第11課-Channel Study For Create Custom Restful Service
这节课我们一起学习利用Mirth Connect的HTTP Listener源通道与JavaScript Writer目的通道搭建自定义Restful风格webapi服务。
1.新建名为‘Custom Restful api’的信道,指定源通道与目的通道的输入输出消息格式
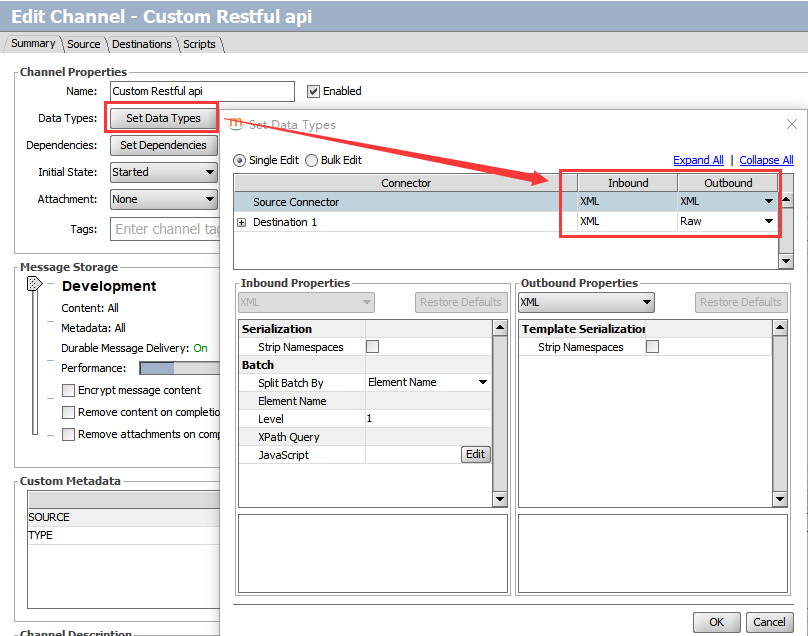
2.设置HTTP Listener类型源通道参数
- 把 "Response" 响应指定为 destination 1
- 输入‘Base context path’ 为
/myrestservice
- 设置 "Message Content" 为 XML Body
- 设置默认"Response Content Type" 为
text/plain; charset=UTF-8我们将在目的通道中通过channel map重写它的值为application/xml或application/json
- 设置 "Response Status Code" 响应码为
${responseStatusCode}我们将在目的通道中通过channel map重写它的值为200(成功)或500(失败)
- 在 "Response Header" 中添加一个变量 "Content-Type" ,指定其值为
${responseContentType}我们将在目的通道中通过channel map重写它的值为application/xml或application/json
3.设置JavaScript Writer目的通道参数并编写JS实现脚本
// Mirth strings don't support startsWith() in Mirth 3
// If necessary, add a method to the String prototype.
if (!String.prototype.startsWith) {
String.prototype.startsWith = function(searchString, position){
position = position || 0;
return this.substr(position, searchString.length) === searchString;
};
} /*
Incoming message looks like this: <HttpRequest>
<RemoteAddress>71.127.40.115</RemoteAddress>
<RequestUrl>http://www.example.com:8080/myrestservice</RequestUrl>
<Method>GET</Method>
<RequestPath>foo=bar</RequestPath>
<RequestContextPath>/myrestservice/param1/param2</RequestContextPath>
<Parameters>
<foo>bar</foo>
</Parameters>
<Header>
<Host>www.example.com:8080</Host>
<Accept-Encoding>identity</Accept-Encoding>
<User-Agent>Wget/1.18 (darwin15.5.0)</User-Agent>
<Connection>keep-alive</Connection>
<Accept>application/xml</Accept>
</Header>
<Content/>
</HttpRequest> <HttpRequest>
<RemoteAddress>71.127.40.115</RemoteAddress>
<RequestUrl>http://www.example.com:8080/myrestservice</RequestUrl>
<Method>GET</Method>
<RequestPath>foo=bar</RequestPath>
<RequestContextPath>/myrestservice/param1/param2</RequestContextPath>
<Parameters>
<foo>bar</foo>
</Parameters>
<Header>
<Host>www.example.com:8080</Host>
<Accept-Encoding>identity</Accept-Encoding>
<User-Agent>Wget/1.18 (darwin15.5.0)</User-Agent>
<Connection>keep-alive</Connection>
<Accept>application/json</Accept>
</Header>
<Content/>
</HttpRequest>
*/ // Just in case we fail, set a sane responseContentType
channelMap.put('responseContentType', 'text/plain'); var msg = XML(connectorMessage.getRawData());
logger.info(msg);
// Get the REST data from the "context path" which is actually
// the "path info" of the request, so it will start with '/myrestservice'.
