SilverLight: 数据绑定(1)-绑定到数据对象
ylbtech-SilverLight-DataBinding: Binding to Data Objects(绑定到数据对象) |
- 1.A, Building a Data Object(创建一个数据对象)
- 1.B, Displaying a Data Object with Datacontext(显示一个数据对象与DataContext)
- 1.C, Storing a Data Object as a Resource(存储一个数据对象作为一个资源)
- 1.D, Editing with Two-Way Bindings(编辑与双向绑定)[未实现]
1.A, Building a Data Object(创建一个数据对象)返回顶部 |
using System; using System.Collections.Generic;
using System.Linq;
using System.ComponentModel.DataAnnotations;
namespace SLYlbtechApp.Access
{
/// <summary>
/// 产品类
/// </summary>
public class Product
{
int productId;
/// <summary>
/// 编号【PK】
/// </summary>
public int ProductId
{
get { return productId; }
set { productId = value; }
}
string productName;
/// <summary>
/// 产品名称
/// </summary>
public string ProductName
{
get
{
return productName;
}
set
{
if (value=="") throw new ArgumentException("不能为空");
productName = value;
}
}
string quantityPerUnit;
/// <summary>
/// 产品规格
/// </summary>
public string QuantityPerUnit
{
get { return quantityPerUnit; }
set { quantityPerUnit = value; }
}
/// <summary>
/// 单位价格
/// 注意 不要使用 decimal 类型,在 UserControl.Resounrce 资源绑定是引发类型转换异常
/// </summary>
double unitPrice;
[Display(Name="价格",Description="价格不能小于0")]
public double UnitPrice
{
get { return unitPrice; }
set
{
if (value < ) throw new ArgumentException("不能小于0");
unitPrice = value;
}
}
string description;
/// <summary>
/// 描述
/// </summary>
public string Description
{
get { return description; }
set { description = value; }
}
string productImagePath;
/// <summary>
/// 产品图片地址
/// </summary>
public string ProductImagePath
{
get { return productImagePath; }
set { productImagePath = value; }
}
string categoryName;
/// <summary>
/// 类别名称
/// </summary>
public string CategoryName
{
get { return categoryName; }
set { categoryName = value; }
} /// <summary>
/// 空参构造
/// </summary>
public Product()
{ }
/// <summary>
/// 全参构造
/// </summary>
/// <param name="productId"></param>
/// <param name="productName"></param>
/// <param name="quantityPerUnit"></param>
/// <param name="unitPrice"></param>
/// <param name="description"></param>
/// <param name="productImagePath"></param>
/// <param name="categoryName"></param>
public Product(int productId, string productName, string quantityPerUnit, double unitPrice, string description
, string productImagePath, string categoryName)
{
ProductId = productId;
ProductName=productName;
QuantityPerUnit=quantityPerUnit;
UnitPrice=unitPrice;
Description=description; ProductImagePath=productImagePath;
CategoryName=categoryName;
} /// <summary>
/// 获取全部产品
/// </summary>
/// <returns></returns>
public static IList<Product> GetAll()
{
IList<Product> dals = new List<Product>();
string categoryName = string.Empty;
string productImagePath = string.Empty;
string description = "嗟夫!草木无情,有时飘零。人为动物,惟物之灵。百忧感其心,万事劳其形,有动于中,必摇其精。而况思其力之所不及,忧其智之所不能,宜其渥然丹者为槁木,黟然黑者为星星。奈何以非金石之质,欲与草木而争荣?念谁为之戕贼,亦何恨乎秋声!";
#region Add Product #region 饮料
categoryName = "饮料";
productImagePath = "../Images/pencil.jpg"; dals.Add(new Product()
{
ProductId = ,
ProductName = "啤酒",
QuantityPerUnit = "1箱*6听",
UnitPrice = 10d,
CategoryName = categoryName
,
ProductImagePath = productImagePath,
Description = description
}); dals.Add(new Product()
{
ProductId = ,
