[iOS基础控件 - 5.4] 广告分页代码(UIScrollView制作)
// 总页数
@property(nonatomic) NSInteger numberOfPages; // default is 0
// 当前页码
@property(nonatomic) NSInteger currentPage;
// 只有一页的时候隐藏页码
@property(nonatomic) BOOL hidesForSinglePage; // hide the the indicator if there is only one page. default is NO
// 其他页码指示颜色
@property(nonatomic,retain) UIColor *pageIndicatorTintColor;
// 当前页码指示颜色
@property(nonatomic,retain) UIColor *currentPageIndicatorTintColor;
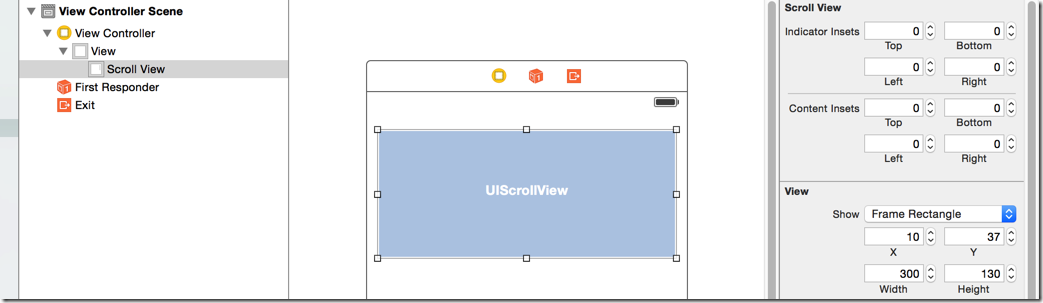
CGFloat imageWidth = ;
CGFloat imageHeight = ;
CGFloat imageY = ; for (int i=; i<; i++) {
CGFloat imageX = i * imageWidth;
UIImageView *currentImageView = [[UIImageView alloc] initWithImage:[UIImage imageNamed:[NSString stringWithFormat:@"img_%02d", i+]]];
currentImageView.frame = CGRectMake(imageX, imageY, imageWidth, imageHeight); [self.scrollView addSubview:currentImageView];
}
// 设置可以拖曳的范围,只允许横向拖曳,而且范围是所有的图片宽度总和
self.scrollView.contentSize = CGSizeMake( * imageWidth, );
// 开启翻页模式,每次拖曳都会滑动一个UIScrollView宽度单位
self.scrollView.pagingEnabled = YES;
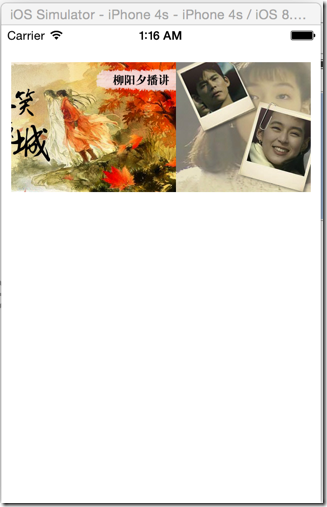
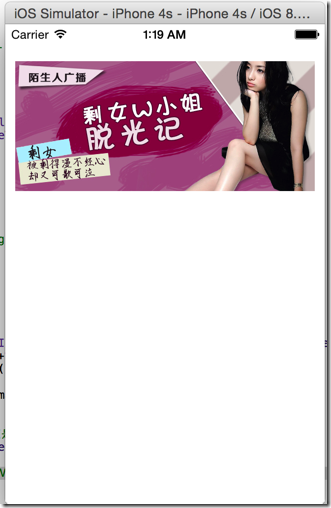

// 总页数
@property(nonatomic) NSInteger numberOfPages; // default is 0
// 当前页码
@property(nonatomic) NSInteger currentPage;
// 只有一页的时候隐藏页码
@property(nonatomic) BOOL hidesForSinglePage; // hide the the indicator if there is only one page. default is NO
// 其他页码指示颜色
@property(nonatomic,retain) UIColor *pageIndicatorTintColor;
// 当前页码指示颜色
@property(nonatomic,retain) UIColor *currentPageIndicatorTintColor;
// 设置pageControl
self.pageControl.numberOfPages = ;
self.pageControl.pageIndicatorTintColor = [UIColor blackColor]; //设置delegate
self.scrollView.delegate = self;
/** 当scrollView滚动的时候调用 */
