2018湘潭邀请赛 AFK题解 其他待补...
A.HDU6276:Easy h-index
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 1181 Accepted Submission(s): 415
http://acm.hdu.edu.cn/downloads/2018ccpc_hn.pdf
The h-index of an author is the largest h where he has at least h papers with citations not less than h.
Bobo has published many papers.
Given a0,a1,a2,…,an which means Bobo has published ai papers with citations exactly i, find the h-index of Bobo.
The first line of each test case contains an integer n.
The second line contains (n+1) integers a0,a1,…,an.
## Constraint
* 1≤n≤2⋅105
* 0≤ai≤109
* The sum of n does not exceed 250,000.
#include <stdio.h>
#include <string.h>
#include <string>
#include <map>
#include <queue>
#include <iostream>
#include <algorithm>
#define ll long long
#define exp 1e-8
using namespace std;
const int N = 2e5 +;
const int INF = 2e9+;
const int mod = 1e9+;
ll a[N];
int main() {
int n;
while (~scanf("%d",&n)){
for (int i = ; i <= n; i++) {
scanf("%lld",&a[i]);
}
ll ans = ;
for (int i =n; i >= ; i--){
ans += a[i];
if (ans >= i){
printf("%d\n",i);
break ;
}
}
}
return ;
}
F.HDU6281:Sorting
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 1482 Accepted Submission(s): 407
He would like to find the lexicographically smallest permutation p1,p2,…,pn of 1,2,…,n such that for i∈{2,3,…,n} it holds that
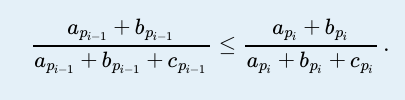
The first line of each test case contains an integer n.
The i-th of the following n lines contains 3 integers ai, bi and ci.
DO NOT print trailing spaces.
## Constraint
* 1≤n≤103
* 1≤ai,bi,ci≤2×109
* The sum of n does not exceed 104.
#include <stdio.h>
#include <string.h>
#include <string>
#include <map>
#include <queue>
#include <iostream>
#include <algorithm>
#define ll long long
#define exp 1e-8
using namespace std;
const int N = 1e3 +;
const int INF = 2e9+;
const int mod = 1e9+;
struct node{
int id;
ll a,b,c;
}p[N];
bool cmp(node x,node y){
ll ans1 = (x.a+x.b)*y.c;
ll ans2 = (y.a+y.b)*x.c;
if (ans1 == ans2){
return x.id<y.id;
}
return ans1 < ans2;
}
int main() {
int n;
while (~scanf("%d",&n)){
for (int i = ; i < n; i++) {
p[i].id = i + ;
scanf("%lld%lld%lld",&p[i].a,&p[i].b,&p[i].c);
}
sort(p,p+n,cmp);
for (int i = ; i < n-; i++){
printf("%d ",p[i].id);
}
printf("%d\n",p[n-].id);
}
return ;
}
K.HDU6286:
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 908 Accepted Submission(s): 457
Each test case contains four integers a,b,c,d.
## Constraint
* 1≤a≤b≤109,1≤c≤d≤109
* The number of tests cases does not exceed 104.
#include <stdio.h>
#include <string.h>
#include <string>
#include <map>
#include <queue>
#include <iostream>
#include <algorithm>
#define ll long long
#define ull unsigned ll
#define exp 1e-8
using namespace std;
const int N = 1e3 +;
const int INF = 2e9+;
const int mod = 1e9+;
int main() {
ll a,b,c,d;
while (cin>>a>>b>>c>>d){
ll ans = ;
ll x1 =b/ - (a-)/; //a~b中2018的倍数 × all
ans += x1 * (d-c+);
ll x2 = b/-(a-)/ - x1; //a~b中偶数(除了x1)的个数 × 1009倍数
ans += x2 * (d/ - (c-)/);
ll x3 = b/ - (a-)/ - x1;//a~b中1009的奇数倍 × 偶数
ans += x3 * (d/ - (c-)/);
ll x4 = (b-a+) - (b/-(a-)/) - x3;//a~b中奇数的个数 × 2018倍数
ans += x4 * (d/ - (c-)/);
//printf("%lld %lld %lld %lld \n",x1,x2,x3,x4);
cout << ans << '\n';
}
return ;
}
2018湘潭邀请赛 AFK题解 其他待补...的更多相关文章
- 2018湘潭邀请赛C题(主席树+二分)
题目地址:https://www.icpc.camp/contests/6CP5W4knRaIRgU 比赛的时候知道这题是用主席树+二分,可是当时没有学主席树,就连有模板都不敢套,因为代码实在是太长了 ...
- 湘潭邀请赛+蓝桥国赛总结暨ACM退役总结
湘潭邀请赛已经过去三个星期,蓝桥也在上个星期结束,今天也是时候写一下总结了,这应该也是我的退役总结了~ --------------------------------湘潭邀请赛----------- ...
