【Spring学习笔记-MVC-18.1】Spring MVC实现RESTful风格-同一资源,多种展现:xml-json-html
概要
1. 同一个资源,如果需要返回不同的形式,如:json、xml等;
- /user/getUserJson
- /user/getUserXML
2. 对同一资源的CRUD操作
- /user/addUser/
- /user/getUser/123
- /user/deleteUser/123
- /user/updateUser/123
内容协商介绍
RESTful服务中很重要的一个特性即是同一资源,多种表述,也即如下面描述的三种方式:
- 方式1:使用http request header: Accept
- GET /user/123 HTTP/1.1
- Accept: application/xml //将返回xml格式数据
- GET /user/123 HTTP/1.1
- Accept: application/json //将返回json格式数据
- 方式2:使用扩展名
- /user/123.xml 将返回xml格式数据
- /user/123.json 将返回json格式数据
- /user/123.html 将返回html格式数据
- 方式3:使用参数
- /user/123?format=xml //将返回xml数据
- /user/123?format=json //将返回json数据
以上三种优缺点比较
- 使用Accept header ==>由于浏览器的差异,一般不使用此种方式
- chrome:
- Accept:application/xml,application/xhtml+xml,textml;q=0.9,text/plain;q=0.8,image/png,*/*;q=0.5
- firefox:
- Accept:text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8
- IE8:
- Accept:image/gif, image/jpeg, image/pjpeg, image/pjpeg, application/x-shockwave-flash, application/x-silverlight, application/x-ms-application, application/x-ms-xbap, application/vnd.ms-xpsdocument, application/xaml+xml, */*
- 使用扩展名
- 使用参数
使用ContentNegotiatingViewResolver内容协商视图解析器
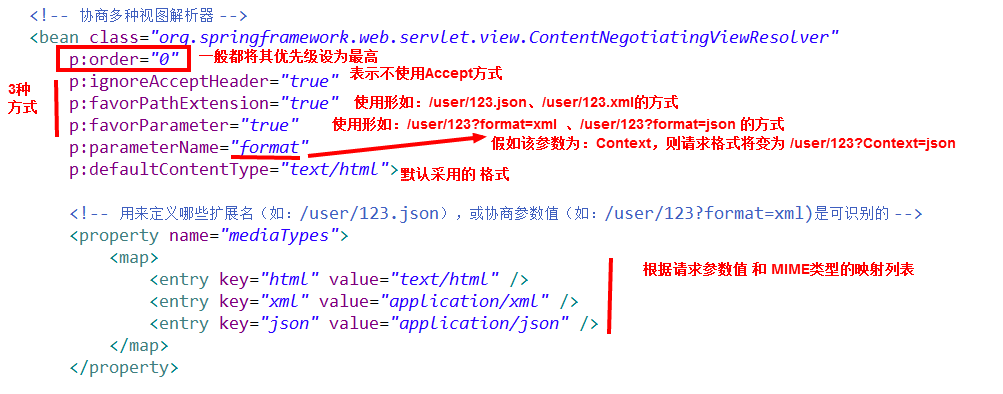

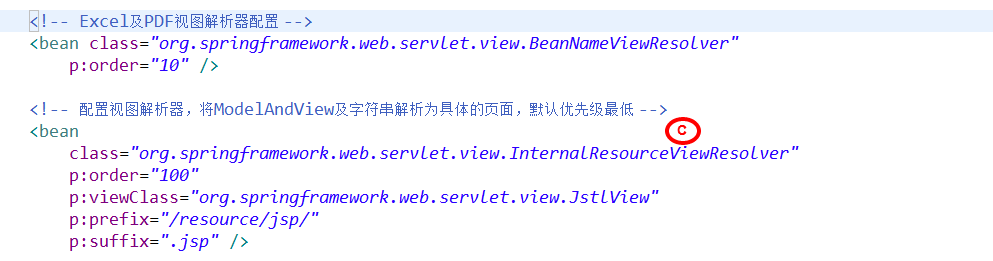
