poj1981 Circle and Points 单位圆覆盖问题
转载请注明出处: http://www.cnblogs.com/fraud/ ——by fraud
Time Limit: 5000MS | Memory Limit: 30000K | |
Total Submissions: 6850 | Accepted: 2443 | |
Case Time Limit: 2000MS |
Description
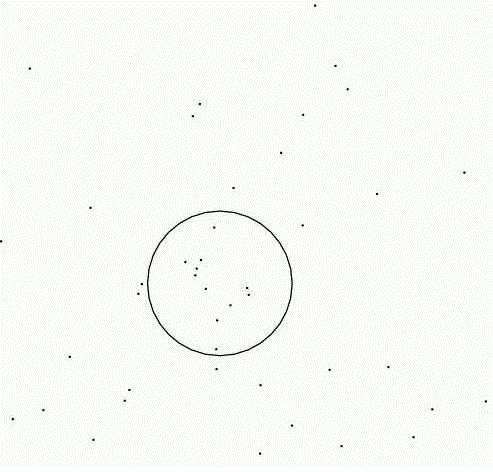
Fig 1. Circle and Points
Input
You may assume 1 <= N <= 300, 0.0 <= X <= 10.0, and 0.0 <= Y <= 10.0. No two points are closer than 0.0001. No two points in a data set are approximately at a distance of 2.0. More precisely, for any two points in a data set, the distance d between the two never satisfies 1.9999 <= d <= 2.0001. Finally, no three points in a data set are simultaneously very close to a single circle of radius one. More precisely, let P1, P2, and P3 be any three points in a data set, and d1, d2, and d3 the distances from an arbitrarily selected point in the xy-plane to each of them respectively. Then it never simultaneously holds that 0.9999 <= di <= 1.0001 (i = 1, 2, 3).
Output
Sample Input
3
6.47634 7.69628
5.16828 4.79915
6.69533 6.20378
6
7.15296 4.08328
6.50827 2.69466
5.91219 3.86661
5.29853 4.16097
6.10838 3.46039
6.34060 2.41599
8
7.90650 4.01746
4.10998 4.18354
4.67289 4.01887
6.33885 4.28388
4.98106 3.82728
5.12379 5.16473
7.84664 4.67693
4.02776 3.87990
20
6.65128 5.47490
6.42743 6.26189
6.35864 4.61611
6.59020 4.54228
4.43967 5.70059
4.38226 5.70536
5.50755 6.18163
7.41971 6.13668
6.71936 3.04496
5.61832 4.23857
5.99424 4.29328
5.60961 4.32998
6.82242 5.79683
5.44693 3.82724
6.70906 3.65736
7.89087 5.68000
6.23300 4.59530
5.92401 4.92329
6.24168 3.81389
6.22671 3.62210
0
Sample Output
2
5
5
11
题目就是给你n个点,然后用一个单位圆去覆盖,问最多同时能够覆盖到几个点
对于这个题目,首先很容易想到一种n^3的解法,那就是先枚举两个点,这两个点能够得到一个或者两个单位圆。然后在枚举所有点来统计一遍。
方法比较简单,我就不贴代码了。
对于此题,还有一种O(n^2logn)的解法。
首先以每个点为圆心 ,做一个单位圆,那么被同时覆盖到单位圆的数目最多的地方,就是我们需要求的圆心的地方。
于是,我们可以依次枚举所有圆,然后,对于每一个圆,我们在枚举其他的圆,如果有交点的话,求出两个交点,然后按照极角排序,求出被覆盖次数的最多的圆弧,因为被覆盖次数最多的圆弧也就是目标圆心的位置。
求极角的时候可以利用atan2函数,然后加上或者减去一个acos的角度即可
注意atan2使用方法
/**
* code generated by JHelper
* More info: https://github.com/AlexeyDmitriev/JHelper
* @author xyiyy @https://github.com/xyiyy
*/ #include <iostream>
#include <fstream> //#####################
//Author:fraud
//Blog: http://www.cnblogs.com/fraud/
//#####################
//#pragma comment(linker, "/STACK:102400000,102400000")
#include <iostream>
#include <sstream>
#include <ios>
#include <iomanip>
#include <functional>
#include <algorithm>
#include <vector>
#include <string>
#include <list>
#include <queue>
#include <deque>
#include <stack>
#include <set>
#include <map>
#include <cstdio>
#include <cstdlib>
#include <cmath>
#include <cstring>
#include <climits>
#include <cctype> using namespace std;
#define mp(X, Y) make_pair(X,Y)
#define rep(X, N) for(int X=0;X<N;X++) //
// Created by xyiyy on 2015/8/10.
