hdu 1828 Picture(线段树 || 普通hash标记)
http://acm.hdu.edu.cn/showproblem.php?pid=1828
Picture
Time Limit: 6000/2000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) Total Submission(s): 2135 Accepted Submission(s): 1134
Write a program to calculate the perimeter. An example with 7 rectangles is shown in Figure 1.
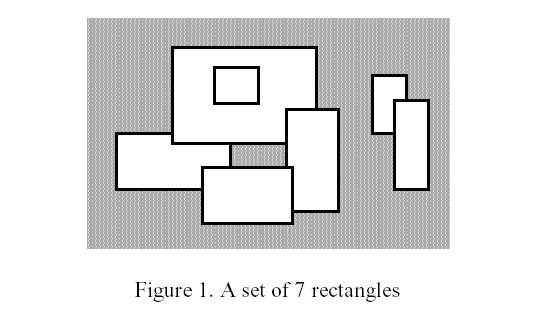
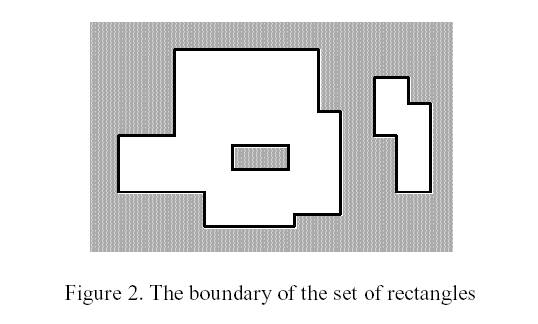
0 <= number of rectangles < 5000 All coordinates are in the range [-10000,10000] and any existing rectangle has a positive area.
Please process to the end of file.
{
int s,t; //存始末位置
int x;
int flag; //标记是否是起始边 1 -1
}linex[N<<1],liney[N<<1]; //分别安x与y构造线段
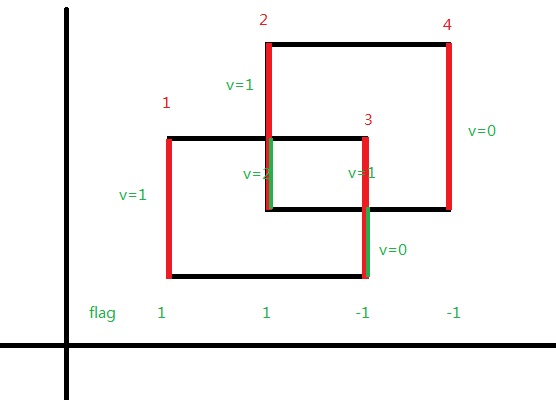
#include <iostream>
#include <string.h>
#include <stdio.h>
#include <algorithm>
#define N 5010
using namespace std; struct Line
{
int s,t; //存始末位置
int x;
int flag; //标记是否是起始边 1 -1
}linex[N<<],liney[N<<]; //分别安x与y构造线段 int visit[N<<]; //判断是否访问过 bool cmp(Line a,Line b) //sort排序用
{
return a.x<b.x;
} int cal_x(int n) //统计按x排序的平行于y的线段长
{
int i,j,cnt=;
for(i=;i<=n;i++)
{
if(linex[i].flag == ) //如果是起始边
{
for(j=linex[i].s;j<linex[i].t;j++)
{
if(visit[j]==) cnt++; //起始边,没访问过cnt++
visit[j]++; //标记+1
}
}
else //如果是结束边
{
for(j=linex[i].s;j<linex[i].t;j++)
{
if(visit[j]==) cnt++; //结束边,访问过cnt++
visit[j]--; //标记-1,等待下一个起始边的进入
}
}
}
return cnt;
} int cal_y(int n) //同上,统计按y排序的平行于x的线段长
{
int i,j,cnt=;
for(i=;i<=n;i++)
{
if(liney[i].flag == )
{
for(j=liney[i].s;j<liney[i].t;j++)
{
if(visit[j]==) cnt++;
visit[j]++;
}
}
else
{
for(j=liney[i].s;j<liney[i].t;j++)
{
if(visit[j]==) cnt++;
visit[j]--;
}
}
}
return cnt;
} int main()
{
int n;
while(~scanf("%d",&n))
{
int i,cnt=;
for(i=;i<n;i++)
{
int x1,y1,x2,y2;
scanf("%d%d%d%d",&x1,&y1,&x2,&y2);
x1+=N<<;
x2+=N<<;
y1+=N<<;
y2+=N<<; //平移N<<1,排除负数情况 cnt++;
linex[cnt].x = x1;
linex[cnt].s = y1;
linex[cnt].t = y2;
linex[cnt].flag = ; liney[cnt].x = y1;
liney[cnt].s = x1;
liney[cnt].t = x2;
liney[cnt].flag = ; cnt++;
linex[cnt].x = x2;
linex[cnt].s = y1;
linex[cnt].t = y2;
linex[cnt].flag = -; liney[cnt].x = y2;
liney[cnt].s = x1;
liney[cnt].t = x2;
liney[cnt].flag = -;
} sort(linex+,linex++cnt,cmp); //线段按x进行排序
