POJ2195 Going Home (最小费最大流||二分图最大权匹配) 2017-02-12 12:14 131人阅读 评论(0) 收藏
Description
a house. The task is complicated with the restriction that each house can accommodate only one little man.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates
there is a little man on that point.
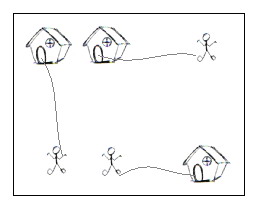
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Input
N and M are between 2 and 100, inclusive. There will be the same number of 'H's and 'm's on the map; and there will be at most 100 houses. Input will terminate with 0 0 for N and M.
Output
Sample Input
2 2
.m
H.
5 5
HH..m
.....
.....
.....
mm..H
7 8
...H....
...H....
...H....
mmmHmmmm
...H....
...H....
...H....
0 0
Sample Output
2
10
28
#include <iostream>
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <cmath>
#include <string>
#include <queue>
#include <vector>
#include <stack>
#include <set>
#include <map>
using namespace std;
const int MAXN=10000;
const int MAXM=100000;
const int INF=0x3f3f3f3f;
struct Edge{
int to,next,cap,flow,cost;
} edge[MAXM];
int head[MAXN],tol;
int pre[MAXN],dis[MAXN];
bool vis[MAXN];
int N;
struct point{
int x,y;
};
void init(int n)
{
N=n;
tol=0;
memset(head,-1,sizeof head);
}
void addedge(int u,int v,int cap,int cost)
{
edge[tol].to=v;
edge[tol].cap=cap;
edge[tol].flow=0;
edge[tol].cost=cost;
edge[tol].next=head[u];
head[u]=tol++;
edge[tol].to=u;
edge[tol].cap=0;
edge[tol].flow=0;
edge[tol].cost=-cost;
edge[tol].next=head[v];
head[v]=tol++;
} bool spfa(int s,int t)
{
queue<int>q;
for(int i=0;i<N;i++)
{
dis[i]=INF;
vis[i]=false;
pre[i]=-1;
}
dis[s]=0;
vis[s]=true;
q.push(s);
while(!q.empty())
{
int u=q.front();
q.pop();
vis[u]=false;
for(int i=head[u];i!=-1;i=edge[i].next)
{
int v=edge[i].to;
if(edge[i].cap>edge[i].flow&&dis[v]>dis[u]+edge[i].cost)
{
dis[v]=dis[u]+edge[i].cost;
pre[v]=i;
if(!vis[v])
{
vis[v]=true;
q.push(v);
}
}
}
}
if(pre[t]==-1)return false;
return true;
}
int MincostMaxflow(int s,int t)
{
int flow=0;
int cost=0;
while(spfa(s,t))
{
int Min=INF;
for(int i=pre[t];i!=-1;i=pre[edge[i^1].to])
{
if(Min>edge[i].cap-edge[i].flow)
Min=edge[i].cap-edge[i].flow;
}
for(int i=pre[t];i!=-1;i=pre[edge[i^1].to])
{
edge[i].flow+=Min;
edge[i^1].flow-=Min;
cost+=edge[i].cost*Min;
}
flow+=Min;
}
return cost;
} int main()
{
char mp[105][105];
int m,n;
while(~scanf("%d%d",&n,&m)&&(m||n))
{
point H[105],P[105];
int h=0,p=0;
for(int i=0;i<n;i++)
{
scanf("%s",&mp[i]);
for(int j=0;j<m;j++)
{
if(mp[i][j]=='H')
{
H[h].x=i;
H[h].y=j;
h++;
}
else if(mp[i][j]=='m')
{
P[p].x=i;
P[p].y=j;
p++;
}
}
}
init(p+h+2);
for(int i=0;i<h;i++)
for(int j=0;j<p;j++)
{