var rest = msg['RequestContextPath'];
logger.info(rest);
var myServicePrefix = '/myrestservice';
var minimumURLParameterCount = 4; // This is the minimum you require to do your work
var maximumExpectedURLParameterCount = 5; // however many you expect to get
var params = rest.substring(myServicePrefix).split('/', maximumExpectedURLParameterCount);
if(params.length < minimumURLParameterCount)
return Packages.com.mirth.connect.server.userutil.ResponseFactory.getErrorResponse('Too few parameters in request');
var mrn = params[1]; // params[0] will be an empty string
logger.info(mrn);
// Now, determine the client's preference for what data type to return (XML vs. JSON).
// We will default to XML.
var clientWantsJSON = false;
var responseContentType = 'text/xml'; // If we see any kind of JSON before any kind of XML, we'll use
// JSON. Otherwise, we'll use XML.
//
// Technically, this is incorrect resolution of the "Accept" header,
// but it's good enough for an example.
var mimeTypes = msg['Header']['Accept'].split(/\s*,\s*/);
for(var i=0; i<mimeTypes.length; ++i) {
var mimeType = mimeTypes[i].toString();
if(mimeType.startsWith('application/json')) {
clientWantsJSON = true;
responseContentType = 'application/json';
break;
} else if(mimeType.startsWith('application/xml')) {
clientWantsJSON = false;
responseContentType = 'application/xml';
break;
} else if(mimeType.startsWith('text/xml')) {
clientWantsJSON = false;
responseContentType = 'text/xml';
break;
}
} var xml;
var json; if(clientWantsJSON)
json = { status : '' };
else
xml = new XML('<response></response>'); try {
/*
Here is where you do whatever your service needs to actually do.
*/ if(clientWantsJSON) {
json.data = { foo: 1,
bar: 'a string',
baz: [ 'list', 'of', 'strings']
};
} else {
xml['@foo'] = 1;
xml['bar'] = 'a string';
xml['baz'][0] = 'list';
xml['baz'][1] = 'of';
xml['baz'][3] = 'strings';
} // Set the response code and content-type appropriately.
// http://www.mirthproject.org/community/forums/showthread.php?t=12678 channelMap.put('responseStatusCode', 200); if(clientWantsJSON) {
json.status = 'success';
var content = JSON.stringify(json);
channelMap.put('responseContent', content);
channelMap.put('responseContentType', responseContentType);
return content;
} else {
channelMap.put('responseContentType', responseContentType);
var content = xml.toString();
channelMap.put('responseContent', content);
return content;
}
}
catch (err)
{
channelMap.put('responseStatusCode', '500');
if(clientWantsJSON) {
json.status = 'error';
if(err.javaException) {
