Javascript函数重载,存在呢—还是存在呢?
1.What's is 函数重载?
2.静态语言的重载
#include <iostream>
using namespace std;
void print(int i) {
cout <<"Here is num " << i << endl;
}
void print(string str) {
cout << "Here is string " <<str<< endl;
}
int main() {
print(10);//Here is int 10
print("ten");//Here is string ten
}
function print(i) {
console.log("Here is num"+i);
}
function print(str) {
console.log("Here is string "+str);
}
print(100);//Here is string 100
print("ten");//Here is string ten
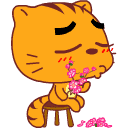
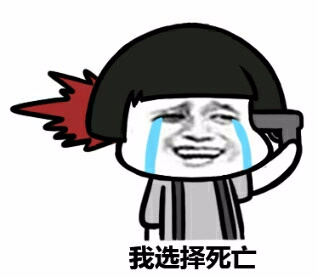
3.如何实现具有Javascript特色的函数重载
- Using different names in the first place(使用不同的函数名)
function printNum(num) {
console.log("Here is num "+num);
}
function printString(str) {
console.log("Here is string "+str);
}
printNum(100);//Here is num 100
printString("ten");//Here is string ten
- Using optional arguments like
y = y || 'default'(检测是否提供实参,否则采用默认值)
举例说明,现在我们要同时打印一个数字和一个字符串
function print(num,str){
var inner_num=num||0;
var inner_str=str||"default";
console.log("Here is num "+inner_num);
console.log("Here is string "+inner_str);
}
print();
//Here is num 0
//Here is string default
print(10);
//Here is num 10
//Here is string default
print(10,"ten");
//Here is num 10
//Here is string ten
- Using number of arguments(根据参数数量来实现重载)
function print(num,str){
var len=arguments.length;
if (len===1) {
console.log("Here is num "+num);
}
else if (len===2) {
console.log("Here is num "+num);
console.log("Here is string "+str);
}
else{
console.log("看....,飞碟!");
}
}
print();//看....,飞碟!
print(10);//Here is num 10
print(10,"ten");
//Here is num 10
//Here is string ten
- Checking types of arguments (根据参数类型来实现重载)
function print(num,str){
if (typeof num=="number") {
console.log("Here is num "+num);
}
if (typeof str=="string") {
console.log("Here is string "+str);
}
}
print();//无输出
print(10);//Here is num 10
print('',"ten");//Here is string ten
print(10,"ten");
// Here is num 10
// Here is string ten
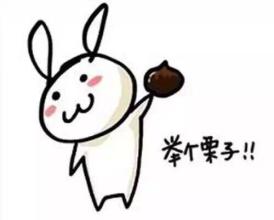
function print(num,str){
var len=arguments.length;
if((len===1)&& (typeof arguments[0]==="number")){
console.log("Here is num "+arguments[0]);
}
else if ((len===1)&& (typeof arguments[0]==="string")) {
console.log("Here is string "+arguments[0]);
}
else if((len===2)&& (typeof arguments[0]==="number")&& (typeof arguments[1]==="string")) {
console.log("Here is num "+arguments[0]);
console.log("Here is string "+arguments[1]);
}
else{
console.log("看....,飞碟!");
}
}
print();//看....,飞碟!
print(10);//Here is num 10
print("ten");//Here is string ten
print(10,"ten");
// Here is num 10
// Here is string ten
function foo(a, b, opts) {
}
foo(1, 2, {"method":"add"});
foo(3, 4, {"test":"equals", "bar":"tree"});
function sayName(name){
alert(name);
}
function sum(num1, num2){
return num1 + num2;
}
function sayHi(){
alert("hi");
}
alert(sayName.length); //1
alert(sum.length); //2
alert(sayHi.length); //0
■ 通过其length属性,可以知道声明了多少命名参数
■ 通过arguments.length,可以知道在调用时传入了多少参数
下面介绍一下大牛是如何实现借助这个特性实现函数的重载的
var ninja = {};
addMethod(ninja,'whatever',function(){ /* do something */ });
addMethod(ninja,'whatever',function(a){ /* do something else */ });
addMethod(ninja,'whatever',function(a,b){ /* yet something else */ });
function addMethod(object, name, fn) {
var old = object[name]; //保存原有的函数,因为调用的时候可能不匹配传入的参数个数
object[name] = function() { // 重写了object[name]的方法,是一个匿名函数
// 如果该匿名函数的形参个数和实参个数匹配,就调用该函数
if(fn.length === arguments.length) {
return fn.apply(this, arguments);
// 如果传入的参数不匹配,就调用原有的参数
} else if(typeof old === "function") {
return old.apply(this, arguments);
}
}
}
- 第一个为要绑定方法的对象,
- 第二个为绑定方法所用的属性名称,
- 第三个为需要绑定的方法(一个匿名函数)
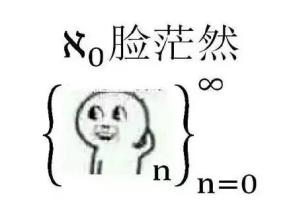
//addMethod
function addMethod(object, name, fn) {
var old = object[name];
object[name] = function() {
if(fn.length === arguments.length) {
return fn.apply(this, arguments);
} else if(typeof old === "function") {
return old.apply(this, arguments);
}
}
}
var printer= {};
// 不传参数时,打印“飞碟”
addMethod(printer, "print", function() {
console.log("看,飞碟!");
});
// 传一个参数时,打印数字
addMethod(printer, "print", function(num) {
console.log("Here is num "+num);
});
// 传两个参数时,打印数字和字符串
addMethod(printer, "print", function(num,str) {
console.log("Here is num "+ num);
console.log("Here is string "+str);
});
// 测试:
printer.print(); //看,飞碟!
