HDU-4255
A Famous Grid
Time Limit: 10000/3000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 1496 Accepted Submission(s): 567
Construct the grid like the following figure. (The grid is actually infinite. The figure is only a small part of it.)
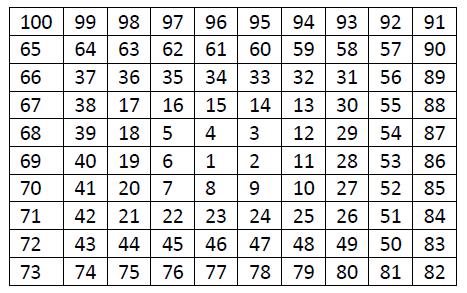
Considering
traveling in it, you are free to any cell containing a composite number
or 1, but traveling to any cell containing a prime number is
disallowed. You can travel up, down, left or right, but not diagonally.
Write a program to find the length of the shortest path between pairs of
nonprime numbers, or report it's impossible.
each test case, display its case number followed by the length of the
shortest path or "impossible" (without quotes) in one line.
- /**
- 题意:给出两个数,问两点之间的最短距离
- 做法:蛇形矩阵 + bfs + 优先队列
- **/
- #include <iostream>
- #include <stdio.h>
- #include <cmath>
- #include <algorithm>
- #include <string.h>
- #include <queue>
- #define maxn 40000
- using namespace std;
- int mmap[][];
- int a[][];
- int vis[][];
- int dx[] = {,,-,};
- int dy[] = {,-,,};
- int n,m;
- bool num[maxn];
- void is_prime()
- {
- int tot = ;
- memset(num,false,sizeof(num));
- num[] = true;
- for(long long i=; i<maxn; i++)
- {
- if(!num[i])
- {
- for(long long j=i*i; j<=maxn; j+=i)
- {
- num[j] = true;
- }
- }
- }
- }
- struct Node
- {
- int x;
- int y;
- int step;
- Node() {}
- Node(int _x,int _y,int _step)
- {
- x = ;
- y = ;
- step =;
- }
- } start,endd;
- struct cmp
- {
- bool operator () (const Node &a,const Node &b)
- {
- return a.step>b.step;
- }
- };
- int check(int x,int y)
- {
- if(x>= && x < && y >= && y < && num[a[x][y]] == true&& !vis[x][y]) return ;
- return ;
- }
- priority_queue<Node,vector<Node>,cmp >que;
- bool bfs()
- {
- memset(vis,,sizeof(vis));
- Node tmp,now;
- while(!que.empty()) que.pop();
- que.push(start);
- vis[start.x][start.y] = ;
- start.step = ;
- while(!que.empty())
- {
- now = que.top();
- que.pop();
- //cout<<now.x<<" "<<now.y<<" "<<now.step<<endl;
- if(now.x == endd.x && now.y == endd.y)
- {
- endd.step = now.step;
- return true;
- }
- for(int i=; i<; i++)
- {
- tmp.x = now.x + dx[i];
- tmp.y = now.y + dy[i];
- tmp.step = now.step + ;
- if(check(tmp.x,tmp.y))
- {
- vis[tmp.x][tmp.y] = ;
- que.push(tmp);
- }
- }
- }
- return false;
- }
- void init()
- {
- int x = ;
- int y = ;
- int nn = ;
- int num=a[][]=;
- while(num>)
- {
- while((y+)<nn&&!a[x][y+]) a[x][++y]= --num;
- while((x+)<nn&&!a[x+][y]) a[++x][y]= --num;
- while((y-)>=&&!a[x][y-]) a[x][--y]= --num;
- while((x-)>=&&!a[x-][y]) a[--x][y]= --num;
- }
- }
- int main()
- {
- //freopen("in.txt","r",stdin);
- init();
- is_prime();
- int Case = ;
- while(~scanf("%d %d",&n,&m))
- {
- for(int i=; i<; i++)
- {
- for(int j=; j<; j++)
- {
- if(a[i][j] == n)
- {
- start.x = i;
- start.y = j;
- }
- if(a[i][j] == m)
- {
- endd.x= i;
- endd.y = j;
- }
- }
- }
- bool prime = false;
- prime = bfs();
- printf("Case %d: ",Case++);
- if(prime) printf("%d\n",endd.step);
- else printf("impossible\n");
- }
- return ;
- }
HDU-4255的更多相关文章
- hdu 4255 A Famous Grid
题目连接 http://acm.hdu.edu.cn/showproblem.php?pid=4255 A Famous Grid Description Mr. B has recently dis ...