- XTU 1264 - Partial Sum - [2017湘潭邀请赛E题(江苏省赛)]
2017江苏省赛的E题,当时在场上看错了题目没做出来,现在补一下…… 题目链接:http://202.197.224.59/OnlineJudge2/index.php/Problem/read/id ...
- 2018宁夏邀请赛 L Continuous Intervals(单调栈+线段树)
2018宁夏邀请赛 L Continuous Intervals(单调栈+线段树) 传送门:https://nanti.jisuanke.com/t/41296 题意: 给一个数列A 问在数列A中有多 ...
- 1250 Super Fast Fourier Transform(湘潭邀请赛 暴力 思维)
湘潭邀请赛的一题,名字叫"超级FFT"最终暴力就行,还是思维不够灵活,要吸取教训. 由于每组数据总量只有1e5这个级别,和不超过1e6,故先预处理再暴力即可. #include&l ...
- 湘潭邀请赛 Hamiltonian Path
湘潭邀请赛的C题,哈密顿路径,边为有向且给定的所有边起点小于终点,怎么感觉是脑筋急转弯? 以后一定要牢记思维活跃一点,把复杂的事情尽量简单化而不是简单的事情复杂化. #include<cstdi ...
- 湘潭邀请赛 2018 I Longest Increasing Subsequence
题意: 给出一个长度为n的序列,序列中包含0.定义f(i)为把所有0变成i之后的Lis长度,求∑ni=1i⋅f(i). 题解: 设不考虑0的Lis长度为L,那么对于每个f(i),值为L或L+1. 预处 ...
- 湘潭邀请赛 2018 E From Tree to Graph
题意: 给出一棵树以及m,a,b,x0,y0.之后加m条边{(x1,LCA(x1,y1)),(x2,LCA(x2,y2))...(xm,LCA(xm,ym))}.定义z = f(0)^f(1)^... ...
- 湘潭邀请赛 2018 D Circular Coloring
题意: 给一个环,环上有n+m个点.给n个点染成B,m个点染成W.求所有染色情况的每段长度乘积之和. 题解: 染成B的段数和染成W的段数是一样的(因为是环). 第一段是可以移动的,例如BBWWW移动为 ...
随机推荐
- Git的提交与查看差异
本文转载于:http://blog.csdn.net/crylearner/article/details/7685158 代码提交 代码提交一般有五个步骤: 1.查看目前代码的修改状态 2.查看代码 ...
- PHP 试题(1)
1.__FILE__表示什么意思?(5分)文件的完整路径和文件名.如果用在包含文件中,则返回包含文件名.自 PHP 4.0.2 起,__FILE__ 总是包含一个绝对路径,而在此之前的版本有时会包含一 ...
- ubuntu 运行级别initlevel
Linux 系统任何时候都运行在一个指定的运行级上,并且不同的运行级的程序和服务都不同,所要完成的工作和要达到的目的都不同,系统可以在这些运行级之间进行切换,以完成不同的工作.Ubuntu 的系统运行 ...
- tensorflow学习笔记(四十五):sess.run(tf.global_variables_initializer()) 做了什么?
当我们训练自己的神经网络的时候,无一例外的就是都会加上一句 sess.run(tf.global_variables_initializer()) ,这行代码的官方解释是 初始化模型的参数.那么,它到 ...
- CentOS7在防火墙与端口上的操作
https://jingyan.baidu.com/article/cdddd41cb3bf6c53cb00e1ac.html CentOS7在安装软件包或类库的时候,常常会因为防火墙的拦截和端口未开 ...
- 2018-6-29-PTA-6-2-多项式求值
title author date CreateTime categories PTA 6-2 多项式求值 lindexi 2018-06-29 15:24:28 +0800 2018-6-14 22 ...
- ASP.NET MVC4.0+EF+LINQ+bui+bootstrap+网站+角色权限管理系统(4)
接下来就是菜单管理了,菜单分为两部分,一部分是菜单管理,另一部分是左边的树形菜单 数据库添加菜单表Menus USE [MVCSystem] GO /****** Object: Table [dbo ...
- 土旦:移动端 Vue+Vant 的Uploader 实现 :上传、压缩、旋转图片
面向百度开发 html <van-uploader :after-read="onRead" accept="image/*"> <img s ...
- C#面试题整理(带答案)
1.维护数据库的完整性.一致性.你喜欢用触发器还是自写业务逻辑?为什么? 答:尽可能用约束(包括CHECK.主键.唯一键.外键.非空字段)实现,这种方式的效率最好:其次用触发器,这种方式可以保证无论何 ...
- kube-batch 解析
kube-batch https://github.com/kubernetes-sigs/kube-batch 一. 做什么的? 官方介绍: A batch scheduler of kuberne ...