poj 1195:Mobile phones(二维树状数组,矩阵求和)
Time Limit: 5000MS | Memory Limit: 65536K | |
Total Submissions: 14489 | Accepted: 6735 |
Description
Write a program, which receives these reports and answers queries about the current total number of active mobile phones in any rectangle-shaped area.
Input
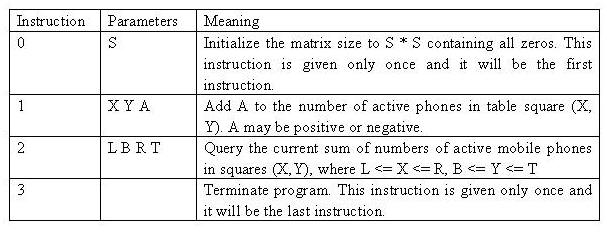
The values will always be in range, so there is no need to check them. In particular, if A is negative, it can be assumed that it will not reduce the square value below zero. The indexing starts at 0, e.g. for a table of size 4 * 4, we have 0 <= X <= 3 and 0 <= Y <= 3.
Table size: 1 * 1 <= S * S <= 1024 * 1024
Cell value V at any time: 0 <= V <= 32767
Update amount: -32768 <= A <= 32767
No of instructions in input: 3 <= U <= 60002
Maximum number of phones in the whole table: M= 2^30
Output
Sample Input
- 0 4
- 1 1 2 3
- 2 0 0 2 2
- 1 1 1 2
- 1 1 2 -1
- 2 1 1 2 3
- 3
Sample Output
- 3
- 4
Source

- void Add(int x,int y,int a)
- {
- int i=x;
- while(i<=s){
- int j=y;
- while(j<=s){
- c[i][j]+=a;
- j+=lowbit(j);
- }
- i+=lowbit(i);
- }
- }
2)求和:对左上角为(l,r),右下角为(b,t)的矩阵求和,即求该矩阵中所有元素的和。先求l到r的行矩阵的和,在求这个行矩阵和的时候,每一行要计算对应的b到t列的元素和。具体过程类似上述过程,将+lowbit()改为-lowbit()即可。限界为>=1。
代码:
- #include <iostream>
- #include <stdio.h>
- #include <string.h>
- using namespace std;
- #define MAXN 1100
- int c[MAXN][MAXN],s;
- int lowbit(int x)
- {
- return x&-x;
- }
- void Add(int x,int y,int a) //加数
- {
- int i=x;
- while(i<=s){ //行
- int j=y;
- while(j<=s){ //列
- c[i][j]+=a;
- j+=lowbit(j);
- }
- i+=lowbit(i);
- }
- }
- int Sum(int l,int r,int b,int t) //求和
- {
- l--,b--;
- int suml=,sumr=;
- //求行矩阵和,l以上矩阵
- while(l>=){
- int i=b,j=t;
- int sumb=,sumt=;
- //求列矩阵和
- while(i>=){
- sumb+=c[l][i];
- i-=lowbit(i);
- }
- while(j>=){
- sumt+=c[l][j];
- j-=lowbit(j);
- }
- suml+=sumt-sumb;
- l-=lowbit(l);
- }
- //求行矩阵和,r以上矩阵
- while(r>=){
- int i=b,j=t;
- int sumb=,sumt=;
- //求列矩阵和
- while(i>=){
- sumb+=c[r][i];
- i-=lowbit(i);
- }
- while(j>=){
- sumt+=c[r][j];
- j-=lowbit(j);
- }
- sumr+=sumt-sumb;
- r-=lowbit(r);
- }
- return sumr-suml;
- }
- int main()
- {
- int cmd,x,y,a,l,r,b,t;
- while(scanf("%d",&cmd)!=EOF){
- switch(cmd){
- case : //初始化矩阵
- scanf("%d",&s);
- memset(c,,sizeof(c));
- break;
- case : //加数
- scanf("%d%d%d",&x,&y,&a);
- Add(x+,y+,a);
- break;
- case : //求矩阵和
- scanf("%d%d%d%d",&l,&b,&r,&t);
- printf("%d\n",Sum(l+,r+,b+,t+));
- break;
- case : //退出程序
- return ;
- default:
- break;
- }
- }
- return ;
- }
Freecode : www.cnblogs.com/yym2013
poj 1195:Mobile phones(二维树状数组,矩阵求和)的更多相关文章
- poj 1195 Mobile phones(二维树状数组)
树状数组支持两种操作: Add(x, d)操作: 让a[x]增加d. Query(L,R): 计算 a[L]+a[L+1]……a[R]. 当要频繁的对数组元素进行修改,同时又要频繁的查询数组内任一 ...
