POJ 1330:Nearest Common Ancestors
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 20940 | Accepted: 11000 |
Description
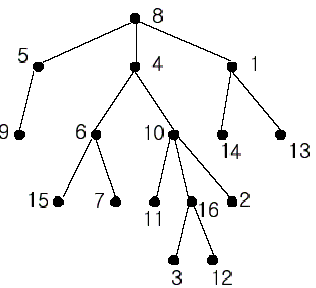
In the figure, each node is labeled with an integer from {1, 2,...,16}. Node 8 is the root of the tree. Node x is an ancestor of node y if node x is in the path between the root and node y. For example, node 4 is an ancestor of node 16. Node 10 is also an ancestor
of node 16. As a matter of fact, nodes 8, 4, 10, and 16 are the ancestors of node 16. Remember that a node is an ancestor of itself. Nodes 8, 4, 6, and 7 are the ancestors of node 7. A node x is called a common ancestor of two different nodes y and z if node
x is an ancestor of node y and an ancestor of node z. Thus, nodes 8 and 4 are the common ancestors of nodes 16 and 7. A node x is called the nearest common ancestor of nodes y and z if x is a common ancestor of y and z and nearest to y and z among their common
ancestors. Hence, the nearest common ancestor of nodes 16 and 7 is node 4. Node 4 is nearer to nodes 16 and 7 than node 8 is.
For other examples, the nearest common ancestor of nodes 2 and 3 is node 10, the nearest common ancestor of nodes 6 and 13 is node 8, and the nearest common ancestor of nodes 4 and 12 is node 4. In the last example, if y is an ancestor of z, then the nearest
common ancestor of y and z is y.
Write a program that finds the nearest common ancestor of two distinct nodes in a tree.
Input
N. Each of the next N -1 lines contains a pair of integers that represent an edge --the first integer is the parent node of the second integer. Note that a tree with N nodes has exactly N - 1 edges. The last line of each test case contains two distinct integers
whose nearest common ancestor is to be computed.
Output
Sample Input
2
16
1 14
8 5
10 16
5 9
4 6
8 4
4 10
1 13
6 15
10 11
6 7
10 2
16 3
8 1
16 12
16 7
5
2 3
3 4
3 1
1 5
3 5
Sample Output
4
3
并查集。之前在hihoCoder第十二周做过类似的。
代码:
#include <iostream>
#include <vector>
#include <string>
#include <cstring>
#include <algorithm>
#pragma warning(disable:4996)
using namespace std; int father[10005]; void result(int test1,int test2)
{
int node2=test2;
while(father[test1]!=test1)
{
node2=test2;
while(father[node2]!=node2)
{
if(test1==node2)
{
cout<<test1<<endl;
return;
}
node2=father[node2];
}
test1=father[test1];
}
cout<<test1<<endl;
return;
} int main()
{
int count;
cin>>count; while(count--)
{
int fa,son,node,i;
cin>>node; for(i=1;i<10005;i++)
{
father[i]=i;
} for(i=1;i<=node-1;i++)
{
cin>>fa>>son;
father[son]=fa;
}
int test1,test2;
cin>>test1>>test2; result(test1,test2);
}
return 0;
}
版权声明:本文为博主原创文章,未经博主允许不得转载。
POJ 1330:Nearest Common Ancestors的更多相关文章
- 【51.64%】【POJ 1330】Nearest Common Ancestors
Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 26416 Accepted: 13641 Description A roote ...
- 【Poj 1330】Nearest Common Ancestors
http://poj.org/problem?id=1330 题目意思就是T组树求两点LCA. 这个可以离线DFS(Tarjan)-----具体参考 O(Tn) 0ms 还有其他在线O(Tnlogn) ...
- 【POJ 1330】 Nearest Common Ancestors
[题目链接] 点击打开链接 [算法] 倍增法求最近公共祖先 [代码] #include <algorithm> #include <bitset> #include <c ...
