LinkedList为什么增删快、查询慢
- ArrayList:长于随机访问元素,中间插入和移除元素比较慢,在插入时,必须创建空间并将它的所有引用向前移动,这会随着ArrayList的尺寸增加而产生高昂的代价,底层由数组支持。
- LinkedList:通过代价较低的在List中间进行插入和删除操作,只需要链接新的元素,而不必修改列表中剩余的元素,无论列表尺寸如何变化,其代价大致相同,提供了优化的顺序访问,随机访问相对较慢,特性较ArrayList更大,而且还添加了可以使其作为栈、队列或双端队列的方法,底层由双向链表实现。
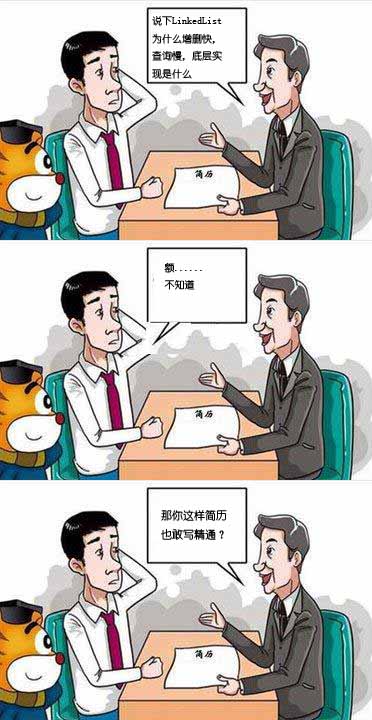
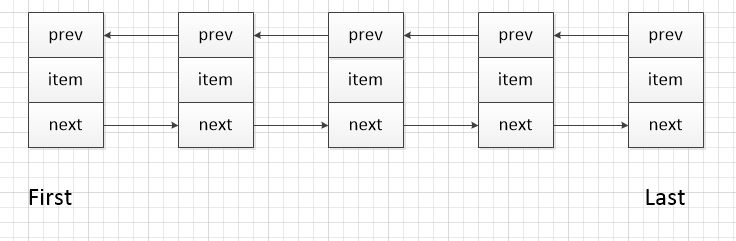
- //关于Node构造函数
- private static class Node<E> {
- E item;
- Node<E> next;
- Node<E> prev;
- Node(Node<E> prev, E element, Node<E> next) {
- this.item = element;
- this.next = next;
- this.prev = prev;
- }
- }
- //transient 保证以下几个属性不被序列化
- /**
- * The number of times this list has been <i>structurally modified</i>.
- * 该字段表示list结构上被修改的次数。结构上的修改指的是那些改变了list的长度
- * 大小或者使得遍历过程中产生不正确的结果的其它方式。
- *
- */
- protected transient int modCount = 0;
- transient int size = 0;
- /**
- * Pointer to first node.
- * Invariant: (first == null && last == null) ||
- * (first.prev == null && first.item != null)
- */
- transient Node<E> first;
- /**
- * Pointer to last node.
- * Invariant: (first == null && last == null) ||
- * (last.next == null && last.item != null)
- */
- transient Node<E> last;
- public boolean add(E e) {
- linkLast(e);
- return true;
- }
- /**
- * Links e as last element.
- */
- void linkLast(E e) {
- final Node<E> l = last;
- final Node<E> newNode = new Node<>(l, e, null);
- last = newNode;
- if (l == null)
- first = newNode;
- else
- l.next = newNode;
- size++;
- modCount++;
- }
- 获得最后一个节点last作为当前节点l
- 用当前节点l、添加参数e、null创建一个新的Node对象
- 将新创建的Node对象链接到最后节点,也就是last
- 如果当前的LinkedList为空,那么添加的node就是first,也是last
- 当前LinkedList的size+1,表示结构改变次数的对象modCount+1
- public boolean remove(Object o) {
- if (o == null) {
- for (Node<E> x = first; x != null; x = x.next) {
- if (x.item == null) {
- unlink(x);
- return true;
- }
- }
- } else {
- for (Node<E> x = first; x != null; x = x.next) {
- if (o.equals(x.item)) {
- unlink(x);
- return true;
- }
- }
- }
- return false;
- }
LinkedList的删除中,会根据传递进来的参数进行null判断,因为在LinkedList的元素移除中,null和非null的处理不一样,对于nul使用==去判断是否匹配,对于非null使用.equals(Object o)去判断,至于==和equals的区别,读者自行百度。
- E unlink(Node<E> x) {
- // assert x != null;
- final E element = x.item;
- final Node<E> next = x.next;
- final Node<E> prev = x.prev;
- if (prev == null) {
- first = next;
- } else {
- prev.next = next;
- x.prev = null;
- }
- if (next == null) {
- last = prev;
- } else {
- next.prev = prev;
- x.next = null;
- }
- x.item = null;
- size--;
- modCount++;
- return element;
- }
- 如果前一个节点prev为null,即第一个节点元素,则链表first = x下一个节点;
- 如果前一个节点prev不为null,即不是第一个节点元素,则将当前节点的next赋值给prev.next,x.prev置为null,也就是当前节点x的prev和next和当前链表中的其他元素不存在任何联系;
- 如果下一个节点next为null,即为最后一个元素,则链表last = x前一个节点;
- 如果下一个节点next不为null,即不为最后一个元素,则将当前节点的prev赋值给next.prev,同样当前节点x的prev和next和当前链表中的其他元素不存在任何联系;
- public E get(int index) {
- checkElementIndex(index);
- return node(index).item;
- }
- private void checkElementIndex(int index) {
- if (!isElementIndex(index))
- throw new IndexOutOfBoundsException(outOfBoundsMsg(index));
- }
- private boolean isElementIndex(int index) {
- return index >= 0 && index < size;
- }
- Node<E> node(int index) {
- // assert isElementIndex(index);
- if (index < (size >> 1)) {
- Node<E> x = first;
- for (int i = 0; i < index; i++)
- x = x.next;
- return x;
- } else {
- Node<E> x = last;
- for (int i = size - 1; i > index; i--)
- x = x.prev;
- return x;
- }
- }
- 根据传入的index去判断是否为LinkedList中的元素,判断逻辑为index是否在0和size之间,如果在则调用node(index)方法,否则抛出IndexOutOfBoundsException;
- 调用node(index)方法,将size右移1位,即size/2,判断传入的size在LinkedList的前半部分还是后半部分
- 如果在前半部分,即index < size/2,则从fisrt节点开始遍历匹配
- 如果在后半部分,即index > size/2,则从last节点开始遍历匹配
LinkedList为什么增删快、查询慢的更多相关文章
- 集合之LinkedList源码分析
转载请注明出处:http://www.cnblogs.com/qm-article/p/8903893.html 一.介绍 在介绍该源码之前,先来了解一下链表,接触过数据结构的都知道,有种结构叫链表, ...