// #ifndef JHELPER_EXAMPLE_PROJECT_P_HPP
#define JHELPER_EXAMPLE_PROJECT_P_HPP const double EPS = 1e-; double add(double a, double b) {
if (fabs(a + b) < EPS * (fabs(a) + fabs(b)))return ;
return a + b;
} class P {
public:
double x, y; P() { } P(double x, double y) : x(x), y(y) { } P operator+(const P &p) {
return P(add(x, p.x), add(y, p.y));
} P operator-(const P &p) {
return P(add(x, -p.x), add(y, -p.y));
} P operator*(const double &d) {
return P(x * d, y * d);
} P operator/(const double &d) {
return P(x / d, y / d);
} double dot(P p) {
return add(x * p.x, y * p.y);
} double abs() {
return sqrt(abs2());
} double abs2() {
return dot(*this);
} }; //直线和直线的交点
/*P isLL(P p1,P p2,P q1,P q2){
double d = (q2 - q1).det(p2 - p1);
if(sig(d)==0)return NULL;
return intersection(p1,p2,q1,q2);
}*/ //四点共圆判定
/*bool onC(P p1,P p2,P p3,P p4){
P c = CCenter(p1,p2,p3);
if(c == NULL) return false;
return add((c - p1).abs2(), -(c - p4).abs2()) == 0;
}*/ //三点共圆的圆心 #endif //JHELPER_EXAMPLE_PROJECT_P_HPP const int MAXN = ;
P ps[MAXN];
pair<double, bool> arc[MAXN]; class poj1981 {
public:
void solve(std::istream &in, std::ostream &out) {
int n;
P t;
while (in >> n && n) {
rep(i, n)in >> ps[i].x >> ps[i].y;
int ans = ;
rep(i, n) {
int num = ;
rep(j, n) {
if (i == j)continue;
double d;
if ((d = (ps[i] - ps[j]).abs()) <= ) {
double a = acos(d / );
double b = atan2((ps[j].y - ps[i].y), (ps[j].x - ps[i].x));
arc[num++] = mp(b - a, );
arc[num++] = mp(b + a, );
}
}
sort(arc, arc + num);
int res = ;
rep(j, num) {
if (arc[j].second)res++;
else res--;
ans = max(ans, res);
}
}
out << ans << endl;
}
}
}; int main() {
std::ios::sync_with_stdio(false);
std::cin.tie();
poj1981 solver;
std::istream &in(std::cin);
std::ostream &out(std::cout);
solver.solve(in, out);
return ;
}
代码君
poj1981 Circle and Points 单位圆覆盖问题的更多相关文章
- POJ-1981 Circle and Points 单位圆覆盖
题目链接:http://poj.org/problem?id=1981 容易想到直接枚举两个点,然后确定一个圆来枚举,算法复杂度O(n^3). 这题还有O(n^2*lg n)的算法.将每个点扩展为单位 ...
- bzoj1338: Pku1981 Circle and Points单位圆覆盖
题目链接:http://www.lydsy.com/JudgeOnline/problem.php?id=1338 1338: Pku1981 Circle and Points单位圆覆盖 Time ...
