POJ 1556 The Doors(线段相交+最短路)
题目:
Description
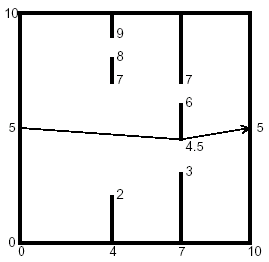
Input
2
4 2 7 8 9
7 3 4.5 6 7
The first line contains the number of interior walls. Then there is a line for each such wall, containing five real numbers. The first number is the x coordinate of the wall (0 < x < 10), and the remaining four are the y coordinates of the ends of the doorways in that wall. The x coordinates of the walls are in increasing order, and within each line the y coordinates are in increasing order. The input file will contain at least one such set of data. The end of the data comes when the number of walls is -1.
Output
Sample Input
1
5 4 6 7 8
2
4 2 7 8 9
7 3 4.5 6 7
-1
Sample Output
10.00
10.06
题意:给一个10*10的正方形 其中有数堵墙 每堵墙上都有两个门 给出两个门(每个门为一段区间)的坐标 求从(0,5)到(10,5)的最短路
思路:枚举每个门向后所有可直达的点的距离 然后建边跑最短路 因为数据很小 所以随便挑一个最短路跑就可以了
代码:
#include <iostream>
#include <cstdio>
#include <cstdlib>
#include <cmath>
#include <string>
#include <cstring>
#include <algorithm> using namespace std;
typedef long long ll;
typedef unsigned long long ull;
const double inf=0x3f3f3f3f;
const double eps=1e-;
const int maxn=;
int n;
double x,y_1,y2,y3,y4;
double dis[maxn][maxn]; int dcmp(double x){
if(fabs(x)<eps) return ;
if(x<) return -;
else return ;
} struct Point{
double x,y;
Point(){}
Point(double _x,double _y){
x=_x,y=_y;
}
Point operator + (const Point &b) const{
return Point(x+b.x,y+b.y);
}
Point operator - (const Point &b) const{
return Point(x-b.x,y-b.y);
}
double operator * (const Point &b) const{
return x*b.x+y*b.y;
}
double operator ^ (const Point &b) const{
return x*b.y-y*b.x;
}
}; struct Line{
Point s,e;
Line(){}
Line(Point _s,Point _e){
s=_s,e=_e;
}
}line[maxn]; bool inter(Line l1,Line l2){
return
max(l1.s.x,l1.e.x)>=min(l2.s.x,l2.e.x) &&
max(l2.s.x,l2.e.x)>=min(l1.s.x,l1.e.x) &&
max(l1.s.y,l1.e.y)>=min(l2.s.y,l2.e.y) &&
max(l2.s.y,l2.e.y)>=min(l1.s.y,l1.e.y) &&
dcmp((l2.s-l1.s)^(l1.e-l1.s))*dcmp((l2.e-l1.s)^(l1.e-l1.s))<= &&
dcmp((l1.s-l2.s)^(l2.e-l2.s))*dcmp((l1.e-l2.s)^(l2.e-l2.s))<=;
} double dist(Point a,Point b){
return sqrt((b-a)*(b-a));
} int main(){
while(~scanf("%d",&n)){
if(n==-) break;
for(int i=;i<=n;i++){
scanf("%lf%lf%lf%lf%lf",&x,&y_1,&y2,&y3,&y4);
line[*i-]=Line(Point(x,y_1),Point(x,y2));
line[*i]=Line(Point(x,y3),Point(x,y4));
}
for(int i=;i<=*n+;i++){
for(int j=;j<=*n+;j++){
if(i==j) dis[i][j]=;
else dis[i][j]=inf;
}
}
for(int i=;i<=*n;i++){
int lid=(i+)/;
int flag=;
Point tmp;
if(i&) tmp=line[(i+)/].s;
else tmp=line[(i+)/].e;
for(int j=;j<lid;j++){
if(inter(line[*j-],Line(Point(,),tmp))==false
&& inter(line[*j],Line(Point(,),tmp))==false)
flag=;
}
if(flag) dis[][i]=dis[i][]=dist(Point(,),tmp);
flag=;
for(int j=lid+;j<=n;j++){
if(inter(line[*j-],Line(Point(,),tmp))==false
&& inter(line[*j],Line(Point(,),tmp))==false)
flag=;
}
if(flag) dis[*n+][i]=dis[i][*n+]=dist(Point(,),tmp);
}
for(int i=;i<=*n;i++)
for(int j=i+;j<=*n;j++){
int lid1=(i+)/;
int lid2=(j+)/;
int flag=;
Point p1,p2;
if(i&) p1=line[(i+)/].s;
else p1=line[(i+)/].e;
if(j&) p2=line[(j+)/].s;
else p2=line[(j+)/].e;
for(int k=lid1+;k<lid2;k++){
if(inter(line[*k-],Line(p1,p2))==false
&& inter(line[*k],Line(p1,p2))==false)
flag=;
}
if(flag) dis[i][j]=dis[j][i]=dist(p1,p2);
}
int flag=;
for(int i=;i<=n;i++){
if(inter(line[*i-],Line(Point(,),Point(,)))==false
&& inter(line[*i],Line(Point(,),Point(,)))==false)
flag=;
}
if(flag) dis[][*n+]=dis[*n+][]=;
for(int k=;k<*n+;k++)
for(int i=;i<=*n+;i++)
for(int j=;j<=*n+;j++)
if(dis[i][j]>dis[i][k]+dis[k][j])
dis[i][j]=dis[i][k]+dis[k][j];
printf("%.2f\n",dis[][*n+]);
}
return ;
}
POJ 1556 The Doors(线段相交+最短路)的更多相关文章
- POJ 1556 - The Doors 线段相交不含端点
POJ 1556 - The Doors题意: 在 10x10 的空间里有很多垂直的墙,不能穿墙,问你从(0,5) 到 (10,5)的最短距离是多少. 分析: 要么直达,要么 ...