- POJ 1330 Nearest Common Ancestors(Tree)
题目:Nearest Common Ancestors 根据输入建立树,然后求2个结点的最近共同祖先. 注意几点: (1)记录每个结点的父亲,比较层级时要用: (2)记录层级: (3)记录每个结点的孩 ...
- POJ 1330 Nearest Common Ancestors 【LCA模板题】
任意门:http://poj.org/problem?id=1330 Nearest Common Ancestors Time Limit: 1000MS Memory Limit: 10000 ...
- POJ 1330 Nearest Common Ancestors 倍增算法的LCA
POJ 1330 Nearest Common Ancestors 题意:最近公共祖先的裸题 思路:LCA和ST我们已经很熟悉了,但是这里的f[i][j]却有相似却又不同的含义.f[i][j]表示i节 ...
- POJ - 1330 Nearest Common Ancestors(基础LCA)
POJ - 1330 Nearest Common Ancestors Time Limit: 1000MS Memory Limit: 10000KB 64bit IO Format: %l ...
- POJ 1330 Nearest Common Ancestors / UVALive 2525 Nearest Common Ancestors (最近公共祖先LCA)
POJ 1330 Nearest Common Ancestors / UVALive 2525 Nearest Common Ancestors (最近公共祖先LCA) Description A ...
- POJ.1330 Nearest Common Ancestors (LCA 倍增)
POJ.1330 Nearest Common Ancestors (LCA 倍增) 题意分析 给出一棵树,树上有n个点(n-1)条边,n-1个父子的边的关系a-b.接下来给出xy,求出xy的lca节 ...
随机推荐
- JS中字符串的编码 解码
DEPTNAME 是一个字符串 编码: DEPTNAME = encodeURI(encodeURI(DEPTNAME)); 解码: DEPTNAME = decodeURI(DEPTNAME,&qu ...
- linux安装jdk并设置环境变量(看这一篇文章即可)
1.查看linux位数 查看linux是32位还是64位,影响需要下载JDK的版本 系统位数 jdk位数 x86(32位) 32位 x86_64(64位) 32位 64位 在linux命令输入: ...
- kubernetes 使用flannel网络模式 错误分析
今天按照网上和书上的要求,将目前的kubernetes网络换成flannel.其实配置起来还是很简单的,但是一旦出现了问题,将很难解决. 配置方法我这边不给出了.因为网上这样的教程一大把,在说下去也无 ...
- Hadoop大实验——MapReduce的操作
日期:2019.10.30 博客期:114 星期三 实验6:Mapreduce实例——WordCount 实验说明: 1. 本次实验是第六次上机,属于验证性实验.实验报告上交截止 ...
- RadioButton 用法
@Html.RadioButton("rdoNotice", "1ST", true, new { id = "rdoFirstNotice" ...
- list.OfType()
将两个类型不同是实体存入到一个object可以通过OfType方法分别取出来 //将两个类型不同是实体存入到一个object可以通过OfType方法分别取出来 List<Phone> Ph ...
- LLDB常用指令
1.help:列举所有的命令,也可以用于查询某个命令的说明,比如,help print,help help 2.print:打印,简写为,prin,pri,p,打印的结果比如,$10代表时该结果, ...
- 任意两点之间的最短路(floyed)
F.Moving On Firdaws and Fatinah are living in a country with nn cities, numbered from 11 to nn. Each ...
- Vue 路由组件
目录 组件数据局部化处理 路由逻辑跳转 组件传参 父传子 子传父 组件的生命周期钩子 路由传参 全家配置自定义 CSS 与 js 总结: 组件数据局部化处理 不管页面组件还是小组件,都可能会被多次复用 ...
- android 基础学习(6)-----sqlite3查看表结构
原文:http://blog.csdn.net/richnaly/article/details/7831933 sqlite3查看表结构 在android下通过adb shell命令可以进入sqli ...