- 数据结构-List接口-LinkedList类-Set接口-HashSet类-Collection总结
一.数据结构:4种--<需补充> 1.堆栈结构: 特点:LIFO(后进先出);栈的入口/出口都在顶端位置;压栈就是存元素/弹栈就是取元素; 代表类:Stack; 其 ...
- ArrayList和LinkedList的共同点和区别
ArrayList和LinkedList的相同点和不同点 共同点:都是单列集合中List接口的实现类.存取有序,有索引,可重复 不同点: 1.底层实现不同: ArrayList底层实现是数组,Link ...
- List-ApI及详解
1.API : add(Object o) remove(Object o) clear() indexOf(Object o) get(int i) size() iterator() isEmpt ...
- javaList容器中容易忽略的知识点
在集合类框架中,List是使用比较多的一种 List |---Arraylist 内部维护的是一个数组,查找快增删慢 |---LinkedList 底层是链表,增删快查询慢. |---Vctor线程安 ...
- java_List集合及其实现类
第一章:List集合_List接口介绍 1).特点 1).有序的: 2).可以存储重复元素: 3).可以通过索引访问: List<String> list = new Arra ...
- java基础-day17
第06天 集合 今日内容介绍 u 集合&迭代器 u 增强for & 泛型 u 常见数据结构 u List子体系 第1章 集合&迭代器 1.1 集合体系结构 1.1 ...
- BAT面试必备——Java 集合类
本文首发于我的个人博客:尾尾部落 1. Iterator接口 Iterator接口,这是一个用于遍历集合中元素的接口,主要包含hashNext(),next(),remove()三种方法.它的一个子接 ...
- 集合之四:List接口
查阅API,看List的介绍.有序的 collection(也称为序列).此接口的用户可以对列表中每个元素的插入位置进行精确地控制.用户可以根据元素的整数索引(在列表中的位置)访问元素,并搜索列表中的 ...
随机推荐
- 网络流--最大流--Dinic模板矩阵版(当前弧优化+非当前弧优化)
//非当前弧优化版 #include <iostream> #include <cstdio> #include <math.h> #include <cst ...
- Spring Cloud学习 之 Spring Cloud Ribbon(负载均衡器源码分析)
文章目录 AbstractLoadBalancer: BaseLoadBalancer: DynamicServerListLoadBalancer: ServerList: ServerListUp ...
- OpenCV Error: Unspecified Error(The Function is not implemented)
Ubuntu 或者 Debian 系统显示窗口的时候遇到了这个问题 error: (-2:Unspecified error) The function is not implemented. Reb ...
- Centos最小安装
网络配置 虚拟机安装使用的NAT模式 #enoXXX 为自己的网卡名称, 使用ip addr 可以查看 将/etc/sysconfig/network-scripts/ifcfg-enoXXX中的ON ...
- transition完成事件
当transition事件完成时调用函数(移动端导航的动画消失效果). <!doctype html> <html> <head> <meta charset ...
- OpenCV开发笔记(五十六):红胖子8分钟带你深入了解多种图形拟合逼近轮廓(图文并茂+浅显易懂+程序源码)
若该文为原创文章,未经允许不得转载原博主博客地址:https://blog.csdn.net/qq21497936原博主博客导航:https://blog.csdn.net/qq21497936/ar ...
- CCF ISBN
题目原文 问题描述(题目链接登陆账号有问题,要从这个链接登陆,然后点击“模拟考试”,进去找本题目) 试题编号: 201312-2 试题名称: ISBN号码 时间限制: 1.0s 内存限制: 256 ...
- Redis的几种集群方式分析
一 单机版 分析: 无论多少用户,都访问这一台服务器 .服务器一旦挂了,所有用户都无法访问.风险很大,一般不会有人使用. 二 主从模式(master/slaver) 分析: 主从模式中, 无论多少用户 ...
- docker+headless+robotframework+jenkins实现web自动化持续集成
在Docker环境使headless实现web自动化持续集成 一.制作镜像 原则:自动化测试基于基础制作镜像 命令:docker run --privileged --name=$1 --net=ho ...
- MySQL++:Liunx - MySQL 主从复制
目标:搭建两台MySQL服务器,一台作为主服务器,一台作为从服务器,实现主从复制 环境:虚拟机 主数据库:192.168.211.101 从数据库:192.168.211.102 MySQL 安装可参 ...