Prime Path(素数筛选+bfs)
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 9519 | Accepted: 5458 |
Description
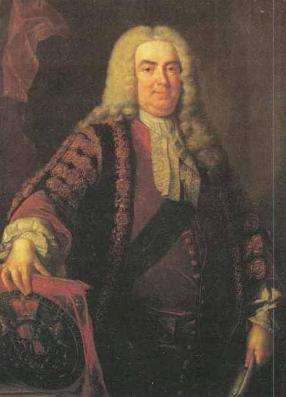
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033
1733
3733
3739
3779
8779
8179
The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
Input
Output
Sample Input
- 3
- 1033 8179
- 1373 8017
- 1033 1033
Sample Output
- 6
- 7
- 0
题意:给定两个四位数,求从前一个数变到后一个数最少需要几步,改变的原则是每次只能改变某一位上的一个数,而且每次改变得到的必须是一个素数;- 思路:将四位数以内的素数筛选出来,bfs时,枚举四位数的每一位上的每一个数;
- #include<stdio.h>
- #include<string.h>
- #include<queue>
- using namespace std;
- struct node
- {
- int num;
- int step;
- };
- queue<struct node>que;
- int n,m,flag;
- bool p[],vis[];
- //素数筛;
- void prime_search()
- {
- memset(p,,sizeof(p));
- for(int i = ; i <= ; i+=)
- p[i] = ;
- for(int i = ; i <= ; i++)
- {
- if(p[i])
- {
- for(int j = i+i; j <= ; j += i)
- p[j] = ;
- }
- }
- }
- int bfs()
- {
- while(!que.empty())
- que.pop();
- que.push((struct node){n,});
- vis[n] = ;
- int res,tmp,r,t,s;
- while(!que.empty())
- {
- struct node u = que.front();
- que.pop();
- if(u.num == m)
- return u.step;
- //枚举个位数
- r = u.num%;
- for(int k = -; k <= ; k++)
- {
- t = r+k;
- if(t >= && t <= )
- {
- res = (u.num/)*+t;
- if(p[res] && !vis[res])
- {
- que.push((struct node){res,u.step+});
- vis[res] = ;
- }
- }
- }
- //枚举十位数
- tmp = u.num/;
- r = tmp%;
- s = tmp/;
- for(int k = -; k <= ; k++)
- {
- t = r+k;
- if(t >= && t <= )
- {
- res = (s*+t)*+u.num%;
- if(p[res] && !vis[res])
- {
- que.push((struct node){res,u.step+});
- vis[res] = ;
- }
- }
- }
- //枚举百位数
- int tmp = u.num/;
- r = tmp%;
- s = tmp/;
- for(int k = -; k <= ; k++)
- {
- t = r+k;
- if(t >= && t <= )
- {
- res = (s*+t)*+u.num%;
- if(p[res] && !vis[res])
- {
- que.push((struct node){res,u.step+});
- vis[res] = ;
- }
- }
- }
- //枚举千位数
- r = u.num/;
- for(int k = -; k <= ; k++)
- {
- t = r+k;
- if(t > && t <= )
- {
- res = t*+u.num%;
- if(p[res] && !vis[res])
- {
- que.push((struct node){res,u.step+});
- vis[res] = ;
- }
- }
- }
- }
- }
- int main()
- {
- int test;
- prime_search();
- scanf("%d",&test);
- while(test--)
- {
- memset(vis,,sizeof(vis));
- scanf("%d %d",&n,&m);
- int ans = bfs();
- printf("%d\n",ans);
- }
- return ;
- }
Prime Path(素数筛选+bfs)的更多相关文章
- POJ - 3126 Prime Path 素数筛选+BFS
Prime Path The ministers of the cabinet were quite upset by the message from the Chief of Security s ...
- Prime Path素数筛与BFS动态规划
埃拉托斯特尼筛法(sieve of Eratosthenes ) 是古希腊数学家埃拉托斯特尼发明的计算素数的方法.对于求解不大于n的所有素数,我们先找出sqrt(n)内的所有素数p1到pk,其中k = ...
