DP专题训练之HDU 1506 Largest Rectangle in a Histogram
Description
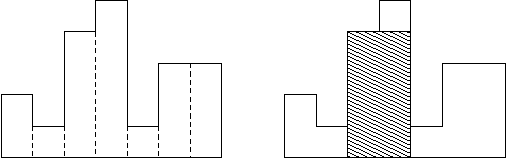
Usually,
histograms are used to represent discrete distributions, e.g., the
frequencies of characters in texts. Note that the order of the
rectangles, i.e., their heights, is important. Calculate the area of the
largest rectangle in a histogram that is aligned at the common base
line, too. The figure on the right shows the largest aligned rectangle
for the depicted histogram.
Input
histogram and starts with an integer n, denoting the number of
rectangles it is composed of. You may assume that 1 <= n <=
100000. Then follow n integers h1, ..., hn, where 0 <= hi <=
1000000000. These numbers denote the heights of the rectangles of the
histogram in left-to-right order. The width of each rectangle is 1. A
zero follows the input for the last test case.
Output
rectangle in the specified histogram. Remember that this rectangle must
be aligned at the common base line.
Sample Input
Sample Output
看别人的题解才知道大概的思路。还是要多做题,多想题~~
题目意思很简单,就算看不懂题目,看图+样例也可以了解。
大意就是:给你一串数字,代表着几个互相连接的矩形,每个数字代表着一个矩形的高,让你求可以得到的最大的矩形面积。(结合题目的图更易看懂~)
看上去我们似乎又要求长,又要求高,似乎很难~~(当时我就是这么想的~~)
但其实,我们可以其中的一个点为中心,然后找左边不大于中心点的矩形的个数,再找右边不大于中心点的矩形的个数,两边个数相加就是矩形的长,而这个中心点的高度就是我们求的矩形的高度~~具体代码如下:
//Asimple
#include <iostream>
#include <sstream>
#include <algorithm>
#include <cstring>
#include <cstdio>
#include <vector>
#include <cctype>
#include <cstdlib>
#include <stack>
#include <cmath>
#include <set>
#include <map>
#include <string>
#include <queue>
#include <limits.h>
#include <time.h>
#define INF 0xfffffff
#define mod 1000000
#define swap(a,b,t) t = a, a = b, b = t
#define CLS(a, v) memset(a, v, sizeof(a))
#define debug(a) cout << #a << " = " << a <<endl
#define abs(x) x<0?-x:x
#define srd(a) scanf("%d", &a)
#define src(a) scanf("%c", &a)
#define srs(a) scanf("%s", a)
#define srdd(a,b) scanf("%d %d",&a, &b)
#define srddd(a,b,c) scanf("%d %d %d",&a, &b, &c)
#define prd(a) printf("%d\n", a)
#define prdd(a,b) printf("%d %d\n",a, b)
#define prs(a) printf("%s\n", a)
#define prc(a) printf("%c", a)
using namespace std;
typedef long long ll;
const int maxn = ;
ll n, m, num, T, k;
ll a[maxn], l[maxn], r[maxn]; void input() {
while( ~scanf("%lld", &n) && n ) {
for(int i=; i<=n; i++) {
scanf("%lld", &a[i]);
}
l[] = ;
r[n] = n;
//左边不小于a[i]的数
for(int i=; i<=n; i++) {
k = i;
while( k > && a[i]<=a[k-] ) {
k = l[k-];
}
l[i] = k;
}
//右边不小于a[i]的数
for(int i=n-; i>=; i--) {
k = i;
while( k<n && a[i]<=a[k+]) {
k = r[k+];
}
r[i] = k;
}
ll Max = ;
for(int i=; i<=n; i++) {
if( (r[i]-l[i]+)*a[i] > Max ) {
Max = (r[i]-l[i]+)*a[i];
}
}
printf("%lld\n", Max);
}
} int main(){
input();
return ;
}
DP专题训练之HDU 1506 Largest Rectangle in a Histogram的更多相关文章
- HDU 1506 Largest Rectangle in a Histogram (dp左右处理边界的矩形问题)
E - Largest Rectangle in a Histogram Time Limit:1000MS Memory Limit:32768KB 64bit IO Format: ...
