POJ 1066 - Treasure Hunt - [枚举+判断线段相交]
题目链接:http://poj.org/problem?id=1066
Time Limit: 1000MS Memory Limit: 10000K
Description
An example is shown below:
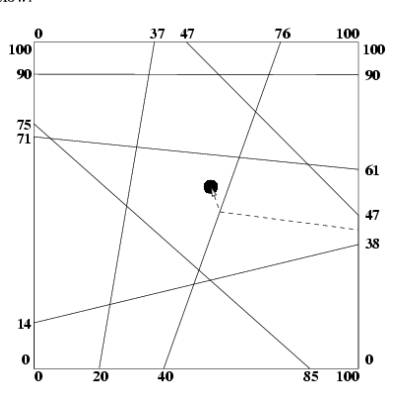
Input
Output
Sample Input
- 7
- 20 0 37 100
- 40 0 76 100
- 85 0 0 75
- 100 90 0 90
- 0 71 100 61
- 0 14 100 38
- 100 47 47 100
- 54.5 55.4
Sample Output
- Number of doors = 2
题意:
一个正方形底的金字塔,坐标为(0,0)->(100,100),里面情况类似于上图,有许多直接连接在最外层正方形上的墙,把整个金字塔底部分割成许多小房间;
现在专家们已经确定,其中某一个房间为宝藏房,并且得到了一个位于该房间内的点坐标,记为点p;
现在专家们要从最外面进行爆破开门法,一直炸到宝藏房,求最少需要开多少扇门。
题解:
枚举(0,0)-(0,100)-(100,100)-(100,0)这个正方形上的所有点(其实就是所有墙的端点),记为点q;
连接p与q两点得到一条线段,再去枚举所有的墙,通过判断是否规范相交确定一路上要经过多少堵墙,记为cnt;
顺便把(0,0)、(0,100)、(100,100)、(100,0)这四个点也按上面的办法去算一下cnt;
取所有cnt中最小的,加上1(外墙上还要开扇门),即为答案;
AC代码:
- #include<cstdio>
- #include<cmath>
- #include<iostream>
- using namespace std;
- const double eps = 1e-;
- struct Point{
- double x,y;
- Point(double tx=,double ty=):x(tx),y(ty){}
- };
- typedef Point Vctor;
- //向量的加减乘除
- Vctor operator + (Vctor A,Vctor B){return Vctor(A.x+B.x,A.y+B.y);}
- Vctor operator - (Point A,Point B){return Vctor(A.x-B.x,A.y-B.y);}
- Vctor operator * (Vctor A,double p){return Vctor(A.x*p,A.y*p);}
- Vctor operator / (Vctor A,double p){return Vctor(A.x/p,A.y/p);}
- int dcmp(double x)
- {
- if(fabs(x)<eps) return ;
- else return (x<)?(-):();
- }
- bool operator == (Point A,Point B){return dcmp(A.x-B.x)== && dcmp(A.y-B.y)==;}
- double Cross(Vctor A,Vctor B){return A.x*B.y-A.y*B.x;}
- //判断线段是否规范相交
- bool SegmentProperIntersection(Point a1,Point a2,Point b1,Point b2)
- {
- double c1 = Cross(a2 - a1,b1 - a1), c2 = Cross(a2 - a1,b2 - a1),
- c3 = Cross(b2 - b1,a1 - b1), c4 = Cross(b2 - b1,a2 - b1);
- return dcmp(c1)*dcmp(c2)< && dcmp(c3)*dcmp(c4)<;
- }
- int n;
- struct Seg{
- Point a,b;
- }wall[];
- Point p;
- int test(const Point& q)
- {
- int cnt=;
- for(int i=;i<=n;i++)
- if(SegmentProperIntersection(p,q,wall[i].a,wall[i].b)) cnt++;
- return cnt;
- }
- int main()
- {
- cin>>n;
- for(int i=;i<=n;i++) scanf("%lf%lf%lf%lf",&wall[i].a.x,&wall[i].a.y,&wall[i].b.x,&wall[i].b.y);
- cin>>p.x>>p.y;
- int ans=0x3f3f3f3f;
- for(int i=,tmp;i<=n;i++)
- {
- ans=min(test(wall[i].a),ans);
- ans=min(test(wall[i].b),ans);
- }
- ans=min(test(Point(,)),ans);
- ans=min(test(Point(,)),ans);
- ans=min(test(Point(,)),ans);
- ans=min(test(Point(,)),ans);
- cout<<"Number of doors = "<<ans+<<endl;
- }
POJ 1066 - Treasure Hunt - [枚举+判断线段相交]的更多相关文章
- POJ 1066 Treasure Hunt --几何,线段相交
题意: 正方形的房子,给一些墙,墙在区域内是封闭的,给你人的坐标,每穿过一道墙需要一把钥匙,问走出正方形需要多少把钥匙. 解法: 因为墙是封闭的,所以绕路也不会减少通过的墙的个数,还不如不绕路走直线, ...
