POJ1179Polygon(DP)
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 4456 | Accepted: 1856 |
Description
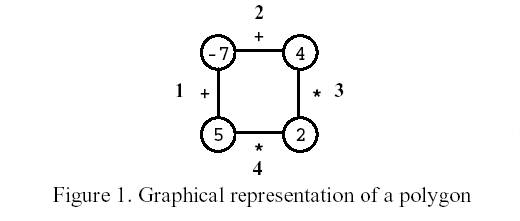
On the first move, one of the edges is removed. Subsequent moves involve the following steps:
�pick an edge E and the two vertices V1 and V2 that are linked by E; and
�replace them by a new vertex, labelled with the result of performing the operation indicated in E on the labels of V1 and V2.
The game ends when there are no more edges, and its score is the label of the single vertex remaining.
Consider the polygon of Figure 1. The player started by removing
edge 3. After that, the player picked edge 1, then edge 4, and, finally,
edge 2. The score is 0.
Write a program that, given a polygon, computes the highest possible
score and lists all the edges that, if removed on the first move, can
lead to a game with that score.
Input
program is to read from standard input. The input describes a polygon
with N vertices. It contains two lines. On the first line is the number
N. The second line contains the labels of edges 1, ..., N, interleaved
with the vertices' labels (first that of the vertex between edges 1 and
2, then that of the vertex between edges 2 and 3, and so on, until that
of the vertex between edges N and 1), all separated by one space. An
edge label is either the letter t (representing +) or the letter x
(representing *).
3 <= N <= 50
For any sequence of moves, vertex labels are in the range [-32768,32767].
Output
program is to write to standard output. On the first line your program
must write the highest score one can get for the input polygon. On the
second line it must write the list of all edges that, if removed on the
first move, can lead to a game with that score. Edges must be written in
increasing order, separated by one space.
Sample Input
4
t -7 t 4 x 2 x 5
Sample Output
33
1 2 题目的意思就是给n个数,n个两两数之间的运算符(只有+和*)问首先去掉哪个运算符号之后可以让其他的数按照一定的方法计算后结果最大。
其实结题思路还是比较好想到的,枚举(枚举去掉的符号)+DP(记忆化搜索)就可以做到。但这里有一个天坑,就是负负得正,所以不能单一的枚举最大值,而要同时DP最小值。
计算最大值:
加法 max(i,j) = max(i,k)+max(k,j);
乘法 max(i,j) = MAX(max(i,k)*max(k,j),max(i,k)*min(k,j),max(k,j)*min(i,k),min(i,k)*min(k,j));(i=<k<=j)
计算最小值:
加法 min(i,j) = min(i,k)+min(k,j);
乘法 min(i,j) = MIN(max(i,k)*max(k,j),min(i,k)*min(k,j),max(k,j)*min(i,k),min(i,k)*min(k,j));(i=<k<=j)
见代码:
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <iostream>
#include <stack>
#include <set>
#include <queue>
#define MAX(a,b) (a) > (b)? (a):(b)
#define MIN(a,b) (a) < (b)? (a):(b)
#define mem(a) memset(a,0,sizeof(a))
#define INF 1000000007
#define MAXN 20005
using namespace std; bool op[];
int num[],dp_max[], dp_min[], n;
bool vis_max[],vis_min[];
int DP_MIN(int i,int j);
int DP_MAX(int i,int j); int DP_MAX(int i,int j)//DP求区间最大值
{
int u = i*+j;
if(vis_max[u])return dp_max[u];
vis_max[u]=;
if(j-i <= )
{
if(j==i)return dp_max[u]=num[i-];
if(!op[i])return dp_max[u]=num[i-]+num[i];
else return dp_max[u]=num[i-]*num[i];
}
dp_max[u] = -INF;
for(int k=i;k<j;k++)
{
int l=DP_MIN(i,k);
int r=DP_MIN(k+,j);
int ll=DP_MAX(i,k);
int rr=DP_MAX(k+,j);
if(!op[k])dp_max[u] = MAX(dp_max[u], ll+rr);
else dp_max[u] = MAX(dp_max[u], MAX(ll*rr,MAX(l*r,MAX(l*rr,r*ll))));
}
return dp_max[u];
} int DP_MIN(int i,int j)//DP求区间最小值
{
int u = i*+j;
if(vis_min[u])return dp_min[u];
vis_min[u]=;
if(j-i <= )
{
if(j==i)return dp_min[u]=num[i-];
if(!op[i])return dp_min[u]=num[i-]+num[i];
else return dp_min[u]=num[i-]*num[i];
}
dp_min[u] = INF;
for(int k=i;k<j;k++)
{
int l=DP_MIN(i,k);
int r=DP_MIN(k+,j);
int ll=DP_MAX(i,k);
int rr=DP_MAX(k+,j);
if(!op[k])dp_min[u] = MIN(dp_min[u], l+r);
else dp_min[u] = MIN(dp_min[u], MIN(ll*rr,MIN(l*r,MIN(l*rr,r*ll))));
}
return dp_min[u];
} int main()
{
while(~scanf("%d%*c",&n))
{
mem(op);mem(dp_max);
mem(num);mem(vis_min);
mem(vis_max);
int max=-INF,i;
char ch;
for(i=;i<n;i++)
{
scanf("%c %d%*c",&ch,&num[i]);
op[i]=op[i+n]=(ch=='x');
num[i+n]=num[i];
}
for(i=;i<n;i++)
{
max=MAX(max,DP_MAX(i+,i+n));
}
printf("%d\n",max);
int ok=;
for(i=;i<n;i++)
{
if(DP_MAX(i+,i+n) == max)
{
if(ok){printf("%d",i+);ok=;}
else printf(" %d",i+);
}
}
printf("\n");
}
return ;
}
POJ1179Polygon(DP)的更多相关文章
- POJ1179Polygon(区间dp)
啊~~ 被dp摁在地上摩擦的人 今天做了一道区间dp的题(POJ1179Polygon) 题目: Polygon Time Limit: 1000MS Memory Limit: 10000K T ...