- (void)scrollViewDidScroll:(UIScrollView *)scrollView {
CGFloat scrollWidth = scrollView.frame.size.width; // 当一副图片拖曳超过一半的时候就重新计算页码
int page = (scrollView.contentOffset.x + scrollWidth * 0.5) / scrollWidth; self.pageControl.currentPage = page;
}
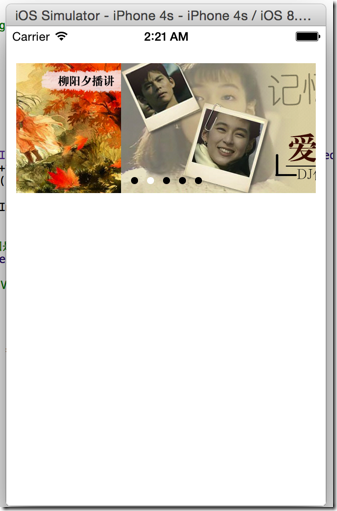
// 创建定时器
NSTimer *timer = [NSTimer scheduledTimerWithTimeInterval: target:self selector:@selector(nextImage) userInfo:nil repeats:YES];
- (void) nextImage {
// 1.增加pageControl的页码,这里不能直接操作currentPage,因为这样会和scrollViewDidScroll的页码计算冲突,应该是滚动画面,触发scrollViewDidScroll进行页码转换
int pageNo = ;
if (self.pageControl.currentPage == (IMAGE_COUNT - )) {
pageNo = ;
}
else {
pageNo = self.pageControl.currentPage + ;
} // 2.计算scrollView的滑动位置
CGFloat offsetX = pageNo * self.scrollView.frame.size.width;
CGPoint offset = CGPointMake(offsetX, ); // 移动一页,带动画效果
[self.scrollView setContentOffset:offset animated:YES];
}
// 开始手动拖曳的时候,销毁定时器
- (void)scrollViewWillBeginDragging:(UIScrollView *)scrollView {
[self.timer invalidate]; // 调用了invalidate之后再不可用了
self.timer = nil;
} // 结束手动拖曳,重新定义定时器
- (void)scrollViewDidEndDragging:(UIScrollView *)scrollView willDecelerate:(BOOL)decelerate {
[self addTimer];
} // 添加定时器
- (void) addTimer {
self.timer = [NSTimer scheduledTimerWithTimeInterval: target:self selector:@selector(nextImage) userInfo:nil repeats:YES];
}

// 添加定时器
- (void) addTimer {
self.timer = [NSTimer scheduledTimerWithTimeInterval: target:self selector:@selector(nextImage) userInfo:nil repeats:YES]; // 获得主线程资源,防止另外的如可滚动控件的资源全占用
[[NSRunLoop currentRunLoop] addTimer:self.timer forMode:NSRunLoopCommonModes];
}
//
// ViewController.m
// ScrollViewPage
//
// Created by hellovoidworld on 14/11/28.
// Copyright (c) 2014年 hellovoidworld. All rights reserved.
