POJ 1459:Power Network 能源网络
Time Limit: 2000MS | Memory Limit: 32768K | |
Total Submissions: 25414 | Accepted: 13247 |
Description
0 <= c(u) <= min(s(u),cmax(u)) of power, and may deliver an amount d(u)=s(u)+p(u)-c(u) of power. The following restrictions apply: c(u)=0 for any power station, p(u)=0 for any consumer, and p(u)=c(u)=0 for any dispatcher. There is at most one power
transport line (u,v) from a node u to a node v in the net; it transports an amount 0 <= l(u,v) <= lmax(u,v) of power delivered by u to v. Let Con=Σuc(u) be the power consumed in the net. The problem is to compute the maximum value of
Con.
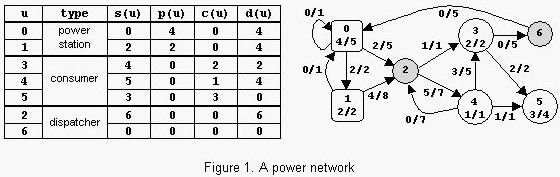
An example is in figure 1. The label x/y of power station u shows that p(u)=x and pmax(u)=y. The label x/y of consumer u shows that c(u)=x and cmax(u)=y. The label x/y of power transport line (u,v) shows that l(u,v)%3��J�ub>max(u,v)=y.
The power consumed is Con=6. Notice that there are other possible states of the network but the value of Con cannot exceed 6.
Input
(u,v)z, where u and v are node identifiers (starting from 0) and 0 <= z <= 1000 is the value of lmax(u,v). Follow np doublets (u)z, where u is the identifier of a power station and 0 <= z <= 10000 is the value of pmax(u). The data set
ends with nc doublets (u)z, where u is the identifier of a consumer and 0 <= z <= 10000 is the value of cmax(u). All input numbers are integers. Except the (u,v)z triplets and the (u)z doublets, which do not contain white spaces, white spaces can
occur freely in input. Input data terminate with an end of file and are correct.
Output
Sample Input
2 1 1 2 (0,1)20 (1,0)10 (0)15 (1)20
7 2 3 13 (0,0)1 (0,1)2 (0,2)5 (1,0)1 (1,2)8 (2,3)1 (2,4)7
(3,5)2 (3,6)5 (4,2)7 (4,3)5 (4,5)1 (6,0)5
(0)5 (1)2 (3)2 (4)1 (5)4
Sample Output
15
6
Hint
data set encodes the network from figure 1.
在能源网络中,有一些是发电站,有一些是转发站,有一些是消耗站。给了网络中边的容量,以及哪些是发电站,能发出多少电。哪些是消耗站,消耗多少站。问在能源网络中,最大流是多少。
建立一个超级源点,将超级源点与各个发电站相连,边得容量是发电站发的电。
建立一个超级汇点,将超级汇点与各个消耗站相连,边的容量是消耗站小号的电。
求添加了这两个点之后的网络流的最大值。
代码:
#include <iostream>
#include <algorithm>
#include <cmath>
#include <vector>
#include <string>
#include <queue>
#include <cstring>
#pragma warning(disable:4996)
using namespace std; const int sum = 200;
const int INF = 99999999;
int cap[sum][sum],flow[sum][sum],a[sum],p[sum]; int n,np,nc,m; void Edmonds_Karp()
{
int u,t,result=0;
queue <int> s;
while(s.size())s.pop(); while(1)
{
memset(a,0,sizeof(a));
memset(p,0,sizeof(p)); a[0]=INF;
s.push(0); while(s.size())
{
u=s.front();
s.pop(); for(t=0;t<=n+1;t++)
{
if(!a[t]&&flow[u][t]<cap[u][t])
{
s.push(t);
p[t]=u;
a[t]=min(a[u],cap[u][t]-flow[u][t]);//要和之前的那个点,逐一比较,到M时就是整个路径的最小残量
}
}
}
if(a[n+1]==0)
break;
result += a[n+1]; for(u=n+1;u!=0;u=p[u])
{
flow[p[u]][u] += a[n+1];
flow[u][p[u]] -= a[n+1];
}
}
cout<<result<<endl;
} int main()
{
int i,u,v,value; while(scanf("%d%d%d%d",&n,&np,&nc,&m)==4)
{
memset(cap,0,sizeof(cap));
memset(flow,0,sizeof(flow)); for(i=1;i<=m;i++)
{
scanf(" (%d,%d)%d",&u,&v,&value);
cap[u+1][v+1] += value;
}
for(i=1;i<=np;i++)
{
scanf(" (%d)%d",&u,&value);
cap[0][u+1] += value;
}
for(i=1;i<=nc;i++)
{
scanf(" (%d)%d",&v,&value);
cap[v+1][n+1] += value;
}
Edmonds_Karp();
}
return 0;
}
版权声明:本文为博主原创文章,未经博主允许不得转载。
POJ 1459:Power Network 能源网络的更多相关文章
- POJ 1459 Power Network / HIT 1228 Power Network / UVAlive 2760 Power Network / ZOJ 1734 Power Network / FZU 1161 (网络流,最大流)
POJ 1459 Power Network / HIT 1228 Power Network / UVAlive 2760 Power Network / ZOJ 1734 Power Networ ...