- /user/123.json 或
- /user/123?format=json
- /user/123.xml
- /user/123?format=xml
- xpp3_min-xxx.jar;
- xstream-xxx.jar。
- /user/123.html
- /user/123?format=html
- /user/123
<?xml version="1.0" encoding="UTF-8" ?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd">
<!-- 扫描web包,应用Spring的注解 -->
<context:component-scan base-package="com.ll.web"/>
<mvc:annotation-driven/>
<!-- 协商多种视图解析器 -->
<bean class="org.springframework.web.servlet.view.ContentNegotiatingViewResolver"
p:order="0"
p:ignoreAcceptHeader="true"
p:favorPathExtension="true"
p:favorParameter="true"
p:parameterName="format"
p:defaultContentType="text/html">
<!-- 用来定义哪些扩展名(如:/user/123.json),或协商参数值(如:/user/123?format=xml)是可识别的 -->
<property name="mediaTypes">
<map>
<entry key="html" value="text/html" />
<entry key="xml" value="application/xml" />
<entry key="json" value="application/json" />
</map>
</property>
<property name="defaultViews">
<list>
<!-- for application/json -->
<bean class="org.springframework.web.servlet.view.json.MappingJacksonJsonView"/>
<!-- for application/xml -->
<bean class="org.springframework.web.servlet.view.xml.MarshallingView">
<property name="marshaller">
<bean class="org.springframework.oxm.xstream.XStreamMarshaller"/>
</property>
