POJ训练计划2299_Ultra-QuickSort(线段树/单点更新)
解题报告
题意:
求逆序数。
思路:
线段树离散化处理。
#include <algorithm>
#include <iostream>
#include <cstring>
#include <cstdio>
#define LL long long
using namespace std;
LL sum[2001000],num[501000],_hash[501000];
void push_up(int rt)
{
sum[rt]=sum[rt*2]+sum[rt*2+1];
}
void update(int rt,int l,int r,int p,LL v)
{
if(l==r)
{
sum[rt]=+v;
return;
}
int mid=(l+r)/2;
if(p<=mid)update(rt*2,l,mid,p,v);
else update(rt*2+1,mid+1,r,p,v);
push_up(rt);
}
LL q_sum(int rt,int l,int r,int ql,int qr)
{
if(ql>r||qr<l)return 0;
if(ql<=l&&r<=qr)return sum[rt];
int mid=(l+r)/2;
return q_sum(rt*2,l,mid,ql,qr)+q_sum(rt*2+1,mid+1,r,ql,qr);
}
int main()
{
int n,i,j;
while(~scanf("%d",&n))
{
LL ans=0;
if(!n)break;
memset(_hash,0,sizeof(_hash));
memset(num,0,sizeof(num));
memset(sum,0,sizeof(sum));
for(i=0; i<n; i++)
{
scanf("%LLd",&num[i]);
_hash[i]=num[i];
}
sort(_hash,_hash+n);
int m=unique(_hash,_hash+n)-_hash;
for(i=0; i<n; i++)
{
int t=lower_bound(_hash,_hash+m,num[i])-_hash+1;
ans+=q_sum(1,1,m,t+1,m);
update(1,1,m,t,1);
}
printf("%lld\n",ans);
}
return 0;
}
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 41278 | Accepted: 14952 |
Description
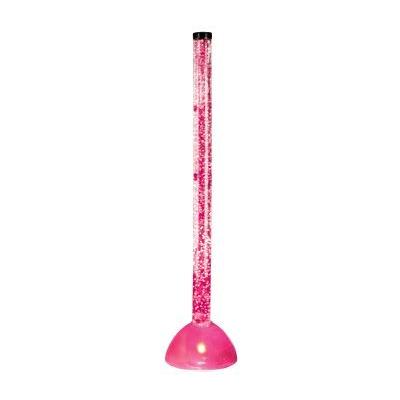
sorted in ascending order. For the input sequence
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
Sample Input
5
9
1
0
5
4
3
1
2
3
0
Sample Output
6
0
Source
POJ训练计划2299_Ultra-QuickSort(线段树/单点更新)的更多相关文章
- poj 2892---Tunnel Warfare(线段树单点更新、区间合并)
题目链接 Description During the War of Resistance Against Japan, tunnel warfare was carried out extensiv ...
- POJ 1804 Brainman(5种解法,好题,【暴力】,【归并排序】,【线段树单点更新】,【树状数组】,【平衡树】)
Brainman Time Limit: 1000MS Memory Limit: 30000K Total Submissions: 10575 Accepted: 5489 Descrip ...
- POJ.3321 Apple Tree ( DFS序 线段树 单点更新 区间求和)
POJ.3321 Apple Tree ( DFS序 线段树 单点更新 区间求和) 题意分析 卡卡屋前有一株苹果树,每年秋天,树上长了许多苹果.卡卡很喜欢苹果.树上有N个节点,卡卡给他们编号1到N,根 ...
- POJ.2299 Ultra-QuickSort (线段树 单点更新 区间求和 逆序对 离散化)
POJ.2299 Ultra-QuickSort (线段树 单点更新 区间求和 逆序对 离散化) 题意分析 前置技能 线段树求逆序对 离散化 线段树求逆序对已经说过了,具体方法请看这里 离散化 有些数 ...
- HDU 1754 I Hate It 线段树单点更新求最大值
题目链接 线段树入门题,线段树单点更新求最大值问题. #include <iostream> #include <cstdio> #include <cmath> ...
