python实现基于CGI的Web应用
python实现基于CGI的Web应用
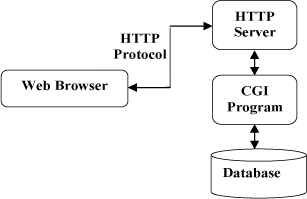

from http.server import HTTPServer, CGIHTTPRequestHandler port = 8081 httpd = HTTPServer(('', port), CGIHTTPRequestHandler)
print("Starting simple_httpd on port: " + str(httpd.server_port))
httpd.serve_forever()

2、index.html
首页;URL: “http://localhost:8081/cgi-bin/book_list_view.py” 将调用 cgi-bin文件夹下的book_list_view.py

<html>
<head>
<title>BookStore</title>
</head>
<body>
<h1>Welcome to My Book Store.</h1>
<img src="resource/books.png">
<h3>
please choose your favorite book, click <a href="cgi-bin/book_list_view.py">here</a>.
</h3>
<p>
<strong> Enjoy!</strong>
</p>
</body>
</html>

3、book_list_view.py
图书清单页面。用户选择要查看的图书,提交表单,然后调动图书详细界面。

#Python标准库中定义的CGI跟踪模块:cgibt
import cgitb
cgitb.enable()
#启用这个模块时,会在web浏览器上显示详细的错误信息。enable()函数打开CGI跟踪
#CGI脚本产生一个异常时,Python会将消息显示在stderr(标准输出)上。CGI机制会忽略这个输出,因为它想要的只是CGI的标准输出(stdout) import template.yate as yate
import service.book_service as book_service #CGI标准指出,服务器端程序(CGI脚本)生成的任何输出都将会由Web服务器捕获,并发送到等待的web浏览器。具体来说,会捕获发送到Stdout(标准输出)的所有内容
#一个CGI脚本由2部分组成, 第一部分输出 Response Headers, 第二部分输出常规的html.
print("Content-type:text/html\n")#Response Headers
#网页内容:有html标签组成的文本
print('<html>')
print('<head>')
print('<title>Book List</title>')
print('</head>')
print('<body>')
print('<h2>Book List:</h2>')
print(yate.start_form('book_detail_view.py'))
book_dict=book_service.get_book_dict()
for book_name in book_dict:
print(yate.radio_button('bookname',book_dict[book_name].name))
print(yate.end_form('detail'))
print(yate.link("/index.html",'Home'))
print('</body>')
print('</html>')

4、yate.py
自定义的简单模板,用于快捷生成html

def start_form(the_url, form_type="POST"):
return('<form action="' + the_url + '" method="' + form_type + '">') def end_form(submit_msg="Submit"):
return('<input type=submit value="' + submit_msg + '"></form>') def radio_button(rb_name, rb_value):
return('<input type="radio" name="' + rb_name +
'" value="' + rb_value + '"> ' + rb_value + '<br />') def u_list(items):
u_string = '<ul>'
for item in items:
u_string += '<li>' + item + '</li>'
u_string += '</ul>'
return(u_string) def header(header_text, header_level=2):
return('<h' + str(header_level) + '>' + header_text +
'</h' + str(header_level) + '>')
def para(para_text):
return('<p>' + para_text + '</p>') def link(the_link,value):
link_string = '<a href="' + the_link + '">' + value + '</a>'
return(link_string)

5、book_detail_view.py
图书详细页面

import cgitb
cgitb.enable() import cgi
import template.yate as yate
import service.book_service as book_service
import template.yate as yate #使用cig.FieldStorage() 访问web请求发送给web服务器的数据,这些数据为一个Python字典
form_data = cgi.FieldStorage() print("Content-type:text/html\n")
print('<html>')
print('<head>')
print('<title>Book List</title>')
print('</head>')
print('<body>')
print(yate.header('Book Detail:'))
try:
book_name = form_data['bookname'].value
book_dict=book_service.get_book_dict()
book=book_dict[book_name]
print(book.get_html)
except KeyError as kerr:
print(yate.para('please choose a book...'))
print(yate.link("/index.html",'Home'))
print(yate.link("/cgi-bin/book_list_view.py",'Book List'))
print('</body>')
print('</html>')

