Pipe(点积叉积的应用POJ1039)
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 9723 | Accepted: 2964 |
Description
each component of the pipe. Note that the material which the pipe is made from is not transparent and not light reflecting.
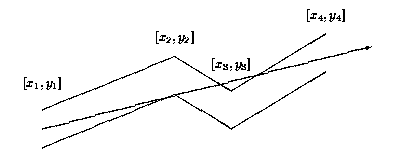
Each pipe component consists of many straight pipes connected tightly together. For the programming purposes, the company developed the description of each component as a sequence of points [x1; y1], [x2; y2], . . ., [xn; yn], where x1 < x2 < . . . xn . These
are the upper points of the pipe contour. The bottom points of the pipe contour consist of points with y-coordinate decreased by 1. To each upper point [xi; yi] there is a corresponding bottom point [xi; (yi)-1] (see picture above). The company wants to find,
for each pipe component, the point with maximal x-coordinate that the light will reach. The light is emitted by a segment source with endpoints [x1; (y1)-1] and [x1; y1] (endpoints are emitting light too). Assume that the light is not bent at the pipe bent
points and the bent points do not stop the light beam.
Input
by space. The last block is denoted with n = 0.
Output
or the message Through all the pipe.. The real value is the desired maximal x-coordinate of the point where the light can reach from the source for corresponding pipe component. If this value equals to xn, then the message Through all the pipe. will appear
in the output file.
Sample Input
4
0 1
2 2
4 1
6 4
6
0 1
2 -0.6
5 -4.45
7 -5.57
12 -10.8
17 -16.55
0
Sample Output
4.67
Through all the pipe.
Source
题意:光线从(x0,uy0),(x0,y0-1)之间射入,向四面八方直线传播,问光线最远能射到哪里,或者能穿透整个通道.
思路:如果一条直线没有碰到一个端点,不是最优的,可以通过平移使之变成最优的,如果只碰到一个端点,也可以通过旋转使之交到两个端点,所以现在的任务就是枚举端点,找最大值
黑书(P359)
#include <set>
#include <map>
#include <list>
#include <stack>
#include <cmath>
#include <vector>
#include <queue>
#include <string>
#include <cstdio>
#include <cstdlib>
#include <cstring>
#include <iostream>
#include <algorithm>
using namespace std;
typedef long long LL;
const double eps = 1e-5;
const int INF = 0x3f3f3f3f;
struct node
{
double x;
double y;
}up[21],down[21]; //折点
int n;
int dblcmp(double p)//判断值的正负
{
if(fabs(p)<=eps)
{
return 0;
}
return p<0?-1:1;
} double det(double x1,double y1,double x2,double y2)//计算叉积
{
return x1*y2-x2*y1;
} double cross(node a,node b,node c)//计算a,b,c的有向面积;
{
return det(b.x-a.x,b.y-a.y,c.x-a.x,c.y-a.y);
} bool check(node a,node b,node c,node d)//判断线段ab与线段cd是否相交;
{
return (dblcmp(cross(a,b,c))*dblcmp(cross(a,b,d)))<=0;
} double Intersect(node a,node b,node c,node d)//计算相交的点的交点
{
double area1=cross(a,b,c);
double area2=cross(a,b,d);
int c1=dblcmp(area1);
int c2=dblcmp(area2);
if(c1*c2<0)//如果小于零,规范相交
{
return (area2*c.x-area1*d.x)/(area2-area1);//计算交点的公式
}
else if(c1*c2==0)//和端点相交,判断和哪一个端点相交
{
if(c1==0)
{
return c.x;
}
else if(c2==0)
{
return d.x;
}
}
return -INF;
}
int main()
{
while(scanf("%d",&n)&&n)
{
for(int i=1; i<=n; i++)
{
scanf("%lf %lf",&up[i].x,&up[i].y);//上端点
down[i].x=up[i].x;
down[i].y=up[i].y-1;//下端点
}
bool flag=false;
double Max_x=-INF;
for(int i=1; i<=n; i++)
{
for(int j=1; j<=n; j++)
{
if(i!=j)
{
int k;
for(k=1; k<=n; k++)
{
if(!check(up[i],down[j],up[k],down[k]))//计算光线的相交的管壁
{
break;
}
}
if(k>n)//光线通过了整个管道
{
flag=true;
break;
}
if(k!=1)//注意判断,1的时候不用处理,否则会WA;
{
double tmp=Intersect(up[i],down[j],up[k],up[k-1]);
if(Max_x<tmp)
{
Max_x=tmp;
}
tmp=Intersect(up[i],down[j],down[k],down[k-1]);
if(Max_x<tmp)
{
Max_x=tmp;
}
}
}
}
if(flag)
{
break;
}
}
if(flag)
{
printf("Through all the pipe.\n");
}
else
{
printf("%.2f\n",Max_x);//输出的是最大的x坐标,不是长度
}
}
return 0;
}
Pipe(点积叉积的应用POJ1039)的更多相关文章
- 向量 dot cross product 点积叉积 几何意义
向量 dot cross product 点积叉积 几何意义 有向量 a b 点积 a * b = |a| * |b| * cosθ 几何意义: 1. a * b == 0,则 a ⊥ b 2. a ...
