POJ1330(LCA入门题)
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 23388 | Accepted: 12195 |
Description
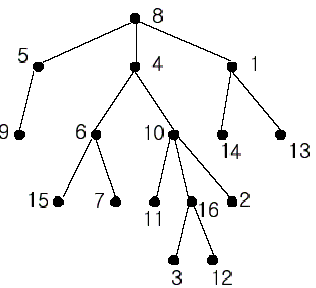
For other examples, the nearest common ancestor of nodes 2 and 3 is node 10, the nearest common ancestor of nodes 6 and 13 is node 8, and the nearest common ancestor of nodes 4 and 12 is node 4. In the last example, if y is an ancestor of z, then the nearest common ancestor of y and z is y.
Write a program that finds the nearest common ancestor of two distinct nodes in a tree.
Input
Output
Sample Input
2
16
1 14
8 5
10 16
5 9
4 6
8 4
4 10
1 13
6 15
10 11
6 7
10 2
16 3
8 1
16 12
16 7
5
2 3
3 4
3 1
1 5
3 5
Sample Output
4
3
#include"cstdio"
#include"cstring"
using namespace std;
const int MAXN=;
struct Edge{
int to,next;
}es[MAXN];
int V,E;
int head[MAXN];
int root;
void add_edge(int u,int v)
{
es[E].to=v;
es[E].next=head[u];
head[u]=E++;
}
int par[MAXN];
void prep()
{
for(int i=;i<MAXN;i++)
{
par[i]=i;
}
}
int fnd(int x)
{
if(par[x]==x)
{
return x;
}
return par[x]=fnd(par[x]);
}
void unite(int x,int y)
{
par[y]=fnd(x);
}
int dep[MAXN];
int fa[MAXN];
void dfs(int u,int f,int d)
{
dep[u]=d;
fa[u]=f;
for(int i=head[u];i!=-;i=es[i].next)
{
int to=es[i].to;
if(f!=to) dfs(to,u,d+);
}
}
int LCA(int u,int v)
{
while(dep[u]>dep[v]) u=fa[u];
while(dep[v]>dep[u]) v=fa[v];
while(u!=v)
{
u=fa[u];
v=fa[v];
}
return u;
}
int main()
{
int T;
scanf("%d",&T);
while(T--)
{
prep();
memset(head,-,sizeof(head));
scanf("%d",&V);
E=;
for(int i=;i<=V-;i++)
{
int u,v;
scanf("%d%d",&u,&v);
add_edge(u,v);
unite(u,v);
}
root=fnd();
dfs(root,-,);
int x,y;
scanf("%d%d",&x,&y);
int lca=LCA(x,y);
printf("%d\n",lca);
}
return ;
}
POJ1330(LCA入门题)的更多相关文章
- LCA入门题集小结
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=2586 题目: How far away ? Time Limit: 2000/1000 MS (Jav ...
- poj 1330(RMQ&LCA入门题)
传送门:Problem 1330 https://www.cnblogs.com/violet-acmer/p/9686774.html 参考资料: http://dongxicheng.org/st ...
- lca入门———树上倍增法(博文内含例题)
倍增求LCA: father[i][j]表示节点i往上跳2^j次后的节点 可以转移为 father[i][j]=father[father[i][j-1]][j-1] 整体思路: 先比较两个点的深度, ...
- hdu 3695:Computer Virus on Planet Pandora(AC自动机,入门题)
Computer Virus on Planet Pandora Time Limit: 6000/2000 MS (Java/Others) Memory Limit: 256000/1280 ...
- poj 2524:Ubiquitous Religions(并查集,入门题)
Ubiquitous Religions Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 23997 Accepted: ...
- poj 3984:迷宫问题(广搜,入门题)
迷宫问题 Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 7635 Accepted: 4474 Description ...
- hdu 1754:I Hate It(线段树,入门题,RMQ问题)
I Hate It Time Limit: 9000/3000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total S ...
- poj 3254 状压dp入门题
1.poj 3254 Corn Fields 状态压缩dp入门题 2.总结:二进制实在巧妙,以前从来没想过可以这样用. 题意:n行m列,1表示肥沃,0表示贫瘠,把牛放在肥沃处,要求所有牛不能相 ...
- zstu.4194: 字符串匹配(kmp入门题&& 心得)
4194: 字符串匹配 Time Limit: 1 Sec Memory Limit: 128 MB Submit: 206 Solved: 78 Description 给你两个字符串A,B,请 ...
随机推荐
- Zend API:深入 PHP 内核
Introduction Those who know don't talk. Those who talk don't know. Sometimes, PHP "as is" ...
- C#中方法中 ref 和 out的使用
案例1: static void Main() { , , , }; int numLargerThan10,numLargerThan100,numLargerThan1000 ; Proc(ary ...
- Android:实现两个Activity相互切换而都不走onCreate()
本文要实现的目的是: 有3个Activity: A,B,C.从A中能够进入B,B中能够进入C.而且B和C之间可能须要多次相互切换,因此不能使用普通的startActivity-finish方式,由于又 ...
- mongo 介绍
[介绍]:MongoDB 是由C++语言编写的,是一个基于分布式文件存储的开源数据库系统.在高负载的情况下,添加更多的节点,可以保证服务器性能.MongoDB 旨在为WEB应用提供可扩展的高性能数据存 ...
- go test 上篇
前言 Go语言本身集成了轻量级的测试框架,由go test命令和testing包组成.包含单元测试和压力测试,是保证我们编写健壮Golang程序的有效工具. 演示环境 $ uname -a Darwi ...
- 【BZOJ1604】[Usaco2008 Open]Cow Neighborhoods 奶牛的邻居 Treap+并查集
[BZOJ1604][Usaco2008 Open]Cow Neighborhoods 奶牛的邻居 Description 了解奶牛们的人都知道,奶牛喜欢成群结队.观察约翰的N(1≤N≤100000) ...
- NHibernate学习系列一
NHibernate是一个面向.NET环境的对象/关系数据库映射工具.对象/关系数据库映射(object/relational mapping,ORM)这个术语表示一种技术,用来把对象模型表示的对象映 ...
- vue-cli与后台数据交互增删改查
1. 安装vue-resource npm install vue-resource --save 2.访问后台地址,在vue中会出现跨域的问题,以下为解决方案 在config下的index.js 中 ...
- SpringMVC @ResponseBody和@RequestBody使用
@ResponseBody用法 作用: 该注解用于将Controller的方法返回的对象,根据HTTP Request Header的Accept的内容,通过适当的HttpMessageConvert ...
- 关于spring的bean
1 spring bean的单例和多例 singleton 单例指的是,在需要该bean的地方,spring framework返回的是同一个值. prototype 多例指的是,在需要该bean的地 ...