</bean>
</list>
</property>
</bean>
<!-- Excel及PDF视图解析器配置 -->
<bean class="org.springframework.web.servlet.view.BeanNameViewResolver"
p:order="10" />
<!-- 配置视图解析器,将ModelAndView及字符串解析为具体的页面,默认优先级最低 -->
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver"
p:order="100"
p:viewClass="org.springframework.web.servlet.view.JstlView"
p:prefix="/resource/jsp/"
p:suffix=".jsp" />
<!-- spring mvc对静态资源的访问 -->
<mvc:resources mapping="/resource/**" location="/resource/**" />
</beans>
控制层代码
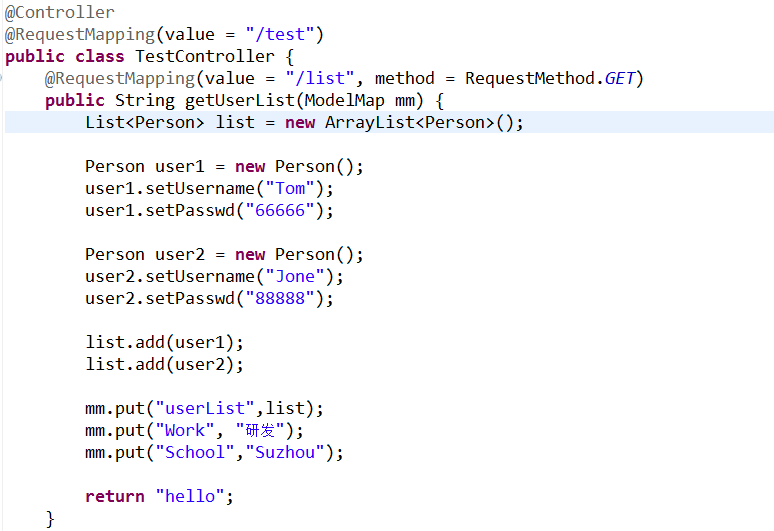
测试
1. json格式

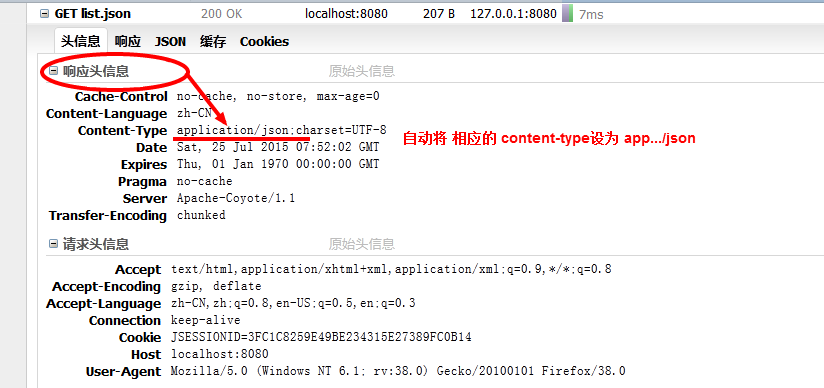
2. xml格式

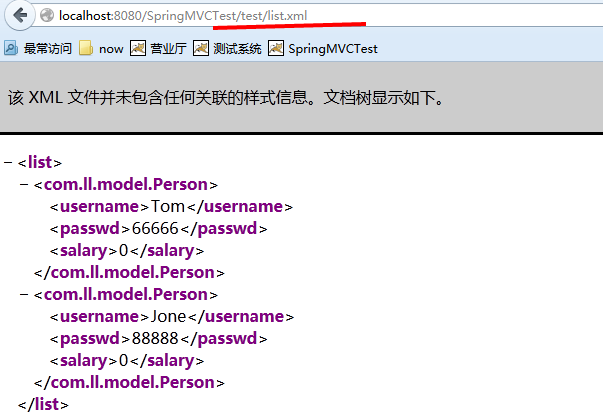
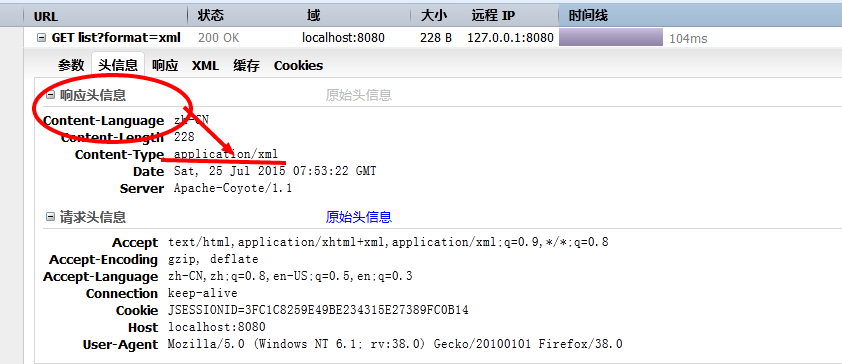

4. 不带任何参数



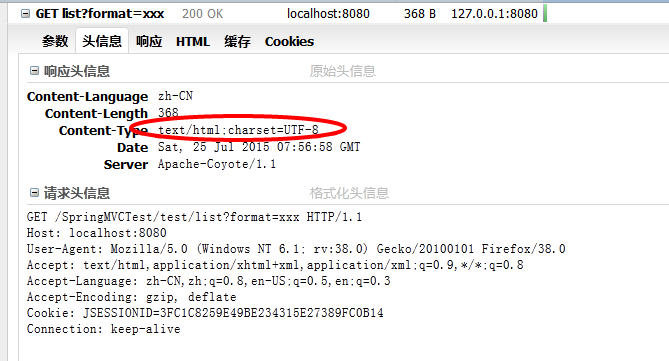
其他
博客:
附件列表
【Spring学习笔记-MVC-18.1】Spring MVC实现RESTful风格-同一资源,多种展现:xml-json-html的更多相关文章
- 【Spring学习笔记-MVC-15.1】Spring MVC之异常处理=404界面
作者:ssslinppp 异常处理请参考前篇博客:<[Spring学习笔记-MVC-15]Spring MVC之异常处理>http://www.cnblogs.com/sssl ...
- 【Spring学习笔记-MVC-13.2】Spring MVC之多文件上传
作者:ssslinppp 1. 摘要 前篇文章讲解了单文件上传<[Spring学习笔记-MVC-13]Spring MVC之文件上传>http://www.cnblogs.co ...
- 【Spring学习笔记-MVC-5】利用spring MVC框架,实现ajax异步请求以及json数据的返回
作者:ssslinppp 时间:2015年5月26日 15:32:51 1. 摘要 本文讲解如何利用spring MVC框架,实现ajax异步请求以及json数据的返回. Spring MV ...
