POJ 3084 Panic Room(最大流最小割)
Description
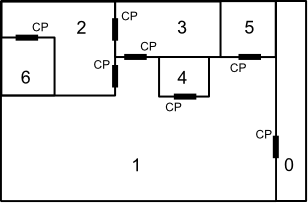
Input
- Start line – a single line "m n" (1 <=m<= 20; 0 <=n<= 19) where m indicates the number of rooms in the house and n indicates the room to secure (the panic room).
- Room list – a series of m lines. Each line lists, for a single room, whether there is an intruder in that room ("I" for intruder, "NI" for no intruder), a count of doors c (0 <= c <= 20) that lead to other rooms and have a control panel in this room, and a list of rooms that those doors lead to. For example, if room 3 had no intruder, and doors to rooms 1 and 2, and each of those doors' control panels were accessible from room 3 (as is the case in the above layout), the line for room 3 would read "NI 2 1 2". The first line in the list represents room 0. The second line represents room 1, and so on until the last line, which represents room m - 1. On each line, the rooms are always listed in ascending order. It is possible for rooms to be connected by multiple doors and for there to be more than one intruder!
Output
题目大意:有n个房间,若干扇门,门是单向的,只能从一边上锁(如一道门连接a→b,任何时刻都能从a走到b,但上了锁之后就不能从b走到a了)。现在有些坏蛋入侵了一些房间,你不想让他们来到你的房间,问最少要锁上多少扇门,若全锁上都木有用……就……
思路:新建一个源点S,从S到坏蛋们入侵的房间连一条容量为无穷大的边,对每扇门a→b,连一条边a→b容量为无穷大,连b→a容量为1。若最大流≥无穷大(我是增广到一条容量为无穷大的路径直接退出),则无解(死定啦死定啦),否则最大流为答案。实则为求最小割,好像不需要解释了挺好理解的……
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <queue>
using namespace std; const int MAXN = ;
const int MAXE = ;
const int INF = 0x3fff3fff; struct SAP {
int head[MAXN], dis[MAXN], pre[MAXN], cur[MAXN], gap[MAXN];
int to[MAXE], next[MAXE], flow[MAXE];
int n, st, ed, ecnt; void init() {
memset(head, , sizeof(head));
ecnt = ;
} void add_edge(int u, int v, int c) {
to[ecnt] = v; flow[ecnt] = c; next[ecnt] = head[u]; head[u] = ecnt++;
to[ecnt] = u; flow[ecnt] = ; next[ecnt] = head[v]; head[v] = ecnt++;
//printf("%d->%d flow = %d\n", u, v, c);
} void bfs() {
memset(dis, 0x3f, sizeof(dis));
queue<int> que; que.push(ed);
dis[ed] = ;
while(!que.empty()) {
int u = que.front(); que.pop();
++gap[dis[u]];
for(int p = head[u]; p; p = next[p]) {
int &v = to[p];
if(flow[p ^ ] && dis[v] > n) {
dis[v] = dis[u] + ;
que.push(v);
}
}
}
} int Max_flow(int ss, int tt, int nn) {
st = ss; ed = tt; n = nn;
int ans = , minFlow = INF, u;
for(int i = ; i <= n; ++i) {
cur[i] = head[i];
gap[i] = ;
}
u = pre[st] = st;
bfs();
while(dis[st] < n) {
bool flag = false;
for(int &p = cur[u]; p; p = next[p]) {
int &v = to[p];
if(flow[p] && dis[u] == dis[v] + ) {
flag = true;
minFlow = min(minFlow, flow[p]);
pre[v] = u;
u = v;
if(u == ed) {
if(minFlow == INF) return INF;//no ans
ans += minFlow;
while(u != st) {
u = pre[u];
flow[cur[u]] -= minFlow;
flow[cur[u] ^ ] += minFlow;
}
minFlow = INF;
}
break;
}
}
if(flag) continue;
int minDis = n - ;
for(int p = head[u]; p; p = next[p]) {
int &v = to[p];
if(flow[p] && minDis > dis[v]) {
minDis = dis[v];
cur[u] = p;
}
}
if(--gap[dis[u]] == ) break;
gap[dis[u] = minDis + ]++;
u = pre[u];
}
return ans;
}
} G; char s[];
int n, ss, tt, T, c, x; int main() {
scanf("%d", &T);
while(T--) {
scanf("%d%d", &n, &tt);
ss = n;
G.init();
for(int i = ; i < n; ++i) {
scanf("%s%d", s, &c);
while(c--) {
scanf("%d", &x);
G.add_edge(i, x, INF);
G.add_edge(x, i, );
}
if(s[] == 'I') G.add_edge(ss, i, INF);
}
int ans = G.Max_flow(ss, tt, ss);
if(ans == INF) puts("PANIC ROOM BREACH");
else printf("%d\n", ans);
}
}
POJ 3084 Panic Room(最大流最小割)的更多相关文章
- POJ 3084 Panic Room (最小割建模)
[题意]理解了半天--大意就是,有一些房间,初始时某些房间之间有一些门,并且这些门是打开的,也就是可以来回走动的,但是这些门是确切属于某个房间的,也就是说如果要锁门,则只有在那个房间里才能锁. 现在一 ...
