《挑战程序设计竞赛》2.2 贪心法-区间 POJ2376 POJ1328 POJ3190
POJ2376
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 14585 | Accepted: 3718 |
Description
the last being shift T.
Each cow is only available at some interval of times during the day for work on cleaning. Any cow that is selected for cleaning duty will work for the entirety of her interval.
Your job is to help Farmer John assign some cows to shifts so that (i) every shift has at least one cow assigned to it, and (ii) as few cows as possible are involved in cleaning. If it is not possible to assign a cow to each shift, print -1.
Input
* Lines 2..N+1: Each line contains the start and end times of the interval during which a cow can work. A cow starts work at the start time and finishes after the end time.
Output
Sample Input
3 10
1 7
3 6
6 10
Sample Output
2
Hint
INPUT DETAILS:
There are 3 cows and 10 shifts. Cow #1 can work shifts 1..7, cow #2 can work shifts 3..6, and cow #3 can work shifts 6..10.
OUTPUT DETAILS:
By selecting cows #1 and #3, all shifts are covered. There is no way to cover all the shifts using fewer than 2 cows.
Source
题意
John有N头牛,其土地的耕作时间是1-T,给出每头牛的工作区间,问最少需要几头牛可以满足全部耕作时间都至少有一头牛在工作。
思路
标准区间贪心,先按照每头牛的开始时间排序,每次选开始时间小于未覆盖区间的开始时间的牛中,结束时间最大的那个。
代码
Source Code Problem: 2376 User: liangrx06
Memory: 1308K Time: 360MS
Language: C++ Result: Accepted
Source Code
#include <iostream>
#include <cstdio>
#include <set>
#include <algorithm>
using namespace std; typedef pair<int, int> P;
struct cmp {
bool operator()(const P& a, const P& b)const {
return a.first < b.first;
}
};
typedef set<P, cmp> S; int n, t;
S s; void input()
{
scanf("%d%d", &n, &t);
s.clear();
int i, b, e;
S::iterator it;
for (i = 0; i < n; i ++) {
scanf("%d%d", &b, &e);
it = s.find(P(b, e));
if (it == s.end())
s.insert(P(b, e));
else if ((*it).second < e) {
s.erase(it);
s.insert(P(b, e));
}
}
//for (it = s.begin(); it != s.end(); it ++)
// cout << (*it).first << " " << (*it).second << endl;
} int solve()
{
int res = 0;
int begin = 1;
S::iterator it, it1;
while (s.size()) {
int end = 0;
for (it = s.begin(); it != s.end() && (*it).first <= begin; it ++) {
if ((*it).second > end) {
end = (*it).second;
it1 = it;
}
}
if (end == 0) return -1;
s.erase(it1);
begin = end + 1;
//printf("begin=%d\n", begin);
res ++;
if (begin > t)
return res;
}
return -1;
} int main(void)
{
input(); printf("%d\n", solve()); return 0;
}
POJ1328
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 67495 | Accepted: 15139 |
Description
sea can be covered by a radius installation, if the distance between them is at most d. We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the
sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
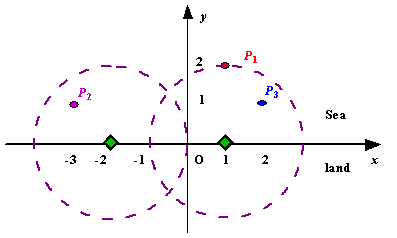
Input
two integers representing the coordinate of the position of each island. Then a blank line follows to separate the cases. The input is terminated by a line containing pair of zeros
