UVA 1085 House of Cards(对抗搜索)
Description
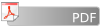
Axel and Birgit like to play a card game in which they build a house of cards, gaining (or losing) credits as they add cards to the house. Since they both have very steady hands, the house of cards never collapses. They use half a deck of standard playing cards. A standard deck has four suits, two are red and two are black. Axel and Birgit use only two suits, one red, one black. Each suit has 13 ranks. We use the notation 1R, 2R, ..., 13R, 1B, 2B, ..., 13B to denote ranks and colors.
The players begin by selecting a subset of the cards, usually all cards of rank up to some maximum value M. After shuffling the modified deck, they take eight cards from the top of the deck and place them consecutively from left to right to form four ``peaks." For instance, if M = 13 and if the first ten cards (from 26) are:
6B 3R 5B 2B 1B 5R 13R 7B 11R 1R ...
then the game starts off with four peaks and three valleys as shown in Figure 7.

The remaining cards are placed face up, in a single row.
Each player is identified with a color, red or black. Birgit is always black and Axel is always red. The color of the first card used for the peaks and valleys determines which player has the first turn. For the example in Figure 7, Birgit has the first turn since the first card is 6B.
Players alternate taking turns. A turn consists of removing the card from the front of the row of cards and then doing one of the following:
- Holding the card until the next turn (this is a ``held card").
- Covering the valley between two peaks with the drawn card or the held card, forming a ``floor". The remaining card, if any, is held.
- Placing two cards over a floor, forming a peak (one of the cards must be a ``held'' card).
Not all options are always available. At most one card may be held at any time, so the first option is possible only if the player is not already holding a card.
Since the cards in the row are face up, both players know beforehand the order in which the cards are drawn.
If the player forms a downward-pointing triangle by adding a floor, or an upward-pointing triangle by adding a peak, then the scores are updated as follows. The sum of the ranks of the three cards in the triangle is added to the score of the player whose color is the same as the majority of cards in the triangle. If no triangle is formed during a play, both scores remain unchanged.
In the example from Figure 7, if Birgit places her card (11R) on the middle valley, she gains 14 points. If she places her card on the left valley, Axel gains 19 points. If she places her card on the right valley, Axel gains 29 points.
If no more cards remain to be drawn at the end of a turn, the game is over. If either player holds a card at this time, the rank of that card is added to (subtracted from) that player's score if the color of the card is the same as (different from) that player's color.
When the game is over, the player with the lower score pays a number of Swedish Kronor (Kronor is the plural of Krona) equal to the difference between the two scores to the other player. In case of a tie there is no pay out.
You must write a program that takes a description of a shuffled deck and one of the players' names and find the highest amount that player can win (or the player's minimum loss), assuming that the other player always makes the best possible moves.
Input
The input file contains multiple test cases representing different games. Each test case consists of a name (either `Axel' or `Birgit'), followed by a maximum rank M ( 5M
13), followed by the rank and color of the 2M cards in the deck in their shuffled order. Every combination of rank (from 1 through M) and color will appear once in this list. The first eight cards form the initial row of peaks from left to right in the order drawn, and the remaining items show the order of the rest of the cards.
The final test case is followed by a line containing the word `End'.
Output
For each test case, print the test case number (starting with 1), the name of the player for the test case, and the amount that the player wins or loses. If there is a tie, indicate this instead of giving an amount. Follow the sample output format.