- HDU 1506 Largest Rectangle in a Histogram(区间DP)
题目网址:http://acm.hdu.edu.cn/showproblem.php?pid=1506 题目: Largest Rectangle in a Histogram Time Limit: ...
- HDU 1506 Largest Rectangle in a Histogram(DP)
Largest Rectangle in a Histogram Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 ...
- HDU 1506 Largest Rectangle in a Histogram set+二分
Largest Rectangle in a Histogram Problem Description: A histogram is a polygon composed of a sequenc ...
- hdu 1506 Largest Rectangle in a Histogram 构造
题目链接:HDU - 1506 A histogram is a polygon composed of a sequence of rectangles aligned at a common ba ...
- Hdu 1506 Largest Rectangle in a Histogram 分类: Brush Mode 2014-10-28 19:16 93人阅读 评论(0) 收藏
Largest Rectangle in a Histogram Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 ...
- hdu 1506 Largest Rectangle in a Histogram(单调栈)
L ...
- HDU -1506 Largest Rectangle in a Histogram&&51nod 1158 全是1的最大子矩阵 (单调栈)
单调栈和队列讲解:传送门 HDU -1506题意: 就是给你一些矩形的高度,让你统计由这些矩形构成的那个矩形面积最大 如上图所示,如果题目给出的全部是递增的,那么就可以用贪心来解决 从左向右依次让每一 ...
- HDU 1506 Largest Rectangle in a Histogram【DP】
题意:坐标轴上有连续的n个底均为1,高为h[i]的矩形,求能够构成的最大矩形的面积. 学习的别人的代码 @_@ 看底的坐标怎么找的看了好一会儿--- 记l[i]为矩形的底的左边的坐标,就将它一直向左扩 ...
随机推荐
- selenium webdriver自动化测试
selenium家族介绍 Selenium IDE:Selenium IDE是嵌入到Firefox浏览器中的一个插件,实现简单的浏览器操作的录制与回放功能. Selenium ...
- 总结-javascript
是否可见 $('.btn-accomplish').is(':visible') 通过ajax提交时, {a: vA | ''}; vA没有时,服务器得到的a为"0".如果是两丨, ...
- 模拟jquery的$()选择器的实现
<html> <head> </head> <body> <div id="div1">div1</div> ...
- Apache Kafka - Schema Registry
关于我们为什么需要Schema Registry? 参考, https://www.confluent.io/blog/how-i-learned-to-stop-worrying-and-love- ...
- Vmware安装Centos NAT方式设置静态IP
[Vmware中在搭建集群环境等,DHCP自动获取IP方式不方便,为了固定IP减少频繁更改配置信息,建议使用静态IP来配置,网络连接主要有三种方式 1.nat 2.桥接,3主机模式 ,在这里主要介NA ...
- 退出recoveyr模式的iOS设备
大致分析了一下几款工具,大概流程是: 使用AMDRestoreRegisterForDeviceNotifications来监听设备的连接. 监听设备连接的回调函数中获取设备的句柄. 调用AMReco ...
- js滚动条滚动到某个元素位置
scrollTo(0,document.getElementById('xxx').offset().top);
- vert.x学习(一),开篇之hello world
今天决定学习下vert.x这个框架,记录下学习笔记. 下面列下我的开发环境: Java版本 1.8 maven版本 3.3 IDEA版本 2016 在idea中使用vert.x不用下载或安装其他东西了 ...
- 正则表达式中的exec和match方法的区别
正则表达式中的exec和match方法的区别 字符串的正则方法有:match().replace().search().split() 正则对象的方法有:exec().test() 1.match m ...
- chrome一直提示adobe flash player 因过期而遭阻止
链接:https://www.zhihu.com/question/32223811/answer/128088278 很多新用户在安装了Chrome浏览器或者更新过的的时候,经常提示 adobe f ...