6、Book.py
图书类

from template import yate class Book:
def __init__(self,name,author,price):
self.name=name
self.author=author
self.price=price @property
def get_html(self):
html_str=''
html_str+=yate.header('BookName:',4)+yate.para(self.name)
html_str+=yate.header('Author:',4)+yate.para(self.author)
html_str+=yate.header('Price:',4)+yate.para(self.price)
return(html_str)

7、book_service.py
图书业务逻辑类

from model.Book import Book def get_book_dict():
book_dict={}
try:
with open('book.txt','r') as book_file:
for each_line in book_file:
book=parse(each_line)
book_dict[book.name]=book
except IOError as ioerr:
print("IOErr:",ioerr)
return(book_dict) def parse(book_info):
(name,author,price)=book_info.split(';')
book=Book(name,author,price)
return(book)

8、book.txt
待显示的图书信息(书名;作者;价格)
The Linux Programming Interface: A Linux and UNIX System Prog;Michael Kerrisk;$123.01
HTML5 and CSS3, Illustrated Complete (Illustrated Series);Jonathan Meersman Sasha Vodnik;$32.23
Understanding the Linux Kernel;Daniel P. Bovet Marco Cesati;$45.88
Getting Real;Jason Fried, Heinemeier David Hansson, Matthew Linderman;$87.99
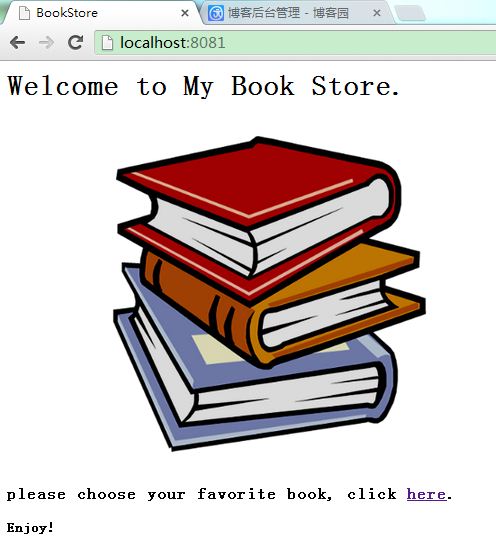

