poj 1279 -- Art Gallery (半平面交)
鏈接:http://poj.org/problem?id=1279
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 5337 | Accepted: 2277 |
Description
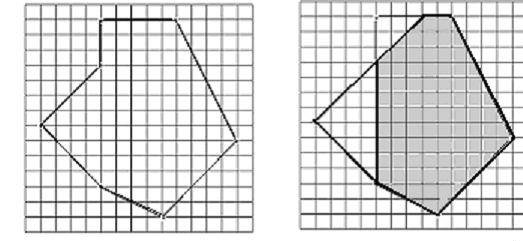
Input
Output
Sample Input
1
7
0 0
4 4
4 7
9 7
13 -1
8 -6
4 -4
Sample Output
80.00
Source
#include <stdio.h>
#include <math.h>
#include <string.h>
#include <stdlib.h>
#include <iostream>
#include <algorithm> #define eps 1e-8
#define MAXX 1510 typedef struct point
{
double x;
double y;
}point; point p[MAXX],s[MAXX]; using namespace std;
bool dy(double x,double y)
{
return x>y+eps;
}
bool xy(double x,double y)
{
return x<y-eps;
}
bool dyd(double x,double y)
{
return x>y-eps;
}
bool xyd(double x,double y)
{
return x<y+eps;
}
bool dd(double x,double y)
{
return fabs(x-y)<eps;
} double crossProduct(point a,point b,point c)
{
return (c.x-a.x)*(b.y-a.y)-(c.y-a.y)*(b.x-a.x);
} point IntersectPoint(point u1,point u2,point v1,point v2)
{
point ans=u1;
double t = ((u1.x-v1.x)*(v1.y-v2.y)-(u1.y-v1.y)*(v1.x-v2.x))/
((u1.x-u2.x)*(v1.y-v2.y)-(u1.y-u2.y)*(v1.x-v2.x));
ans.x += (u2.x-u1.x)*t;
ans.y += (u2.y-u1.y)*t;
return ans;
} double Area(point p[],int n)
{
double ans=0.0;
for(int i=; i<n-; i++)
{
ans += crossProduct(p[],p[i],p[i+]);
}
return fabs(ans)/2.0;
} double dist(point a,point b)
{
return sqrt((a.x-b.x)*(a.x-b.x)+(a.y-b.y)*(a.y-b.y));
} bool cmp(point a,point b)
{
double tmp=crossProduct(p[],a,b);
if(dd(tmp,0.0))
return dy(dist(p[],a),dist(p[],b));
return xy(tmp,0.0);
} point Getsort(int n)
{
int tmp=;
for(int i=; i<n; i++)
{
if(xy(p[i].x,p[tmp].x) || dd(p[i].x,p[tmp].x)&&xy(p[i].y,p[tmp].y))
{
tmp=i;
}
}// printf("%d^^",tmp);
swap(p[],p[tmp]);
sort(p+,p+n,cmp);
} void cut(point p[],point s[],int n,int &len)
{
point tp[MAXX];
p[n]=p[];
for(int i=; i<=n; i++)
{
tp[i]=p[i];
}
int cp=n,tc;
for(int i=; i<n; i++)
{
tc=;
for(int k=; k<cp; k++)
{
if(xyd(crossProduct(p[i],p[i+],tp[k]),0.0))
s[tc++]=tp[k];
if(xy(crossProduct(p[i],p[i+],tp[k])*
crossProduct(p[i],p[i+],tp[k+]),0.0))
s[tc++]=IntersectPoint(p[i],p[i+],tp[k],tp[k+]);
}
s[tc]=s[];
for(int k=; k<=tc; k++)
tp[k]=s[k];
cp=tc;
}
len=cp;
} int main()
{
int t,n,m,i,j;
scanf("%d",&t);
while(t--)
{
scanf("%d",&n);
for(i=; i<n; i++)
{
scanf("%lf%lf",&p[i].x,&p[i].y);
}
//point tmp=IntersectPoint(p[0],p[1],p[2],p[3]);
//printf("%lf %lf\n",tmp.x,tmp.y);
Getsort(n);//for(i=0; i<n; i++)printf("%lf**%lf*\n",p[i].x,p[i].y);
int len;
cut(p,s,n,len);//for(i=0; i<len; i++)printf("%lf==%lf=\n",s[i].x,s[i].y);
double area=Area(s,len);
printf("%.2lf\n",area);
}
return ;
}
利用面积正负来判断顺or逆,这种代码是以逆时针为主,我的面积顺时针为正,
需要改变方向
#include <stdio.h>