// If you want to unpack a Java exception, this is how you do it:
json.errorType = String(err.javaException.getClass().getName());
json.errorMessage = String(err.javaException.getMessage());
} channelMap.put('responseContentType', responseContentType); // Return an error with our "error" JSON
return Packages.com.mirth.connect.server.userutil.ResponseFactory.getErrorResponse(JSON.stringify(json));
} else {
if(err.javaException) {
xml['response']['error']['@type'] = String(err.javaException.getClass().getName());
xml['response']['error']['@message'] = String(err.javaException.getMessage());
} channelMap.put('responseContentType', responseContentType); // Return an error with our "error" XML
return Packages.com.mirth.connect.server.userutil.ResponseFactory.getErrorResponse(xml.toString());
}
}
我们通过目的通道以上JS脚本,学习到以下特别重要的知识:
- 获取输入请求的原始消息并自动格式化为XML格式:
var xml = new XML(connectorMessage.getRawData())
- 设置响应类型,如:
channelMap.put('responseContentType', 'application/json')
- 设置响应码,如:
channelMap.put('responseStatusCode', '200')
- 设置响应内容并通过JS脚本返回XML实体或者Json实体的字符串格式值
- 异常处理通过JS脚本调用Mirth的API函数Packages.com.mirth.connect.server.userutil.ResponseFactory.getErrorResponse(string)返回字符串格式错误消息
4.部署信道并测试
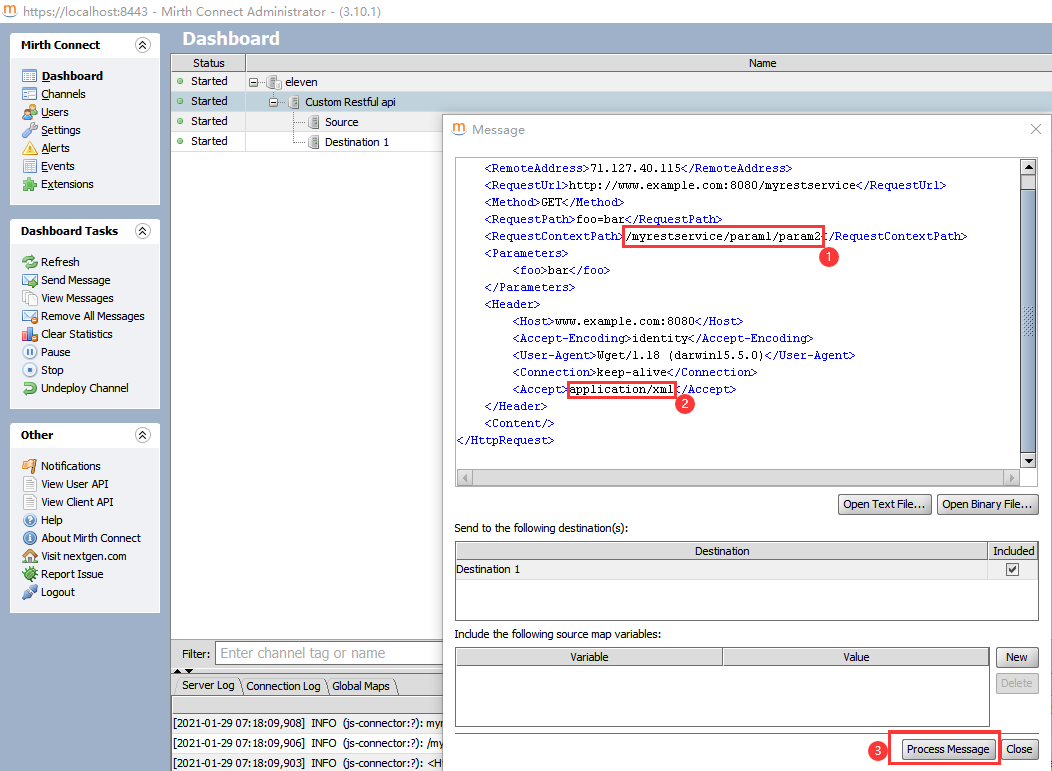
发送消息要区分application/json和application/xml,可以看到响应值格式会相应变化
<HttpRequest>
<RemoteAddress>71.127.40.115</RemoteAddress>
<RequestUrl>http://www.example.com:8080/myrestservice</RequestUrl>
<Method>GET</Method>
<RequestPath>foo=bar</RequestPath>
<RequestContextPath>/myrestservice/param1/param2</RequestContextPath>
<Parameters>
<foo>bar</foo>
</Parameters>
<Header>
<Host>www.example.com:8080</Host>
<Accept-Encoding>identity</Accept-Encoding>
<User-Agent>Wget/1.18 (darwin15.5.0)</User-Agent>
<Connection>keep-alive</Connection>
<Accept>application/json</Accept>
</Header>
<Content/>
</HttpRequest>
<HttpRequest>
<RemoteAddress>71.127.40.115</RemoteAddress>
<RequestUrl>http://www.example.com:8080/myrestservice</RequestUrl>
<Method>GET</Method>
<RequestPath>foo=bar</RequestPath>
<RequestContextPath>/myrestservice/param1/param2</RequestContextPath>
<Parameters>
<foo>bar</foo>
</Parameters>
<Header>
<Host>www.example.com:8080</Host>
<Accept-Encoding>identity</Accept-Encoding>
<User-Agent>Wget/1.18 (darwin15.5.0)</User-Agent>
<Connection>keep-alive</Connection>
<Accept>application/xml</Accept>
</Header>
<Content/>
</HttpRequest>
第11課-Channel Study For Create Custom Restful Service的更多相关文章
- How to Create Custom Filters in AngularJs
http://www.codeproject.com/Tips/829025/How-to-Create-Custom-Filters-in-AngularJs Introduction Filter ...
