[iOS微博项目 - 3.5] - 封装业务
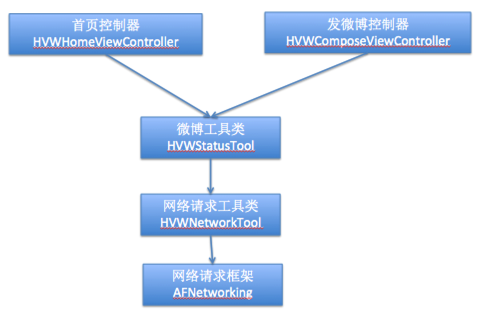
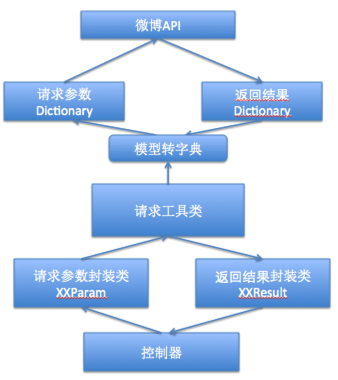
//
// HVWBaseParam.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/9.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import <Foundation/Foundation.h> @interface HVWBaseParam : NSObject /** 访问令牌 */
@property(nonatomic, copy) NSString *access_token; @end
//
// HVWBaseParam.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/9.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWBaseParam.h"
#import "HVWAccountInfo.h"
#import "HVWAccountInfoTool.h" @implementation HVWBaseParam - (NSString *)access_token {
if (nil == _access_token) {
_access_token = [HVWAccountInfoTool accountInfo].access_token;
}
return _access_token;
} @end
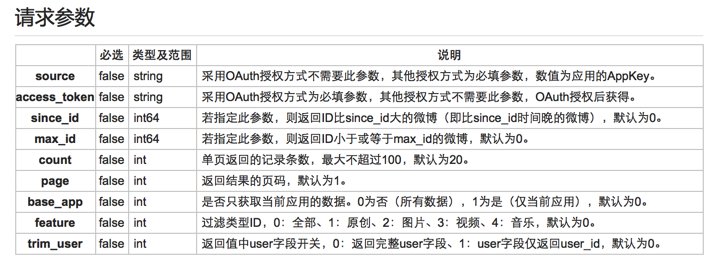
//
// HVWHomeStatusParam.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/9.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWBaseParam.h" @interface HVWHomeStatusParam : HVWBaseParam /** false string 采用OAuth授权方式不需要此参数,其他授权方式为必填参数,数值为应用的AppKey。 */
@property(nonatomic, copy) NSString *source; /** false int64 若指定此参数,则返回ID比since_id大的微博(即比since_id时间晚的微博),默认为0。 */
@property(nonatomic, strong) NSNumber *since_id; /** false int64 若指定此参数,则返回ID小于或等于max_id的微博,默认为0。 */
@property(nonatomic, strong) NSNumber *max_id; /** false int 单页返回的记录条数,最大不超过100,默认为20。 */
@property(nonatomic, strong) NSNumber *count; /** false int 返回结果的页码,默认为1。 */
@property(nonatomic, strong) NSNumber *page; /** false int 是否只获取当前应用的数据。0为否(所有数据),1为是(仅当前应用),默认为0。 */
@property(nonatomic, strong) NSNumber *base_app; /** false int 过滤类型ID,0:全部、1:原创、2:图片、3:视频、4:音乐,默认为0。 */
@property(nonatomic, strong) NSNumber *feature; /** false int 返回值中user字段开关,0:返回完整user字段、1:user字段仅返回user_id,默认为0。 */
@property(nonatomic, strong) NSNumber *trim_user; @end
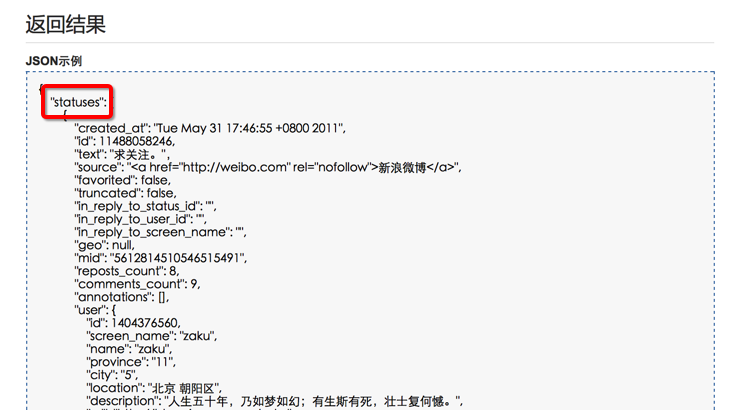
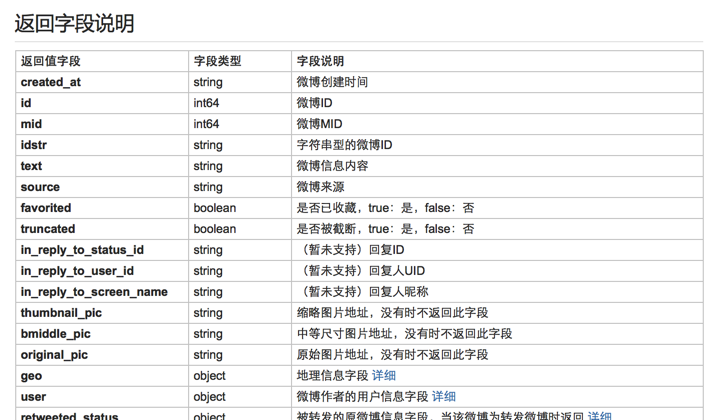
//
// HVWHomeStatusResult.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/9.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import <Foundation/Foundation.h>
#import "HVWStatus.h" @interface HVWHomeStatusResult : NSObject /** 微博数组,里面装的HVWStatus模型 */
@property(nonatomic, strong) NSArray *statuses; /** 近期微博总数 */
@property(nonatomic, assign) int total_number; @end
//
// HVWHomeStatusResult.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/9.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWHomeStatusResult.h"
#import "MJExtension.h" @implementation HVWHomeStatusResult /** 指明将json数组元素转为什么模型类 */
- (NSDictionary *)objectClassInArray {
return @{@"statuses":[HVWStatus class]};
} @end
//
// HVWBaseTool.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/10.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import <Foundation/Foundation.h> @interface HVWBaseTool : NSObject /** GET请求 */
+ (void) getWithUrl:(NSString *)url parameters:(id)parameters resultClass:(Class)resultClass success:(void (^)(id))success failure:(void (^)(NSError *))failure; /** POST请求(不带文件参数) */
+ (void) postWithUrl:(NSString *)url parameters:(id)parameters resultClass:(Class)resultClass success:(void (^)(id))success failure:(void (^)(NSError *))failure; /** POST请求(带文件参数) */