ProductName = "绿茶",
QuantityPerUnit = "500ml",
UnitPrice = 3d,
CategoryName = categoryName
,
ProductImagePath = productImagePath,
Description = description
}); dals.Add(new Product()
{
ProductId = ,
ProductName = "红茶",
QuantityPerUnit = "500ml",
UnitPrice = 3d,
CategoryName = categoryName
,
ProductImagePath = productImagePath,
Description = description
});
dals.Add(new Product()
{
ProductId = ,
ProductName = "纯净水",
QuantityPerUnit = "500ml",
UnitPrice = 1.5d,
CategoryName = categoryName
,
ProductImagePath = productImagePath,
Description = description
});
#endregion #region 零食
categoryName = "零食";
productImagePath = "../Images/pencil2.jpg"; dals.Add(new Product()
{
ProductId = ,
ProductName = "奥利奥巧克力饼干",
QuantityPerUnit = "10 boxes x 20 bags",
UnitPrice = 30d,
CategoryName = categoryName
,
ProductImagePath = productImagePath,
Description = description
}); dals.Add(new Product()
{
ProductId = ,
ProductName = "玻璃 海苔",
QuantityPerUnit = "20g",
UnitPrice = 13d,
CategoryName = categoryName
,
ProductImagePath = productImagePath,
Description = description
}); dals.Add(new Product()
{
ProductId = ,
ProductName = "好丽友 薯片",
QuantityPerUnit = "100g",
UnitPrice = 3d,
CategoryName = categoryName
,
ProductImagePath = productImagePath,
Description = description
});
dals.Add(new Product()
{
ProductId = ,
ProductName = "统一 老坛酸菜面",
QuantityPerUnit = "1 盒",
UnitPrice = 8.5d,
CategoryName = categoryName
,
ProductImagePath = productImagePath,
Description = description
});
#endregion #endregion
return dals;
}
/// <summary>
/// GetModel
/// </summary>
/// <returns></returns>
public static Product GetModel()
{
Product dal = null; string categoryName = string.Empty;
string productImagePath = string.Empty;
string description = "嗟夫!草木无情,有时飘零。人为动物,惟物之灵。百忧感其心,万事劳其形,有动于中,必摇其精。而况思其力之所不及,忧其智之所不能,宜其渥然丹者为槁木,黟然黑者为星星。奈何以非金石之质,欲与草木而争荣?念谁为之戕贼,亦何恨乎秋声!"; categoryName = "饮料";
productImagePath = "Images/pencil.jpg";
dal = new Product()
{
ProductId = ,
ProductName = "啤酒",
QuantityPerUnit = "1箱*6听",
UnitPrice=3.5,
CategoryName = categoryName
,
ProductImagePath = productImagePath,
Description = description
};
//dal._unitPrice = 10d;
return dal;
}
/// <summary>
/// GetModel
/// </summary>
/// <param name="id">产品编号</param>
/// <returns></returns>
public static Product GetModel(int id)
{
Product dal = null;
IList<Product> dals = GetAll();
try
{
dal = dals.Single(p => p.ProductId == id);
}
catch { }
finally
{
dals = null;
}
return dal;
}
}
}
4,
1.B, Displaying a Data Object with Datacontext(显示一个数据对象与DataContext)返回顶部 |
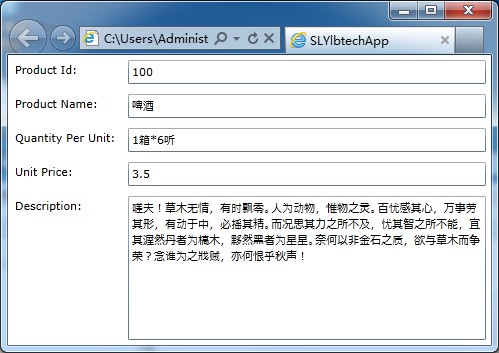
<Grid x:Name="gridDetailProduct">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="*"></RowDefinition>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="115"></ColumnDefinition>
<ColumnDefinition Width="*"></ColumnDefinition>
</Grid.ColumnDefinitions>
<TextBlock Grid.Row="0" Grid.Column="0" Margin="7">Product Id:</TextBlock>
<TextBox Grid.Row="0" Grid.Column="1" Margin="5" Text="{Binding ProductId}"></TextBox> <TextBlock Grid.Row="1" Grid.Column="0" Margin="7">Product Name:</TextBlock>
<TextBox Grid.Row="1" Grid.Column="1" Margin="5" Text="{Binding ProductName}"></TextBox> <TextBlock Grid.Row="2" Grid.Column="0" Margin="7">Quantity Per Unit:</TextBlock>