- poj1981 Circle and Points
地址:http://poj.org/problem?id=1981 题目: Circle and Points Time Limit: 5000MS Memory Limit: 30000K To ...
- poj1981Circle and Points(单位圆覆盖最多的点)
链接 O(n^3)的做法: 枚举任意两点为弦的圆,然后再枚举其它点是否在圆内. 用到了两个函数 atan2反正切函数,据说可以很好的避免一些特殊情况 #include <iostream> ...
- poj 1981 Circle and Points
Circle and Points Time Limit: 5000MS Memory Limit: 30000K Total Submissions: 8131 Accepted: 2899 ...
- poj 1981(单位圆覆盖最多点问题模板)
Circle and Points Time Limit: 5000MS Memory Limit: 30000K Total Submissions: 7327 Accepted: 2651 ...
- 【POJ 1981 】Circle and Points
当两个点距离小于直径时,由它们为弦确定的一个单位圆(虽然有两个圆,但是想一想知道只算一个就可以)来计算覆盖多少点. #include <cstdio> #include <cmath ...
- hdu 1077(单位圆覆盖问题)
Catching Fish Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)To ...
- Codeforces 1036E Covered Points (线段覆盖的整点数)【计算几何】
<题目链接> <转载于 >>> > 题目大意: 在二维平面上给出n条不共线的线段(线段端点是整数),问这些线段总共覆盖到了多少个整数点. 解题分析: 用GC ...
随机推荐
- 机器学习之python: kNN
################################################## # kNN : k Nearest Neighbour # Author : Monne # Da ...
- PreparedStatement可以有效地防止sql被注入
import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import jav ...
- 生产环境搭建MySQL复制的教程(转)
[导读] 网络上有很多关于MySQL复制搭建的步骤和范例,以及手册上有一章完整的篇幅,讲述MySQL复制的原理.搭建步骤.优化等,但依然存在很多刚开始学习MySQL知识或者刚进入DBA行业的朋友咨询, ...
- IDEA启动自动进入最后一个项目
每次打开IDEA的时候总会加载上级最后打开的工程,可能这个工程并不是我需要的,我就得重新去打开我需要的工程,感觉这一点非常鸡肋. 使用如下方法可以在启动的时候,选择启动哪个工程,而不是直接进入. Fi ...
- 转:MFC文件操作
讲到文件操作我们会联想到自己手动操作文件会涉及到哪些内容.很容易想到的是查看文件(文件夹)是否存在,创建,复制,删除,剪切文件(文件夹).另外就是设置文件的属性. 那MFC中一些操作文件的类也差不多是 ...
- 黑马程序员_JavaIO流(一)
IO(Input Output)流 概述: IO流(数据流)用来处理设备之间的数据传输. Java对数据的操作是通过流的方式. Java用于操作流的对象都在IO包中. 流按操作数据分为两种:字节流与字 ...
- Dijkstra优先队列优化
Dijkstra算法的核心思想就是两步排序,一个是对于一个点而言,他的最小边要经过所有其他点最小边的测试才能确认,也就是说要在这其中找一个最大的边出来:第二个是对于每次循环而言的,每次的更新d数组都是 ...
- HDFS文件读取详解
客户端与HDFS文件读取 创建HDFS文件系统实例 FileSystem fs = FileSystem.get(new URI("hdfs://ns1"), new Config ...
- N - Picture - poj 1177(扫描线求周长)
题意:求周长的,把矩形先进行融合后的周长,包括内周长 分析:刚看的时候感觉会跟棘手,让人无从下手,不过学过扫描线之后相信就很简单了吧(扫描线的模板- -),还是不说了,下面是一精确图,可以拿来调试数据 ...
- 《图解HTTP》 第11章 web的攻击技术
11.1 针对Web的攻击技术 简单的HTTP协议本身并不存在安全性问题,所以协议本身几乎不会成为攻击的对象. 11.1.1 HTTP不具备必要的安全功能 11.1.2 在客户端即可篡改请求 在HTT ...