// #import "ViewController.h" #define IMAGE_COUNT 5 @interface ViewController () <UIScrollViewDelegate>
@property (weak, nonatomic) IBOutlet UIScrollView *scrollView; // 滚动控件
@property (weak, nonatomic) IBOutlet UIPageControl *pageControl; // 页码控件 @property(nonatomic, strong) NSTimer *timer; // 定时器 @end @implementation ViewController - (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib. CGFloat imageWidth = ;
CGFloat imageHeight = ;
CGFloat imageY = ; for (int i=; i<IMAGE_COUNT; i++) {
CGFloat imageX = i * imageWidth;
UIImageView *currentImageView = [[UIImageView alloc] initWithImage:[UIImage imageNamed:[NSString stringWithFormat:@"img_%02d", i+]]];
currentImageView.frame = CGRectMake(imageX, imageY, imageWidth, imageHeight); [self.scrollView addSubview:currentImageView];
} // 设置可以拖曳的范围,只允许横向拖曳,而且范围是所有的图片宽度总和
self.scrollView.contentSize = CGSizeMake(IMAGE_COUNT * imageWidth, ); // 开启翻页模式,每次拖曳都会滑动一个UIScrollView宽度单位
self.scrollView.pagingEnabled = YES; // 设置pageControl
self.pageControl.numberOfPages = ;
self.pageControl.pageIndicatorTintColor = [UIColor blackColor];
self.pageControl.currentPageIndicatorTintColor = [UIColor redColor]; // 设置delegate
self.scrollView.delegate = self; // 创建定时器
[self addTimer];
} - (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
} /** 当scrollView滚动的时候调用 */
- (void)scrollViewDidScroll:(UIScrollView *)scrollView {
CGFloat scrollWidth = scrollView.frame.size.width; // 当一副图片拖曳超过一半的时候就重新计算页码
int page = (scrollView.contentOffset.x + scrollWidth * 0.5) / scrollWidth; self.pageControl.currentPage = page;
} - (void) nextImage {
// 1.增加pageControl的页码,这里不能直接操作currentPage,因为这样会和scrollViewDidScroll的页码计算冲突,应该是滚动画面,触发scrollViewDidScroll进行页码转换
int pageNo = ;
if (self.pageControl.currentPage == (IMAGE_COUNT - )) {
pageNo = ;
}
else {
pageNo = self.pageControl.currentPage + ;
} // 2.计算scrollView的滑动位置
CGFloat offsetX = pageNo * self.scrollView.frame.size.width;
CGPoint offset = CGPointMake(offsetX, ); // 移动一页,带动画效果
[self.scrollView setContentOffset:offset animated:YES];
} // 开始手动拖曳的时候,销毁定时器
- (void)scrollViewWillBeginDragging:(UIScrollView *)scrollView {
[self.timer invalidate]; // 调用了invalidate之后再不可用了
self.timer = nil;
} // 结束手动拖曳,重新定义定时器
- (void)scrollViewDidEndDragging:(UIScrollView *)scrollView willDecelerate:(BOOL)decelerate {
[self addTimer];
} // 添加定时器
- (void) addTimer {
self.timer = [NSTimer scheduledTimerWithTimeInterval: target:self selector:@selector(nextImage) userInfo:nil repeats:YES]; // 获得主线程资源,防止另外的如可滚动控件的资源全占用
[[NSRunLoop currentRunLoop] addTimer:self.timer forMode:NSRunLoopCommonModes];
} @end
[iOS基础控件 - 5.4] 广告分页代码(UIScrollView制作)的更多相关文章
- [iOS基础控件 - 5.5] 代理设计模式 (基于”APP列表"练习)
A.概述 在"[iOS基础控件 - 4.4] APP列表 进一步封装,初见MVC模式”上进一步改进,给“下载”按钮加上效果.功能 1.按钮点击后,显示为“已下载”,并且不 ...
- [iOS基础控件 - 6.6.1] 展示团购数据代码
1.主控制器: // // ViewController.m // GroupPurchase // // Created by hellovoidworld on 14/12/3. // Cop ...
- iOS 基础控件(下)
上篇介绍了UIButton.UILabel.UIImageView和UITextField,这篇就简短一点介绍UIScrollView和UIAlertView. UIScrollView 顾名思义也知 ...