Output
Sample Input
3 2
1 2
-3 1
2 1 1 2
0 2 0 0
Sample Output
Case 1: 2
Case 2: 1
Source
题意:
将一条海岸线看成X轴,X轴上面是大海,海上有若干岛屿,给出雷达的覆盖半径和岛屿的位置,要求在海岸线上建雷达,在雷达能够覆盖全部岛屿情况下,求雷达的最少使用量。
思路:
贪心法求解。先计算雷达覆盖区域与X轴的相交区间(如果不相交则此题解为-1)后,以做区间左值为依据排序。
而后贪心法选择雷达所建位置,下一个雷达建的位置是未覆盖岛屿中最小的区间右值,然后据此更新覆盖的岛屿。
代码:
<pre name="code" class="cpp">Source Code Problem: 1328 User: liangrx06
Memory: 244K Time: 47MS
Language: C++ Result: Accepted
Source Code
#include <iostream>
#include <cstdio>
#include <cmath>
#include <algorithm>
using namespace std; const int N = 1000; struct P {
double x, y;
double l, r;
}; int n;
double d;
P p[N]; int input()
{
cin >> n >> d;
if (!n && !d)
return 0;
int flag = 1;
for (int i = 0; i < n; i ++) {
cin >> p[i].x >> p[i].y;
double z = d*d - p[i].y*p[i].y;
if (z < 0) flag = -1;
z = sqrt(z);
p[i].l = p[i].x - z;
p[i].r = p[i].x + z;
}
if (d < 0) flag = -1;
return flag;
} bool cmp(const P &a, const P &b)
{
return a.l < b.l;
} int solve()
{
sort(p, p+n, cmp); int res = 0, i = 0;
double posL, posR;
while (i < n) {
posL = p[i].l;
posR = p[i].r;
i ++;
while ( i < n && p[i].l <= posR ) {
posL = p[i].l;
posR = (p[i].r < posR) ? p[i].r : posR;
i ++;
}
res ++;
}
return res;
} int main(void)
{
int c, flag, res; c = 0;
while ( flag = input() ) {
c ++;
res = -1;
if (flag > 0)
res = solve();
printf("Case %d: %d\n", c, res);
} return 0;
}
POJ3190
Time Limit: 1000MS | Memory Limit: 65536K | |||
Total Submissions: 4315 | Accepted: 1545 | Special Judge |
Description
stall each cow can be assigned for her milking time. Of course, no cow will share such a private moment with other cows. Help FJ by determining:
- The minimum number of stalls required in the barn so that each cow can have her private milking period
- An assignment of cows to these stalls over time
Many answers are correct for each test dataset; a program will grade your answer.
Input
Output
Sample Input
5
1 10
2 4
3 6
5 8
4 7
Sample Output
4
1
2
3
2
4
Hint
Here's a graphical schedule for this output:
Time 1 2 3 4 5 6 7 8 9 10 Stall 1 c1>>>>>>>>>>>>>>>>>>>>>>>>>>> Stall 2 .. c2>>>>>> c4>>>>>>>>> .. .. Stall 3 .. .. c3>>>>>>>>> .. .. .. .. Stall 4 .. .. .. c5>>>>>>>>> .. .. ..
Other outputs using the same number of stalls are possible.
Source
《挑战程序设计竞赛》2.2 贪心法-区间 POJ2376 POJ1328 POJ3190的更多相关文章
- 挑战程序设计竞赛》P345 观看计划
<挑战程序设计竞赛>P345 观看计划 题意:一周一共有M个单位的时间.一共有N部动画在每周si时 ...
- Aizu 2249Road Construction 单源最短路变形《挑战程序设计竞赛》模板题
King Mercer is the king of ACM kingdom. There are one capital and some cities in his kingdom. Amazin ...
- 《挑战程序设计竞赛》2.3 动态规划-优化递推 POJ1742 3046 3181
POJ1742 http://poj.org/problem?id=1742 题意 有n种面额的硬币,面额个数分别为Ai.Ci,求最多能搭配出几种不超过m的金额? 思路 据说这是传说中的男人8题呢,对 ...
- POJ 2386 Lake Counting 题解《挑战程序设计竞赛》
地址 http://poj.org/problem?id=2386 <挑战程序设计竞赛>习题 题目描述Description Due to recent rains, water has ...
