HDU 6086 Rikka with String AC自动机 + DP
Rikka with String
Yuta has n 01 strings si, and he wants to know the number of 01 antisymmetric strings of length 2L which contain all given strings si as continuous substrings.
A 01 string s is antisymmetric if and only if s[i]≠s[|s|−i+1] for all i∈[1,|s|].
It is too difficult for Rikka. Can you help her?
In the second sample, the strings which satisfy all the restrictions are 000111,001011,011001,100110.
For each testcase, the first line contains two numbers n,L(1≤n≤6,1≤L≤100).
Then n lines follow, each line contains a 01 string si(1≤|si|≤20).
2 2
011
001
2 3
011
001
4
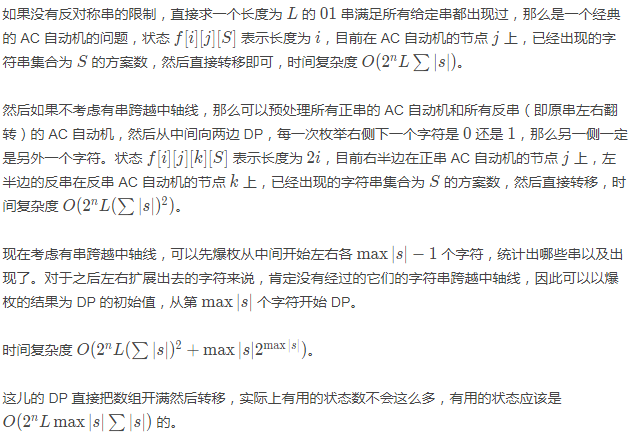
#include<bits/stdc++.h>
using namespace std;
#pragma comment(linker, "/STACK:102400000,102400000")
#define ls i<<1
#define rs ls | 1
#define mid ((ll+rr)>>1)
#define pii pair<int,int>
#define MP make_pair
typedef long long LL;
const long long INF = 1e18+1LL;
const double pi = acos(-1.0);
const int N = 1e4+, M = 1e3+,inf = 2e9; const LL mod = 998244353LL; int dp[][][][],sum[][N];
int nex[][N][],cnt0,cnt1,head1,tail1,head0,tail0,q[][N],fail[][N]; void insert(char *s,int p) {
int now = ,len = strlen(s);
for(int i = ; i < len; ++i) {
int index = s[i] - '';
if(!nex[][now][index])
nex[][now][index] = ++cnt0;
sum[][nex[][now][index]] |= sum[][now];
now = nex[][now][index];
//cout<<now<<" "<<index<<endl;
}
sum[][now] |= (<<p); now = ;
for(int i = len-; i >= ; --i) {
int index = s[i] - '';
if(!nex[][now][index])
nex[][now][index] = ++cnt1;
sum[][nex[][now][index]] |= sum[][now];
now = nex[][now][index];
//cout<<now<<" "<<index<<endl;
}
sum[][now] |= (<<p);
} void build_fail() {
head0 = , tail0 = ;head1 = , tail1 = ;
for(int i = ; i < ; ++i)
nex[][][i] = ,nex[][][i] = ; fail[][] = ,fail[][] = ;
q[][tail0++] = ;q[][tail1++] = ;
while(head0 != tail0) {
int now = q[][head0++];
sum[][now] |= sum[][fail[][now]];
for(int i = ; i < ; ++i) {
int p = fail[][now];
if(!nex[][now][i]) {
nex[][now][i] = nex[][p][i];continue;
}
fail[][nex[][now][i]] = nex[][p][i];
q[][tail0++] = nex[][now][i];
}
}
while(head1 != tail1) {
int now = q[][head1++];
sum[][now] |= sum[][fail[][now]];
for(int i = ; i < ; ++i) {
int p = fail[][now];
if(!nex[][now][i]) {
nex[][now][i] = nex[][p][i];continue;
}
fail[][nex[][now][i]] = nex[][p][i];
q[][tail1++] = nex[][now][i];
}
}
}
int len[N],mx,n,L;
char a[N];
int dfs() {
int now = ;
int ret = ;
for(int i = ; i <= *mx; ++i) {
now = nex[][now][len[i]];
ret |= sum[][now];
}
return ret;
}
int ma(int p) {
int now = ;
if(p)
for(int i = mx; i >= ; --i)
now = nex[][now][len[i]];
else
for(int i = mx+; i <= *mx; ++i)
now = nex[][now][len[i]];
return now;
}
void init() {
memset(dp,,sizeof(dp));
memset(nex,,sizeof(nex));
cnt0 = ;mx = -;cnt1 = ;
memset(fail,,sizeof(fail));
memset(sum,,sizeof(sum));
}
int main() {
int T;
scanf("%d",&T);
while(T--) {
scanf("%d%d",&n,&L);
init();
for(int i = ; i <= n; ++i) {
scanf("%s",a);
insert(a,i-);
mx = max(mx,(int)strlen(a));
}
int ff = ;
mx-=;
build_fail();
for(int i = ; i < (<<mx); ++i) {
for(int j = ; j <= mx; ++j) len[j] = ((i>>(j-))&);
for(int j = mx+; j <= *mx; ++j) len[j] = ^(len[*mx - j + ]);
int now = dfs();
int z = ma(),f = ma();
dp[ff][z][f][now] += ;
dp[ff][z][f][now] %= mod;
// cout<<i<<" "<<now<<" "<<z<<" "<<f<<endl;
} for(int i = mx; i < L; i++) {
memset(dp[ff^],,sizeof(dp[ff^]));
for(int j = ; j < tail1; ++j) {
for(int k = ; k < tail0; ++k) {
for(int h = ; h < (<<n); ++h) { if(!dp[ff][q[][j]][q[][k]][h]) continue; int p = nex[][q[][j]][],np = nex[][q[][k]][];
int tmp = (h|sum[][p]);
tmp |= sum[][np]; dp[ff^][p][np][tmp] += dp[ff][q[][j]][q[][k]][h];
dp[ff^][p][np][tmp] %= mod; p = nex[][q[][j]][],np = nex[][q[][k]][];
tmp = (h|sum[][p]);
tmp |= sum[][np]; dp[ff^][p][np][tmp] += dp[ff][q[][j]][q[][k]][h];
dp[ff^][p][np][tmp] %= mod; }
}
}
ff^=;
}
LL ans = ;
for(int i = ; i < tail1; ++i)
for(int j = ; j < tail0; ++j)
ans = ( ans + dp[ff][q[][i]][q[][j]][(<<n)-]) % mod;
printf("%lld\n",ans);
}
return ;
}
HDU 6086 Rikka with String AC自动机 + DP的更多相关文章
- hdu 6086 -- Rikka with String(AC自动机 + 状压DP)
题目链接 Problem Description As we know, Rikka is poor at math. Yuta is worrying about this situation, s ...