- BZOJ 1911: [Apio2010]特别行动队 [斜率优化DP]
1911: [Apio2010]特别行动队 Time Limit: 4 Sec Memory Limit: 64 MBSubmit: 4142 Solved: 1964[Submit][Statu ...
- 2013 Asia Changsha Regional Contest---Josephina and RPG(DP)
题目链接 http://acm.hdu.edu.cn/showproblem.php?pid=4800 Problem Description A role-playing game (RPG and ...
- AEAI DP V3.7.0 发布,开源综合应用开发平台
1 升级说明 AEAI DP 3.7版本是AEAI DP一个里程碑版本,基于JDK1.7开发,在本版本中新增支持Rest服务开发机制(默认支持WebService服务开发机制),且支持WS服务.RS ...
- AEAI DP V3.6.0 升级说明,开源综合应用开发平台
AEAI DP综合应用开发平台是一款扩展开发工具,专门用于开发MIS类的Java Web应用,本次发版的AEAI DP_v3.6.0版本为AEAI DP _v3.5.0版本的升级版本,该产品现已开源并 ...
- BZOJ 1597: [Usaco2008 Mar]土地购买 [斜率优化DP]
1597: [Usaco2008 Mar]土地购买 Time Limit: 10 Sec Memory Limit: 162 MBSubmit: 4026 Solved: 1473[Submit] ...
- [斜率优化DP]【学习笔记】【更新中】
参考资料: 1.元旦集训的课件已经很好了 http://files.cnblogs.com/files/candy99/dp.pdf 2.http://www.cnblogs.com/MashiroS ...
- BZOJ 1010: [HNOI2008]玩具装箱toy [DP 斜率优化]
1010: [HNOI2008]玩具装箱toy Time Limit: 1 Sec Memory Limit: 162 MBSubmit: 9812 Solved: 3978[Submit][St ...
- px、dp和sp,这些单位有什么区别?
DP 这个是最常用但也最难理解的尺寸单位.它与“像素密度”密切相关,所以 首先我们解释一下什么是像素密度.假设有一部手机,屏幕的物理尺寸为1.5英寸x2英寸,屏幕分辨率为240x320,则我们可以计算 ...
随机推荐
- bzoj1564
嗯,这是一道简单题 注意二叉搜索树的子树中序一定是连续的 又因为取值修改是任意的并且修改代价与权值无关 不难想到把权值离散化,然后按找数据值排序,然后dp f[i][j][w]表示从i~j的节点构成一 ...
- Qt之QTableView添加复选框(QAbstractTableModel)
简述 使用QTableView,经常会遇到复选框,要实现一个好的复选框,除了常规的功能外,还应注意以下几点: 三态:不选/半选/全选 自定义风格(样式) 下面我们介绍一下常见的实现方式: 编辑委托. ...
- wdcp系统升级mysql5.7.11
1.下载解压 下载地址为:http://dev.mysql.com/get/Downloads/MySQL-5.7/mysql-5.7.12-linux-glibc2.5-x86_64.tar.gz ...
- OK - A byte of python - 读书笔记
看这本书的目的:再熟悉基本概念. 大部分都是知道,但是需要 明确 出来的 概念. - 欢迎吐槽错误,非常感谢. <A byte of python> - THIS 1. 组织行 - 形式: ...
- jvm内部现成运行
hi,all 最近抽时间把JVM运行过程中产生的一些线程进行了整理,主要是围绕着我们系统jstack生成的文件为参照依据. 前段时间因为系统代码问题,造成性能瓶颈,于是就dump了一份stack出来 ...
- 【转】第一次使用Android Studio时你应该知道的一切配置(三):gradle项目构建
原文网址:http://www.cnblogs.com/smyhvae/p/4456420.html [声明] 欢迎转载,但请保留文章原始出处→_→ 生命壹号:http://www.cnblogs.c ...
- Android-根据ImageView的大小来压缩Bitmap,避免OOM
本文转自:http://www.cnblogs.com/tianzhijiexian/p/4254110.html Bitmap是引起OOM的罪魁祸首之一,当我们从网络上下载图片的时候无法知道网络图片 ...
- 【转】使用NetBeans和Eclipse开发PHP应用程序
[51CTO独家特稿]各位用户如果单独看NetBeans和Eclipse的市场占有率,你可能会认为使用其中任何一种IDE开发PHP应用程序都没有 问题,例如: 1.NetBeans:一款开源的集成开发 ...
- hdu 2473 Junk-Mail Filter(并查集_虚节点)2008 Asia Regional Hangzhou
感觉有些难的题,刚开始就想到了设立虚节点,但是实现总是出错,因为每次设立了虚节点之后,无法将原节点和虚节点分开,导致虚节点根本无意义. 以上纯属废话,可以忽略…… 题意—— 给定n个点(0, 1, 2 ...
- 我们究竟什么时候可以使用Ehcache缓存
一.Ehcache是什么 EhCache是Hibernate的二级缓存技术之一,可以把查询出来的数据存储在内存或者磁盘,节省下次同样查询语句再次查询数据库,大幅减轻数据库压力. 二.Ehcache的使 ...