【转】RESTful Webservice创建
RESTful Web Services with Java
REST is an architectural style. HTTP is a protocol which contains the set of REST architectural constraints.
REST fundamentals
- Everything in REST is considered as a resource.
- Every resource is identified by an URI.
- Uses uniform interfaces. Resources are handled using POST, GET, PUT, DELETE operations which are similar to Create, Read, update and Delete(CRUD) operations.
- Be stateless. Every request is an independent request. Each request from client to server must contain all the information necessary to understand the request.
- Communications are done via representations. E.g. XML, JSON
RESTful Web Services
RESTful Web Services have embraced by large service providers across the web as an alternative to SOAP based Web Services due to its simplicity. This post will demonstrate how to create a RESTful Web Service and client using Jersey framework which extends JAX-RS API. Examples are done using Eclipse IDE and Java SE 6.
Creating RESTful Web Service
- In Eclipse, create a new dynamic web project called "RESTfulWS"

- Download Jersey zip bundle from here. Jersey version used in these examples is 1.17.1. Once you unzip it you'll have a directory called "jersey-archive-1.17.1". Inside it find the lib directory. Copy following jars from there and paste them inside WEB-INF -> lib folder in your project. Once you've done that, add those jars to your project build path as well.
- asm-3.1.jar
- jersey-client-1.17.1.jar
- jersey-core-1.17.1.jar
- jersey-server-1.17.1.jar
- jersey-servlet-1.17.1.jar
- jsr311-api-1.1.1.jar
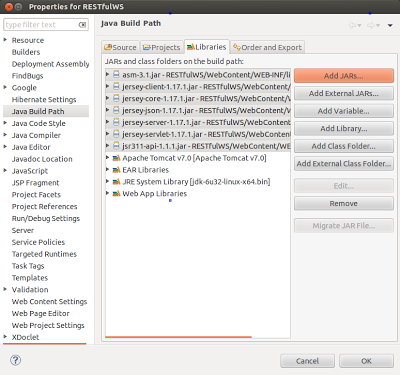

- In your project, inside Java Resources -> src create a new package called "com.eviac.blog.restws". Inside it create a new java class called "UserInfo". Also include the given web.xml file inside WEB-INF folder.
UserInfo.java
- package com.eviac.blog.restws;
- import javax.ws.rs.GET;
- import javax.ws.rs.Path;
- import javax.ws.rs.PathParam;
- import javax.ws.rs.Produces;
- import javax.ws.rs.core.MediaType;
- /**
- *
- * @author pavithra
- *
- */
- // @Path here defines class level path. Identifies the URI path that
- // a resource class will serve requests for.
- @Path("UserInfoService")
- public class UserInfo {
- // @GET here defines, this method will method will process HTTP GET
- // requests.
- @GET
- // @Path here defines method level path. Identifies the URI path that a
- // resource class method will serve requests for.
- @Path("/name/{i}")
- // @Produces here defines the media type(s) that the methods
- // of a resource class can produce.
- @Produces(MediaType.TEXT_XML)
- // @PathParam injects the value of URI parameter that defined in @Path
- // expression, into the method.
- public String userName(@PathParam("i") String i) {
- String name = i;
- return "<User>" + "<Name>" + name + "</Name>" + "</User>";
- }
- @GET
- @Path("/age/{j}")
- @Produces(MediaType.TEXT_XML)
- public String userAge(@PathParam("j") int j) {
- int age = j;
- return "<User>" + "<Age>" + age + "</Age>" + "</User>";
- }
- }
web.xml
- <?xml version="1.0" encoding="UTF-8"?>
- <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5">
- <display-name>RESTfulWS</display-name>
- <servlet>
- <servlet-name>Jersey REST Service</servlet-name>
- <servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class>
- <init-param>
- <param-name>com.sun.jersey.config.property.packages</param-name>
- <param-value>com.eviac.blog.restws</param-value>
- </init-param>
- <load-on-startup>1</load-on-startup>
- </servlet>
- <servlet-mapping>
- <servlet-name>Jersey REST Service</servlet-name>
- <url-pattern>/rest/*</url-pattern>
- </servlet-mapping>
- </web-app>
- To run the project, right click on it and click on run as ->run on server.
- Execute the following URL in your browser and you'll see the output.
