=0,j;
for(j=1;j<=n;j++)
if(!vis[j]&&d[j]<min){
min=d[j];
x=j;
}
vis[x]=true;
sum+=min;
for(j=1;j<=n;j++){
if(!vis[j]&&d[j]>map[x][j]){
d[j]=map[x][j];
}
}
}
System.out.println(sum);
}
}
}
Jungle Roads_hdu_1301(prim算法)的更多相关文章
- 最小生成树问题------------Prim算法(TjuOj_1924_Jungle Roads)
遇到一道题,简单说就是找一个图的最小生成树,大概有两种常用的算法:Prim算法和Kruskal算法.这里先介绍Prim.随后贴出1924的算法实现代码. Prim算法 1.概览 普里姆算法(Prim算 ...
- 图的生成树(森林)(克鲁斯卡尔Kruskal算法和普里姆Prim算法)、以及并查集的使用
图的连通性问题:无向图的连通分量和生成树,所有顶点均由边连接在一起,但不存在回路的图. 设图 G=(V, E) 是个连通图,当从图任一顶点出发遍历图G 时,将边集 E(G) 分成两个集合 T(G) 和 ...
- 最小生成树のprim算法
Problem A Time Limit : 1000/1000ms (Java/Other) Memory Limit : 32768/32768K (Java/Other) Total Sub ...
- 数据结构代码整理(线性表,栈,队列,串,二叉树,图的建立和遍历stl,最小生成树prim算法)。。持续更新中。。。
//归并排序递归方法实现 #include <iostream> #include <cstdio> using namespace std; #define maxn 100 ...
- 最小生成树——prim算法
prim算法是选取任意一个顶点作为树的一个节点,然后贪心的选取离这棵树最近的点,直到连上所有的点并且不够成环,它的时间复杂度为o(v^2) #include<iostream>#inclu ...
- 洛谷 P3366 【模板】最小生成树 prim算法思路 我自己的实现
网上有很多prim算法 用邻接矩阵 加什么lowcost数组 我觉得不靠谱 毕竟邻接矩阵本身就不是存图的好方法 所以自己写了一个邻接表(边信息表)版本的 注意我还是用了优先队列 每次新加入一个点 ...
- 最小生成树算法——prim算法
prim算法:从某一点开始,去遍历相邻的边,然后将权值最短的边加入集合,同时将新加入边集中的新点遍历相邻的边更新边值集合(边值集合用来找出新的最小权值边),注意每次更新都需将cost数组中的点对应的权 ...
- 贪心算法-最小生成树Kruskal算法和Prim算法
Kruskal算法: 不断地选择未被选中的边中权重最轻且不会形成环的一条. 简单的理解: 不停地循环,每一次都寻找两个顶点,这两个顶点不在同一个真子集里,且边上的权值最小. 把找到的这两个顶点联合起来 ...
- Prim算法(三)之 Java详解
前面分别通过C和C++实现了普里姆,本文介绍普里姆的Java实现. 目录 1. 普里姆算法介绍 2. 普里姆算法图解 3. 普里姆算法的代码说明 4. 普里姆算法的源码 转载请注明出处:http:// ...
随机推荐
- PHP 博客收集
https://lvwenhan.com/ www.chrisyue.com https://silex.symfony.com/ https://www.chrisyue.com/translati ...
- js判断某年某月有多少天
function getCountDays(ym) { var curDate = new Date(ym); /* 获取当前月份 */ var curMonth = curDate.getMonth ...
- 回文树练习 Part1
URAL - 1960 Palindromes and Super Abilities 回文树水题,每次插入时统计数量即可. #include<bits/stdc++.h> using ...
- gp数据库运维
最近需要将一份db2导出的历史数据入库gp集群,然后把每天的增量数据导出成txt文件和对应的log日志,再ftp传输给另外一台机器.其中陆续碰到一些坑,在此记录 历史文件数据清洗 列分隔符的选择 碰到 ...
- APPKIT打造稳定、灵活、高效的运营配置平台
一.背景 美团App.大众点评App都是重运营的应用.对于App里运营资源.基础配置,需要根据城市.版本.平台.渠道等不同的维度进行运营管理.如何在版本快速迭代过程中,保持运营资源能够被高效.稳定和灵 ...
- SERVLET API中forward()与redirect()的区别?
前者仅是容器中控制权的转向,在客户端浏览器地址栏中不会显示出转向后的地址:后者则是完全的跳转,浏览器将会得到跳转的地址,并重新发送请求链接.这样,从浏览器的地址栏中可以看到跳转后的链接地址.所以,前者 ...
- 项目Alpha冲刺——代码规范、本次冲刺任务与计划
作业格式 课程名称:软件工程1916|W(福州大学) 作业要求:项目Alpha冲刺(团队) 团队名称: 那周余嘉熊掌将得队 作业目标:代码规范.本次冲刺任务与计划 团队信息: 队员学号 队员姓名 博客 ...
- HAproxy 代理技术原理探究
HAproxy 技术分享 简介 HAProxy是一款提供高可用性.负载均衡以及基于TCP(第四层)和HTTP(第七层)应用的代理软件 Features 1.免费 2.能够做到4层以上代理 3.高性能 ...
- Python Django 中的STATIC_URL 设置和使用解析
使用Django静态设置时,遇到很多问题,经过艰苦的Baidu, stack overflow, Django原档阅读,终于把静态图片给搞出来了.特记录下来. 关键的概念:Django中,静态资源的存 ...
- 线性表之顺序表C++实现
线性表之顺序表 一.头文件:SeqList.h //顺序线性表的头文件 #include<iostream> ; //定义顺序表SeqList的模板类 template<class ...