[08] AOP基本概念和使用
1、什么是AOP
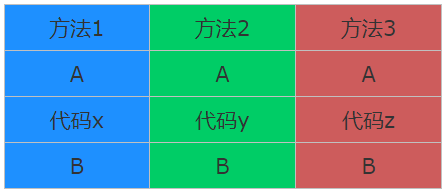
2、Spring AOP
- 切面:封装通用业务逻辑的组件,即我们想要插入的代码内容
- 切入点:指定哪些Bean组件的哪些方法使用切面组件
- 通知:用于指定具体作用的位置,是方法之前或之后等等
- 前置通知(before) - 在目标方法被调用之前调用通知功能
- 后置通知(after) - 在目标方法完成之后调用通知(不论程序是否出现异常),此时不会关心方法的输出是什么
- 返回通知(after-returning) - 在目标方法成功执行之后调用通知
- 异常通知(after-throwing) - 在目标方法抛出异常后调用通知
- 环绕通知(around) - 通知包裹了被通知的方法,在被通知的方法调用之前和调用之后执行自定义的行为
- 目标程序,某个需要被插入通用代码片段的方法
- 切面程序,即通用代码,用来插入方法的那些代码片段(无返回类型,参数类型与通知类型有关)
- 配置文件,用来指定切入点和通知
3、Demo示例和说明
3.1相关配置环境
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>4.3.16.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aspects</artifactId>
<version>4.3.16.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>4.3.16.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aspects</artifactId>
<version>4.3.16.RELEASE</version>
</dependency>
3.2 准备一个目标程序
public class Target {
public void print(String param) {
System.out.println("I'm a target function and my param is [" + param + "]" );
}
}
public class Target {
public void print(String param) {
System.out.println("I'm a target function and my param is [" + param + "]" );
}
}
3.3 编写切面程序
- 切面程序必须无返回值,即void
- org.aspectj.lang.JoinPoint 封装了切面方法的参数和对象等,可通过它获取相关内容
- 切面程序的参数除JoinPoint外,还与通知类型有关,如后置通知则可以获取目标程序返回值(需要配置,此处不展开)
public class Section {
public void writeLog(JoinPoint point) {
System.out.println("write logs start");
System.out.println("获取目标函数的参数: " + Arrays.toString(point.getArgs()));
System.out.println("获取目标函数的反射对象: " + point.getSignature());
System.out.println("获取目标函数的所在对象: " + point.getTarget());
System.out.println("write logs end");
}
}
public class Section {
public void writeLog(JoinPoint point) {
System.out.println("write logs start");
System.out.println("获取目标函数的参数: " + Arrays.toString(point.getArgs()));
System.out.println("获取目标函数的反射对象: " + point.getSignature());
System.out.println("获取目标函数的所在对象: " + point.getTarget());
System.out.println("write logs end");
}
}
3.4 编写AOP配置文件
<bean id="target" class="dulk.learn.aop.Target"/>
<bean id="section" class="dulk.learn.aop.Section"/>
<!-- AOP配置的根标签,所有AOP配置都在其内部 -->
<aop:config>
<!-- 配置AOP的切入点,expression为切入点表达式 -->
<aop:pointcut id="targetPointCut" expression="execution(* dulk.learn.aop.Target.print(*))" />
<!-- 配置切面,ref 切面对象 -->
<aop:aspect ref="section">
<!-- 配置通知为前置,method为方法,pointcut-ref作用在哪些切入点 -->
<aop:before method="writeLog" pointcut-ref="targetPointCut"/>
</aop:aspect>
</aop:config>
<bean id="target" class="dulk.learn.aop.Target"/>
<bean id="section" class="dulk.learn.aop.Section"/>
<!-- AOP配置的根标签,所有AOP配置都在其内部 -->
<aop:config>
<!-- 配置AOP的切入点,expression为切入点表达式 -->
<aop:pointcut id="targetPointCut" expression="execution(* dulk.learn.aop.Target.print(*))" />
<!-- 配置切面,ref 切面对象 -->
<aop:aspect ref="section">
<!-- 配置通知为前置,method为方法,pointcut-ref作用在哪些切入点 -->
<aop:before method="writeLog" pointcut-ref="targetPointCut"/>
</aop:aspect>
</aop:config>
3.4.1 切入表达式
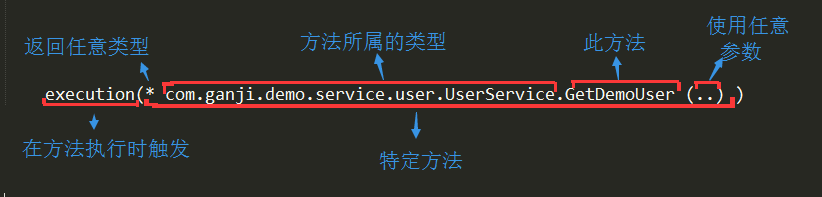
3.4.2 切面配置说明
<!-- 配置切面,ref 切面对象的beanId -->
<aop:aspect ref="section">
<!-- before表通知为前置,method为插入的方法,pointcut-ref作用在哪些切入点(aop:pointcut id) -->
<aop:before method="writeLog" pointcut-ref="targetPointCut"/>
</aop:aspect>
<!-- 配置切面,ref 切面对象的beanId -->
<aop:aspect ref="section">
<!-- before表通知为前置,method为插入的方法,pointcut-ref作用在哪些切入点(aop:pointcut id) -->
<aop:before method="writeLog" pointcut-ref="targetPointCut"/>
</aop:aspect>
3.5 测试程序和结果
public class AOPTest {
@Test
public void testAOP(){
ApplicationContext context = new ClassPathXmlApplicationContext("/applicationContext.xml");
Target target = (Target) context.getBean("target");
target.print("balabala");
}
}
public class AOPTest {
@Test
public void testAOP(){
ApplicationContext context = new ClassPathXmlApplicationContext("/applicationContext.xml");
Target target = (Target) context.getBean("target");
target.print("balabala");
}
}
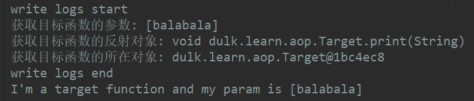
4、参考链接
[08] AOP基本概念和使用的更多相关文章
- Spring AOP基本概念
Spring AOP基本概念 目录 Spring AOP定义 AOP基本术语 通知类型 AOP定义 AOP基本术语 切面( Aspect ):一个能横切多个对象的模块化的关注点.对Spring AOP ...