#include <math.h>
#include <string.h>
#include <stdlib.h>
#include <iostream>
#include <algorithm> #define eps 1e-8
#define MAXX 1510 typedef struct point
{
double x;
double y;
}point; point p[MAXX],s[MAXX]; using namespace std;
bool dy(double x,double y)
{
return x>y+eps;
}
bool xy(double x,double y)
{
return x<y-eps;
}
bool dyd(double x,double y)
{
return x>y-eps;
}
bool xyd(double x,double y)
{
return x<y+eps;
}
bool dd(double x,double y)
{
return fabs(x-y)<eps;
} double crossProduct(point a,point b,point c)
{
return (c.x-a.x)*(b.y-a.y)-(c.y-a.y)*(b.x-a.x);
} point IntersectPoint(point u1,point u2,point v1,point v2)
{
point ans=u1;
double t = ((u1.x-v1.x)*(v1.y-v2.y)-(u1.y-v1.y)*(v1.x-v2.x))/
((u1.x-u2.x)*(v1.y-v2.y)-(u1.y-u2.y)*(v1.x-v2.x));
ans.x += (u2.x-u1.x)*t;
ans.y += (u2.y-u1.y)*t;
return ans;
} double Area(point p[],int n)
{
double ans=0.0;
p[n]=p[];
point tmp;
tmp.x=,tmp.y=;
for(int i=; i<n; i++)
{
ans += crossProduct(tmp,p[i],p[i+]);
}
return ans/2.0;
} void changeWise(point p[],int n)
{
for(int i=; i<n/; i++)
swap(p[i],p[n-i-]);
} double dist(point a,point b)
{
return sqrt((a.x-b.x)*(a.x-b.x)+(a.y-b.y)*(a.y-b.y));
}
/*
bool cmp(point a,point b)
{
double tmp=crossProduct(p[0],a,b);
if(dd(tmp,0.0))
return dy(dist(p[0],a),dist(p[0],b));
return xy(tmp,0.0);
} point Getsort(int n)
{
int tmp=0;
for(int i=1; i<n; i++)
{
if(xy(p[i].x,p[tmp].x) || dd(p[i].x,p[tmp].x)&&xy(p[i].y,p[tmp].y))
{
tmp=i;
}
}// printf("%d^^",tmp);
swap(p[0],p[tmp]);
sort(p+1,p+n,cmp);
}
*/
void cut(point p[],point s[],int n,int &len)
{
point tp[MAXX];
p[n]=p[];
for(int i=; i<=n; i++)
{
tp[i]=p[i];
}
int cp=n,tc;
for(int i=; i<n; i++)
{
tc=;
for(int k=; k<cp; k++)
{
if(xyd(crossProduct(p[i],p[i+],tp[k]),0.0))
s[tc++]=tp[k];
if(xy(crossProduct(p[i],p[i+],tp[k])*
crossProduct(p[i],p[i+],tp[k+]),0.0))
s[tc++]=IntersectPoint(p[i],p[i+],tp[k],tp[k+]);
}
s[tc]=s[];
for(int k=; k<=tc; k++)
tp[k]=s[k];
cp=tc;
}
len=cp;
} int main()
{
int t,n,m,i,j;
scanf("%d",&t);
while(t--)
{
scanf("%d",&n);
for(i=; i<n; i++)
{
scanf("%lf%lf",&p[i].x,&p[i].y);
}
double tmp=Area(p,n);
if(dy(tmp,0.0))
changeWise(p,n);
//point tmp=IntersectPoint(p[0],p[1],p[2],p[3]);
//printf("%lf %lf\n",tmp.x,tmp.y);
//Getsort(n);for(i=0; i<n; i++)printf("%lf**%lf*\n",p[i].x,p[i].y);
int len;
cut(p,s,n,len);//for(i=0; i<len; i++)printf("%lf==%lf=\n",s[i].x,s[i].y);
double area=Area(s,len);
printf("%.2lf\n",fabs(area));
}
return ;
}
poj 1279 -- Art Gallery (半平面交)的更多相关文章
- POJ 1279 Art Gallery 半平面交/多边形求核
http://poj.org/problem?id=1279 顺时针给你一个多边形...求能看到所有点的面积...用半平面对所有边取交即可,模版题 这里的半平面交是O(n^2)的算法...比较逗比.. ...