+ (void) postWithUrl:(NSString *)url parameters:(id)parameters filesData:(NSArray *)filesData resultClass:(Class)resultClass success:(void (^)(id))success failure:(void (^)(NSError *))failure; @end
//
// HVWBaseTool.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/10.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWBaseTool.h"
#import "HVWNetworkTool.h"
#import "MJExtension.h" @implementation HVWBaseTool /** GET请求 */
+ (void) getWithUrl:(NSString *)url parameters:(id)parameters resultClass:(Class)resultClass success:(void (^)(id))success failure:(void (^)(NSError *))failure { // 解析出参数
NSDictionary *param = [parameters keyValues]; [HVWNetworkTool get:url parameters:param success:^(id responseObject) {
if (success) {
id result = [resultClass objectWithKeyValues:responseObject];
success(result);
}
} failure:^(NSError *error) {
if (failure) {
if (failure) {
failure(error);
}
}
}];
} /** POST请求(不带文件参数) */
+ (void) postWithUrl:(NSString *)url parameters:(id)parameters resultClass:(Class)resultClass success:(void (^)(id))success failure:(void (^)(NSError *))failure { // 解析出参数
NSDictionary *param = [parameters keyValues]; [HVWNetworkTool post:url parameters:param success:^(id responseObject) {
if (success) {
id result = [resultClass objectWithKeyValues:responseObject];
success(result);
}
} failure:^(NSError *error) {
if (failure) {
if (failure) {
failure(error);
}
}
}];
} /** POST请求(带文件参数) */
+ (void) postWithUrl:(NSString *)url parameters:(id)parameters filesData:(NSArray *)filesData resultClass:(Class)resultClass success:(void (^)(id))success failure:(void (^)(NSError *))failure { // 解析出参数
NSDictionary *param = [parameters keyValues]; [HVWNetworkTool post:url parameters:param filesData:filesData success:^(id responseObject) {
if (success) {
id result = [resultClass objectWithKeyValues:responseObject];
success(result);
}
} failure:^(NSError *error) {
if (failure) {
if (failure) {
failure(error);
}
}
}];
} @end
//
// HVWStatusTool.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/9.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import <Foundation/Foundation.h>
#import "HVWBaseTool.h"
#import "HVWHomeStatusParam.h"
#import "HVWHomeStatusResult.h" @interface HVWStatusTool : HVWBaseTool /** 获取首页微博数据 */
+ (void) statusWithParameters:(HVWHomeStatusParam *)parameters success:(void (^)(HVWHomeStatusResult *statusResult))success failure:(void (^)(NSError *error))failure; @end
//
// HVWStatusTool.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/9.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWStatusTool.h"
#import "MJExtension.h" @implementation HVWStatusTool /** 获取首页微博数据 */
+ (void) statusWithParameters:(HVWHomeStatusParam *)parameters success:(void (^)(HVWHomeStatusResult *statusResult))success failure:(void (^)(NSError *error))failure {
// 发送请求
[self getWithUrl:@"https://api.weibo.com/2/statuses/home_timeline.json" parameters:parameters resultClass:[HVWHomeStatusResult class] success:success failure:failure];
} @end
[iOS微博项目 - 3.5] - 封装业务的更多相关文章
- [iOS微博项目 - 2.5] - 封装授权和用户信息读写业务
github: https://github.com/hellovoidworld/HVWWeibo A.封装授权业务 1.把app的授权信息移动到HVWWeibo-Prefix.pch中作为公共 ...