<TextBox Grid.Row="2" Grid.Column="1" Margin="5" Text="{Binding QuantityPerUnit}"></TextBox> <TextBlock Grid.Row="3" Grid.Column="0" Margin="7">Unit Price:</TextBlock>
<TextBox Grid.Row="3" Grid.Column="1" Margin="5" Text="{Binding UnitPrice}"></TextBox> <TextBlock Grid.Row="4" Grid.Column="0" Margin="7">Description:</TextBlock>
<TextBox Grid.Row="4" Grid.Column="1" Grid.RowSpan="2" Margin="5"
Text="{Binding Description}" TextWrapping="Wrap" ></TextBox>
</Grid>
2.3/3,
using System.Windows.Controls; using SLYlbtechApp.Access;
namespace SLYlbtechApp.ABindingToDataObjects
{
public partial class Template1 : UserControl
{
public Template1()
{
InitializeComponent(); this.gridDetailProduct.DataContext = Product.GetModel(); //显示一个数据对象与DataContext
}
}
}
3,
1.C, Storing a Data Object as a Resource(存储一个数据对象作为一个资源)返回顶部 |
xmlns:local="clr-namespace:SLYlbtechApp.Access"
2.2/3,
<!--用户空间资源 begin-->
<!--remark:请在UserControl 里注册引用空间 xmlns:local="clr-namespace:SLYlbtechApp.Access"-->
<!--Problem:UnitPrice 类型转换异常-->
<UserControl.Resources>
<local:Product x:Name="resourceProduct" ProductId="100" ProductName="苹果" QuantityPerUnit="1斤" UnitPrice="7"
Description="一天一个苹果,病害远离我。"></local:Product>
</UserControl.Resources>
<!--用户空间资源 end--> <Grid x:Name="LayoutRoot" Background="White">
<Grid x:Name="gridDetailProduct" DataContext="{StaticResource resourceProduct}">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="Auto"></RowDefinition>
<RowDefinition Height="*"></RowDefinition>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="115"></ColumnDefinition>
<ColumnDefinition Width="*"></ColumnDefinition>
</Grid.ColumnDefinitions>
<TextBlock Grid.Row="0" Grid.Column="0" Margin="7">Product Id:</TextBlock>
<TextBox Grid.Row="0" Grid.Column="1" Margin="5" Text="{Binding ProductId}"></TextBox> <TextBlock Grid.Row="1" Grid.Column="0" Margin="7">Product Name:</TextBlock>
<TextBox Grid.Row="1" Grid.Column="1" Margin="5"
Text="{Binding ProductName,Source={StaticResource resourceProduct}}"></TextBox> <TextBlock Grid.Row="2" Grid.Column="0" Margin="7">Quantity Per Unit:</TextBlock>
<TextBox Grid.Row="2" Grid.Column="1" Margin="5" Text="{Binding QuantityPerUnit}"></TextBox> <TextBlock Grid.Row="3" Grid.Column="0" Margin="7">Unit Price:</TextBlock>
<TextBox Grid.Row="3" Grid.Column="1" Margin="5" Text="{Binding UnitPrice}"></TextBox> <TextBlock Grid.Row="4" Grid.Column="0" Margin="7">Description:</TextBlock>
<TextBox Grid.Row="4" Grid.Column="1" Grid.RowSpan="2" Margin="5"
Text="{Binding Description}" TextWrapping="Wrap" ></TextBox>
</Grid>
</Grid>
2.3/3,[无]
<TextBox Grid.Row="1" Grid.Column="1" Margin="5" Text="{Binding ProductName,Source={StaticResource resourceProduct}}"></TextBox>
1.D, Editing with Two-Way Bindings(编辑与双向绑定)[未实现]返回顶部 |
1.E, 返回顶部 |
![]() |
作者:ylbtech 出处:http://ylbtech.cnblogs.com/ 本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。 |
SilverLight: 数据绑定(1)-绑定到数据对象的更多相关文章
- Silverlight数据绑定之 绑定一个int类型的属性
还就真心不会啊! 在类FunctionPanel中作如下定义: /// <summary> /// 鼠标状态 属性 /// </summary> public Dependen ...
- class 绑定的数据对象不必内联定义在模板里
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8&quo ...
- Silverlight数据绑定之DataGrid
Silverlight数据绑定之DataGrid 时间:2011-08-03 01:59来源:网易博客 作者:Wilson. 点击:次 注:所有代码以C#为例 DataGrid绑定的数据对象: 1.D ...