- http://localhost:8080/RESTfulWS/rest/UserInfoService/name/Pavithra
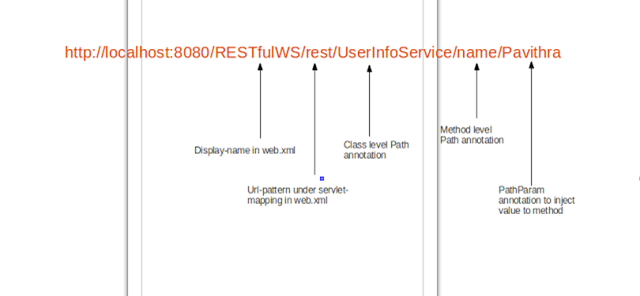
output
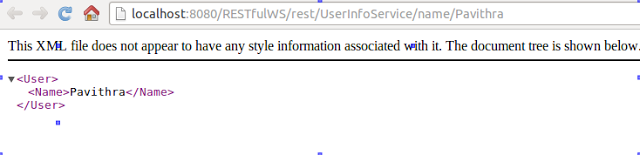
Creating Client
- Create a package called "com.eviac.blog.restclient". Inside it create a java class called "UserInfoClient".
UserInfoClient.java
- package com.eviac.blog.restclient;
- import javax.ws.rs.core.MediaType;
- import com.sun.jersey.api.client.Client;
- import com.sun.jersey.api.client.ClientResponse;
- import com.sun.jersey.api.client.WebResource;
- import com.sun.jersey.api.client.config.ClientConfig;
- import com.sun.jersey.api.client.config.DefaultClientConfig;
- /**
- *
- * @author pavithra
- *
- */
- public class UserInfoClient {
- public static final String BASE_URI = "http://localhost:8080/RESTfulWS";
- public static final String PATH_NAME = "/UserInfoService/name/";
- public static final String PATH_AGE = "/UserInfoService/age/";
- public static void main(String[] args) {
- String name = "Pavithra";
- int age = 25;
- ClientConfig config = new DefaultClientConfig();
- Client client = Client.create(config);
- WebResource resource = client.resource(BASE_URI);
- WebResource nameResource = resource.path("rest").path(PATH_NAME + name);
- System.out.println("Client Response \n"
- + getClientResponse(nameResource));
- System.out.println("Response \n" + getResponse(nameResource) + "\n\n");
- WebResource ageResource = resource.path("rest").path(PATH_AGE + age);
- System.out.println("Client Response \n"
- + getClientResponse(ageResource));
- System.out.println("Response \n" + getResponse(ageResource));
- }
- /**
- * Returns client response.
- * e.g :
- * GET http://localhost:8080/RESTfulWS/rest/UserInfoService/name/Pavithra
- * returned a response status of 200 OK
- *
- * @param service
- * @return
- */
- private static String getClientResponse(WebResource resource) {
- return resource.accept(MediaType.TEXT_XML).get(ClientResponse.class)
- .toString();
- }
- /**
- * Returns the response as XML
- * e.g : <User><Name>Pavithra</Name></User>
- *
- * @param service
- * @return
- */
- private static String getResponse(WebResource resource) {
- return resource.accept(MediaType.TEXT_XML).get(String.class);
- }
- }
- Once you run the client program, you'll get following output.
- Client Response
- GET http://localhost:8080/RESTfulWS/rest/UserInfoService/name/Pavithra returned a response status of 200 OK
- Response
- <User><Name>Pavithra</Name></User>
- Client Response
- GET http://localhost:8080/RESTfulWS/rest/UserInfoService/age/25 returned a response status of 200 OK
- Response
- <User><Age>25</Age></User>
From: http://blog.eviac.com/2013/11/restful-web-services-with-java.html
【转】RESTful Webservice创建的更多相关文章
- Eclipse + Jersey 发布RESTful WebService(一)了解Maven和Jersey,创建一个WS项目(成功!)
一.下文中需要的资源地址汇总 Maven Apache Maven网站 http://maven.apache.org/ Maven下载地址: http://maven.apache.org/down ...
- SOAP Webservice和RESTful Webservice
http://blog.sina.com.cn/s/blog_493a845501012566.html REST是一种架构风格,其核心是面向资源,REST专门针对网络应用设计和开发方式,以降低开发的 ...