- POJ 3308 Paratroopers(最大流最小割の最小点权覆盖)
Description It is year 2500 A.D. and there is a terrible war between the forces of the Earth and the ...
- POJ 2987 Firing(最大流最小割の最大权闭合图)
Description You’ve finally got mad at “the world’s most stupid” employees of yours and decided to do ...
- POJ 1815 Friendship(最大流最小割の字典序割点集)
Description In modern society, each person has his own friends. Since all the people are very busy, ...
- HDU 1569 方格取数(2)(最大流最小割の最大权独立集)
Description 给你一个m*n的格子的棋盘,每个格子里面有一个非负数. 从中取出若干个数,使得任意的两个数所在的格子没有公共边,就是说所取数所在的2个格子不能相邻,并且取出的数的和最大. ...
- hiho 第116周,最大流最小割定理,求最小割集S,T
小Hi:在上一周的Hiho一下中我们初步讲解了网络流的概念以及常规解法,小Ho你还记得内容么? 小Ho:我记得!网络流就是给定了一张图G=(V,E),以及源点s和汇点t.每一条边e(u,v)具有容量c ...
- hihocoder 网络流二·最大流最小割定理
网络流二·最大流最小割定理 时间限制:10000ms 单点时限:1000ms 内存限制:256MB 描述 小Hi:在上一周的Hiho一下中我们初步讲解了网络流的概念以及常规解法,小Ho你还记得内容么? ...
- [HihoCoder1378]网络流二·最大流最小割定理
思路: 根据最大流最小割定理可得最大流与最小割相等,所以可以先跑一遍EdmondsKarp算法.接下来要求的是经过最小割切割后的图中$S$所属的点集.本来的思路是用并查集处理所有前向边构成的残量网络, ...
- FZU 1844 Earthquake Damage(最大流最小割)
Problem Description Open Source Tools help earthquake researchers stay a step ahead. Many geological ...
随机推荐
- hashMap 和 linkedHashMap 的区别和联系
直接举例说明. 运行如下例子程序 mport java.util.HashMap; import java.util.Iterator; import java.util.LinkedHashMap; ...
- jQuery入门简单实现反选与全选
//html代码<input type="checkbox" id= 'all' value="全选"> 选择全部 一键上路 <input t ...
- 执行SQL查询导致磁盘耗尽故障演示
a fellow in IMG wechat group 2 met an error about running out of disk space when using MySQL ...
- 编译升级至openssh7.6
1.概述 目的:下载源码包(https://openbsd.hk/pub/OpenBSD/OpenSSH/portable/openssh-7.6p1.tar.gz),编译升级为openssh为7.6 ...
- html中的定位
html中的定位体系 一. 分类 1.常规流static 2.浮动float 3.相对定位relative 4.绝对定位absolute 5.固定定位fixed 二.使用时的区分 在网页布局中,常常都 ...
- 史上更全的 MySQL 高性能优化实战总结!
1 前言 2 优化的哲学 3 优化思路 3.1 优化什么 3.2 优化的范围有哪些 3.3 优化维度 4 优化工具有啥? 4.1 数据库层面 4.2 数据库层面问题解决思路 4.3 系统层面 4.4 ...
- VIM 键
输入 vimtutor命令,可以打开Linux使用手册(基本使用). ***. 插入键: A: 行尾插入 a: 字符后面插入 i: 字符前面插入 I: 行首插入 r:只替换一次字符 R:一直替换,直 ...
- Noip 2011 Day 1 & Day 2
Day 1 >>> T1 >> 水题一道 . 我们只需要 for 一遍 , 由于地毯是从下往上铺的 , 我们只需要记录该位置最上面的地毯的编号 , 每一次在当前地 ...
- Eclipse安装Java Class反编译插件
第一步:没有安装之前 第二步:从Eclipse Marketplace里,安装反编译插件jadclipse. 第三步:安装反编译插件之后,多了一个查看器,把"类反编译查看器"设置为 ...
- BZOJ3293_分金币_KEY
题目传送门 设x[i]表示i+1向i传的糖果数,x[n]表示1向n传的糖果数,a'=(a[1]+...a[N])/N a[1]+x[1]−x[n]=a' a[2]+x[2]−x[1]=a' a[3]+ ...