UVA 1351 十三 String Compression
Time Limit:3000MS Memory Limit:0KB 64bit IO Format:%lld & %llu
System Crawler (2015-08-21)
Description
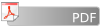
Run Length Encoding(RLE) is a simple form of compression. RLE consists of the process for searching for a repeated runs of a single character in a string to be compressed, and replacing them by a single instance of the character and a run count. For example, a stringabcccddddddefgggggggggghijk is encoded into a string ab3c6def10ghijk by RLE.
A new compression method similar to RLE is devised and the rule of the method is as follows: if a substring S<tex2html_verbatim_mark> is repeated k<tex2html_verbatim_mark> times, replace k<tex2html_verbatim_mark> copies of S<tex2html_verbatim_mark> by k(S)<tex2html_verbatim_mark> . For example, letsgogogo is compressed into lets3(go). The length of letsgogogo is 10, and the length of lets3(go) is 9. In general, the length of k(S)<tex2html_verbatim_mark> is (number of digits in k<tex2html_verbatim_mark> ) + (length of S<tex2html_verbatim_mark> ) + 2 (for `(' and `)'). For example, the length of 123(abc) is 8. It is also possible to nest compression, so the substring S<tex2html_verbatim_mark> may itself be a compressed string. For example,nowletsgogogoletsgogogo could be compressed as a now2(lets3(go)), and nowletsgogogoletsgogogoandrunrunrun could be compressed as now2(lets3(go))and3(run).
Write a program that, for a given string, gives a shortest compressed string using the compression rules as described above.
Input
Your program is to read from standard input. The input consists of T<tex2html_verbatim_mark> test cases. The number of test cases T<tex2html_verbatim_mark> is given in the first line of the input. Each test case consists of a single line containing one string of no more than 200 characters drawn from a lower case alphabet. The length of shortest input string is 1.
Output
Your program is to write to standard output. Print exactly one line for each test case. For each test case, print the length of the shortest compressed string.
The following shows sample input and output for four test cases.
Sample Input
4
ababcd
letsgogogo
nowletsgogogoletsgogogo
nowletsgogogoletsgogogoandrunrunrun
Sample Output
6
9
15
24
#include <stdio.h>
#include <string.h>
#include <algorithm>
using namespace std; char s[];
int dp[][]; int check(int l,int r,int k)
{
int i,j;
if(l>r)
return ;
for(i=l;i<=r;i++)
{
for(j=;i+j*k<=r;j++)
{
if(s[i]!=s[i+j*k])
return ;
}
}
return ;
} int digit(int a)
{
int s=;
while(a)
{
s++;
a=a/;
}
return s;
} int main()
{
int T;
int i,j,k,p,len;
scanf("%d",&T);
while(T--)
{
scanf("%s",s);
len=strlen(s);
for(i=;i<len;i++)
{
for(j=;j<len;j++)
{
dp[i][j]=;
}
}
for(i=;i<len;i++)
dp[i][i]=;
for(i=;i<=len;i++)
{
for(j=;j+i-<len;j++)
{
int b=j,e=j+i-;
for(k=b;k<e;k++)
{
dp[b][e]=min(dp[b][e],dp[b][k]+dp[k+][e]);
}
for(p=;p<=i/;p++)
{
if(i%p==)
{
if(check(b,e,p))
{
dp[b][e]=min(dp[b][e],digit(i/p)++dp[b][b+p-]);
}
}
}
}
}
printf("%d\n",dp[][len-]);
}
return ;
}
UVA 1351 十三 String Compression的更多相关文章
- 区间DP UVA 1351 String Compression
题目传送门 /* 题意:给一个字符串,连续相同的段落可以合并,gogogo->3(go),问最小表示的长度 区间DP:dp[i][j]表示[i,j]的区间最小表示长度,那么dp[i][j] = ...
- uva live 4394 String painter 间隔dp
// uva live 4394 String painter // // 问题是,在培训指导dp运动主题,乍一看,我以为只是一点点复杂 // A A磕磕磕,两个半小时后,.发现超过例子.然而,鉴于他 ...
- 【leetcode】443. String Compression
problem 443. String Compression Input ["a","a","b","b"," ...
- 443. String Compression
原题: 443. String Compression 解题: 看到题目就想到用map计数,然后将计数的位数计算处理,这里的解法并不满足题目的额外O(1)的要求,并且只是返回了结果array的长度,并 ...
- CF825F String Compression 解题报告
CF825F String Compression 题意 给定一个串s,其中重复出现的子串可以压缩成 "数字+重复的子串" 的形式,数字算长度. 只重复一次的串也要压. 求压缩后的 ...
- 213. String Compression【LintCode java】
Description Implement a method to perform basic string compression using the counts of repeated char ...
- 213. String Compression【easy】
Implement a method to perform basic string compression using the counts of repeated characters. For ...
- codeforces 825F F. String Compression dp+kmp找字符串的最小循环节
/** 题目:F. String Compression 链接:http://codeforces.com/problemset/problem/825/F 题意:压缩字符串后求最小长度. 思路: d ...
- Codeforces 825F - String Compression
825F - String Compression 题意 给出一个字符串,你要把它尽量压缩成一个短的字符串,比如一个字符串ababab你可以转化成3ab,长度为 3,比如bbbacacb转化成3b2a ...
随机推荐
- 夺命雷公狗---DEDECMS----30dedecms数据dede_archives主表进行查询l操作
在plus目录下编写一个test2.php的文件,取出dede_archives的所有信息 <?php //编写test2.php这个文件,主要是为了实现可以取出dede_archives表的所 ...
- Java魔法堂:注释和注释模板 (转)
http://www.cnblogs.com/fsjohnhuang/p/3988883.html 一.注释 1. 注释类型 [a]. 单行注释 // 单行注释 String type = &qu ...
- com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException
Exception in thread "main" com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: You ...
- [tp3.2.1]开启URL(重写模式),省略URL中的index.php
重写模式(省略url中的index.php) 在apache配置文件httpd.conf中,查找 1.mod_rewrite.so, 启动此模块 2.AllowOverride , 值= All 3. ...
- Connection termination(by client)” 错误的处理方法
背景: 在一些项目,当我们使用LR录制脚本的时候,在我们安装认证我们无法启动[网址= ] HTTPS [/url]的IE插件,页面显示空白,没有事件的记录,在Firefox也一样. 在记录日志,我们会 ...
- unix
BSD (Berkeley Software Distribution,伯克利软件套件)是Unix的衍生系统,在1977至1995年间由加州大学伯克利分校开发和发布的.历史上, BSD曾经被认为是UN ...
- 使用 TFDConnection 的 pooled 连接池
从开始看到这个属性,就一直认为他可以提供一个连接池管理功能, 苦于文档资料太少, 甚至在帮助中对该属性的使用都没有任何介绍,如果你搜索百度,也会发现基本没资料. 最后终于在其官方网站看到了其完整相关的 ...
- linux-kernel 学习计划
[资料] http://www.ibm.com/developerworks/cn/views/linux/libraryview.jsp http://www.kerneltravel.net/ [ ...
- JSP/Servlet 中的汉字编码问题
JSP/Servlet 中的汉字编码问题 1.问题的起源 每个国家(或区域)都规定了计算机信息交换用的字符编码集,如美国的 ASCII,中国的 GB2312 -80,日本的 JIS 等,作为该国家/区 ...
- 将edit ctrL弄的像个dos
case WM_CTLCOLOREDIT: { HWND hShellText = GetDlgItem(hDlg,IDC_TXT_SHELL); if (hShellText == (HWND)lP ...