- HDU 1166 敌兵布阵(线段树单点更新)
敌兵布阵 单点更新和区间更新还是有一些区别的,应该注意! [题目链接]敌兵布阵 [题目类型]线段树单点更新 &题意: 第一行一个整数T,表示有T组数据. 每组数据第一行一个正整数N(N< ...
- HDU 1166 敌兵布阵(线段树单点更新,板子题)
敌兵布阵 Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others) Total Submi ...
- HDU 1166 敌兵布阵(线段树单点更新,区间查询)
描述 C国的死对头A国这段时间正在进行军事演习,所以C国间谍头子Derek和他手下Tidy又开始忙乎了.A国在海岸线沿直线布置了N个工兵营地,Derek和Tidy的任务就是要监视这些工兵营地的活动情况 ...
- HDUOJ----1166敌兵布阵(线段树单点更新)
敌兵布阵 Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submis ...
- HDU.1166 敌兵布阵 (线段树 单点更新 区间查询)
HDU.1166 敌兵布阵 (线段树 单点更新 区间查询) 题意分析 加深理解,重写一遍 代码总览 #include <bits/stdc++.h> #define nmax 100000 ...
随机推荐
- Spring框架针对dao层的jdbcTemplate操作crud之update修改数据库操作
使用jdbcTemplate 原理是把加载驱动Class.forName("com.mysql.jdbc.Driver"); 和连接数据库Connection conn=Drive ...
- BZOJ 1968_P1403 [AHOI2005]约数研究--p2260bzoj2956-模积和∑----信息学中的数论分块
第一部分 P1403 [AHOI2005]约数研究 题目描述 科学家们在Samuel星球上的探险得到了丰富的能源储备,这使得空间站中大型计算机“Samuel II”的长时间运算成为了可能.由于在去年一 ...
- ixcache的蜜汁突发故障
公元2018年 7月29日 晚上21点整,我司一直正常运行的ixcache线路异常断开. ??? 公司业务包含提供互联网接入服务,所以这个现象将会导致用户上网体验变差,网速变慢,看视频的速度下降等等, ...
- 专访Vue作者尤雨溪:Vue CLI 3.0重构的原因
1.为什么要对 Vue CLI 进行大规模修改? 尤雨溪认为旧版本的 Vue CLI 本质上只是从 GitHub 拉取模版,这种拉模版的方式有几个问题: (1) 在单个模版里面同时支持太多选项会导致模 ...
- 条款14:在资源管理类中心copying行为(Think carefully about copying behavior in resource-manage classes)
NOTE: 1.复制RAII 对象必须一并赋值它所管理的资源,所以资源的copying行为决定RAII对象的copying行为. 2.普遍而常见的RAII class copying 行为是: 抑制c ...
- Couchbase第一印象(架构特性)
Couchbase第一印象(架构特性) 面向文档 保存的字节流总有一个 DOCUMENT ID(Object_ID) 高并发性,高灵活性,高拓展性,容错性好 面向文档的集群存储系统 每个文档用一个唯一 ...
- Git上传的使用步骤
Git上传的使用步骤 首先 git branch 查看当前的分支是否为本地自己分支 接着 git stash 保存本地自己的保存 git checkout earemote 查看本地共有开发分支 gi ...
- 『NYIST』第九届河南省ACM竞赛队伍选拔赛[正式赛二]--Codeforces -35D. Animals
D. Animals time limit per test 2 seconds memory limit per test 64 megabytes input input.txt output o ...
- [HDU4417]Super Mario(主席树+离散化)
传送门 又是一道主席树模板题,注意数组从0开始,还有主席树耗费空间很大,数组开大点,之前开小了莫名其妙TLE.QAQ ——代码 #include <cstdio> #include < ...
- 转载:K-means聚类算法
转载地址:http://www.cnblogs.com/jerrylead/archive/2011/04/06/2006910.html K-means也是聚类算法中最简单的一种了,但是里面包含的思 ...