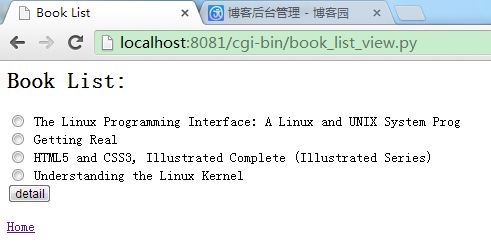
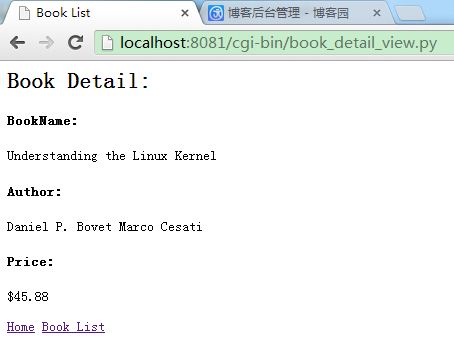
python实现基于CGI的Web应用的更多相关文章
- Python flask 基于 Flask 提供 RESTful Web 服务
转载自 http://python.jobbole.com/87118/ 什么是 REST REST 全称是 Representational State Transfer,翻译成中文是『表现层状态转 ...
- python web编程-CGI帮助web服务器处理客户端编程
这几篇博客均来自python核心编程 如果你有任何疑问,欢迎联系我或者仔细查看这本书的地20章 另外推荐下这本书,希望对学习python的同学有所帮助 概念预热 eb客户端通过url请求web服务器里 ...
- [ Python ] Flask 基于 Web开发 大型程序的结构实例解析
作为一个编程入门新手,Flask是我接触到的第一个Web框架.想要深入学习,就从<FlaskWeb开发:基于Python的Web应用开发实战>这本书入手,本书由于是翻译过来的中文版,理解起 ...
- 什么是 WSGI -- Python 中的 “CGI” 接口简介
今天在 git.oschina 的首页上看到他们推出演示平台,其中,Python 的演示平台支持 WSGI 接口的应用.虽然,这个演示平台连它自己提供的示例都跑不起来,但是,它还是成功的勾起了我对 W ...
- Python全栈开发:web框架们
Python的WEB框架 Bottle Bottle是一个快速.简洁.轻量级的基于WSIG的微型Web框架,此框架只由一个 .py 文件,除了Python的标准库外,其不依赖任何其他模块. 1 2 3 ...
- 【python之路42】web框架们的具体用法
Python的WEB框架 (一).Bottle Bottle是一个快速.简洁.轻量级的基于WSIG的微型Web框架,此框架只由一个 .py 文件,除了Python的标准库外,其不依赖任何其他模块. p ...
- Python(九)Tornado web 框架
一.简介 Tornado 是 FriendFeed 使用的可扩展的非阻塞式 web 服务器及其相关工具的开源版本.这个 Web 框架看起来有些像web.py 或者 Google 的 webapp,不过 ...
- 用Python写一个简单的Web框架
一.概述 二.从demo_app开始 三.WSGI中的application 四.区分URL 五.重构 1.正则匹配URL 2.DRY 3.抽象出框架 六.参考 一.概述 在Python中,WSGI( ...
- Flask —— 使用Python和OpenShift进行即时Web开发
最近Packtpub找到了我,让我给他们新出版的关于Flask的书写书评.Flask是一个很流行的Python框架.那本书是Ron DuPlain写的<Flask 即时Web开发>.我决定 ...
随机推荐
- 关于重写ID3 Algorithm Based On MapReduceV1/C++/Streaming的一些心得体会
心血来潮,同时想用C++连连手.面对如火如荼的MP,一阵念头闪过,如果把一些ML领域的玩意整合到MP里面是不是很有意思 确实很有意思,可惜mahout来高深,我也看不懂.干脆自动动手丰衣足食,加上自己 ...
- .NET:从 Mono、.NET Core 说起
魅力 .NET:从 Mono..NET Core 说起 前段时间,被问了这样一个问题:.NET 应用程序是怎么运行的? 当时大概愣了好久,好像也没说出个所以然,得到的回复是:这是 .NET 程序员最基 ...
- C语言库函数大全及应用实例十一
原文:C语言库函数大全及应用实例十一 [编程资料]C语言库函数大全及应用实例十一 函数名: setbkcolor 功 能 ...
- Hibernate实体映射配置(XML)简单三步完美配置
我们在使用Hibernate框架的时候,非常纠结的地方就是实体和表之间的映射,今天借助汤老师的思路写了小教程,以后配置不用纠结了! 第一步:写注释 格式为:?属性,表达的是本对象与?的?关系. 例:“ ...
- Windows下结束tomcat进程,dos命令
Microsoft Windows [版本 6.1.7601]版权所有 (c) 2009 Microsoft Corporation.保留所有权利. C:\Users\Administrator> ...
- java中Integer包装类的具体解说(java二进制操作,全部进制转换)
程序猿都非常懒,你懂的! 今天为大家分享的是Integer这个包装类.在现实开发中,我们往往须要操作Integer,或者各种进制的转换等等.我今天就为大家具体解说一下Integer的使用吧.看代码: ...
- 有趣的游戏:Google XSS Game
Google最近出了一XSS游戏: https://xss-game.appspot.com/ 我这个菜鸟看提示,花了两三个小时才全过了.. 这个游戏的规则是仅仅要在攻击网页上弹出alert窗体就能够 ...
- ExtJS4 自己定义基于配置的高级查询1
今天在编码过程中遇到一个问题,临时还没解决,先记录下来 上面是我做的高级查询面板..字段名和值都是读取配置文件,依据用户选择不同的字段名,自己主动载入不同的值列表,关系是与或 问题来了,我在字段名那个 ...
- C# 以嵌入到窗体的方式打开外部exe
using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using S ...
- 使用sqlldr向Oracle导入大的文本(txt)文件
我们有多种方法可以向Oracle数据库里导入文本文件,但如果导入的文本文件过大,例如5G,10G的文本文件,有些方法就不尽如意了,例如PLSQL Developer中的导入文本功能,如果文本文件过大, ...