springboot(五)使用FastJson返回Json视图
FastJson简介:
本文目标:
一、项目搭建
项目搭建目录及数据库
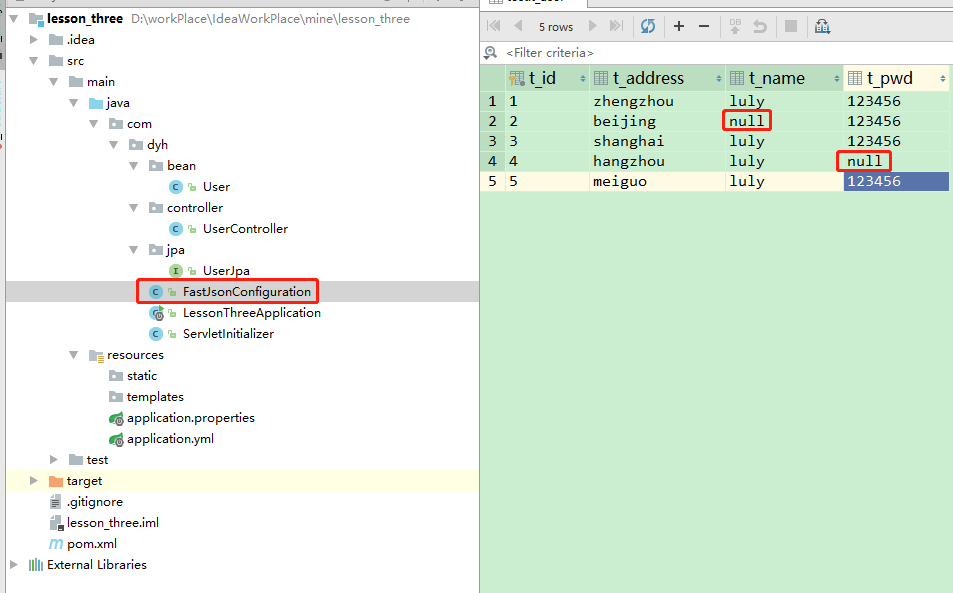
二、添加依赖(FastJson依赖)
spring-boot-stater-tomcat依赖的scope属性一定要注释掉,我们才能在IntelliJ IDEA工具使用SpringBootApplication的形式运行项目!
依赖地址:mvnrepository.com/artifact/com.alibaba/fastjson/1.2.31
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.2.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.dyh</groupId>
<artifactId>lesson_three</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>lesson_three</name>
<description>Demo project for Spring Boot</description> <properties>
<java.version>1.8</java.version>
</properties> <dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency> <dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<!--<scope>provided</scope>-->
</dependency> <dependency>
<!--fastJson-->
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.51</version>
</dependency> <dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies> <build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build> </project>
三、配置文件(该项目用Spring Data JPA架构)
spring:
datasource:
url: jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT
driverClassName: com.mysql.cj.jdbc.Driver
username: root
password: root jpa:
database: MySQL
show-sql: true
hibernate:
# naming_strategy: org.hibernate.cfg.ImprovedNamingStrategy
# ddl-auto: create
四、创建一个FastJsonConfiguration配置信息类。
添加@Configuration注解让SpringBoot自动加载类内的配置,有一点要注意我们继承了WebMvcConfigurerAdapter这个类,这个类是SpringBoot内部提供专门处理用户自行添加的配置,里面不仅仅包含了修改视图的过滤还有其他很多的方法,包括我们后面章节要讲到的拦截器,过滤器,Cors配置等。
@Configuration
public class FastJsonConfiguration extends WebMvcConfigurerAdapter
{
/**
* 修改自定义消息转换器
* @param converters 消息转换器列表
*/
@Override
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
//调用父类的配置
super.configureMessageConverters(converters);
//创建fastJson消息转换器
FastJsonHttpMessageConverter fastConverter = new FastJsonHttpMessageConverter();
//创建配置类
FastJsonConfig fastJsonConfig = new FastJsonConfig();
//修改配置返回内容的过滤
fastJsonConfig.setSerializerFeatures(
SerializerFeature.DisableCircularReferenceDetect,
SerializerFeature.WriteMapNullValue,
SerializerFeature.WriteNullStringAsEmpty
);
fastConverter.setFastJsonConfig(fastJsonConfig);
//将fastjson添加到视图消息转换器列表内
converters.add(fastConverter);
}
}
FastJson SerializerFeatures
WriteNullListAsEmpty :List字段如果为null,输出为[],而非null
五、测试,并比较用了FastJson与没有用之间的比较
使用前:

使用后:
name的值从NULL变成了"",那么证明我们的fastJson消息的转换配置完美生效了
springboot(五)使用FastJson返回Json视图的更多相关文章
- SpringBoot 03_利用FastJson返回Json数据
自上一节:SpringBoot 02_返回json数据,可以返回json数据之后,由于有些人习惯于不同的Json框架,比如fastjson,这里介绍一下如何在SpringBoot中集成fastjson ...