sort(liney+,liney++cnt,cmp); //线段按y进行排序 int ans = ;
memset(visit,,sizeof(visit));
ans += cal_x(cnt); //统计x
memset(visit,,sizeof(visit));
ans += cal_y(cnt); //统计y printf("%d\n",ans);
}
return ;
}
【题解2】:线段树
线段树代码是参照别人的
【code】:
#include<iostream>
#include<string>
#include<stdio.h>
#include<algorithm>
#include<cmath>
using namespace std; struct node
{
int l;
int r;
int len; //该区间可与下一个将要插入的线段组成并面积的长度
int cover; //该区间覆盖了几根线段
int num; //记录该区间由几个子区间组成了可与下一个将要插入的线段组成并面积,即可并子区间的数目
int r_cover; //记录该区间右节点是否被可并区间覆盖
int l_cover; //。。。。。坐。。。。。。。。。
}; node tree[];
int n;
int yy[],len; struct Line
{
int l;
int r;
int x;
int cover;
}; Line line[]; int cmp(Line a,Line b)
{
return a.x<b.x;
} int find(int x)
{
int l=,r=len,mid;
while(l<=r)
{
mid=(l+r)/;
if(yy[mid]==x)
return mid;
if(yy[mid]<x)
l=mid+;
else
r=mid-;
}
return l;
} void build(int i,int l,int r)
{
tree[i].l=l;
tree[i].r=r;
tree[i].cover=tree[i].l_cover=tree[i].r_cover=tree[i].len=tree[i].num=;
if(l+==r)
return;
int mid=(l+r)/;
build(*i,l,mid);
build(*i+,mid,r);
} void fun(int i)
{
if(tree[i].cover) //整个被覆盖
{
tree[i].len=yy[tree[i].r]-yy[tree[i].l]; //可用长度为整个区间
tree[i].l_cover=tree[i].r_cover=; //左右节点都被覆盖了
tree[i].num=; //由一个区间组成,即该区间
}
else if(tree[i].l+==tree[i].r) //叶子区间
{
tree[i].len=;
tree[i].l_cover=tree[i].r_cover=;
tree[i].num=;
}
else
{
tree[i].len=tree[*i].len+tree[*i+].len; //dp
tree[i].l_cover=tree[*i].l_cover; //左节点是否覆盖,取决于左子区间的左节点是否被覆盖
tree[i].r_cover=tree[*i+].r_cover; //同理
tree[i].num=tree[*i].num+tree[*i+].num-tree[*i].r_cover*tree[*i+].l_cover; //该线段可用长度的区间组成数等于左右子区间的组成数
} //但是,当左右子区间是连续的时候,结果要减一
} void updata(int i,int l,int r,int w)
{
if(tree[i].l>r || tree[i].r<l)
return;
if(tree[i].l>=l && tree[i].r<=r)
{
tree[i].cover+=w;
fun(i);
return;
}
updata(*i,l,r,w);
updata(*i+,l,r,w);
fun(i);
} int main()
{
int i,x1,x2,y1,y2,m,a,b;
//freopen("in.txt","r",stdin);
while(scanf("%d",&n)!=EOF)
{
m=;
for(i=;i<n;i++)
{
scanf("%d%d%d%d",&x1,&y1,&x2,&y2);
yy[m]=y1;
line[m].cover=;
line[m].x=x1;
line[m].l=y1;
line[m++].r=y2; yy[m]=y2;
line[m].cover=-;
line[m].x=x2;
line[m].l=y1;
line[m++].r=y2;
}
sort(yy,yy+m);
sort(line,line+m,cmp);
len=;
for(i=;i<m;i++) //?