题目大意:略。
思路:剪枝搜索。
代码(1895MS):
#include <cstdio>
#include <iostream>
#include <cstring>
#include <algorithm>
#include <vector>
using namespace std; const int UP = ;
const int FLOOR = ;
const int DOWN = ;
const int INF = 0x7fffffff; int deck[];
char s[], c;
int n; inline int abs(int x) {int y = x >> ; return (x + y) ^ y;} inline int getScore(int a, int b, int c) {
int ret = abs(a) + abs(b) + abs(c);
int sgn = (a > ) + (b > ) + (c > );
if(sgn > ) return ret;
else return -ret;
} struct State {
int card[], type[];
int hold[];
int pos, score; State() {
for(int i = ; i < ; ++i) {
card[i] = deck[i];
type[i] = (i % == ) ? UP : DOWN;
}
hold[] = hold[] = score = ;
pos = ;
} bool isFinal() {
if(pos == * n) {
score += hold[] + hold[];
hold[] = hold[] = ;
return true;
}
return false;
} State child() const {
State s;
memcpy(&s, this, sizeof(s));
s.pos = pos + ;
return s;
} void expand(int player, vector<State> &ret) const {
int &cur = deck[pos];
if(hold[player] == ) {
State s = child();
s.hold[player] = cur;
ret.push_back(s);
}
for(int i = ; i < ; ++i) if(type[i] == DOWN && type[i + ] == UP) {
State s = child();
s.score += getScore(card[i], card[i + ], cur);
s.type[i] = s.type[i + ] = FLOOR;
s.card[i] = s.card[i + ] = cur;
ret.push_back(s);
if(hold[player] != ) {
State s = child();
s.score += getScore(card[i], card[i + ], hold[player]);
s.type[i] = s.type[i + ] = FLOOR;
s.card[i] = s.card[i + ] = hold[player];
s.hold[player] = cur;
ret.push_back(s);
}
}
if(hold[player] != ) {
for(int i = ; i < ; ++i) if(type[i] == FLOOR && type[i + ] == FLOOR && card[i] == card[i + ]) {
State s = child();
s.score += getScore(card[i], hold[player], cur);
s.type[i] = UP; s.type[i + ] = DOWN;
s.card[i] = cur; s.card[i + ] = hold[player]; s.hold[player] = ;
ret.push_back(s);
swap(s.card[i], s.card[i + ]);
ret.push_back(s);
}
}
}
}; int alphabeta(State &s, int player, int alpha, int beta) {
if(s.isFinal()) return s.score;
vector<State> children;
s.expand(player, children);
int n = children.size();
for(int i = ; i < n; ++i) {
int v = alphabeta(children[i], player ^ , alpha, beta);
if(!player) alpha = max(alpha, v);
else beta = min(beta, v);
if(beta <= alpha) break;
}
return !player ? alpha : beta;
} int main() {
int t = ;
while(scanf("%s", s) != EOF && *s != 'E') {
scanf("%d", &n);
for(int i = ; i < * n; ++i) {
scanf("%d%c", &deck[i], &c);
if(c == 'B') deck[i] = -deck[i];
}
int start = !(deck[] > );
State beg;
int ans = alphabeta(beg, start, -INF, INF);
printf("Case %d: ", ++t);
if(s[] == 'B') ans = -ans;
if(ans == ) puts("Axel and Birgit tie");
else if(ans > ) printf("%s wins %d\n", s, ans);
else printf("%s loses %d\n", s, -ans);
}
}
UVA 1085 House of Cards(对抗搜索)的更多相关文章
- UVA - 10118Free Candies(记忆化搜索)
题目:UVA - 10118Free Candies(记忆化搜索) 题目大意:给你四堆糖果,每一个糖果都有颜色.每次你都仅仅能拿随意一堆最上面的糖果,放到自己的篮子里.假设有两个糖果颜色同样的话,就行 ...
- BZOJ 3106: [cqoi2013]棋盘游戏(对抗搜索)
题目:http://www.lydsy.com/JudgeOnline/problem.php?id=3106 对抗搜索,f[x][y][a][b][c][d]表示当前谁走,走了几步,及位置. (因为 ...
- BZOJ.5248.[九省联考2018]一双木棋chess(对抗搜索 记忆化)
BZOJ 洛谷P4363 [Update] 19.2.9 重做了遍,感觉之前写的有点扯= = 首先棋子的放置情况是阶梯状的. 其次,无论已经放棋子的格子上哪些是黑棋子哪些是白棋子,之前得分如何,两人在 ...
- P2962 [USACO09NOV]灯Lights 对抗搜索
\(\color{#0066ff}{题目描述}\) 贝希和她的闺密们在她们的牛棚中玩游戏.但是天不从人愿,突然,牛棚的电源跳闸了,所有的灯都被关闭了.贝希是一个很胆小的女生,在伸手不见拇指的无尽的黑暗 ...
