POJ 2549 Sumsets
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 10593 | Accepted: 2890 |
Description
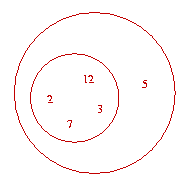
Input
Output
Sample Input
5
2
3
5
7
12
5
2
16
64
256
1024
0
Sample Output
12
no solution
Source
#include <cstdio>
#include <algorithm>
using namespace std; const int MAXN = 1000+5;
int n;
int a[MAXN]; struct S{
int val;
int i, j;
bool operator < (const S& b)const
{
return val < b.val;
}
};
S l[MAXN*MAXN], r[MAXN*MAXN]; bool ok(S& a, S& b)
{
return a.i != b.i && a.j != b.j && a.i != b.j && a.j != b.i;
} void solve()
{
int lcnt = 0;
for(int i = 0; i < n; ++i){
for(int j = i+1; j < n; ++j){
l[lcnt].val = a[i]+a[j];
l[lcnt].i = i;
l[lcnt++].j = j;
}
}
int rcnt = 0;
for(int i = 0; i < n; ++i){
for(int j = i+1; j < n; ++j){
r[rcnt].val = a[i]-a[j];
r[rcnt].i = i;
r[rcnt++].j = j;
r[rcnt].val = a[j]-a[i];
r[rcnt].i = j;
r[rcnt++].j = i;
}
}
sort(l, l+lcnt);
int res = 0xffffffff;
for(int i = 0; i < rcnt; ++i){
int d = lower_bound(l, l+lcnt, r[i])-l;
if(ok(l[d], r[i]) && l[d].val == r[i].val){
res = max(res, r[i].val+a[r[i].j]);
}
}
if(res == 0xffffffff)
printf("no solution\n");
else
printf("%d\n", res);
} int main()
{
while(scanf("%d", &n), n){
for(int i = 0; i < n; ++i)
scanf("%d", &a[i]);
solve();
}
return 0;
}
POJ 2549 Sumsets的更多相关文章
- POJ 2549 Sumsets(折半枚举+二分)
Sumsets Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 11946 Accepted: 3299 Descript ...
- POJ 2549 Sumsets hash值及下标
题目大意:找到几何中的4个数字使他们能够组成 a+b+c=d , 得到最大的d值 我们很容易想到a+b = d-c 那么将所有a+b的值存入hash表中,然后查找能否在表中找到这样的d-c的值即可 因 ...
- [poj] 2549 Sumsets || 双向bfs
原题 在集合里找到a+b+c=d的最大的d. 显然枚举a,b,c不行,所以将式子移项为a+b=d-c,然后双向bfs,meet int the middle. #include<cstdio&g ...
- UVA 10125 - Sumsets(POJ 2549) hash
http://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem&p ...
- Divide and conquer:Sumsets(POJ 2549)
数集 题目大意:给定一些数的集合,要你求出集合中满足a+b+c=d的最大的d(每个数只能用一次) 这题有两种解法, 第一种就是对分,把a+b的和先求出来,然后再枚举d-c,枚举的时候输入按照降序搜索就 ...
- POJ 2549:Subsets(哈希表)
[题目链接] http://poj.org/problem?id=2549 [题目大意] 给出一个数集,从中选择四个元素,使得a+b+c=d,最小化d [题解] 我们对a+b建立Hash_table, ...
- POJ 2229 Sumsets
Sumsets Time Limit: 2000MS Memory Limit: 200000K Total Submissions: 11892 Accepted: 4782 Descrip ...
- POJ 2549 二分+HASH
题目链接:http://poj.org/problem?id=2002 题意:给定一个n个数字组成的序列,然后求出4个数使得a+b+c=d,求d的最大值.其中a,b,c,d要求是给定序列的数,并且不能 ...
- POJ 2549
Sumsets Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 8235 Accepted: 2260 Descripti ...
随机推荐
- Chp11: Sorting and Searching
Common Sorting Algo: Bubble Sort: Runime: O(n2) average and worst case. Memory: O(1). void BubbleSor ...
- poj 2253 Frogger (最短路变种,连通图的最长边)
题目 这里的dijsktra的变种代码是我看着自己打的,终于把代码和做法思路联系上了,也就是理解了算法——看来手跟着画一遍真的有助于理解. #define _CRT_SECURE_NO_WARNING ...
- POJ 1258 Agri-Net(最小生成树,模板题)
用的是prim算法. 我用vector数组,每次求最小的dis时,不需要遍历所有的点,只需要遍历之前加入到vector数组中的点(即dis[v]!=INF的点).但其实时间也差不多,和遍历所有的点的方 ...
- POJ1860Currency Exchange(SPFA)
http://poj.org/problem?id=1860 题意: 题目中主要是说存在货币兑换点,然后现在手里有一种货币,要各种换来换去,最后再换回去的时候看能不能使原本的钱数增多,每一种货币都有 ...
- Spring整合Ibatis
Spring整合Ibatis javaibatisspring 所需jar清单 ibatis-2.*.jar *为任意版本,下同,ibatis工作包 sp ...
- Project Euler 87 :Prime power triples 素数幂三元组
Prime power triples The smallest number expressible as the sum of a prime square, prime cube, and pr ...
- RN学习1——前奏,app插件化和热更新的探索
react_native_banner-min.png React Native(以下简称RN)有大量前端开发者的追捧.前端开发是一个活跃的社区,一直尝试着一统前后端,做一个全栈开发,RN就是他们在客 ...
- Photoshop:制作方块背景
1.填充背景色 2.滤镜->杂色->添加杂色 3.滤镜->像素化->马赛克 4.添加横线,明度-10 最终效果 附: 利用:查找边缘.最小值,还可以做这样的效果
- No compiler is provided in this environment. Perhaps you are running on a JRE rather than a JDK? 问题
maven编译项目时出错,提示信息如下: [ERROR] Failed to execute goal org.apache.maven.plugins:maven-compiler-plugin:3 ...
- Android Studio 初探
前言 上周由于写了一篇关于"Eclipse+ADT+Android SDK 搭建安卓开发环境" 的博文,其他博主们表示相当的不悦,都什么年代了还用Eclipse+ADT开发安卓应用 ...