Spring Security(三十):9.5 Access-Control (Authorization) in Spring Security
The main interface responsible for making access-control decisions in Spring Security is the AccessDecisionManager
. It has a decide
method which takes an Authentication
object representing the principal requesting access, a "secure object" (see below) and a list of security metadata attributes which apply for the object (such as a list of roles which are required for access to be granted).
9.5.1 Security and AOP Advice
If you’re familiar with AOP, you’d be aware there are different types of advice available: before, after, throws and around. An around advice is very useful, because an advisor can elect whether or not to proceed with a method invocation, whether or not to modify the response, and whether or not to throw an exception. Spring Security provides an around advice for method invocations as well as web requests. We achieve an around advice for method invocations using Spring’s standard AOP support and we achieve an around advice for web requests using a standard Filter.
9.5.2 Secure Objects and the AbstractSecurityInterceptor
So what is a "secure object" anyway? Spring Security uses the term to refer to any object that can have security (such as an authorization decision) applied to it. The most common examples are method invocations and web requests.
Each supported secure object type has its own interceptor class, which is a subclass of AbstractSecurityInterceptor
. Importantly, by the time the AbstractSecurityInterceptor
is called, the SecurityContextHolder
will contain a valid Authentication
if the principal has been authenticated.
AbstractSecurityInterceptor
provides a consistent workflow for handling secure object requests, typically:
- Look up the "configuration attributes" associated with the present request
查找与当前请求关联的“配置属性”
- Submitting the secure object, current
Authentication
and configuration attributes to theAccessDecisionManager
for an authorization decision将安全对象,当前身份验证和配置属性提交给AccessDecisionManager以进行授权决策 - Optionally change the
Authentication
under which the invocation takes place(可选)更改进行调用的身份验证 - Allow the secure object invocation to proceed (assuming access was granted)
允许安全对象调用继续(假设已授予访问权限)
- Call the
AfterInvocationManager
if configured, once the invocation has returned. If the invocation raised an exception, theAfterInvocationManager
will not be invoked.调用返回后,调用AfterInvocationManager(如果已配置)。如果调用引发异常,则不会调用AfterInvocationManager。
What are Configuration Attributes?
A "configuration attribute" can be thought of as a String that has special meaning to the classes used by AbstractSecurityInterceptor
. They are represented by the interface ConfigAttribute
within the framework. They may be simple role names or have more complex meaning, depending on the how sophisticated the AccessDecisionManager
implementation is. The AbstractSecurityInterceptor
is configured with a SecurityMetadataSource
which it uses to look up the attributes for a secure object. Usually this configuration will be hidden from the user.
<intercept-url pattern='/secure/**' access='ROLE_A,ROLE_B'/>
in the namespace introduction, this is saying that the configuration attributes ROLE_A
and ROLE_B
apply to web requests matching the given pattern. In practice, with the default AccessDecisionManager
configuration, this means that anyone who has a GrantedAuthority
matching either of these two attributes will be allowed access. Strictly speaking though, they are just attributes and the interpretation is dependent on the AccessDecisionManager
implementation. The use of the prefix ROLE_
is a marker to indicate that these attributes are roles and should be consumed by Spring Security’s RoleVoter
. This is only relevant when a voter-based AccessDecisionManager
is in use. We’ll see how the AccessDecisionManager
is implemented in the authorization chapter.RunAsManager
Assuming AccessDecisionManager
decides to allow the request, the AbstractSecurityInterceptor
will normally just proceed with the request. Having said that, on rare occasions users may want to replace the Authentication
inside the SecurityContext
with a different Authentication
, which is handled by the AccessDecisionManager
calling a RunAsManager
. This might be useful in reasonably unusual situations, such as if a services layer method needs to call a remote system and present a different identity. Because Spring Security automatically propagates security identity from one server to another (assuming you’re using a properly-configured RMI or HttpInvoker remoting protocol client), this may be useful.
AfterInvocationManager
Following the secure object invocation proceeding and then returning - which may mean a method invocation completing or a filter chain proceeding - the AbstractSecurityInterceptor
gets one final chance to handle the invocation. At this stage the AbstractSecurityInterceptor
is interested in possibly modifying the return object. We might want this to happen because an authorization decision couldn’t be made "on the way in" to a secure object invocation. Being highly pluggable, AbstractSecurityInterceptor
will pass control to an AfterInvocationManager
to actually modify the object if needed. This class can even entirely replace the object, or throw an exception, or not change it in any way as it chooses. The after-invocation checks will only be executed if the invocation is successful. If an exception occurs, the additional checks will be skipped.
AbstractSecurityInterceptor
and its related objects are shown in Figure 9.1, “Security interceptors and the "secure object" model”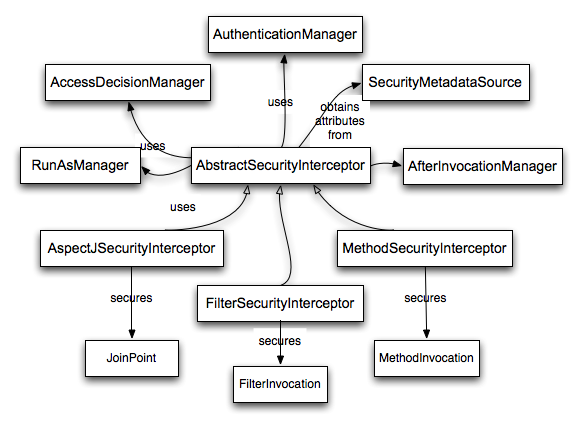
Extending the Secure Object Model
Only developers contemplating an entirely new way of intercepting and authorizing requests would need to use secure objects directly. For example, it would be possible to build a new secure object to secure calls to a messaging system. Anything that requires security and also provides a way of intercepting a call (like the AOP around advice semantics) is capable of being made into a secure object. Having said that, most Spring applications will simply use the three currently supported secure object types (AOP Alliance MethodInvocation
, AspectJ JoinPoint
and web request FilterInvocation
) with complete transparency.