- Spring学习笔记(一)—— Spring介绍及入门案例
一.Spring概述 1.1 Spring是什么 Spring是一个开源框架,是于2003年兴起的一个轻量级的Java开发框架, 由Rod Johnson 在其著作<Expert one on ...
- Spring学习笔记4—流程(Spring Web Flow)
Spring Web Flow是Spring框架的子项目,作用是让程序按规定流程运行. 1 安装配置Spring Web Flow 虽然Spring Web Flow是Spring框架的子项目,但它并 ...
- Spring学习笔记(二)Spring基础AOP、IOC
Spring AOP 1. 代理模式 1.1. 静态代理 程序中经常需要为某些动作或事件作下记录,以便在事后检测或作为排错的依据,先看一个简单的例子: import java.util.logging ...
- Spring学习笔记(四)-- Spring事务全面分析
通过本系列的文章对Spring的介绍,我们对Spring的使用和两个核心功能IOC.AOP已经有了初步的了解,结合我个人工作的情况,因为项目是金融系 统.那对事务的控制是不可缺少的.而且是很严格的控制 ...
- Spring学习笔记(四)—— Spring中的AOP
一.AOP概述 AOP(Aspect Oriented Programming),即面向切面编程,可以说是OOP(Object Oriented Programming,面向对象编程)的补充和完善.O ...
- spring学习笔记(二)spring中的事件及多线程
我们知道,在实际开发中为了解耦,或者提高用户体验,都会采用到异步的方式.这里举个简单的例子,在用户注册的sh时候,一般我们都会要求手机验证码验证,邮箱验证,而这都依赖于第三方.这种情况下,我们一般会通 ...
随机推荐
- 腾讯优测干货精选| 安卓开发新技能Get -常用必备小工具汇总
文/腾讯公司 陈江峰 优测小优有话说: 移动研发及测试干货哪里找?腾讯优测-优社区你值得拥有~ 开发同学们都知道,安卓开发路上会碰到很多艰难险阻,一不小心就被KO.这时候,没有新技能傍身怎么行?今天我 ...
- 238. Product of Array Except Self
Given an array of n integers where n > 1, nums, return an array output such that output[i] is equ ...
- 菜鳥日記:為 Github 添加 ssh
這只是一篇求真務實言簡意賅的菜鳥日記 記錄了碼盲在OSX 中為Github添加 ssh 的過程 要從 Github 上克隆個源碼到本地,發現無 ssh 密鈅 於是開到官網幫助照貓畫虎如下: 1.打開 ...
- webrtc编译之libcommonaudio
[170/1600] CXX obj/webrtc/common_audio/common_audio.audio_util.o[171/1600] CXX obj/webrtc/common_aud ...
- JS网址正则验证
function IsURL(str_url){ var strRegex = "^((https|http|ftp|rtsp|mms)?://)" + "?(([0-9 ...
- CSS基础知识点(二)——选择器
在这一篇中,主要总结一下CSS中的选择器. 参考:http://www.cnblogs.com/webblog/archive/2009/07/07/1518274.html CSS中的选择器主要包括 ...
- html5中新的标准属性
属性 值 描述accesskey ...
- leetcode 99 Recover Binary Search Tree ----- java
Two elements of a binary search tree (BST) are swapped by mistake. Recover the tree without changing ...
- HDU-5806 NanoApe Loves Sequence Ⅱ(two-pointer或二分)
题目大意:给一个整数序列,统计<k,m>子序列的数目.<k,m>序列是满足第k大的数字不比m小的连续子序列. 题目分析:维护一个不小于m的数的个数的后缀和数组,可以枚举序列起点 ...
- hdu2647 拓扑序
题意:年终要给 n 个员工发奖金,每个人的起始金额是888,有些人觉得自己做的比另一个人好所以应该多得一些钱,问最少需要花多少钱,如果不能满足所有员工的要求,输出 -1 拓扑排序,从奖金少的向奖金多的 ...