- POJ 1195:Mobile phones 二维树状数组
Mobile phones Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 16893 Accepted: 7789 De ...
- 【poj1195】Mobile phones(二维树状数组)
题目链接:http://poj.org/problem?id=1195 [题意] 给出一个全0的矩阵,然后一些操作 0 S:初始化矩阵,维数是S*S,值全为0,这个操作只有最开始出现一次 1 X Y ...
- POJ 2155 Matrix【二维树状数组+YY(区间计数)】
题目链接:http://poj.org/problem?id=2155 Matrix Time Limit: 3000MS Memory Limit: 65536K Total Submissio ...
- POJ 2155 Matrix(二维树状数组+区间更新单点求和)
题意:给你一个n*n的全0矩阵,每次有两个操作: C x1 y1 x2 y2:将(x1,y1)到(x2,y2)的矩阵全部值求反 Q x y:求出(x,y)位置的值 树状数组标准是求单点更新区间求和,但 ...
- POJ 2155 Matrix (二维树状数组)
Matrix Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 17224 Accepted: 6460 Descripti ...
- POJ 2155 Matrix 【二维树状数组】(二维单点查询经典题)
<题目链接> 题目大意: 给出一个初始值全为0的矩阵,对其进行两个操作. 1.给出一个子矩阵的左上角和右上角坐标,这两个坐标所代表的矩阵内0变成1,1变成0. 2.查询某个坐标的点的值. ...
- POJ 2155 Matrix (二维树状数组)题解
思路: 没想到二维树状数组和一维的比只差了一行,update单点更新,query求和 这里的函数用法和平时不一样,query直接算出来就是某点的值,怎么做到的呢? 我们在更新的时候不止更新一个点,而是 ...
- POJ 2155:Matrix 二维树状数组
Matrix Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 21757 Accepted: 8141 Descripti ...
- POJ 2155 Matrix(二维树状数组)
与以往不同的是,这个树状数组是二维的,仅此而已 #include <iostream> #include <cstdio> #include <cstring> # ...
随机推荐
- django缓存
由于Django是动态网站,所有每次请求均会去数据进行相应的操作,当程序访问量大时,耗时必然会更加明显,最简单解决方式是使用:缓存,缓存将一个某个views的返回值保存至内存或者memcache中,5 ...
- Union-Find Algorithm
Union-Find Algrithm is used to check whether two components are connected or not. Examples: By using ...
- React 源码解读参考,理解原理。
Rubix - ReactJS Powered Admin Template 文档: http://rubix-docs.sketchpixy.com/ ===================== ...
- 【转】ArrayList其实就那么一回事儿之源码浅析
转自:http://www.cnblogs.com/dongying/p/4013271.html?utm_source=tuicool&utm_medium=referral ArrayLi ...
- Android SDK打包
2015年6月18日 14:38:49 星期四 eclipse: 1. 将写好的代码上传版本库 2. 删除 /bin/* 3. eclipse->project->clean... 4. ...
- PHP-FPM的常用操作
PHP-FPM安装完毕之后,没有自带的结束命令,可以通过以下方法结束: 1.首先查看PHP-FPM进程号: ps -ef | grep php-fpm 可以看到master进程号为91790,有两个子 ...
- 50道java算法题(一)
[程序1] 题目:古典问题:有一对兔子,从出生后第3个月起每个月都生一对兔子,小兔子长到第三个月后每个月又生一对兔子,假如兔子都不死,问每个月的兔子总数为多少? 1.程序分析: 兔子的规律为数列1 ...
- ACM/ICPC 之 Prim范例(ZOJ1586-POJ1789(ZOJ2158))
两道Prim解法范例题型,简单的裸Prim,且两题相较以边为重心的Kruskal解法而言更适合以点为重心扩展的Prim解法. ZOJ1586-QS Network 题意:见Code 题解:直接的MST ...
- Thread.Sleep in WinRT
Thread.Sleep in WinRT static void Sleep(int ms) { new System.Threading.ManualResetEvent(false).Wa ...
- WebLogic部署
1.抓取解压WAR包,放在相应目录下 2.登录部署,激活 http://jingyan.baidu.com/article/c74d6000650d470f6b595d72.html Linux环境中 ...