- 《挑战程序设计竞赛》2.2 贪心法-其它 POJ3617 3069 3253 2393 1017 3040 1862 3262
POJ3617 Best Cow Line 题意 给定长度为N的字符串S,要构造一个长度为N的字符串T.起初,T是一个空串,随后反复进行下列任意操作: 从S的头部(或尾部)删除一个字符,加到T的尾部 ...
- poj 3253 Fence Repair 贪心 最小堆 题解《挑战程序设计竞赛》
地址 http://poj.org/problem?id=3253 题解 本题是<挑战程序设计>一书的例题 根据树中描述 所有切割的代价 可以形成一颗二叉树 而最后的代价总和是与子节点和深 ...
- poj 3069 Saruman's Army 贪心 题解《挑战程序设计竞赛》
地址 http://poj.org/problem?id=3069 题解 题目可以考虑贪心 尽可能的根据题意选择靠右边的点 注意 开始无标记点 寻找左侧第一个没覆盖的点 再来推算既可能靠右的标记点为一 ...
- poj 2431 Expedition 贪心 优先队列 题解《挑战程序设计竞赛》
地址 http://poj.org/problem?id=2431 题解 朴素想法就是dfs 经过该点的时候决定是否加油 中间加了一点剪枝 如果加油次数已经比已知最少的加油次数要大或者等于了 那么就剪 ...
- 挑战程序设计竞赛 P131 区间DP
书上好多题没补 PS.整个DP是根据Q来划分的,dalao的代码就是不一样啊 #include<bits/stdc++.h> #define rep(i,j,k) for(int i=j; ...
随机推荐
- lodash random
_.random([min=0], [max=1], [floating]) 产生一个包括 min 与 max 之间的数. 如果只提供一个参数返回一个0到提供数之间的数. 如果 floating 设为 ...
- redis源代码分析(5)——aof
前面几篇基本介绍了redis的主要功能.流程.接下来是一些相对独立的部分,首先看一下持久化. redis持久化支持两种方式:RDB和AOF,我们首先看一下AOF的实现. AOF(Append only ...
- 查看FC HBA卡信息的方法
在配置磁盘阵列或虚拟磁带库时,往往会以FC接口与主机对接,那么就涉及FC HBA卡的查看,本文就这个问题进行了总结与整理. 一.Windows 系统 在Windows系统中,可以使用FC HBA卡厂家 ...
- win7系统扩展双屏幕时,怎样在两个屏幕下都显示任务栏
扩展屏幕下都显示任务栏!!! win7系统本身无法设置该功能(眼下我是不知道) 但能够下载第三方软件来解决该问题. 第一步:Dual Monitor Taskbar 下载软件 下载链接:http:// ...
- oracle data file header replace(測)
SQL> create tablespace rm_tbs datafile 'f1.dbf' size 10m; Tablespace created. SQL> select file ...
- JDK自带监控工具 jps、jinfo、jstat、jmap、jconsole
分类: JVM 2010-10-04 11:05 587人阅读 评论(0) 收藏 举报 工具jdkjava远程连接unixstring 常用有五个命令行工具: jinfo: 可以输出并修改运行时的ja ...
- Spring 中bean的作用、定义
Spring 中bean的作用.定义: 创建一个bean定义,其实质是用该bean定义对应的类来创建真正实例的"配方(recipe)".把bean定义看成一个配方很有意义,它与cl ...
- systemd 对/home等目录的限制
systemd 默认配置了对/root /home等目录的限制 比如ProtectHome=true 意味着启动时应用对这些目录不可写,这是个大坑,谨记
- jQuery校验 表单验证
官网地址:http://bassistance.de/jquery-plugins/jquery-plugin-validation jQuery plugin: Validation 使用说明 转载 ...
- Vue 获取验证码倒计时组件
子组件 <template> <a class="getvalidate":class="{gray: (!stop)}"@click='cl ...