- uva 11178二维几何(点与直线、点积叉积)
Problem D Morley’s Theorem Input: Standard Input Output: Standard Output Morley’s theorem states tha ...
- [转] POJ计算几何
转自:http://blog.csdn.net/tyger/article/details/4480029 计算几何题的特点与做题要领:1.大部分不会很难,少部分题目思路很巧妙2.做计算几何题目,模板 ...
- 【转】POJ题目分类
初级:基本算法:枚举:1753 2965贪心:1328 2109 2586构造:3295模拟:1068 2632 1573 2993 2996 图:最短路径:1860 3259 1062 2253 1 ...
- ACM训练计划step 2 [非原创]
(Step2-500题)POJ训练计划+SGU 经过Step1-500题训练,接下来可以开始Step2-500题,包括POJ训练计划的298题和SGU前两章200题.需要1-1年半时间继续提高解决问题 ...
- ACM计算几何题目推荐
//第一期 计算几何题的特点与做题要领: 1.大部分不会很难,少部分题目思路很巧妙 2.做计算几何题目,模板很重要,模板必须高度可靠. 3.要注意代码的组织,因为计算几何的题目很容易上两百行代码,里面 ...
- POJ训练计划
POJ训练计划 Step1-500题 UVaOJ+算法竞赛入门经典+挑战编程+USACO 请见:http://acm.sdut.edu.cn/bbs/read.php?tid=5321 一.POJ训练 ...
- [知识点]计算几何I——基础知识与多边形面积
// 此博文为迁移而来,写于2015年4月9日,不代表本人现在的观点与看法.原始地址:http://blog.sina.com.cn/s/blog_6022c4720102vxaq.html 1.前言 ...
- 计算几何 : 凸包学习笔记 --- Graham 扫描法
凸包 (只针对二维平面内的凸包) 一.定义 简单的说,在一个二维平面内有n个点的集合S,现在要你选择一个点集C,C中的点构成一个凸多边形G,使得S集合的所有点要么在G内,要么在G上,并且保证这个凸多边 ...
随机推荐
- ios app下载跳到itunes
<body class="box"> <div class="text"> <a href="https://itune ...
- mysql:批量更新
(优化前)一般使用的批量更新的方法: foreach ($display_order as $id => $ordinal) { $sql = "UPDATE categori ...
- 解决多线程调用sql存储过程问题
场景: 我们程序现在改成多线程了,我现在需要把临时表中的数据给插入到TABLE_M中,但这时候可能其他的线程也在插入,我就不能用之前我们的方案了(select max(oid) from Tuning ...
- HDU 5002 Tree(动态树LCT)(2014 ACM/ICPC Asia Regional Anshan Online)
Problem Description You are given a tree with N nodes which are numbered by integers 1..N. Each node ...
- HDU 4816 Bathysphere(数学)(2013 Asia Regional Changchun)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=4816 Problem Description The Bathysphere is a spheric ...
- cometd使用-bayeux协议(读法:贝叶)
bayeux.createChannelIfAbsent("/**", new ServerChannel.Initializer() { @Override public voi ...
- 夺命雷公狗—angularjs—4—继承和修正继承
angularjs 中也有继承的方法,废话不多说,看代码: <!doctype html> <html lang="en"> <head> &l ...
- 夺命雷公狗ThinkPHP项目之----企业网站20之网站前台头尾分离
我们的网站直接让他头尾进行分离即可: 然后在代码里面找到id 为header的这段代码: 然后将整个div的内容都给弄出来,然后在view里面创建一个Public的目录,然后在创建一个header.h ...
- c++ 容器(list学习总结)
list是一个线性双向链表结构,它的数据由若干个节点构成,每一个节点都包括一个信息块(即实际存储的数据).一个前驱指针和一个后驱指针.它无需分配指定的内存大小且可以任意伸缩,这是因为它存储在非连续的内 ...
- jquery表单重置另一种方法
页面中按钮为<a>标签时,点击取消按钮,表单内容重置,需要给form表单id="form": <a class="demo_one1" onc ...