- poj3126 Prime Path 广搜bfs
题目: The ministers of the cabinet were quite upset by the message from the Chief of Security stating ...
- ZOJ 1842 Prime Distance(素数筛选法2次使用)
Prime Distance Time Limit: 2 Seconds Memory Limit: 65536 KB The branch of mathematics called nu ...
- POJ 3126 Prime Path 素数筛,bfs
题目: http://poj.org/problem?id=3126 困得不行了,没想到敲完一遍直接就A了,16ms,debug环节都没进行.人品啊. #include <stdio.h> ...
- POJ2689 - Prime Distance(素数筛选)
题目大意 给定两个数L和U,要求你求出在区间[L, U] 内所有素数中,相邻两个素数差值最小的两个素数C1和C2以及相邻两个素数差值最大的两个素数D1和D2,并且L-U<1,000,000 题解 ...
- POJ 3126 - Prime Path - [线性筛+BFS]
题目链接:http://poj.org/problem?id=3126 题意: 给定两个四位素数 $a,b$,要求把 $a$ 变换到 $b$.变换的过程每次只能改动一个数,要保证每次变换出来的数都是一 ...
- HDOJ(HDU) 2136 Largest prime factor(素数筛选)
Problem Description Everybody knows any number can be combined by the prime number. Now, your task i ...
- Prime Path[POJ3126] [SPFA/BFS]
描述 孤单的zydsg又一次孤单的度过了520,不过下一次不会再这样了.zydsg要做些改变,他想去和素数小姐姐约会. 所有的路口都被标号为了一个4位素数,zydsg现在的位置和素数小姐姐的家也是这样 ...
随机推荐
- 亲测apache
http://www.cnblogs.com/bluewelkin/p/3805107.html 里面是纠正了原文的一些小错误,即可正常安装 1.su 命令 2.安装apr-1.3.5.tar.gz ...
- 高效 css 整理
避免通用规则 请确保规则不以通用类型作为结束! 不要用标签名或 classes 来限制 ID 规则 如果规则的关键选择器为 ID 选择器,则没有必要为规则增加标签名.因为 ID 是唯一的,增加标签只会 ...
- PHP利用超级全局变量$_POST来接收表单数据。
利用$_POST超级全局变量接收表单的数据,然后利用echo输出到页面. 下面是代码: <!doctype html> <html> <head> <titl ...
- rabbitmq 消息持久化之receive and send
二: 任务分发 &消息持久化 启用多个接收端的时候如果某一个receive 关闭要保证消息有反馈是否收到 send端 #-*- coding: UTF-8 -*-import pika ...
- css 实现页面加载中等待效果
<!DOCTYPE html> <html> <head> <title>css实现页面加载中,请稍候效果</title> <meta ...
- js中浮点型运算 注意点
先看张图: 这是一个JS浮点数运算Bug,导致我树状图,数据合计不正确,,,,,,两个小数相加,出来那么多位小数 (这是修该之后的) 网上找到以下解决方式: 方法一:有js自定义函数 <sc ...
- 函数对象的prototype总结
通过看 http://www.cnblogs.com/mindsbook/archive/2009/09/19/javascriptYouMustKnowPrototype.html 该文章和对代码的 ...
- c# 连接oracle 读取数据
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; usin ...
- 用html/css做的一个登入小界面(图片瀑布流)
一个登入效果简易图:(色彩搭配有点乱,嘻嘻,可以在代码处改成自己喜欢的颜色) css样式的代码: style.css: @charset "utf-8";/* CSS Docume ...
- C++ cout cerr 和 clog 的区别
我们都知道C++预定义了cin(标准输入流)和cout(标准输出流).但今天突然又蹦出来两个cerr(标准错误流(非缓冲))和clog(标准错误流(缓冲)),本着学习提高的态度在网上搜索了相关内容,下 ...