- Spring入门篇——AOP基本概念
1.什么是AOP及实现方式 什么是AOP AOP:Aspect Oriented Programming的缩写,意为:面向切面编程,通过预编译方式和运行期动态代理实现程序功能的统一维护的一种技术 主要 ...
- Spring入门篇——第5章 Spring AOP基本概念
第5章 Spring AOP基本概念 本章介绍Spring中AOP的基本概念和应用. 5-1 AOP基本概念及特点 5-2 配置切面aspect ref:引用另外一个Bean 5-3 配置切入点Poi ...
- Spring学习总结(1)——Spring AOP的概念理解
1.我所知道的aop 初看aop,上来就是一大堆术语,而且还有个拉风的名字,面向切面编程,都说是OOP的一种有益补充等等.一下子让你不知所措,心想着:怪不得 很多人都和我说aop多难多难 .当我看进去 ...
- Spring Aop重要概念介绍及应用实例结合分析
转自:http://bbs.csdn.net/topics/390811099 此前对于AOP的使用仅限于声明式事务,除此之外在实际开发中也没有遇到过与之相关的问题.最近项目中遇到了以下几点需求,仔细 ...
- Spring AOP入门——概念和注意事项
AOP什么? AOP在功能方面,它是之前和之后运行一些业务逻辑,一些操作(比方记录日志.或者是推断是否有权限等),这些操作的加入.全然不耦合于原来的业务逻辑.从而对原有业务逻辑全然是透明. 也就是说. ...
- Spring学习(18)--- AOP基本概念及特点
AOP:Aspect Oriented Programing的缩写,意为:面向切面编程,通过预编译方式和运行期动态代理实现程序程序功能的统一维护的一种技术 主要的功能是:日志记录,性能统计,安全控制, ...
- Spring Core Programming(Spring核心编程) - AOP Concepts(AOP基本概念)
1. What is aspect-oriented programming?(什么是面向切面编程?) Aspects help to modularize cross-cutting concern ...
- Spring课程 Spring入门篇 5-1 aop基本概念及特点
概念: 1 什么是aop及实现方式 2 aop的基本概念 3 spring中的aop 1 什么是aop及实现方式 1.1 aop,面向切面编程,比如:唐僧取经需要经过81难,多一难少一难都不行.孙悟空 ...
随机推荐
- php curl中x-www-form-urlencoded与multipart/form-data 方式 Post 提交数据详解
multipart/form-data 方式 post的curl库,模拟post提交的时候,默认的方式 multipart/form-data ,这个算是post提交的几个基础的实现方式. $post ...
- stylus解决移动端1像素边框的问题
首先 我是借用了yo框架的border和他的媒体查询组合 这两个分别是在yo>lib>core>classes>_border.scss(用来获取yo框架封装的border) ...
- python之字典(dict)
字典:一种可变容器模型,且可存储任意类型对象,如字符串.数字.元组等其他容器模型. 字典由键和对应值成对组成 {key:value,key1,value1}, 例如: dic = {'中国': '汉语 ...
- 在vue配置sass
先npm两个插件 npm install sass-loader --save-dev npm install node-sass --save-dev 然后在webpack当中配置 { test: ...
- SSM 开发 Tars
目录结构 tars生成的文件当成 controller 来调用 service ,service 调用 mapper POM 注意如果 mybatis是3.4.1 spring 是4.1.14的话, ...
- @DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") 前台request 获取body的格式是正确的 (2018-03-23 16:44:22) 但是Java 后台却格式化成了yyyy-MM-dd的格式 巨坑(@InitBinder搞得贵)
最近做项目时,同事写的功能总是格式化时间不正确,Java类属性明明注解了@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") 但就是硬 ...
- CGI,FastCGI,PHP-FPM,PHP-CLI,modPHP
This might give you a broader understanding of their difference: CGI: (common gateway interface) It ...
- java基础面试题(Servlet生命周期)
Servlet运行在Servlet容器中,其生命周期由容器来管理.Servlet的生命周期通过javax.servlet.Servlet接口中的init().service()和destroy()方法 ...
- 控件_RadioGroup&&RadioButton(单选按钮)和Toast
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools=&q ...
- sys.argv[]使用
sys.argv[]说白了就是一个从程序外部获取参数的桥梁,这个"外部"很关键,所以那些试图从代码来说明它作用的解释一直没看明白.因为我们从外部取得的参数可以是多个,所以获得的是一 ...