- HDOJ 2111. Saving HDU 贪心 结构体排序
Saving HDU Time Limit: 3000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) Total ...
- 【HDU 3037】Saving Beans Lucas定理模板
http://acm.hdu.edu.cn/showproblem.php?pid=3037 Lucas定理模板. 现在才写,noip滚粗前兆QAQ #include<cstdio> #i ...
- hdu 4859 海岸线 Bestcoder Round 1
http://acm.hdu.edu.cn/showproblem.php?pid=4859 题目大意: 在一个矩形周围都是海,这个矩形中有陆地,深海和浅海.浅海是可以填成陆地的. 求最多有多少条方格 ...
- HDU 4569 Special equations(取模)
Special equations Time Limit:1000MS Memory Limit:32768KB 64bit IO Format:%I64d & %I64u S ...
- HDU 4006The kth great number(K大数 +小顶堆)
The kth great number Time Limit:1000MS Memory Limit:65768KB 64bit IO Format:%I64d & %I64 ...
- HDU 1796How many integers can you find(容斥原理)
How many integers can you find Time Limit:5000MS Memory Limit:32768KB 64bit IO Format:%I64d ...
- hdu 4481 Time travel(高斯求期望)(转)
(转)http://blog.csdn.net/u013081425/article/details/39240021 http://acm.hdu.edu.cn/showproblem.php?pi ...
- HDU 3791二叉搜索树解题(解题报告)
1.题目地址: http://acm.hdu.edu.cn/showproblem.php?pid=3791 2.参考解题 http://blog.csdn.net/u013447865/articl ...
- hdu 4329
problem:http://acm.hdu.edu.cn/showproblem.php?pid=4329 题意:模拟 a. p(r)= R'/i rel(r)=(1||0) R ...
随机推荐
- 《Java程序设计》第六周学习总结 20165218 2017-2018-1
20165218 2017-2018-1 <Java程序设计>第六周学习总结 教材学习内容总结 第8章 常用实用类 String类 不可以有子类 在java.lang 包中,被默认引入 S ...
- IntelliJ IDEA 详细图解最常用的配置 ,适合新人,解决eclipse转idea的烦恼
刚刚使用IntelliJ IDEA 编辑器的时候,会有很多设置,会方便以后的开发,磨刀不误砍柴工. 比如:设置文件字体大小,代码自动完成提示,版本管理,本地代码历史,自动导入包,修改注释,修改tab的 ...
- RGB向yuv的转化最优算法,快得让你吃惊!
朋友曾经给我推荐了一个有关代码优化的pdf文档<让你的软件飞起来>,看完之后,感受颇深.为了推广其,同时也为了自己加深印象,故将其总结为word文档.下面就是其的详细内容总结,希望能于己于 ...
- Just Random HDU - 4790 思维题(打表找规律)分段求解
Coach Pang and Uncle Yang both love numbers. Every morning they play a game with number together. In ...
- unix awk手册读书笔记
http://note.youdao.com/noteshare?id=9ac76eb63a53ac000f7814454642d2b0
- DataGridView导出到Word
#region 使用Interop.Word.dll将DataGridView导出到Word /// <summary> /// 使用Interop.Word.dll将DataGridVi ...
- LightOJ 1226 - One Unit Machine Lucas/组合数取模
题意:按要求完成n个任务,每个任务必须进行a[i]次才算完成,且按要求,第i个任务必须在大于i任务完成之前完成,问有多少种完成顺序的组合.(n<=1000 a[i] <= 1e6 mod ...
- 快速排序Quick sort
快速排序Quick sort 原理,通过一趟扫描将要排序的数据分割成独立的两部分,其中一部分的所有数据都比另外一部分的所有数据都要小,然后再按此方法对这两部分数据分别进行快速排序,整个排序过程可以递归 ...
- C# 如何用多字符分割字符串
用单字符分割字符串大家应该很熟悉,例如: string source = "dfd^Afdf^AAAAAA^Adfdf"; var list= source.Split('A'); ...
- loj6102 「2017 山东二轮集训 Day1」第三题
传送门:https://loj.ac/problem/6102 [题解] 贴一份zyz在知乎的回答吧 https://www.zhihu.com/question/61218881 其实是经典问题 # ...