{
if(yy[i-]!=yy[i])
yy[len++]=yy[i];
}
len--;
build(,,len);
int ans=,pre=;
for(i=;i<m;i++)
{
a=find(line[i].l);
b=find(line[i].r);
updata(,a,b,line[i].cover);
ans+=abs((tree[].len-pre)); //加上y坐标上的周长
if(i==m-)
break;
pre=tree[].len;
ans+=tree[].num*(line[i+].x-line[i].x)*;//加上x坐标上的周长,因为一个y边连着两个x边,所以乘二
}
printf("%d\n",ans);
}
return ;
}
hdu 1828 Picture(线段树 || 普通hash标记)的更多相关文章
- POJ 1177/HDU 1828 picture 线段树+离散化+扫描线 轮廓周长计算
求n个图矩形放下来,有的重合有些重合一部分有些没重合,求最后总的不规则图型的轮廓长度. 我的做法是对x进行一遍扫描线,再对y做一遍同样的扫描线,相加即可.因为最后的轮廓必定是由不重合的线段长度组成的, ...
- HDU 1828 Picture (线段树+扫描线)(周长并)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1828 给你n个矩形,让你求出总的周长. 类似面积并,面积并是扫描一次,周长并是扫描了两次,x轴一次,y ...
- hdu 1828 Picture(线段树轮廓线)
Picture Time Limit: 6000/2000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Sub ...
- HDU 1828 Picture (线段树:扫描线周长)
依然是扫描线,只不过是求所有矩形覆盖之后形成的图形的周长. 容易发现,扫描线中的某一条横边对答案的贡献. 其实就是 加上/去掉这条边之前的答案 和 加上/去掉这条边之后的答案 之差的绝对值 然后横着竖 ...
- HDU 1828 Picture(长方形的周长和)
HDU 1828 Picture 题目链接 题意:给定n个矩形,输出矩形周长并 思路:利用线段树去维护,分别从4个方向扫一次,每次多一段的时候,就查询该段未被覆盖的区间长度,然后周长就加上这个长度,4 ...
- hdu 4031 attack 线段树区间更新
Attack Time Limit: 5000/3000 MS (Java/Others) Memory Limit: 65768/65768 K (Java/Others)Total Subm ...
- hdu 4288 离线线段树+间隔求和
Coder Time Limit: 20000/10000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) Total Su ...
- hdu 3016 dp+线段树
Man Down Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) Total S ...
- cf213E 线段树维护hash
链接 https://codeforces.com/contest/213/problem/E 题目大意 给出两个排列a.b,长度分别为n.m,你需要计算有多少个x,使 得\(a_1 + x; a_2 ...
随机推荐
- C#基础篇--文件(流)
1:Path类是专门用来操作文件路径的(Path类是静态类):当然用字符串的处理办法也能实现. string str = @"C:\Users\成才\Desktop\Hashtable.t ...
- aggregation 详解4(pipeline aggregations)
概述 管道聚合处理的对象是其它聚合的输出(桶或者桶的某些权值),而不是直接针对文档. 管道聚合的作用是为输出增加一些有用信息. 管道聚合大致分为两类: parent 此类聚合的"输入&quo ...
- Java与Android开发环境配置以及遇到的问题解决
0 概述 所有文章涉及的下载地址在文章下方会有汇总,所有软件的版本最好与系统版本一致 建议安装安卓开发软件至一个目录中,以方便查找 1 Java环境配置 1.1 JDK下载: 据说JDK6用的比较多, ...
- Java——String.split()函数
在java doc里有 String[] java.lang.String.split(String regex) Splits this string around matches of the g ...
- 重大发现Android studio 如何简单快速修改package name
好多人都发现Android studio修改包名比较麻烦,只能一级一级的修改,今天偶尔发现了一个快捷方法. 废话不多说: 1 打开项目的AndroidManifest.xml文件 2 鼠标光笔定位到你 ...
- 【转载】java数据库操作
数据库访问几乎每一个稍微成型的程序都要用到的知识,怎么高效的访问数据库也是我们学习的一个重点,今天的任务就是总结java访问数据库的方法和有关API,java访问数据库主要用的方法是JDBC,它是ja ...
- HashMap使用
/* * 测试HashMap的应用,判断 */ import java.util.HashMap; public class HuaWeiTest { private static final Int ...
- 一个layer可以跟着画完的线移动ios程序 好玩啊。
用法:采用的是关键帧实现的. 实验目的:让上层的layer子层能够跟着在另一个子层上花的线进行移动 .即当线画完之后,图形开始移动,并且能够停在最后的那个位置 效果图: 采用是直接在layer图层上进 ...
- Razor引擎学习:RenderBody,RenderPage和RenderSection
ASP.NET MVC 3 已经正式发布了,现在估计许多人都在拼命学,我也不能例外,刚刚看到了一篇文章,介绍了三个非常有用的方法:RenderBody,RenderPage和RenderSection ...
- OC1_汉字拼音转换 练习
// // WordManager.h // OC1_汉字拼音转换 // // Created by zhangxueming on 15/4/27. // Copyright (c) 2015年 z ...