POJ 1655.Balancing Act 树形dp 树的重心
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 14550 | Accepted: 6173 |
Description
For example, consider the tree:
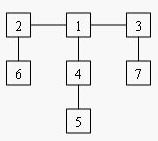
Deleting node 4 yields two trees whose member nodes are {5} and {1,2,3,6,7}. The larger of these two trees has five nodes, thus the balance of node 4 is five. Deleting node 1 yields a forest of three trees of equal size: {2,6}, {3,7}, and {4,5}. Each of these trees has two nodes, so the balance of node 1 is two.
For each input tree, calculate the node that has the minimum balance. If multiple nodes have equal balance, output the one with the lowest number.
Input
Output
Sample Input
1
7
2 6
1 2
1 4
4 5
3 7
3 1
Sample Output
1 2
Source
#include<iostream>
#include<cstdio>
#include<cmath>
#include<cstring>
#include<algorithm>
#include<set>
#include<bitset>
#include<map>
#include<queue>
#include<stack>
#include<vector>
using namespace std;
typedef long long ll;
typedef pair<int,int> P;
#define bug(x) cout<<"bug"<<x<<endl;
#define PI acos(-1.0)
#define eps 1e-8
const int N=1e5+,M=1e5+;
const int inf=0x3f3f3f3f;
const ll INF=1e18+,mod=1e9+;
int n;
vector<int>G[N];
int si[N],maxx[N];
int ans;
int dfs(int u,int fa)
{
for(int i=; i<G[u].size(); i++)
{
int v=G[u][i];
if(v==fa) continue;
si[u]+=dfs(v,u);
maxx[u]=max(maxx[u],si[v]);
}
si[u]++;
maxx[u]=max(maxx[u],n-si[u]);
if(maxx[u]<maxx[ans]) ans=u;
else if(maxx[u]==maxx[ans]&&u<ans) ans=u;
return si[u];
}
int main()
{
int T;
scanf("%d",&T);
while(T--)
{
scanf("%d",&n);
for(int i=; i<n; i++)
{
int u,v;
scanf("%d%d",&u,&v);
G[u].push_back(v);
G[v].push_back(u);
}
memset(si,,sizeof(si));
memset(maxx,,sizeof(maxx));
ans=,maxx[]=inf;
dfs(,);
printf("%d %d\n",ans,maxx[ans]);
for(int i=;i<=n+;i++) G[i].clear();
}
return ;
}
树形dp
POJ 1655.Balancing Act 树形dp 树的重心的更多相关文章
- poj 1655 Balancing Act(找树的重心)
Balancing Act POJ - 1655 题意:给定一棵树,求树的重心的编号以及重心删除后得到的最大子树的节点个数size,如果size相同就选取编号最小的. /* 找树的重心可以用树形dp或 ...
- POJ 1655 Balancing Act (求树的重心)
求树的重心,直接当模板吧.先看POJ题目就知道重心什么意思了... 重心:删除该节点后最大连通块的节点数目最小 #include<cstdio> #include<cstring&g ...
- POJ 1655 Balancing Act(求树的重心)
Description Consider a tree T with N (1 <= N <= 20,000) nodes numbered 1...N. Deleting any nod ...
- POJ 2378.Tree Cutting 树形dp 树的重心
Tree Cutting Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 4834 Accepted: 2958 Desc ...
- poj 1655 Balancing Act 求树的重心【树形dp】
poj 1655 Balancing Act 题意:求树的重心且编号数最小 一棵树的重心是指一个结点u,去掉它后剩下的子树结点数最少. (图片来源: PatrickZhou 感谢博主) 看上面的图就好 ...
- POJ.1655 Balancing Act POJ.3107 Godfather(树的重心)
关于树的重心:百度百科 有关博客:http://blog.csdn.net/acdreamers/article/details/16905653 1.Balancing Act To POJ.165 ...
- POJ 1655 Balancing Act(求树的重心--树形DP)
题意:求树的重心的编号以及重心删除后得到的最大子树的节点个数size,假设size同样就选取编号最小的. 思路:随便选一个点把无根图转化成有根图.dfs一遍就可以dp出答案 //1348K 125MS ...
- POJ 1655 Balancing Act (树状dp入门)
Description Consider a tree T with N (1 <= N <= 20,000) nodes numbered 1...N. Deleting any nod ...
- POJ 1655 Balancing Act【树的重心】
Balancing Act Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 14251 Accepted: 6027 De ...
随机推荐
- Grafana介绍
Grafana是一个开源的度量分析与可视化套件.纯 Javascript 开发的前端工具,通过访问库(如InfluxDB),展示自定义报表.显示图表等.大多使用在时序数据的监控方面,如同Kibana类 ...
- HOOK - 低级鼠标Hook
参考博客 一.SetWindowsHookEx HHOOK WINAPI SetWindowsHookEx( __in int idHook, \\钩子类型 __in HOOKPROC lpfn, \ ...
- Altium Designer9.4局域网内冲突的问题
Altium Designer破解 1.安装Altium Designer原程序.2.运行AD9KeyGen,点击“打开模板”,加载ad9.ini,如想修改注册名,只需修改:TransactorNam ...
- SpringBoot入门篇--关于properties和yml两种配置文件的一些事情
我们在使用SpringBoot这个框架的时候都一定使用或者说是见到过application.properties或者是application.yml,经不住有人就会问这俩文件到底是什么情况,其实说白了 ...
- mysql相关碎碎念
取得当天: SELECT curdate(); mysql> SELECT curdate();+------------+| curdate() |+------------+| 2013- ...
- RobotFramework - AppiumLibrary 之元素定位
一.介绍 AppiumLibrary 是 Robot Framework 的App测试库. 它使用Appium 与Android 和 iOS应用程序进行通信,类似于Selenium WebDriver ...
- servlet cdi注入
@WebServlet("/cdiservlet")//url映射,即@WebServlet告诉容器,如果请求的URL是"/cdiservlet",则由NewS ...
- SpringCloud系列三:SpringSecurity 安全访问(配置安全验证、服务消费端处理、无状态 Session 配置、定义公共安全配置程序类)
1.概念:SpringSecurity 安全访问 2.具体内容 所有的 Rest 服务最终都是暴露在公网上的,也就是说如果你的 Rest 服务属于一些你自己公司的私人业务,这样的结果会直接 导致你信息 ...
- canvas 2.0 图片绘制
绘制图片drawImage 2013.02.21 by 十年灯·一条评论 本文属于<html5 Canvas画图系列教程> 这里的绘制图片是指把一张现成的图片,绘制到Canvas上面. 有 ...
- 编织织物的knit course direction and knit wale direction
来自:http://www.definetextile.com/2013/04/course-wale.html