- [iOS微博项目 - 3.3] - 封装网络请求
github: https://github.com/hellovoidworld/HVWWeibo A.封装网络请求 1.需求 为了避免代码冗余和对于AFN框架的多处使用导致耦合性太强,所以把网 ...
- [iOS微博项目 - 1.6] - 自定义TabBar
A.自定义TabBar 1.需求 控制TabBar内的item的文本颜色(普通状态.被选中状态要和图标一致).背景(普通状态.被选中状态均为透明) 重新设置TabBar内的item位置,为下一步在Ta ...
- [iOS微博项目 - 3.4] - 获取用户信息
github: https://github.com/hellovoidworld/HVWWeibo A.获取用户信息 1.需求 获取用户信息并储存 把用户昵称显示在“首页”界面导航栏的标题上 ...
- [iOS微博项目 - 3.1] - 发微博界面
github: https://github.com/hellovoidworld/HVWWeibo A.发微博界面:自定义UITextView 1.需求 用UITextView做一个编写微博的输 ...
- [iOS微博项目 - 2.6] - 获取微博数据
github: https://github.com/hellovoidworld/HVWWeibo A.新浪获取微博API 1.读取微博API 2.“statuses/home_time ...
- [iOS微博项目 - 1.0] - 搭建基本框架
A.搭建基本环境 github: https://github.com/hellovoidworld/HVWWeibo 项目结构: 1.使用代码构建UI,不使用storyboard ...
- [iOS微博项目 - 4.6] - 微博配图
github: https://github.com/hellovoidworld/HVWWeibo A.微博配图 1.需求 显示原创微博.转发微博的缩略图 4张图使用2x2布局,其他使用3x3布局, ...
- [iOS微博项目 - 4.0] - 自定义微博cell
github: https://github.com/hellovoidworld/HVWWeibo A.自定义微博cell基本结构 1.需求 创建自定义cell的雏形 cell包含:内容.工具条 内 ...
随机推荐
- Away3D引擎学习笔记(三)模型拾取(翻译)
原文详见http://away3d.com/tutorials/Introduction_to_Mouse_Picking.本文若有翻译不对的地方,敬请指出. 本教程详细介绍了Away3D 4.x中鼠 ...
- $q服务的使用
1. 创建一个Service,去服务器读取数据: // $q 是内置服务,所以可以直接使用 ngApp.factory('UserInfo', ['$http', '$q', function ($h ...
- sass 的使用
普通变量 ? 1 $fontSize:12px; 默认变量 ? 1 $fontSize:12px; !default; 变量覆盖:只需要在默认变量之前重新声明下变量即可 ? 1 2 $fontSize ...
- [转]ISTQB FL初级认证考试资料(中文)
[转]ISTQB FL初级认证考试资料(中文) 2015-06-22 ISTQB作为一个专业的提供软件测试认证的机构,得到了全球软件测试人员的认可.目前中国有越来越多的人已经获得或者希望获得ISTQB ...
- 使用JSON Web Tokens和Spring实现微服务
http://www.jdon.com/dl/best/json-web-tokens-spring-cloud-microservices.html
- 解决将Ubuntu下导出的requirements.txt到Centos服务器上面出现pkg-resource的版本为0.0.0
最直接有效的方法: 原因:
- 在容器中使用erase函数,迭代器的处理
在c++编程中,用到迭代器的时候,往往不知道如何删除当前迭代器指向的元素. erase函数: 返回下一个迭代器. 只使用vector的erase函数,记住,该函数是迭代器失效,返回下一个迭代器. ...
- 虚拟化–操作系统级 LXC Linux Containers内核轻量级虚拟化技术
友情提示:非原文链接可能会影响您的阅读体验,欢迎查看原文.(http://blog.geekcome.com) 原文地址:http://blog.geekcome.com/archives/288 软 ...
- SQL语句字符串处理大全
常用的字符串函数有: 一.字符转换函数 1.ASCII() 返回字符表达式最左端字符的ASCII 码值.在ASCII()函数中,纯数字的字符串可不用‘’括起来,但含其它字符的字符串必须用‘’括起来使用 ...
- docker 容器内ping不通外网
其实只要重启docker就好了 systemctl restart docker https://blog.csdn.net/yangzhenping/article/details/43567155