- HDU 3341 Lost's revenge AC自动机+dp
Lost's revenge Time Limit: 15000/5000 MS (Java/Others) Memory Limit: 65535/65535 K (Java/Others)T ...
- HDU 2457 DNA repair(AC自动机+DP)题解
题意:给你几个模式串,问你主串最少改几个字符能够使主串不包含模式串 思路:从昨天中午开始研究,研究到现在终于看懂了.既然是多模匹配,我们是要用到AC自动机的.我们把主串放到AC自动机上跑,并保证不出现 ...
- HDU 6086 Rikka with String ——(AC自动机 + DP)
这是一个AC自动机+dp的问题,在中间的串的处理可以枚举中断点来插入自动机内来实现,具体参见代码. 在这题上不止为何一直MLE,一直找不到结果(lyf相同写法的代码消耗内存较少),还好考虑到这题节点应 ...
- HDU 2425 DNA repair (AC自动机+DP)
DNA repair Time Limit: 5000/2000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total ...
- HDU 6086 Rikka with String
Rikka with String http://acm.hdu.edu.cn/showproblem.php?pid=6086 题意: 求一个长度为2L的,包含所给定的n的串,并且满足非对称. 分析 ...
- HDU 4758 Walk Through Squares(AC自动机+DP)
题目链接 难得出一个AC自动机,我还没做到这个题呢...这题思路不难想,小小的状压出一维来,不过,D和R,让我wa死了,AC自动机,还得刷啊... #include<iostream> # ...
- HDU 2825 Wireless Password【AC自动机+DP】
给m个单词,由这m个单词组成的一个新单词(两个单词可以重叠包含)长度为n,且新单词中包含的基本单词数目不少于k个.问这样的新单词共有多少个? m很小,用二进制表示新单词中包含基本单词的情况. 用m个单 ...
- HDU 4057 Rescue the Rabbit(AC自动机+DP)
题目链接 一个数组开小了一点点,一直提示wa,郁闷,这题比上个题简单一点. #include <iostream> #include <cstring> #include &l ...
随机推荐
- 北京师范大学第十五届ACM决赛-重现赛
Another Server 时间限制:1秒 空间限制:262144K 题目描述 何老师某天在机房里搞事情的时候,发现机房里有n台服务器,从1到n标号,同时有2n-2条网线,从1到2n-2标号,其中第 ...
- 史上最详细的linux关于connect: network is unreachable 问题的解决方案
1.虚拟机常用连接网络方式有两种:桥接和NAT. 使用桥接模式:则保证虚拟机的网段与物理机的网段保持一致.如下: 虚拟机网卡配置: 物理机使用WiFi接入网络(我用的是WiFi,你们可能用的是有线道理 ...
- 查看Linux版本的方法
1)命令: lsb_release -a [root@localhost tmp]# lsb_release -a LSB Version: :core-4.0-amd64:core-4.0-noar ...
- POJ 3104 Drying [二分 有坑点 好题]
传送门 表示又是神题一道 Drying Time Limit: 2000MS Memory Limit: 65536K Total Submissions: 9327 Accepted: 23 ...
- tmux基本操作
安装和移除: // 安装 sudo apt-get install tmux // 移除 sudo apt-get remove tmux 常用命令: tmux [new -s 会话名 -n 窗口名] ...
- GridView动态删除Item
activity_main.xml <?xml version="1.0" encoding="utf-8"?> <LinearLayout ...
- 解决 Mac OS X Retina 屏幕显示环境下 jEdit 字体模糊的方法
Mac OS X Retina 屏幕显示环境下,字体非常清晰.但是 jEdit 仍然很模糊,虽然 jEdit 用的是 Java,但这并不是理由.因为诸如 NetBeans 以及 IntelliJ ID ...
- SGU 分类
http://acm.sgu.ru/problemset.php?contest=0&volume=1 101 Domino 欧拉路 102 Coprime 枚举/数学方法 103 Traff ...
- 《从零开始搭建游戏服务器》Eclipse和Tomcat安装配置
我选择用来进行服务器开发的语言是Java,开发流程更接近于JavaWeb,所以需要先为开发配置一个开发环境,需要配置的主要是Eclipse和Tomcat(Web工程的容器或管理工具). 一.资源下载: ...
- C++ 使用成员初始化列表的一个小坑
注意在成员列表中初始化的顺序并不是列表顺序 而是: 在类中声明的顺序! EventLoop::EventLoop() :looping(false), quit(false),_tid(curThre ...