- Angular4.x 创建组件|绑定数据|绑定属性|数据循环|条件判断|事件|表单处理|双向数据绑定
Angular4.x 创建组件|绑定数据|绑定属性|数据循环|条件判断|事件|表单处理|双向数据绑定 创建 angular 组件 https://github.com/angular/angular- ...
- WPFS数据绑定(要是后台类对象的属性值发生改变,通知在“client界面与之绑定的控件值”也发生改变须要实现INotitypropertyChanged接口)
WPFS数据绑定(要是后台类对象的属性值发生改变,通知在"client界面与之绑定的控件值"也发生改变须要实现INotitypropertyChanged接口) MainWindo ...
- 背水一战 Windows 10 (20) - 绑定: DataContextChanged, UpdateSourceTrigger, 对绑定的数据做自定义转换
[源码下载] 背水一战 Windows 10 (20) - 绑定: DataContextChanged, UpdateSourceTrigger, 对绑定的数据做自定义转换 作者:webabcd 介 ...
- 绑定: DataContextChanged, UpdateSourceTrigger, 对绑定的数据做自定义转换
介绍背水一战 Windows 10 之 绑定 DataContextChanged - FrameworkElement 的 DataContext 发生变化时触发的事件 UpdateSourceTr ...
- Silverlight实例教程 – Datagrid,Dataform数据验证和ValidationSummary(转载)
Silverlight 4 Validation验证实例系列 Silverlight实例教程 - Validation数据验证开篇 Silverlight实例教程 - Validation数据验证基础 ...
- SpringMVC数据绑定全面示例(复杂对象,数组等)
点击链接查询原文 http://www.xdemo.org/springmvc-data-bind/ 已经使用SpringMVC开发了几个项目,平时也有不少朋友问我数据怎么传输,怎么绑定之类的话题,今 ...
随机推荐
- 令人惊叹的sublime text 3 插件
1.ChineseLocalization------语言汉化.(新手必备) 2.SublimeTmpl------打开生成模板.(新手必备) 3.SublimeCodeIntel------代码自 ...
- AtCoder Regular Contest 083
C - Sugar Water Time limit : 3sec / Memory limit : 256MB Score : 300 points Problem Statement Snuke ...
- 矩阵快速幂在ACM中的应用
矩阵快速幂在ACM中的应用 16计算机2黄睿博 首发于个人博客http://www.cnblogs.com/BobHuang/ 作为一个acmer,矩阵在这个算法竞赛中还是蛮多的,一个优秀的算法可以影 ...
- centos7下nginx添加到自定义系统服务中提示Access denied
安装好nginx后,添加到系统服务中 在/usr/lib/systemd/system下创建nginx.service文件内容如下: [Unit] Description=nginx - high p ...
- 【java基础 17】集合中各实现类的性能分析
大致的再回顾一下java集合框架的基本情况 一.各Set实现类的性能分析 1.1,HashSet用于添加.查询 HashSet和TreeSet是Set的两个典型实现,HashSet的性能总是比Tree ...
- debian 切换最新源
deb http://ftp.cn.debian.org/debian sid main#deb http://ftp.debian.org/debian/ wheezy main
- [luoguP2766] 最长递增子序列问题(最大流)
传送门 题解来自网络流24题: [问题分析] 第一问时LIS,动态规划求解,第二问和第三问用网络最大流解决. [建模方法] 首先动态规划求出F[i],表示以第i位为开头的最长上升序列的长度,求出最长上 ...
- Codeforces834D - The Bakery
Portal Description 给出一个\(n(n\leq35000)\)个数的数列\(\{a_i\}\)和\(m(m\leq50)\).将原数列划分成\(m\)个连续的部分,每个部分的权值等于 ...
- BZOJ1150 [CTSC2007]数据备份Backup 【堆 + 链表】
题目 你在一家 IT 公司为大型写字楼或办公楼(offices)的计算机数据做备份.然而数据备份的工作是枯燥乏味 的,因此你想设计一个系统让不同的办公楼彼此之间互相备份,而你则坐在家中尽享计算机游戏的 ...
- python解析yaml文件
YAML语法规则: http://www.ibm.com/developerworks/cn/xml/x-cn-yamlintro/ 下载PyYAML: http://www.yaml.org/ 解压 ...