Spring Security(三十):9.5 Access-Control (Authorization) in Spring Security的更多相关文章
- 精选Spring Boot三十五道必知必会知识点
Spring Boot 是微服务中最好的 Java 框架. 我们建议你能够成为一名 Spring Boot 的专家.本文精选了三十五个常见的Spring Boot知识点,祝你一臂之力! 问题一 Spr ...
- Browser security standards via access control
A computing system is operable to contain a security module within an operating system. This securit ...
- SpringBoot:三十五道SpringBoot面试题及答案
SpringBoot面试前言今天博主将为大家分享三十五道SpringBoot面试题及答案,不喜勿喷,如有异议欢迎讨论! Spring Boot 是微服务中最好的 Java 框架. 我们建议你能够成为一 ...
- Oracle Applications Multiple Organizations Access Control for Custom Code
档 ID 420787.1 White Paper Oracle Applications Multiple Organizations Access Control for Custom Code ...
- spring boot / cloud (十五) 分布式调度中心进阶
spring boot / cloud (十五) 分布式调度中心进阶 在<spring boot / cloud (十) 使用quartz搭建调度中心>这篇文章中介绍了如何在spring ...
- Spring Security(三十五):Part III. Testing
This section describes the testing support provided by Spring Security. 本节介绍Spring Security提供的测试支持. ...
- Spring Security(三十六):12. Spring MVC Test Integration
Spring Security provides comprehensive integration with Spring MVC Test Spring Security提供与Spring MVC ...
- spring boot 常见三十四问
Spring Boot 是微服务中最好的 Java 框架. 我们建议你能够成为一名 Spring Boot 的专家. 问题一 Spring Boot.Spring MVC 和 Spring 有什么区别 ...
- SELINUX、Security Access Control Strategy && Method And Technology Research - 安全访问控制策略及其方法技术研究
catalog . 引言 . 访问控制策略 . 访问控制方法.实现技术 . SELINUX 0. 引言 访问控制是网络安全防范和客户端安全防御的主要策略,它的主要任务是保证资源不被非法使用.保证网络/ ...
- Spring Security(十九):6. Security Namespace Configuration
6.1 Introduction Namespace configuration has been available since version 2.0 of the Spring Framewor ...
随机推荐
- Chapter 5 Blood Type——13
"Kryptonite doesn't bother me, either," he chuckled. “氪星石也不会影响我,” 他笑着说道. "You're not ...
- 使用mongoskin操作MongoDB
mongoskin是一个操作MongoDB的模型工具 相当于数据库类 与之相当的还有mongoose比较出名 安装模块(特地加了版本,这里被坑过,在Ubuntu中开发的好好的,部署到线上centos中 ...
- leetcode — path-sum
/** * Source : https://oj.leetcode.com/problems/path-sum/ * * * Given a binary tree and a sum, deter ...
- vnc server的安装
vnc是一款使用广泛的服务器管理软件,可以实现图形化管理.我在安装vnc server碰到一些问题,也整理下我的安装步骤,希望对博友们有一些帮助. 1 安装对应的软件包 [root@centos6 ~ ...
- GBDT 算法:原理篇
本文由云+社区发表 GBDT 是常用的机器学习算法之一,因其出色的特征自动组合能力和高效的运算大受欢迎. 这里简单介绍一下 GBDT 算法的原理,后续再写一个实战篇. 1.决策树的分类 决策树分为两大 ...
- MySQL备份与恢复之percona-xtrabackup实现增量备份及恢复 实例
innobackupex 的使用方法1.完全备份 参数一是完全备份地址 完全备份到/data/mysql/back_up/all_testdb_20140612 目录下innobackupex --u ...
- MariaDB主从复制的逻辑与实现
一.关系型数据库的劣势 “关系型数据库:指采用了关系模型来组织数据的数据库,而关系模型指的就是二维表格模型,而一个关系型数据库就是由二维表及其之间的联系所组成的一个数据组织.”——Wiki 关系型数据 ...
- Springboot 系列(三)Spring Boot 自动配置原理
注意:本 Spring Boot 系列文章基于 Spring Boot 版本 v2.1.1.RELEASE 进行学习分析,版本不同可能会有细微差别. 前言 关于配置文件可以配置的内容,在 Spring ...
- 第25章 退出外部身份提供商 - Identity Server 4 中文文档(v1.0.0)
当用户注销 IdentityServer并且他们使用外部身份提供程序登录时,可能会将其重定向到注销外部提供程序.并非所有外部提供商都支持注销,因为它取决于它们支持的协议和功能. 要检测是否必须将用户重 ...
- 【代码笔记】Web-CSS-CSS盒子模型
一,效果图. 二,代码. <!DOCTYPE html> <html> <head> <meta charset="utf-8"> ...