int c=fabs(H[i].x-P[j].x)+fabs(H[i].y-P[j].y);
addedge(i+1,h+j+1,1,c);
} for(int i=0;i<h;i++)
{
addedge(0,i+1,1,0);
}
for(int i=0;i<p;i++)
{
addedge(h+1+i,h+p+1,1,0);
}
printf("%d\n",MincostMaxflow(0,h+p+1)); }
}
方法二:
#include <iostream>
#include <cstdio>
#include <string>
#include <cstring>
#include <cmath>
#include <algorithm>
#include <queue>
#include <vector>
#include <set>
#include <stack>
#include <map>
#include <climits>
using namespace std; #define LL long long
const int INF=0x3f3f3f3f;
const int MAXN = 505;
int g[MAXN][MAXN];
int lx[MAXN],ly[MAXN]; //顶标
int linky[MAXN];
int visx[MAXN],visy[MAXN];
int slack[MAXN];
char mp[MAXN][MAXN];
int nx,ny;
bool find(int x)
{
visx[x] = true;
for(int y = 0; y < ny; y++)
{
if(visy[y])
continue;
int t = lx[x] + ly[y] - g[x][y];
if(t==0)
{
visy[y] = true;
if(linky[y]==-1 || find(linky[y]))
{
linky[y] = x;
return true; //找到增广轨
}
}
else if(slack[y] > t)
slack[y] = t;
}
return false; //没有找到增广轨(说明顶点x没有对应的匹配,与完备匹配(相等子图的完备匹配)不符)
} int KM() //返回最优匹配的值
{
int i,j;
memset(linky,-1,sizeof(linky));
memset(ly,0,sizeof(ly));
for(i = 0; i < nx; i++)
for(j = 0,lx[i] = -INF; j < ny; j++)
lx[i] = max(lx[i],g[i][j]);
for(int x = 0; x < nx; x++)
{
for(i = 0; i < ny; i++)
slack[i] = INF;
while(true)
{
memset(visx,0,sizeof(visx));
memset(visy,0,sizeof(visy));
if(find(x)) //找到增广轨,退出
break;
int d = INF;
for(i = 0; i < ny; i++) //没找到,对l做调整(这会增加相等子图的边),重新找
{
if(!visy[i] && d > slack[i])
d = slack[i];
}
for(i = 0; i < nx; i++)
{
if(visx[i])
lx[i] -= d;
}
for(i = 0; i < ny; i++)
{
if(visy[i])
ly[i] += d;
else
slack[i] -= d;
}
}
}
int result = 0;
for(i = 0; i < ny; i++)
if(linky[i]>-1)
result += g[linky[i]][i];
return result;
} int main()
{
int n,m;
while(~scanf("%d%d",&n,&m)&&(n||m))
{
for(int i=0; i<n; i++)
{
scanf("%s",mp[i]);
}
int cnt=0;
int CNT=0;
memset(g,-INF,sizeof g);
for(int i=0; i<n; i++)
for(int j=0; j<m; j++)
{
if(mp[i][j]=='m')
{
int CNT=0;
for(int I=0; I<n; I++)
for(int J=0; J<m; J++)
{
if(mp[I][J]=='H')
{
g[cnt][CNT++]=-(abs(i-I)+abs(j-J));
}
}
cnt++;
} }
nx=ny=cnt;
printf("%d\n",-KM());
}
return 0;
}
POJ2195 Going Home (最小费最大流||二分图最大权匹配) 2017-02-12 12:14 131人阅读 评论(0) 收藏的更多相关文章
- c++ 字符串流 sstream(常用于格式转换) 分类: C/C++ 2014-11-08 17:20 150人阅读 评论(0) 收藏
使用stringstream对象简化类型转换 C++标准库中的<sstream>提供了比ANSI C的<stdio.h>更高级的一些功能,即单纯性.类型安全和可扩展性.在本文中 ...
- HDU1045 Fire Net(DFS枚举||二分图匹配) 2016-07-24 13:23 99人阅读 评论(0) 收藏
Fire Net Problem Description Suppose that we have a square city with straight streets. A map of a ci ...
- POJ1273&&Hdu1532 Drainage Ditches(最大流dinic) 2017-02-11 16:28 54人阅读 评论(0) 收藏
Drainage Ditches Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others) ...
