巡逻机器人(BFS)
巡逻机器人问题(F - BFS,推荐)
Description
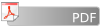
A robot has to patrol around a rectangular area which is in a form of mxn grid (m rows and n columns). The rows are labeled from 1 to m. The columns are labeled from 1 to n. A cell (i, j) denotes the cell in row i and column j in the grid. At each step, the robot can only move from one cell to an adjacent cell, i.e. from (x, y) to (x + 1, y), (x, y + 1), (x - 1, y) or (x, y - 1). Some of the cells in the grid contain obstacles. In order to move to a cell containing obstacle, the robot has to switch to turbo mode. Therefore, the robot cannot move continuously to more than k cells containing obstacles.
Your task is to write a program to find the shortest path (with the minimum number of cells) from cell (1, 1) to cell (m, n). It is assumed that both these cells do not contain obstacles.
Input
The input consists of several data sets. The first line of the input file contains the number of data sets which is a positive integer and is not bigger than 20. The following lines describe the data sets.
For each data set, the first line contains two positive integer numbers m and n separated by space (1m, n
20). The second line contains an integer number k(0
k
20). The ith line of the next m lines contains n integer aij separated by space (i = 1, 2,..., m;j = 1, 2,..., n). The value of aij is 1 if there is an obstacle on the cell (i, j), and is 0 otherwise.
Output
For each data set, if there exists a way for the robot to reach the cell (m, n), write in one line the integer number s, which is the number of moves the robot has to make; -1 otherwise.
Sample Input
- 3
- 2 5
- 0
- 0 1 0 0 0
- 0 0 0 1 0
- 4 6
- 1
- 0 1 1 0 0 0
- 0 0 1 0 1 1
- 0 1 1 1 1 0
- 0 1 1 1 0 0
- 2 2
- 0
- 0 1
- 1 0
- Sample Output
7- 10
- -1
题目大意:
机器人要从一个M*N(1m, n
20)网格的左上角(1,1)走到右下角(M,N)。网格中的一些格子是空地(用0表示),其他格子是障碍(用1表示)。
机器人每次可以往4个方向走一格,但不能连续的穿越k(0k
20)个障碍,求最短路长度。起点和终点保证是空地。
- 分析:
求最短路径,即最优解问题,采用BFS。
1.可以跨过一个障碍,但不能连续跨障碍。
2.边界点没有4个方向
3.要将走过的点进行标记- 代码:
- #include<cstdio>
- #include<iostream>
- #include<cstring>
- #include<queue>
- using namespace std;
- #define MAX 23
- int a[MAX][MAX];
- int nxt[][] = {{,},{,-},{-,},{,}};
- int book[MAX][MAX][MAX];
- int n,m,k;
- struct node
- {
- int x,y;
- int step;
- int kk;
- };
- queue<node>Q;
- void init()
- {
- while (!Q.empty())
- {
- Q.pop();
- }
- memset(book,,sizeof(book));
- }
- int can_move( int x ,int y,int kk )
- {
- if ( x>=&&x<=n&&y>=&&y<=m&&book[x][y][kk]== )
- return ;
- else
- return ;
- }
- int bfs ( node start )
- {
- init();
- if ( start.x==n&&start.y==m )
- {
- return start.step;
- }
- Q.push(start);
- while ( !Q.empty() )
- {
- node now = Q.front();
- Q.pop();
- if ( now.kk < )
- continue;
- for ( int i = ;i < ;i++ )
- {
- node newnode;
- int tx = now.x+nxt[i][], ty = now.y+nxt[i][];
- if ( a[tx][ty]== )
- newnode.kk = now.kk-;
- else
- newnode.kk = k;
- if ( can_move(tx,ty,newnode.kk) )
- {
- if ( tx==n&&ty==m )
- {
- return now.step+;
- }
- newnode.x = tx; newnode.y = ty; newnode.step = now.step+;
- if( newnode.kk >= )
- {
- Q.push(newnode);
- book[tx][ty][newnode.kk] = ;
- }
- }
- }
- }
- return -;
- }
- int main(void)
- {
- int t;scanf("%d",&t);
- while ( t-- )
- {
- scanf("%d%d%d",&n,&m,&k);
- for ( int i = ;i <= n;i++ )
- {
- for ( int j = ;j <= m;j++ )
- {
- scanf("%d",&a[i][j]);
- }
- }
- node start;
- start.x = ; start.y = ;start.step = ; start.kk = k;
- book[start.x][start.y][start.kk] = ;
- int ans = bfs(start);
- if ( ans == - )
- printf("-1\n");
- else
- printf("%d\n",ans);
- }
- return ;
- }
心得:
BFS的题自己还是不会写,要看别人AC的代码才会。还是要多看一些别人的优秀代码来仿写,加油吧,争取自己能写好的代码。
巡逻机器人(BFS)的更多相关文章
- BFS 巡逻机器人
巡逻机器人 题目链接:http://acm.hust.edu.cn/vjudge/contest/view.action?cid=83498#problem/F 题目大意: 机器人在一个矩形区域巡逻, ...