- 小记SpringMVC与SpringBoot 的controller的返回json数据的不同
近期由于项目的改动变更,在使用springmvc和springboot测试的时候发现一个有趣的现象 1.springmvc的controller使用@ResponseBody返回的仅仅是json格式的 ...
- fastjson 返回json字符串,JSON.parse 报错
这是由于转义字符引起的如 : \ , fastjson 处理后是双反斜杠:\\ ,而 JSON.parse 解析时需要4个反斜杠 ,即 js解析json 反斜杠时,需要 4个 解成 1 个 解决方法: ...
- SpringBoot项目中处理返回json的null值
在后端数据接口项目开发中,经常遇到返回的数据中有null值,导致前端需要进行判断处理,否则容易出现undefined的情况,如何便捷的将null值转换为空字符串? 以SpringBoot项目为例,SS ...
- 如何搭建一个WEB服务器项目(五)—— Controller返回JSON字符串
从服务器获取所需数据(JSON格式) 观前提示:本系列文章有关服务器以及后端程序这些概念,我写的全是自己的理解,并不一定正确,希望不要误人子弟.欢迎各位大佬来评论区提出问题或者是指出错误,分享宝贵经验 ...
- SpringBoot 02_返回json数据
在SpringBoot 01_HelloWorld的基础上来返回json的数据,现在前后端分离的情况下多数都是通过Json来进行交互,下面就来利用SpringBoot返回Json格式的数据. 1:新建 ...
- nutz的json视图
2.3. json视图 返回json视图有两种方法: @Ok("json") 与@Ok(“raw:json”) 2.3.1. @Ok("json") (1) ...
- 2.SpringBoot之返回json数据
一.创建一个springBoot个项目 操作详情参考:1.SpringBoo之Helloword 快速搭建一个web项目 二.编写实体类 /** * Created by CR7 on 2017-8- ...
- springboot使用fastJson作为json解析框架
springboot使用fastJson作为json解析框架 springboot默认自带json解析框架,默认使用jackson,如果使用fastjson,可以按照下列方式配置使用 〇.搭建spri ...
随机推荐
- 不就是语法和长难句吗—笔记总结Day4
第六课 英语的特殊结构 1.强调句型 It is...that / who / which(少见)... * 强调句型可以强调句子中所有成分(唯一不能强调谓语)* It is obviously t ...
- BZOJ3242 快餐店
原题传送门 题意 给定一个n条边n个点的连通图,求该图的某一点在该图距离最远的点距离它的距离的最小值. 题解 显然,答案是\(\frac {原图直径}{2}\). 本体的图有 \(n\) 个点 \(n ...
- 序列化和反序列化,请使用MessagePack
官网:https://msgpack.org/ 这个序列化的工具是今天看了dudu 的博客后去尝试使用的,果然差距很大. 对同样的对象进行序列化后,发现msgpack的大小仅有通常压缩工具Newton ...
- css圣杯布局的实现方式
css圣杯布局思路: 外面一个大div,里面三个小div(都是浮动).实现左右两栏宽度固定,中间宽度自适应.中间栏优先渲染. 资源网站大全 https://55wd.com 设计导航https://w ...
- Docker镜像-拉取并且运行
1.docker search : 从Docker Hub查找镜像 docker search [OPTIONS] 镜像名 OPTIONS说明: --automated :只列出 automated ...
- 基本数据类型--------------------集合set()
一.作用:集合.list.tuple.dict一样都可以存放多个值,但是集合主要用于:关系运算.去重 # 1.1 关系运算 friends1 = ["zero","kev ...
- MVC引用asp.net报表(测试小例子)
public class Default1Controller : Controller { // // GET: /Default1/ public ActionResult Index() { r ...
- USTC信息安全期末重点
一.ARP协议问题1. ARP协议的作用是什么.地址解析协议,即IP地址和MAC地址之间的转换. 2. 引入ARP缓存的功能是什么.将这一映射关系保存在 ARP 缓存中,使得不必重复运行 ARP 协议 ...
- 机器学习实战基础(三十八):随机森林 (五)RandomForestRegressor 之 用随机森林回归填补缺失值
简介 我们从现实中收集的数据,几乎不可能是完美无缺的,往往都会有一些缺失值.面对缺失值,很多人选择的方式是直接将含有缺失值的样本删除,这是一种有效的方法,但是有时候填补缺失值会比直接丢弃样本效果更好, ...
- easyui datagrid 中添加combobox
项目需要,如下图所示 <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> &l ...