- POJ 1279 Art Gallery 半平面交求多边形核
第一道半平面交,只会写N^2. 将每条边化作一个不等式,ax+by+c>0,所以要固定顺序,方便求解. 半平面交其实就是对一系列的不等式组进行求解可行解. 如果某点在直线右侧,说明那个点在区域内 ...
- POJ 1279 Art Gallery(半平面交)
题目链接 回忆了一下,半平面交,整理了一下模版. #include <cstdio> #include <cstring> #include <string> #i ...
- POJ 1279 Art Gallery 半平面交 多边形的核
题意:求多边形的核的面积 套模板即可 #include <iostream> #include <cstdio> #include <cmath> #define ...
- poj 1279 Art Gallery - 求多边形核的面积
/* poj 1279 Art Gallery - 求多边形核的面积 */ #include<stdio.h> #include<math.h> #include <al ...
- poj 1279 Art Gallery (Half Plane Intersection)
1279 -- Art Gallery 还是半平面交的问题,要求求出多边形中可以观察到多边形所有边的位置区域的面积.其实就是把每一条边看作有向直线然后套用半平面交.这题在输入的时候应该用多边形的有向面 ...
- POJ 1279 Art Gallery(半平面交求多边形核的面积)
题目链接 题意 : 求一个多边形的核的面积. 思路 : 半平面交求多边形的核,然后在求面积即可. #include <stdio.h> #include <string.h> ...
- [POJ]1279: Art Gallery
题目大意:有一个N边形展馆,问展馆内有多少地方可以看到所有墙壁.(N<=1500) 思路:模板题,半平面交求出多边形的核后计算核的面积. #include<cstdio> #incl ...
- POJ 1279 Art Gallery【半平面交】(求多边形的核)(模板题)
<题目链接> 题目大意: 按顺时针顺序给出一个N边形,求N边形的核的面积. (多边形的核:它是平面简单多边形的核是该多边形内部的一个点集该点集中任意一点与多边形边界上一点的连线都处于这个多 ...
随机推荐
- C++线性方程求解
介绍 程序SolveLinearEquations解决联立方程.该方案需要一个文本文件,其中包含输入和输出方程解决.这个项目是几年前我写在C#中http://www.codeproject.com/A ...
- Linux字符设备驱动结构(一)--cdev结构体、设备号相关知识机械【转】
本文转载自:http://blog.csdn.net/zqixiao_09/article/details/50839042 一.字符设备基础知识 1.设备驱动分类 linux系统将设备分为3类:字符 ...
- 160909、Filter多方式拦截、禁用IE图片缓存、Filter设置字符编码
dispatcher多方式拦截 我们来看一个例子 我们定义一个index.jsp,里面有一个链接跳转到dispatcher.jsp页面 <body> <a href="di ...
- loading等待载入正在加载的动画GIF图片圆形图标
http://www.wtoutiao.com/p/GdfbdM.html
- eclipse Juno Indigo Helios Galileo这几种版本的意思
Eclipse 3.1 版本代号 IO [木卫1,伊奥] Eclipse 3.2, 30-06-2006, Callisto projects, 版本代号 Callisto [木卫四,卡里斯托 ] ...
- git 使用详解(5)-- get log 查看提交历史【转】
转自:http://blog.csdn.net/wh_19910525/article/details/7468549 版权声明:本文为博主原创文章,未经博主允许不得转载. 目录(?)[-] 限制 ...
- jenkins+jmeter+ant搭建接口测试平台
接口测试的重点是检查数据的交换,传递和控制管理过程以及系统间的相互逻辑依赖关系. 接口测试的流程 项目启动后,测试人员要尽早拿到接口测试文档. 开始编写接口测试用例 将接口测试用例部署到持续集成的测试 ...
- Nagios监控Oralce
一.本文说明: 本文是监控本地的Oracle,其实监控远端的Oracle也是跟下面的步骤差不多的. 二.安装Nagios.Nagios插件.NRPE软件: 安装步骤可以参考<Linux下Nagi ...
- Mybatis用法小结
select 1.基本用法 <select id="selectTableOne" resultType="com.test.entity.tableOne&quo ...
- hasOwnproperty详细总结
hasOwnProperty:是用来判断一个对象是否有你给出名称的属性或对象.不过需要注意的是,此方法无法检查该对象的原型链中是否具有该属性,该属性必须是对象本身的一个成员. isPrototypeO ...