- 二分图匹配(KM算法)n^4 分类: ACM TYPE 2014-10-04 11:36 88人阅读 评论(0) 收藏
#include <iostream> #include<cstring> #include<cstdio> #include<cmath> #incl ...
- 二分图匹配(KM算法)n^3 分类: ACM TYPE 2014-10-01 21:46 98人阅读 评论(0) 收藏
#include <iostream> #include<cstring> #include<cstdio> #include<cmath> const ...
- 二分图匹配 分类: ACM TYPE 2014-10-01 19:57 94人阅读 评论(0) 收藏
#include<cstdio> #include<cstring> using namespace std; bool map[505][505]; int n, k; bo ...
- POJ2195 Going Home[费用流|二分图最大权匹配]
Going Home Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 22088 Accepted: 11155 Desc ...
- 【BZOJ 3308】 3308: 九月的咖啡店 (费用流|二分图最大权匹配)
3308: 九月的咖啡店 Time Limit: 30 Sec Memory Limit: 128 MBSubmit: 244 Solved: 86 Description 深绘里在九份开了一家咖 ...
- [hdu1533]二分图最大权匹配 || 最小费用最大流
题意:给一个n*m的地图,'m'表示人,'H'表示房子,求所有人都回到房子所走的距离之和的最小值(距离为曼哈顿距离). 思路:比较明显的二分图最大权匹配模型,将每个人向房子连一条边,边权为曼哈顿距离的 ...
随机推荐
- SQL Server中的事务与其隔离级别之脏读, 未提交读,不可重复读和幻读
原本打算写有关 SSIS Package 中的事务控制过程的,但是发现很多基本的概念还是需要有 SQL Server 事务和事务的隔离级别做基础铺垫.所以花了点时间,把 SQL Server 数据库中 ...
- httpd编译安装
Apache安装问题:configure: error: APR not found . Please read the documentation: Linux上安装Apache时,编译出现错误: ...
- 解决移动端H5海报滑动插件适应大部分手机问题 手机端高度自适应
Html5微信端滑屏海报在各种尺寸的手机上总会有这样那样的问题,经过多次制作总结出来一些小心得,分享下. 我使用的是jquery插件swiper.min.js,动画可以利用animate.css,如果 ...
- 字符串作为freemarker模板的简单实现例子
本文转载自:http://blog.csdn.net/5iasp/article/details/27181365 package com.test.demo; import java.io.IOEx ...
- Java-Runoob-高级教程-实例-环境设置实例:1.Java 实例 – 如何编译一个Java 文件?
ylbtech-Java-Runoob-高级教程-实例-环境设置实例:1.Java 实例 – 如何编译一个Java 文件? 1.返回顶部 1. Java 实例 - 如何编译 Java 文件 Java ...
- Bootstrap-CL:进度条
ylbtech-Bootstrap-CL:进度条 1.返回顶部 1. Bootstrap 进度条 本章将讲解 Bootstrap 进度条.在本教程中,您将看到如何使用 Bootstrap 创建加载.重 ...
- [转]Web前端浏览器兼容
转自: http://www.admin10000.com/document/1900.html 前言 浏览器兼容是前端开发人员必须掌握的一个技能,但是初入前端的同学或者其他后台web开发同学往往容易 ...
- Educational Codeforces Round 37-G.List Of Integers题解
一.题目 二.题目链接 http://codeforces.com/contest/920/problem/G 三.题意 给定一个$t$,表示有t次查询.每次查询给定一个$x$, $p$, $k$,需 ...
- python学习-day 2
1.执行Python脚本的两种方式1)调用解释器 Python +绝对路径+文件名称2)调用解释器 Python +相对路径+文件名称 2.简述位.字节的关系8位为1个字节 3.简述ASCII.uni ...
- Java——复制txt文件
将源文件复制到目标文件,同时输出源文件内容,需要提供一个源文件和目标文件路径参数(如果不存在则自动创建) public static void copyTxt(String sourceFile, S ...