- 博弈论经典算法(一)——对抗搜索与Alpha-Beta剪枝
前言 在一些复杂的博弈论题目中,每一轮操作都可能有许多决策,于是就会形成一棵庞大的博弈树. 而有一些博弈论题没有什么规律,针对这样的问题,我们就需要用一些十分玄学的算法. 例如对抗搜索. 对抗搜索简介 ...
- 【BZOJ3106】[CQOI2013] 棋盘游戏(对抗搜索)
点此看题面 大致题意: 在一张\(n*n\)的棋盘上有一枚黑棋子和一枚白棋子.白棋子先移动,然后是黑棋子.白棋子每次可以向上下左右四个方向中任一方向移动一步,黑棋子每次则可以向上下左右四个方向中任一方 ...
- P4363 [九省联考2018]一双木棋chess(对抗搜索+记忆化搜索)
传送门 这对抗搜索是个啥玩意儿…… 首先可以发现每一行的棋子数都不小于下一行,且局面可由每一行的棋子数唯一表示,那么用一个m+1进制数来表示当前局面,用longlong存,开map记忆化搜索 然后时间 ...
- ccf 201803-4 棋局评估 (对抗搜索)
棋局评估 问题描述 Alice和Bob正在玩井字棋游戏. 井字棋游戏的规则很简单:两人轮流往3*3的棋盘中放棋子,Alice放的是“X”,Bob放的是“O”,Alice执先.当同一种棋子占据一行.一列 ...
- ICPC Asia Nanning 2017 I. Rake It In (DFS+贪心 或 对抗搜索+Alpha-Beta剪枝)
题目链接:Rake It In 比赛链接:ICPC Asia Nanning 2017 Description The designers have come up with a new simple ...
随机推荐
- C# http post、get请求
public string HttpPostJson(string Url, string paramData, Dictionary<string, string> headerDic ...
- 使用第三方《UITableView+FDTemplateLayoutCell》自动计算UITableViewCell高度(Masonry约束)
直接上代码: 1:先自定义cell .h文件中 #import <UIKit/UIKit.h> #import "LBDNewMsgListModel.h" #impo ...
- css3 笔记
1.元字符使用: []: 全部可选项 ||:并列 |:多选一 ?: 0个或者一个 *:0个或者多个 {}: 范围 2.CSS3属性选择器: E[attr]:存在attr属性即可: E[attr=val ...
- cc++面试------17道经典面试题目分析
以下是C/C++面试题目,共计17个题目,其中涵盖了c的各种基础语法和算法, 以函数接口设计和算法设计为主.这17个题目在C/C++面试方面已经流行了多 年,大家需要抽时间掌握好,每一个题目后面附有参 ...
- POJ 1066--Treasure Hunt(判断线段相交)
Treasure Hunt Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 7857 Accepted: 3247 Des ...
- 独木舟(51NOD 1432 )
n个人,已知每个人体重.独木舟承重固定,每只独木舟最多坐两个人,可以坐一个人或者两个人.显然要求总重量不超过独木舟承重,假设每个人体重也不超过独木舟承重,问最少需要几只独木舟? Input 第一行包含 ...
- oracle 11g grid软件安装[20180121]
实验环境: 系统->Redhat 6.5 Oracle软件版本->oracle 11.2.0.4.0 系统初始化 设定hosts主机名和对应IP地 ...
- 转:java中的定时任务
引自:http://www.cnblogs.com/wenbronk/p/6433178.html java中的定时任务, 使用java实现有3种方式: 1, 使用普通thread实现 @Test p ...
- 简易的vuex用法
vuex是vue中用于管理全局状态的一个组件,用于不同组件之间的通信,下面将介绍它的简单用法 首先安装vue与vuex npm install vue npm install vuex --save ...
- Manacher(马拉车)学习笔记
Manacher可以有效的在\(O(n)\)时间内解决一个字符串的回文子串的题目 目录 简介 讲解 推介 简单的练习 恐怖的练习QAQ 小结 简介 开头都说了,Manacher是目前解决回文子串的最有 ...