- POJ 2653 - Pick-up sticks - [枚举+判断线段相交]
题目链接:http://poj.org/problem?id=2653 Time Limit: 3000MS Memory Limit: 65536K Description Stan has n s ...
- 简单几何(线段相交) POJ 1066 Treasure Hunt
题目传送门 题意:从四面任意点出发,有若干障碍门,问最少要轰掉几扇门才能到达终点 分析:枚举入口点,也就是线段的两个端点,然后选取与其他线段相交点数最少的 + 1就是答案.特判一下n == 0的时候 ...
- POJ 2653 Pick-up sticks(判断线段相交)
Pick-up sticks Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 7699 Accepted: 2843 De ...
- poj 1066 Treasure Hunt (Geometry + BFS)
1066 -- Treasure Hunt 题意是,在一个金字塔中有一个宝藏,金字塔里面有很多的墙,要穿过墙壁才能进入到宝藏所在的地方.可是因为某些原因,只能在两个墙壁的交点连线的中点穿过墙壁.问最少 ...
- POJ 2826 An Easy Problem? 判断线段相交
POJ 2826 An Easy Problem?! -- 思路来自kuangbin博客 下面三种情况比较特殊,特别是第三种 G++怎么交都是WA,同样的代码C++A了 #include <io ...
- 【POJ 2653】Pick-up sticks 判断线段相交
一定要注意位运算的优先级!!!我被这个卡了好久 判断线段相交模板题. 叉积,点积,规范相交,非规范相交的简单模板 用了“链表”优化之后还是$O(n^2)$的暴力,可是为什么能过$10^5$的数据? # ...
- POJ 1066 Treasure Hunt(线段相交判断)
Treasure Hunt Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 4797 Accepted: 1998 Des ...
- POJ 1066 Treasure Hunt (线段相交)
题意:给你一个100*100的正方形,再给你n条线(墙),保证线段一定在正方形内且端点在正方形边界(外墙),最后给你一个正方形内的点(保证不再墙上) 告诉你墙之间(包括外墙)围成了一些小房间,在小房间 ...
随机推荐
- aspose导出excel文件
using Aspose.Cells; using System; using System.Collections.Generic; using System.Data; using System. ...
- Git中的文件状态和使用问题解决
(暂存区 即Index In Git) commit 到 local respository的内容,不想push,则使用git reset 将文件状态回转到staged|modified|unstag ...
- dubbo开发前戏--ZooKeeper集群部署(3.4.6)
最近在开发dubbo服务的时候一直用的是公司提供的zk平台,因为使用的人太多或者没人维护老是出问题,导致dubbo服务偶尔可以调通,偶尔调不通的情况,所以花点时间自己部署一套,后面出问题还方便看日志排 ...
- Eclipse cdt解决github导入的项目无法打开声明的bug (cannot open declaration)
概述: 我利用eclipse 的git插件clone github上的远程项目(C++)到本地时遇到一个问题:clone下来的项目没有C++特性,无法使用open declaration等操作,下面是 ...
- OpenGl 知识一
写在前面 啦啦啦,搞了很久的Unity Shaders,越学越觉得基础知识很重要.学Unity Shader的时候,总会想,shader到底是什么呢?shader的pipeline是什么呢?它们是怎么 ...
- 在SELECT DISTINCT 状况下使用 Order BY Newid() 随机数选出记录
在日常作业中,有时候可能是一些活动要抽出得奖人或选出抽查的一些名单, 就常常会使用到 Order BY Newid() 的方式来做随机数选出, 但有可能的状况需是要搭配到 DISTINCT 来选出,这 ...
- SaltStack 批量执行脚本
这里演示如何使用 salt-master 对多台 salt-minion 批量执行脚本,步骤如下: [root@localhost ~]$ cat /srv/salt/top.sls # 先定义入口配 ...
- Memcached 数据导出与导入
我们使用 memcached-tool 命令来导出数据: [root@localhost ~]# memcached-tool dump > /tmp/.txt Dumping memcache ...
- 使用 urllib 解析 URL 链接
urllib 库还提供了 parse 模块,它定义了处理 URL 的标准接口,例如实现 URL 各部分的抽取.合并以及链接转换,常用的方法如下: In []: from urllib.parse im ...
- codeforces水题100道 第二十七题 Codeforces Round #172 (Div. 2) A. Word Capitalization (strings)
题目链接:http://www.codeforces.com/problemset/problem/281/A题意:将一个英文字母的首字母变成大写,然后输出.C++代码: #include <c ...