- create custom launcher icon 细节介绍
create custom launcher icon 是创建你的Android app的图标 点击下一步的时候,出现的界面就是创建你的Android的图标 Foreground: ” Foregro ...
- [转]How to Create Custom Filters in AngularJs
本文转自:http://www.codeproject.com/Tips/829025/How-to-Create-Custom-Filters-in-AngularJs Introduction F ...
- How to: Create Custom Configuration Sections Using ConfigurationSection
https://msdn.microsoft.com/en-us/library/2tw134k3.aspx You can extend ASP.NET configuration settings ...
- java中如何创建自定义异常Create Custom Exception
9.创建自定义异常 Create Custom Exception 马克-to-win:我们可以创建自己的异常:checked或unchecked异常都可以, 规则如前面我们所介绍,反正如果是chec ...
- Custom Data Service Providers
Custom Data Service Providers Introduction Data Services sits above a Data Service Provider, which i ...
- Unable to create Azure Mobile Service: Error 500
I had to go into my existing azure sql database server and under the configuration tab select " ...
- Cannot create container for service peer1.org2.example.com: Conflict. 解决方案
I have a docker-compose.yaml file defining 5 services: orderer.example.com peer0.org1.example.com pe ...
- docker启动报错解决及分析(Cannot create container for service *******: cannot mount volume over existing file, file exists /var/lib/docker/overlay2/)
现象: Cannot create container for service *******: cannot mount volume over existing file, file exists ...
- [转]Create Custom Exception Filter in ASP.NET Core
本文转自:http://www.binaryintellect.net/articles/5df6e275-1148-45a1-a8b3-0ba2c7c9cea1.aspx In my previou ...
随机推荐
- 代码片段管理软件 - 发现 utools 这个工具还行 windows软件
代码片段管理软件 - 发现 utools 这个工具还行 windows软件 介绍 这个软件不是专业的代码片段工具 好在还能凑合用 最完美的还是苹果那个软件,但是用的win没办法了 这个可以粘贴到vsc ...
- ESP8266 下安装esptool.py并使用esptool刷机神助手
一 前记 在使用ESP8266模块时,通常会用到一些刷机软件.官方提供了nodemcu_flasher.ESPFlashDownloadTool.ESP8266Flasher等下载工具,但是缺少更底层 ...
- auto推导类型注意
auto推导类型忽略顶层const,不忽略底层const. 顶层const:指针或引用本身是const不可变,也就是指针指向的内存地址不可变,但指向的内存内容可变. 底层const:指针指向的内存地址 ...
- TX2 核心板 GPIO、IO扩展器、拨码开关、LED灯 使用总结
PS:要转载请注明出处,本人版权所有. PS: 这个只是基于<我自己>的理解, 如果和你的原则及想法相冲突,请谅解,勿喷. 前置说明 本文作为本人csdn blog的主站的备份.(Bl ...
- 使用nodejs从控制台读入内容
在写算法题的时候,基本上都需要输入输出语句,在大多数练题网站上当想用js书写算法题时,发现不知道怎么输入,其实Node是提供了一个readline模块来实现此功能的 tip 笔者用过的练题网站只有le ...
- 记录--P0事故预警
这里给大家分享我在网上总结出来的一些知识,希望对大家有所帮助 背景 某一天,前端小余同学和后端别问我小哥在做登录业务接口对接,出于业务的特殊性和安全性的考虑,她和后端小哥约定"user&qu ...
- 2022北航软件研究生入学考试991考试大纲-数据结构与C
991"数据结构与C语言程序设计"考试大纲 "数据结构与C语言程序设计"考试内容包括"数据结构"与"C语言程序设计"两门 ...
- 使用Razor模板动态生成代码
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; usin ...
- Oracle PL/SQL 中的 CHAR 和 VARCHAR2 比较
https://docs.oracle.com/cd/B14117_01/appdev.101/b10807/b_char.htm SQL 标准要求被比较的两个字符值具有相等的长度.如果比较中的两个值 ...
- 手把手带你用香橙派AIpro开发AI推理应用
本文分享自华为云社区<如何基于香橙派AIpro开发AI推理应用>,作者:昇腾CANN. 01 简介 香橙派AIpro开发板采用昇腾AI技术路线,接口丰富且具有强大的可扩展性,提供8/20T ...