printer.print(10); //Here is num 10
printer.print(10, "ten");
//Here is num 10
//Here is string ten
// Function.prototype.overload - By 沐浴星光
Function.prototype.overload = function(fn) {
var old= this;
return function() {
if (fn.length===arguments.length) {
return fn.apply(this,arguments);
}else{
return old.apply(this,arguments);
}
}
}
// 不传参数时,打印“飞碟”
function printNone() {
console.log("看,飞碟!");
}
// 传一个参数时,打印数字
function printInt(num) {
console.log("Here is num "+num);
}
// 传两个参数时,打印数字和字符串
function printBoth(num,str) {
console.log("Here is num "+ num);
console.log("Here is string "+str);
}
print=function(){};
print=print.overload(printNone).overload(printInt).overload(printBoth);
print();//看,飞碟!
print(10);//Here is num 10
print(10,"ten");
// Here is num 10
// Here is string ten
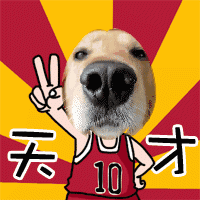
Javascript函数重载,存在呢—还是存在呢?的更多相关文章
- javascript 函数重载 overloading
函数重载 https://en.wikipedia.org/wiki/Function_overloading In some programming languages, function over ...
- JavaScript函数重载
译者按: jQuery之父John Resig巧妙地利用了闭包,实现了JavaScript函数重载. 原文: JavaScript Method Overloading 译者: Fundebug 为了 ...
- [转载]浅谈JavaScript函数重载
原文地址:浅谈JavaScript函数重载 作者:ChessZhang 上个星期四下午,接到了网易的视频面试(前端实习生第二轮技术面试).面了一个多小时,自我感觉面试得很糟糕的,因为问到的很多问题都 ...
- javascript arguments与javascript函数重载
1.所 有的函数都有属于自己的一个arguments对象,它包括了函所要调用的参数.他不是一个数组,如果用typeof arguments,返回的是’object’.虽然我们可以用调用数据的方法来调用 ...
- JavaScript “函数重载”
函数重载(function overloading)必须依赖两件事情:判断传入参数数量的能力和判断传入参数类型的能力. JavaScript的每个函数都带有一个仅在这个函数范围内作用的变量argume ...
- 浅谈JavaScript函数重载
上个星期四下午,接到了网易的视频面试(前端实习生第二轮技术面试).面了一个多小时,自我感觉面试得很糟糕的,因为问到的很多问题都很难,根本回答不上来.不过那天晚上,还是很惊喜的接到了HR面电话.现在HR ...
- javascript 函数重载另一种实现办法
最近在读javascript忍者 感受下jquery作者 john Resig对于js的独到见解. 先上代码: function addMethod(object,name,fn){ var old ...
- 理解javascript函数的重载
javascript其实是不支持重载的,这篇文章会和java语言函数的重载对比来加深对javascript函数重载的理解. 以下我会假设读者不了解什么是重载所以会有一些很基础的概念 ...
- JavaScript模拟函数重载
JavaScript是没有函数重载的,但是我们可以通过其他方法来模拟函数重载,如下所示: <!DOCTYPE html> <html> <head> <met ...
随机推荐
- less简介
Less是一种动态的样式语言.Less扩展了CSS的动态行为,比如说,设置变量(Variables).混合书写模式(mixins).操作(operations)和功能(functions)等等,最棒的 ...
- Thinking in java学习笔记之final
- eclipse注释快捷键(含方法注释)
整段注释: /*public boolean executeUpdate(String sql) { System.out.println(sql); boolean mark=false; try ...
- CLI:如何使用Go开发命令行
CLI或者"command line interface"是用户在命令行下交互的程序.由于通过将程序编译到一个静态文件中来减少依赖,一次Go特别适合开发CLI程序.如果你编写过安装 ...
- mysql-5.7日志设置
环境 Windows10企业版X64 mysql安装目录:D:\mysql-5.7.15-winx64. 在mysql安装目录下手工新建一个log目录:mysql\log. mysql\my.ini内 ...
- [NHibernate]基本配置与测试
目录 写在前面 nhibernate文档 搭建项目 映射文件 持久化类 辅助类 数据库设计与连接配置 测试 总结 写在前面 一年前刚来这家公司,发现项目中使用的ORM是Nhibernate,这个之前确 ...
- LYDSY模拟赛day1 String Master
/* 暴力枚举两个后缀,计算最长能匹配多少前缀. 最优策略一定是贪心改掉前 k 个失配的字符. 时间复杂度 O(n3). */ #include<cstdio> ],b[]; int ma ...
- PHP计算一年有多少周,每周开始日期和结束日期
一年有多个周,每周的开始日期和结束日期 参考代码一:[正在使用的版本] <?php header("Content-type:text/html;charset=utf-8" ...
- 搭建一个简单struts2框架的登陆
第一步:下载struts2对应的jar包,可以到struts官网下载:http://struts.apache.org/download.cgi#struts252 出于学习的目的,可以把整个完整的压 ...
- Visual Studio常用快捷键
1. 代码自动对齐:CTRL+K+F 2. 撤销---使用组合键“Ctrl+Z”进行撤销操作 3. 反撤销---使用组合键“Ctrl+Y”进行反撤销操作 4. 使用组合键“Ctrl+J”或者使用组合键 ...