- POJ 1556 The Doors(线段交+最短路)
#include <iostream> #include <stdio.h> #include <string.h> #include <algorithm& ...
- POJ 1556 计算几何 判断线段相交 最短路
题意: 在一个左下角坐标为(0,0),右上角坐标为(10,10)的矩形内,起点为(0,5),终点为(10,5),中间会有许多扇垂直于x轴的门,求从起点到终点在能走的情况下的最短距离. 分析: 既然是求 ...
- POJ 1556 The Doors 线段交 dijkstra
LINK 题意:在$10*10$的几何平面内,给出n条垂直x轴的线,且在线上开了两个口,起点为$(0, 5)$,终点为$(10, 5)$,问起点到终点不与其他线段相交的情况下的最小距离. 思路:将每个 ...
- POJ 1556 The Doors 线段判交+Dijkstra
The Doors Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 6734 Accepted: 2670 Descrip ...
- POJ 2556 (判断线段相交 + 最短路)
题目: 传送门 题意:在一个左小角坐标为(0, 0),右上角坐标为(10, 10)的房间里,有 n 堵墙,每堵墙都有两个门.每堵墙的输入方式为 x, y1, y2, y3, y4,x 是墙的横坐标,第 ...
- POJ 1556 The Doors --几何,最短路
题意: 给一个正方形,从左边界的中点走到右边界的中点,中间有一些墙,问最短的距离是多少. 解法: 将起点,终点和所有墙的接触到空地的点存下来,然后两两之间如果没有线段(墙)阻隔,就建边,最后跑一个最短 ...
- POJ_1556_The Doors_判断线段相交+最短路
POJ_1556_The Doors_判断线段相交+最短路 Description You are to find the length of the shortest path through a ...
- POJ 1556 The Doors【最短路+线段相交】
思路:暴力判断每个点连成的线段是否被墙挡住,构建图.求最短路. 思路很简单,但是实现比较复杂,模版一定要可靠. #include<stdio.h> #include<string.h ...
- 简单几何(线段相交+最短路) POJ 1556 The Doors
题目传送门 题意:从(0, 5)走到(10, 5),中间有一些门,走的路是直线,问最短的距离 分析:关键是建图,可以保存所有的点,两点连通的条件是线段和中间的线段都不相交,建立有向图,然后用Dijks ...
随机推荐
- LeetCode 613. Shortest Distance in a Line
Table point holds the x coordinate of some points on x-axis in a plane, which are all integers. Writ ...
- 云计算openstack共享组件(3)——消息队列rabbitmq
队列(MQ)概念: MQ 全称为 Message Queue, 消息队列( MQ ) 是一种应用程序对应用程序的通信方法.应用程序通过读写出入队列的消息(针对应用程序的数据)来通信,而无需专用连接来链 ...
- [硬件]超能课堂(181):我们为什么需要4+8pin CPU供电接口?
超能课堂(181):我们为什么需要4+8pin CPU供电接口? https://www.expreview.com/68008.html 之前算过TDP 来计算机器的功耗 发现自己 理解的还是有偏差 ...
- kettle基于时间戳增量更新
思路1: 1.提前建好ts时间表,设置两个字段分别为current_t和load_t,current用于比较原表中日期的上限,load_t则为上次加载的日期,几位原表中日期的下限. create ta ...
- kettle查询-2
模糊匹配: 1.主数据/查询数据 2.模糊匹配 3.输出:jaro/jaro winkler/pair letters similarity(各自算法的匹配度measure value) http c ...
- AtCoder Beginner Contest 122 D - We Like AGC(DP)
题目链接 思路自西瓜and大佬博客:https://www.cnblogs.com/henry-1202/p/10590327.html#_label3 数据范围小 可直接dp f[i][j][a][ ...
- Nginx 容器
L39-40
- 【论文阅读】Sequence to Sequence Learning with Neural Network
Sequence to Sequence Learning with NN <基于神经网络的序列到序列学习>原文google scholar下载. @author: Ilya Sutske ...
- Vue组件以及组件之间的通信
一.组件的注册 1. 全局组件注册 1. 注册基本语法Vue.component Vue.component("my_header", { template: `<div&g ...
- 初步了解Bootstrap4
Bootstrap 是全球最受欢迎的前端组件库,用于开发响应式布局.移动设备优先的 WEB 项目. Bootstrap4 目前是 Bootstrap 的最新版本,是一套用于 HTML.CSS 和 JS ...