iOS 使用两个tableview的瀑布流
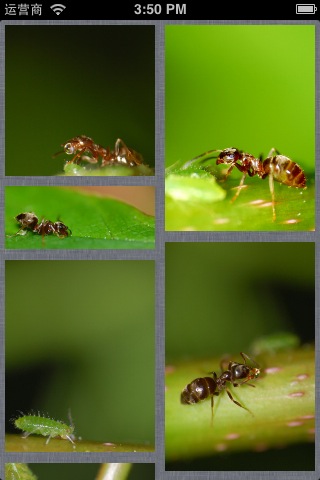
代码
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
|
// // DocViewController.m // getrightbutton // // #import "DocViewController.h" #define heightofimage(image) image.size.height*150.0/image.size.width @interface DocViewController () { NSMutableArray *arrdata; NSMutableArray *arrdata1; NSMutableArray *arrdata2; NSMutableArray *arrdata1_1; NSMutableArray *arrdata2_1; float he1,he2; } @property (weak, nonatomic) IBOutlet UITableView *tableview01; @property (weak, nonatomic) IBOutlet UITableView *tableview02; @end @implementation DocViewController @synthesize tableview01,tableview02; - (void)viewDidLoad { [super viewDidLoad]; // Do any additional setup after loading the view, typically from a nib. [[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(Backhome) name:@"Back" object:nil]; he1 = 0.0; he2 = 0.0; arrdata1 = [[NSMutableArray alloc] initWithCapacity:1]; arrdata2 = [[NSMutableArray alloc] initWithCapacity:1]; arrdata1_1 = [[NSMutableArray alloc] initWithCapacity:1]; arrdata2_1 = [[NSMutableArray alloc] initWithCapacity:1]; for (int i = 1; i < 11; i++) { UIImage *image = [UIImage imageNamed:[NSString stringWithFormat:@"%d.jpeg",i]]; float hecu = image.size.height*150.0/image.size.width; if (he2 >= he1) { he1 = he1 + hecu; NSArray *arr = [[NSArray alloc] initWithObjects:[NSString stringWithFormat:@"%d",i],[NSString stringWithFormat:@"%f",hecu], nil]; // [arrdata1_1 addObject:[NSString stringWithFormat:@"%d",i]]; // [arrdata1_1 addObject:[NSString stringWithFormat:@"%f",hecu]]; [arrdata1 addObject:arr]; }else{ he2 = he2 + hecu; NSArray *arr = [[NSArray alloc] initWithObjects:[NSString stringWithFormat:@"%d",i],[NSString stringWithFormat:@"%f",hecu], nil]; [arrdata2 addObject:arr]; } NSLog(@"%f(h1 = %f,,,,h2 = %f)",hecu,he1,he2); } tableview01.showsVerticalScrollIndicator = NO; tableview02.showsVerticalScrollIndicator = NO; NSLog(@"%@+++++++%@",arrdata1,arrdata2); } - (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section{ // return 100; if (tableView == tableview01) { return [arrdata1 count]; }else{ return [arrdata2 count]; } return 0; } - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath{ NSInteger row = indexPath.row; if (tableView == tableview01) { // tableview02 // [tableview02 setContentOffset:tableview01.contentOffset]; static NSString *id1 = @"sd1"; UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:id1]; if (cell == nil) { NSArray *nib = [[NSBundle mainBundle] loadNibNamed:@"CellView" owner:self options:nil]; if (nib > 0) { cell = _ccell; } } UIImage *image = [UIImage imageNamed:[NSString stringWithFormat:@"%d.jpeg",[[[arrdata1 objectAtIndex:row] objectAtIndex:0] integerValue]]]; UIImageView *imageview = (UIImageView *)[cell viewWithTag:101]; [imageview setImage:image]; CGRect rect = imageview.frame; rect.size.height = [[[arrdata1 objectAtIndex:row] objectAtIndex:1] floatValue]; imageview.frame = rect; return cell; }else{ // [tableview01 setContentOffset:tableview02.contentOffset]; static NSString *id = @"sd"; UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:id]; if (cell == nil) { NSArray *nib = [[NSBundle mainBundle] loadNibNamed:@"CellView" owner:self options:nil]; if (nib > 0) { cell = _ccell; } } UIImage *image = [UIImage imageNamed:[NSString stringWithFormat:@"%d.jpeg",[[[arrdata2 objectAtIndex:row] objectAtIndex:0] integerValue]]]; UIImageView *imageview = (UIImageView *)[cell viewWithTag:101]; [imageview setImage:image]; CGRect rect = imageview.frame; rect.size.height = [[[arrdata2 objectAtIndex:row] objectAtIndex:1] floatValue]; imageview.frame = rect; return cell; } return nil; } - (float)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath{ NSInteger row = indexPath.row; if (tableView == tableview01) { return [[[arrdata1 objectAtIndex:row] objectAtIndex:1] floatValue] +10; }else{ return [[[arrdata2 objectAtIndex:row] objectAtIndex:1] floatValue] +10; } return 0.0; } - (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath{ DocwebViewController *controller = [[DocwebViewController alloc] init]; [self presentModalViewController:controller animated:YES]; } - (void)Backhome{ [self dismissModalViewControllerAnimated:YES]; } - (void)scrollViewDidScroll:(UIScrollView *)scrollView{ if (scrollView == tableview01) { [tableview02 setContentOffset:tableview01.contentOffset]; }else{ [tableview01 setContentOffset:tableview02.contentOffset]; } } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // Dispose of any resources that can be recreated. } @end |
iOS 使用两个tableview的瀑布流的更多相关文章
- iOS 两个tableview的 瀑布流
iOS 两个tableview的 瀑布流1. [代码]Objective-C //// DocViewController.m// getrightbutton//// Created ...