- RESTful WebService入门(转)
原创作品,允许转载,转载时请务必以超链接形式标明文章 原始出处 .作者信息和本声明.否则将追究法律责任.http://lavasoft.blog.51cto.com/62575/229206 REST ...
- CXF发布restful WebService的入门例子(服务器端)
研究了两天CXF对restful的支持. 现在,想实现一个以 http://localhost:9999/roomservice 为入口, http://localhost:9999/roomse ...
- RESTful Webservice (一) 概念
Representational State Transfer(表述性状态转移) RSET是一种架构风格,其核心是面向资源,REST专门针对网络应用设计和开发方式,以降低开发的复杂性,提高系统的可伸缩 ...
- 使用CXF与Spring集成实现RESTFul WebService
以下引用与网络中!!! 一种软件架构风格,设计风格而不是标准,只是提供了一组设计原则和约束条件.它主要用于客户端和服务器交互类的软件.基于这个风格设计的软件可以更简洁,更有层次,更易于实现缓存 ...
- RESTful WebService入门
RESTful WebService入门 RESTful WebService是比基于SOAP消息的WebService简单的多的一种轻量级Web服务,RESTful WebService是没有状 ...
- Web Service进阶(七)浅谈SOAP Webservice和RESTful Webservice
浅谈SOAP Webservice和RESTful Webservice REST是一种架构风格,其核心是面向资源,REST专门针对网络应用设计和开发方式,以降低开发的复杂性,提高系统的可伸缩性.RE ...
- RESTful WebService入门【转】
ESTful WebService是比基于SOAP消息的WebService简单的多的一种轻量级Web服务,RESTful WebService是没有状态的,发布和调用都非常的轻松容易. 下面写一 ...
随机推荐
- Sqlserver远程过程调用失败
这种情况一般是由于有高版本的SqlServer导致的,网上有删除Loaldb之类的建议,这样其实不太好,回头用高版本数据库的话还得装回来.其实可以通过计算机管理->服务和应用程序进行设置,用下面 ...
- Generic XXE Detection
参考连接:https://www.christian-schneider.net/GenericXxeDetection.html In this article I present some tho ...
- 新浪某站CRLF Injection导致的安全问题
CRLF攻击的一篇科普:新浪某站CRLF Injection导致的安全问题(转) 转:https://www.leavesongs.com/PENETRATION/Sina-CRLF-Injectio ...
- springboot06-swagger2 自动化api文档
1.springboot 项目中添加swagger2依赖: <dependency> <groupId>org.springframework.boot</groupId ...
- Andrew NG 机器学习编程作业4 Octave
问题描述:利用BP神经网络对识别阿拉伯数字(0-9) 训练数据集(training set)如下:一共有5000个训练实例(training instance),每个训练实例是一个400维特征的列向量 ...
- faster rcnn相关内容
转自: https://zhuanlan.zhihu.com/p/31426458 faster rcnn的基本结构 Faster RCNN其实可以分为4个主要内容: Conv layers.作为一种 ...
- MySQL安装 8.0.15版本
windows下MySQL 8.0.15的安装和设置 MySQL下载地址:https://dev.mysql.com/downloads/mysql/ 我的百度网盘下载(win64位):链接:http ...
- 洛谷P2251 【质量检测】
无意中刷st表题看到的题目(抄模板),一看到题目,,,没想用st表,直接莫队?????跑起来也不是特别慢... 这里用flag数组记录出现次数,set维护最小值,用的时候直接取头部. 代码也很短 #i ...
- A Bayesian Approach to Deep Neural Network Adaptation with Applications to Robust Automatic Speech Recognition
基于贝叶斯的深度神经网络自适应及其在鲁棒自动语音识别中的应用 直接贝叶斯DNN自适应 使用高斯先验对DNN进行MAP自适应 为何贝叶斯在模型自适应中很有用? 因为自适应问题可以视为后验估计问题 ...
- Censys
Censys Censys持续监控互联网上所有可访问的服务器和设备,以便您可以实时搜索和分析它们,了解你的网络攻击面,发现新的威胁并评估其全球影响.从互联网领先的扫描仪ZMap的创造者来说,我们的使命 ...