- poj 1459 Power Network
题目连接 http://poj.org/problem?id=1459 Power Network Description A power network consists of nodes (pow ...
- poj 1459 Power Network : 最大网络流 dinic算法实现
点击打开链接 Power Network Time Limit: 2000MS Memory Limit: 32768K Total Submissions: 20903 Accepted: ...
- poj 1459 Power Network【建立超级源点,超级汇点】
Power Network Time Limit: 2000MS Memory Limit: 32768K Total Submissions: 25514 Accepted: 13287 D ...
- POJ 1459 Power Network(网络流 最大流 多起点,多汇点)
Power Network Time Limit: 2000MS Memory Limit: 32768K Total Submissions: 22987 Accepted: 12039 D ...
- 2018.07.06 POJ 1459 Power Network(多源多汇最大流)
Power Network Time Limit: 2000MS Memory Limit: 32768K Description A power network consists of nodes ...
- 网络流--最大流--POJ 1459 Power Network
#include<cstdio> #include<cstring> #include<algorithm> #include<queue> #incl ...
- POJ 1459 Power Network(网络最大流,dinic算法模板题)
题意:给出n,np,nc,m,n为节点数,np为发电站数,nc为用电厂数,m为边的个数. 接下来给出m个数据(u,v)z,表示w(u,v)允许传输的最大电力为z:np个数据(u)z,表示发电 ...
- poj 1459 Power Network(增广路)
题目:http://poj.org/problem?id=1459 题意:有一些发电站,消耗用户和中间线路,求最大流.. 加一个源点,再加一个汇点.. 其实,过程还是不大理解.. #include & ...
随机推荐
- 深入 理解char * ,char ** ,char a[ ] ,char *a[] 的区别
转自:https://blog.csdn.net/liusicheng2008_liu/article/details/80412586 1 数组的本质 数组是多个元素的集合,在内存中分布在地址相连的 ...
- 程序员如何 10 分钟用 Python 画出蒙娜丽莎?
之前看到过很多头条,说哪国某人坚持了多少年自学使用excel画画,效果十分惊艳.对于他们的耐心我十分敬佩. 但是作为一个程序员,自然也得挑战一下自己. 这种需求,我们十分钟就可以完成! 基本思路 ...
- 012.CI4框架CodeIgniter, 加载并调用自己的Libraries库
01. 在Libraries目录创建一个Mylib文件,内容是一个简单的类 <?php namespace App\Controllers; class Home extends BaseCon ...
- 点亮一个LED之参数传递规则
1 说明 实验平台: JZ2440 CPU: S3C2440 2 ARM-THUMB Procedure Call Standard(ATPCS: ARM-Thumb过程调用标准) 图1 ...
- DStream-04 Window函数的原理和源码
DStream 中 window 函数有两种,一种是普通 WindowedDStream,另外一种是针对 window聚合 优化的 ReducedWindowedDStream. Demo objec ...
- 【pwnable.kr】 unlink
pwnable.kr 第一阶段的最后一题! 这道题目就是堆溢出的经典利用题目,不过是把堆块的分配与释放操作用C++重新写了一遍,可参考<C和C++安全编码一书>//不是广告 #includ ...
- 108-PHP类成员protected和private成员属性不能被查看数值
<?php class mao{ //定义猫类 public $age; //定义多个成员属性 protected $weight; private $color; } $mao1=new ma ...
- 3.2Adding custom methods to mappers(在映射器中添加自定义方法)
3.2Adding custom methods to mappers(在映射器中添加自定义方法) 有些情况下,我们需要实现一些MapStruct无法直接自动生成的复杂类型间映射.一种方式是复用其他已 ...
- 干货分享:Academic Essay写作套路详解
你想过如何中立的表达自己吗?大概只有10%不到的同学,会真正重视这个细节.但很多留学生能顺利写完作文已经不容易,还要注意什么中立不中立的.我知道这个标准,对许多同学有些过分,但很残酷的告诉你,这的确是 ...
- Python安装bs4
- 需要将pip源设置为国内源,阿里源.豆瓣源.网易源等 - windows (1)打开文件资源管理器(文件夹地址栏中) (2)地址栏上面输入 %appdata% (3)在这里面新建一个文件夹 pip ...