- ios 让两个tableView同时处于选中状态
- (void)tableView:(UITableView *)tableView didHighlightRowAtIndexPath:(NSIndexPath *)indexPath { [_l ...
- iOS瀑布流实现(Swift)
这段时间突然想到一个很久之前用到的知识-瀑布流,本来想用一个简单的方法,发现自己走入了歧途,最终只能狠下心来重写UICollectionViewFlowLayout.下面我将用两种方法实现瀑布流,以及 ...
- IOS 瀑布流
本篇博客应该算的上CollectionView的高级应用了,从iOS开发之窥探UICollectionViewController(一)到今天的(五),可谓是由浅入深的窥探了一下UICollectio ...
- IOS 瀑布流UICollectionView实现
IOS 瀑布流UICollectionView实现 在实现瀑布流之前先来看看瀑布流的雏形(此方法的雏形 UICollectionView) 对于UICollectionView我们有几点注意事项 它和 ...
- iOS横向瀑布流的封装
前段时间, 做一个羡慕, 需要使用到瀑布流! 说道瀑布流, 或许大家都不陌生, 瀑布流的实现也有很多种! 从scrollView 到 tableView 书写的瀑布流, 然后再到2012年iOS6 苹 ...
- iOS 瀑布流之栅格布局
代码地址如下:http://www.demodashi.com/demo/14760.html 一 .效果预览 二.确定需求 由下面的需求示意图可知模块的最小单位是正方形,边长是屏幕宽除去边距间隔后的 ...
- iOS开发:一个瀑布流的设计与实现(已实现缓存池功能,该功能使得瀑布流cell可以循环利用)
一个瀑布流的实现有三种方式: 继承自UIScrollView,仿写UITableView的dataSource和delegate,创造一个缓存池用来实现循环利用cell 写多个UITableview( ...
- iOS开发:代码通用性以及其规范 第一篇(附带,自定义UITextView\进度条\双表显示\瀑布流 代码设计思路)
在iOS团队开发中,我见过一些人的代码,也修改过他们的代码.有的人的代码写的非常之规范.通用,几乎不用交流,就可以知道如何修改以及在它基础上扩展延生.有的人的代码写的很垃圾,一眼看过去,简直会怀疑自己 ...
随机推荐
- vim Project
VIM是Linux和Unix下常用的文本编辑工具,在编写代码和阅读代码中经常使用. 但VIM进行代码项目管理时,没有IDE集成开发工具方便,现在提供一个VIM插件Project,可以对代码项目进行简单 ...
- ubuntu14.04-rocketmq单机搭建
需要环境: jdk(1.6+) git(如果clone源码,需要git,没有git直接下载gar包也行) maven3.x在安装之前确定自己已经安装了jdk:java -version 先获取reck ...
- Windows Server 2012 (2008) 忘记密码重置方法 Windows 10 忘记密码
要使用windows server 2012安装DVD,选择光盘引导进入 进入修复系统---命令提示符---切换目录至系统目录--执行move命令 先备份 utilman.exe(他就是这个程 ...
- package-info.java文件详解
欢迎关注我的社交账号: 博客园地址: http://www.cnblogs.com/jiangxinnju/p/4781259.html GitHub地址: https://github.com/ji ...
- 【bzoj3625】【xsy1729】小朋友和二叉树
[bzoj3625]小朋友与二叉树 题意 我们的小朋友很喜欢计算机科学,而且尤其喜欢二叉树. 考虑一个含有n个互异正整数的序列c[1],c[2],...,c[n].如果一棵带点权的有根二叉树满足其所有 ...
- hdu 2102
简单的3维BFS 大写的YES和NO,这心粗的....唉 #include<iostream> #include<cstdio> #include<queue> u ...
- 《Java程序设计》第十周学习总结
20145224 <Java程序设计>第十周学习总结 网络编程 ·网络编程就是在两个或两个以上的设备(例如计算机)之间传输数据.程序员所作的事情就是把数据发送到指定的位置,或者接收到指定的 ...
- Java GC系列(2):Java垃圾回收是如何工作的?
本文由 ImportNew - 伍翀 翻译自 javapapers. 目录 垃圾回收介绍 垃圾回收是如何工作的? 垃圾回收的类别 垃圾回收监视和分析 本教程是为了理解基本的Java垃圾回收以及它是如何 ...
- Servlet复习1: 一个简单的Servlet的使用
Servlet学习 1. Servlet与JSP的关系 2. Servlet的声明周期 3. 一个简单的Servlet的使用方法 什么是Servlet? 什么又是JSP? 继承了javax.servl ...
- JQ添加移除css样式
1. addClass() - 添加CSS类 $("#target").addClass("newClass"); //#target 指的是需要添加样式的元素 ...