- [iOS基础控件 - 7.0] UIWebView
A.基本使用 1.概念 iOS内置的浏览器控件 Safari浏览器就是通过UIWebView实现的 2.用途:制作简易浏览器 (1)基本请求 创建请求 加载请求 (2)代理监听webView加载, ...
- [iOS基础控件 - 6.11.3] 私人通讯录Demo 控制器的数据传递、存储
A.需求 1.搭建一个"私人通讯录"Demo 2.模拟登陆界面 账号 密码 记住密码开关 自动登陆开关 登陆按钮 3.退出注销 4.增删改查 5.恢复数据(取消修改) 这个代码 ...
- [iOS基础控件 - 6.10.2] PickerView 自定义row内容 国家选择Demo
A.需求 1.自定义一个UIView和xib,包含国家名和国旗显示 2.学习row的重用 B.实现步骤 1.准备plist文件和国旗图片 2.创建模型 // // Flag.h // Co ...
- [iOS基础控件 - 6.9] 聊天界面Demo
A.需求 做出一个类似于QQ.微信的聊天界面 1.每个cell包含发送时间.发送人(头像).发送信息 2.使用对方头像放在左边,我方头像在右边 3.对方信息使用白色背景对话框,我方信息使用蓝色背景对话 ...
- [iOS基础控件 - 6.7] 微博展示 使用代码自定义TableCell(动态尺寸)
A.需求 1.类似于微博内容的展示 2.头像 3.名字 4.会员标志 5.内容 6.分割线 7.配图(可选,可有可无) code source: https://github.com/hellov ...
- [iOS基础控件 - 6.6] 展示团购数据 自定义TableViewCell
A.需求 1.头部广告 2.自定义cell:含有图片.名称.购买数量.价格 3.使用xib设计自定义cell,自定义cell继承自UITableViewCell 4.尾部“加载更多按钮”,以及其被点击 ...
随机推荐
- linux 使用kill命令杀死进程的几个办法
常规篇: 首先,用ps查看进程,方法如下: $ ps -ef ……smx 1822 1 0 11:38 ? 00:00:49 gnome-terminalsmx ...
- jstack和线程dump分析
转自:http://jameswxx.iteye.com/blog/1041173 一:jstack jstack命令的语法格式: jstack <pid>.可以用jps查看java进程 ...
- DOM4J介绍与代码示例【转载】
DOM4J是dom4j.org出品的一个开源XML解析包.Dom4j是一个易用的.开源的库,用于XML,XPath和XSLT.它应用于Java平台,采用了Java集合框架并完全支持DOM,SAX和JA ...
- delphi使用 第三方控件
第三方控件安装时必须把所有的pas,dcu,dpk,res等文件复制到你的Lib目录下 然后通过dpk进行安装 安装后会多出来新的控件面板,新控件就在那里了 当然也有一些控件会安装到原有的面板上 比如 ...
- Angularjs中input的指令和属性
建议添加 novalidate属性(可无值)到form标签上,这样可以保证在表单不合法的情况下阻止浏览器继续提交数据. 可以给表单元素 input 添加 required 属性(可无值),让该表单成为 ...
- Angularjs checkbox的ng属性
angularjs 默认给 input[checkbox] 元素定制了一些属性,如: <input type="checkbox" ng-mudel="name&q ...
- BZOJ2870: 最长道路tree
题解: 子树分治的做法可以戳这里:http://blog.csdn.net/iamzky/article/details/41120733 可是码量... 这里介绍另一种好写又快的方法. 我们还是一颗 ...
- I.MX6 android BatteryService jni hacking
/**************************************************************************** * I.MX6 android Batter ...
- RPi 2B 自动发送获取的IP到固定邮箱
/************************************************************************* * RPi 2B 自动发送获取的IP到固定邮箱 * ...
- 陈正冲老师讲c语言之声明和定义的区别
什么是定义?什么是声明?它们有何区别? 举个例子: A)int i; B)extern int i;(关于extern,后面解释) 哪个是定义?哪个是声明?或者都是定义或者都是声明?我所教过的学生几乎 ...