- UVA 1600 Patrol Robert 巡逻机器人 (启发搜索BFS)
非常适合A*的一道题. 比普通的迷宫问题加一个信息k表示当前穿过的障碍物的数量. #include<cstdio> #include<cstring> #include< ...
- UVA - 1600 Patrol Robot (巡逻机器人)(bfs)
题意:从(1,1)走到(m,n),最多能连续穿越k个障碍,求最短路. 分析:obstacle队列记录当前点所穿越的障碍数,如果小于k可继续穿越障碍,否则不能,bfs即可. #pragma commen ...
- UVA:1600 巡逻机器人
机器人要从一个m*n(m和n的范围都在1到20的闭区间内)的网格的左上角(1,1)走到右下角(m,n).网格中的一些格子是空地,用0表示,其它格子是障碍,用1表示.机器人每次可以往四个方向走一格,但不 ...
- UVa——1600(巡逻机器人)
迷宫求最短路的话一般用bfs都可以解决,但是这个加了个状态,那么就增加一个维度,用来判断k的值.比较简单的三维bfs.写搜索题的话一定要注意细节.这个题花了好长的时间.因为k的原因,一开始用了k的原因 ...
- 6-5 巡逻机器人 uva1600
一开始按照标准bfs来写 标记为二维数组 后来按照三维数组写过了 ps大部分bfs都不会是二维数组搞定!!! 其中有一个bug弄了半个小时... 一开始我是先判断!vis[x][y][v.c] ...
- 中国VR公司的详尽名单
中国VR公司的详尽名单 <VR圈深度投资报告一:2014年以来所有VR/AR融资事件> 特征一.投资机构观望居多 尽管VR在媒体和二级市场炒得很热,但大多风险投资机构却慎于出手,以观望 ...
- 日常英语---十三、MapleStory/Monsters/Level 11-20(邪恶之眼)
日常英语---十三.MapleStory/Monsters/Level 11-20(邪恶之眼) 一.总结 一句话总结: evil ['ivl] A stronger version of Evil E ...
- 洛谷P1126机器人搬重物[BFS]
题目描述 机器人移动学会(RMI)现在正尝试用机器人搬运物品.机器人的形状是一个直径1.6米的球.在试验阶段,机器人被用于在一个储藏室中搬运货物.储藏室是一个N*M的网格,有些格子为不可移动的障碍.机 ...
随机推荐
- CSS Select 标签取选中文本值
$("#userDep").find("option:selected").text()
- xar安装使用方法
xar是一种扩展的归档格式(eXtensible ARchive format),是一种开源的文件格式.xar文件在Mac OS X 10.5里是用于软件安装程序. ---------------- ...
- java.lang.ClassCastException: oracle.sql.TIMESTAMP cannot be cast to java.sql.Timestamp
http://stackoverflow.com/questions/13269564/java-lang-classcastexception-oracle-sql-timestamp-cannot ...
- 如何使用robots不让百度和google收录
如何使用robots不让百度和google收录 有没有想过,如果我们某个站点不让百度和google收录,那怎么办? 搜索引擎已经和我们达成一个约定,如果我们按约定那样做了,它们就不要收录. 这个写 ...
- OTL使用总结
在VC中访问Oracle,可以使用ADO或ODBC,如果你比较强大,也可以直接使用OCI API,但我个人认为OTL是最佳选择,它是一套数据库访问C++模板库,全部代码都在otlv4.h头文件中,通过 ...
- hdoj 1878 欧拉回路(无向图欧拉回路+并查集)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1878 思路分析:该问题给定一个无向图,要求判断该无向图是否存在欧拉回路:无向图判断存在欧拉回路的两个必 ...
- golang实现tcp接入服务器
接入服务器和后端业务服务其维持tcp连接,多个前端请求通过接入服务器访问后端业务服务器,接入服务器可以方便增加路由功能,维护多个业务服务器,根据消息ID路由到具体的业务服务器. 项目目录如下 simp ...
- Winter(bfs&&dfs)
1084 - Winter PDF (English) Statistics Forum Time Limit: 2 second(s) Memory Limit: 32 MB Winter is ...
- HDU 1796 容斥原理 How many integers can you find
题目连接 http://acm.hdu.edu.cn/showproblem.php?pid=1796 处男容斥原理 纪念一下 TMD看了好久才明白DFS... 先贴代码后解释 #includ ...
- C#中继承,集合(Eleventh day)
又到了总结知识的时间,今天在云和学院继续学习了继承的一些运用,和集合的运用.下面就总结下来吧 理论: 显示调用父类的构造方法,关键字: base:构造函数不能被继承:子类对象被实例化的时候会先去主动的 ...